C++ (CPP) can be utilized in Android development to create performance-oriented applications by leveraging the Android Native Development Kit (NDK).
Here’s a simple example of a C++ function that can be called from Java in an Android app:
#include <jni.h>
extern "C"
JNIEXPORT jstring JNICALL
Java_com_example_myapp_MainActivity_stringFromJNI(JNIEnv *env, jobject) {
return env->NewStringUTF("Hello from C++");
}
Understanding the Basics
What is C++?
C++ is a powerful programming language that is widely used for system/software development and game programming due to its high-performance capabilities. It supports both procedural and object-oriented programming, giving developers the flexibility to choose the best approach for their applications. Unlike Java, which is primarily designed for portability and ease of use, C++ provides more control over system resources and performance, making it ideal for performance-critical applications.
Why Use C++ in Android Development?
Using C++ in Android development offers several key advantages:
-
Performance Benefits: C++ generally offers superior performance compared to Java, particularly in computation-heavy applications such as games and real-time simulations. This can lead to smoother user experiences and faster processing times.
-
Reuse of Existing Code: If you have existing C or C++ libraries, you can reuse them in your Android applications, saving time and effort in development.
-
Cross-Platform Compatibility: C++ can be used to target multiple platforms, including iOS and Windows, allowing you to write code once and run it everywhere.
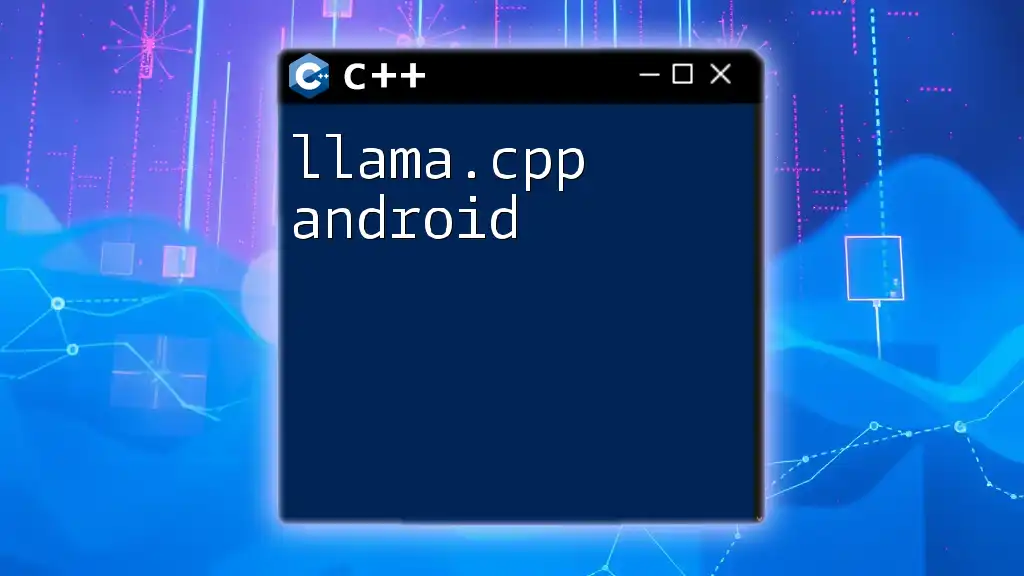
Setting Up Your Development Environment
Installing the Necessary Tools
To develop Android applications using C++, you'll need a suitable Integrated Development Environment (IDE). Android Studio is highly recommended as it provides built-in support for C++ and integrates seamlessly with the Android development ecosystem.
Setting Up the Native Development Kit (NDK)
The Native Development Kit (NDK) is a set of tools that allows you to use C and C++ code in Android applications.
To install the NDK:
- Open Android Studio and navigate to the SDK Manager.
- Find the NDK section and select the latest version.
- Click Apply to install it.
Once installed, you will need to configure your project to use the NDK. In your `build.gradle` file, you can specify the NDK version like this:
android {
...
externalNativeBuild {
cmake {
version "3.10.2"
}
}
}
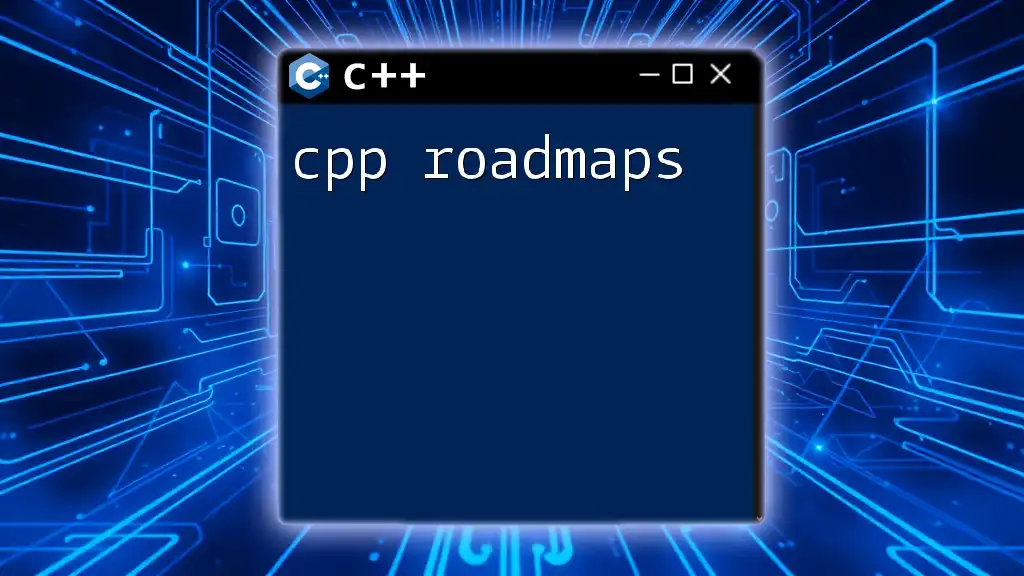
Building Your First C++ Android Application
Creating a New Android Project with C++
When starting a new project in Android Studio, select the option to include C++ support. This will automatically set up the necessary files and configurations for you.
Writing Your First C++ Code Snippet
Let’s get started by creating a simple C++ snippet that will display “Hello from C++”. The following code demonstrates how to use JNI (Java Native Interface) to achieve this:
#include <jni.h>
#include <string>
extern "C" JNIEXPORT jstring JNICALL
Java_com_example_myapp_MainActivity_stringFromJNI(JNIEnv* env, jobject /* this */) {
return env->NewStringUTF("Hello from C++");
}
In this code, we define a JNI function that can be called from your Java/Kotlin code. The function uses the `JNIEnv` pointer to create a new Java string that is returned to the caller.
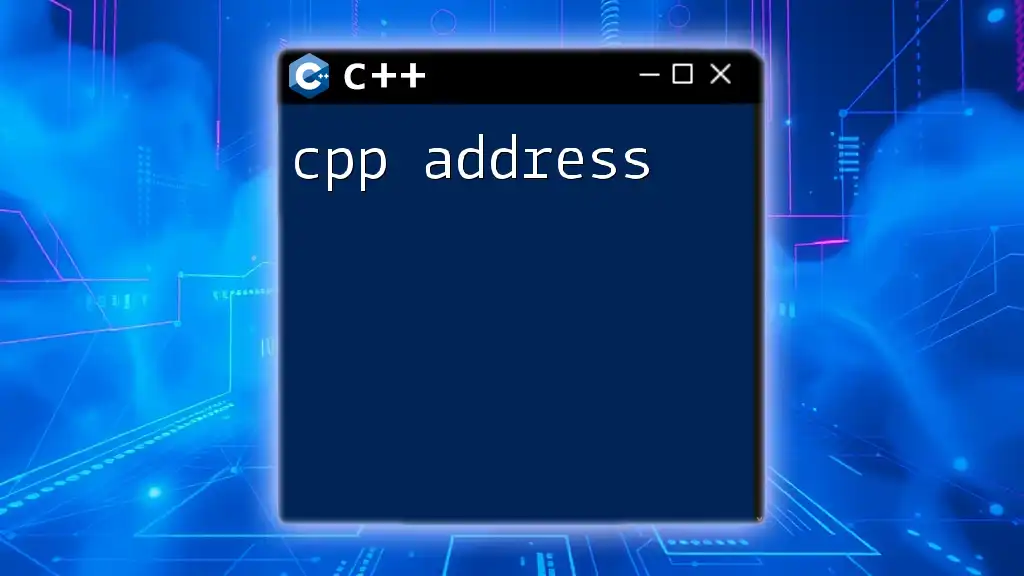
Calling C++ Functions from Java/Kotlin
Using JNI for Communication
Java Native Interface (JNI) is a crucial tool for Android development in C++. It allows Java code to call and be called by native applications and libraries written in C/C++. This interoperability is what enables you to utilize C++ functionalities seamlessly.
For example, to call the `stringFromJNI` function from your Java code, you would declare it as a native method:
public native String stringFromJNI();
This declaration lets Java know that this method is implemented in C++. You can then call this method from your activity, and it will return the string from the C++ function.
Example: Sending Data Between Java and C++
Passing parameters between Java and C++ is straightforward with JNI. Here’s another example demonstrating how to pass integer values to a C++ function and perform operations:
extern "C" JNIEXPORT jint JNICALL
Java_com_example_myapp_MainActivity_addNumbers(JNIEnv* env, jobject /* this */, jint a, jint b) {
return a + b;
}
In this function, `a` and `b` are integers passed from Java. The function returns their sum, which Java can then use.
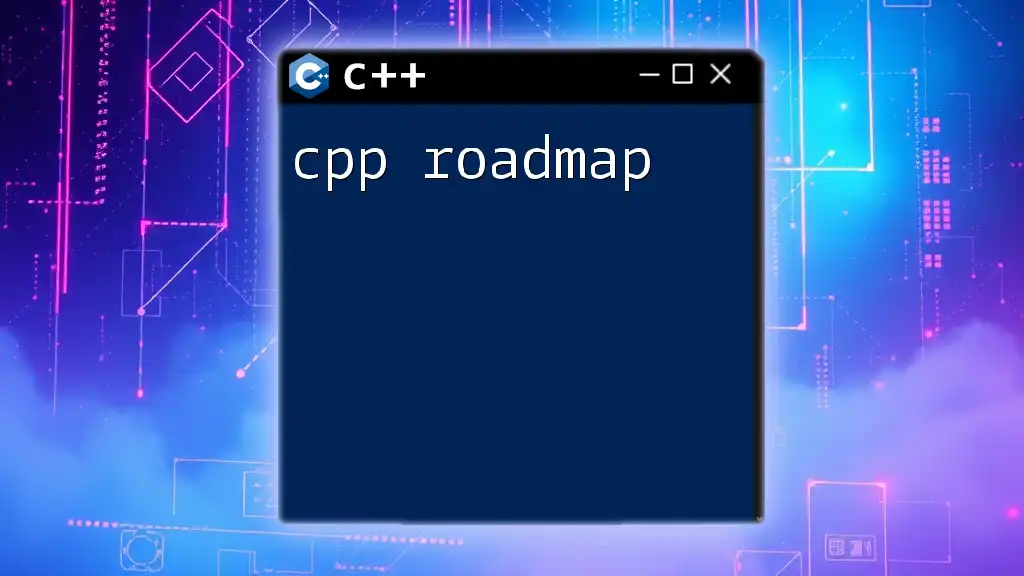
Advanced C++ Techniques for Android Development
Using C++ Libraries in Android Projects
To elevate your application’s functionality, you can incorporate existing C++ libraries like OpenCV for image processing or Boost for utilities. Including a library in your project requires minimal effort. Simply download or clone the library, include it in your project, and modify your `CMakeLists.txt` to link the library:
find_package(OpenCV REQUIRED)
target_link_libraries(native-lib ${OpenCV_LIBS})
Multithreading and Concurrency in C++
In mobile applications, especially games or real-time applications, effective multithreading is essential. C++ provides robust support for multithreading with its `std::thread` library. Here’s a simple example:
#include <thread>
void threadFunction() {
// Code to execute in the new thread
}
std::thread t1(threadFunction);
t1.join(); // Waits for the thread to finish
This example shows how to create and manage threads. Effective use of threads can improve the responsiveness of your app by running processes in parallel.
Performance Tuning C++ Code for Android
Optimizing C++ code for Android involves several strategies, including careful memory management, choosing efficient algorithms, and minimizing resource use. It is also critical to profile your app using the Android Profiler, allowing you to identify performance bottlenecks.
Debugging C++ code can be challenging due to the complexity of compiling native libraries. Use logging and breakpoints effectively to trace your code execution and catch issues early.
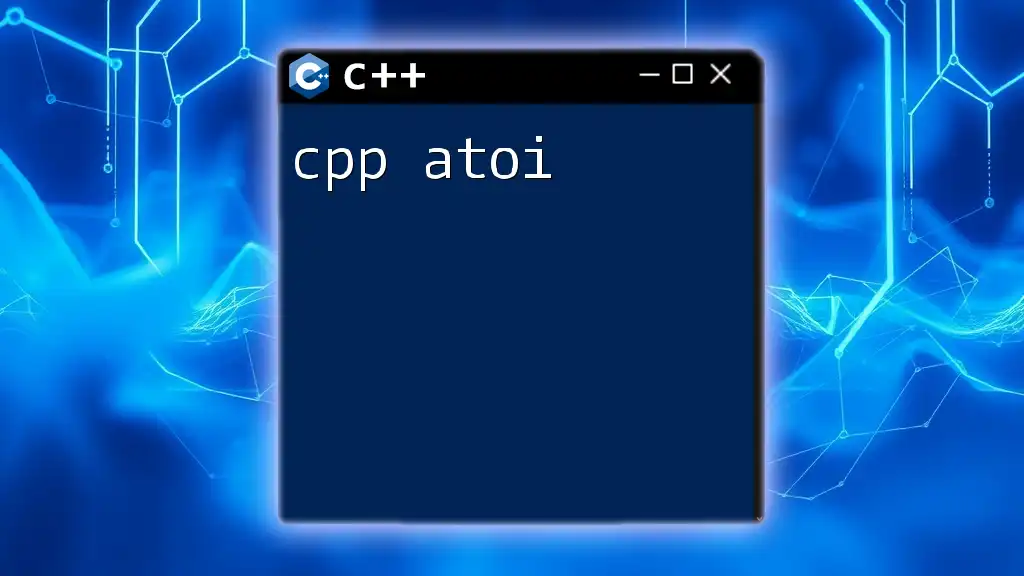
Common Issues and Troubleshooting
Debugging C++ Code on Android
Common issues developers face when implementing C++ in Android projects include JNI misconfigurations, memory access violations, and threading issues. Use tools like the Android Studio Debugger to step through your code and check for errors.
FAQs about C++ and Android Integration
-
Can I use C++ for all Android projects? While it's possible, it's often best to use C++ for performance-critical components and keep the majority of your app in Java or Kotlin.
-
Is learning C++ necessary for Android development? Not strictly, but understanding C++ can provide significant advantages in terms of performance and optimizing your applications.
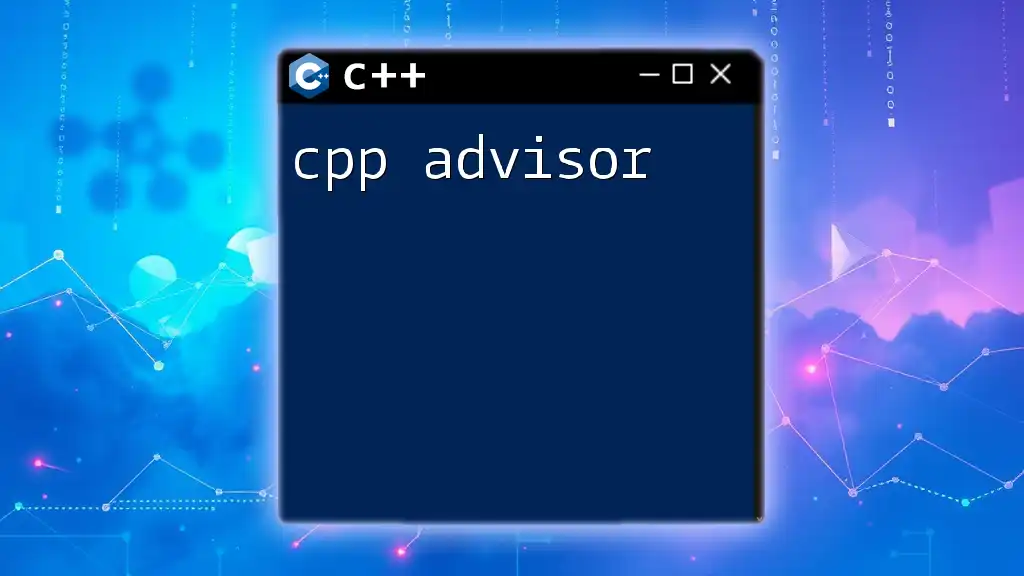
Conclusion
Using C++ in Android development can significantly enhance your application's performance and capabilities. By leveraging the power of C++, you can overcome many of the limitations associated with Java and create more responsive, efficient applications. As you dive deeper into C++ and Android, remember to continuously optimize and experiment with different techniques to make the most out of your development experience.
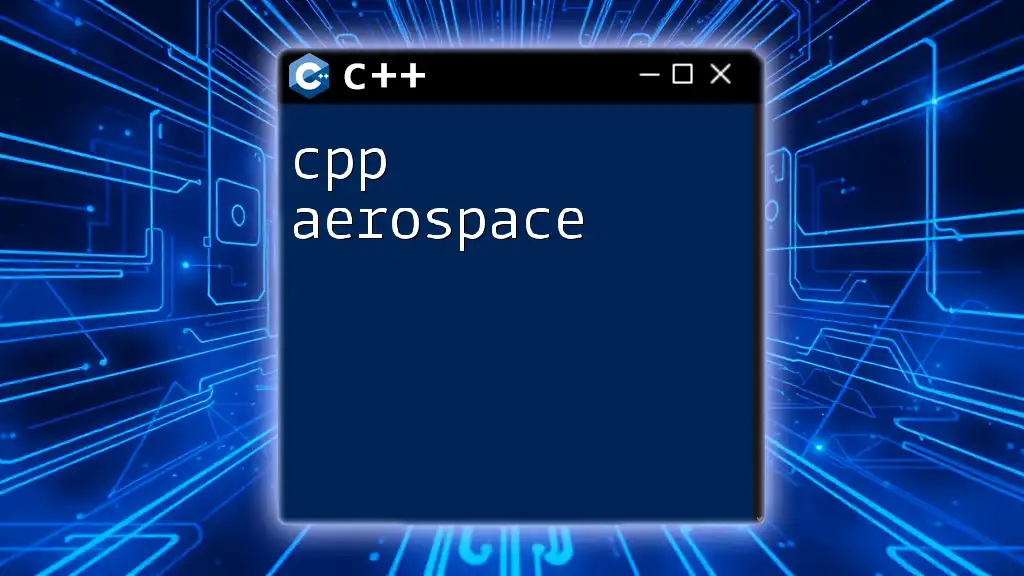
Additional Resources
For further learning, consider checking out the official Android documentation on the [Native Development Kit (NDK)](https://developer.android.com/ndk) and explore community forums for shared insights and knowledge. Books and online courses specifically focused on C++ and Android development can also provide additional depth to your understanding and capabilities.