The `atoi` function in C++ converts a string representation of an integer into its corresponding integer value.
#include <iostream>
#include <cstdlib> // For atoi
#include <string>
int main() {
std::string str = "1234";
int num = atoi(str.c_str());
std::cout << "The integer value is: " << num << std::endl;
return 0;
}
Understanding String Conversion
What is String Conversion?
String conversion refers to the process of converting data in the form of a string into another format, typically a numerical format like an integer. In programming, string conversion is fundamental, especially when dealing with data input from users, files, or network sources. The ability to convert strings to integers is crucial for mathematical operations, data storage, and more.
Common Methods for String Conversion in C++
In C++, there are several functions available for converting strings to integers. While `atoi` is one of the oldest methods, others like `stoi` and `strtol` have become more popular due to their enhanced error handling and support for various numerical bases. It is essential to choose the right function depending on the use case.
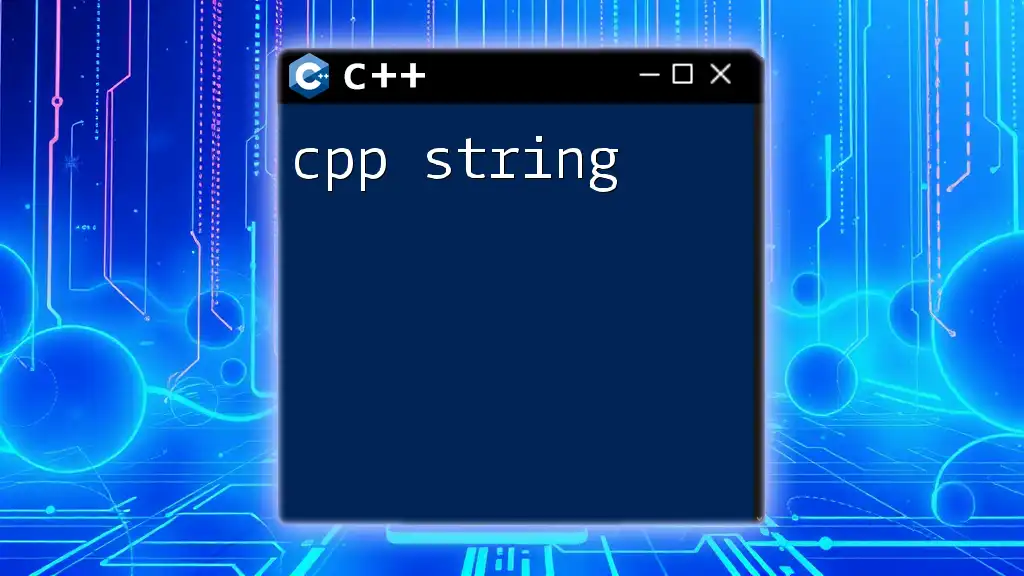
The `atoi` Function Explained
Overview of `atoi`
The `atoi` function, short for "ASCII to integer," is a standard library function used for converting a C-style string (character array) to an `int`. Its syntax is:
int atoi(const char *str);
This function scans the provided string for any leading whitespace characters, converts the subsequent characters into an integer until it hits a non-numeric character, and returns the result. If the conversion fails (for instance, when the string cannot be converted to an integer), `atoi` will return 0, which can sometimes lead to ambiguity in error handling.
Error Handling with `atoi`
One of the significant drawbacks of `atoi` is its lack of explicit error reporting. If the input string is non-numeric or out of the valid range of integers, `atoi` will simply return 0. This behavior can make it difficult to determine if the result is because of a valid conversion leading to 0 or because of an error. This limitation makes `atoi` less preferred in scenarios where reliable error detection is important.
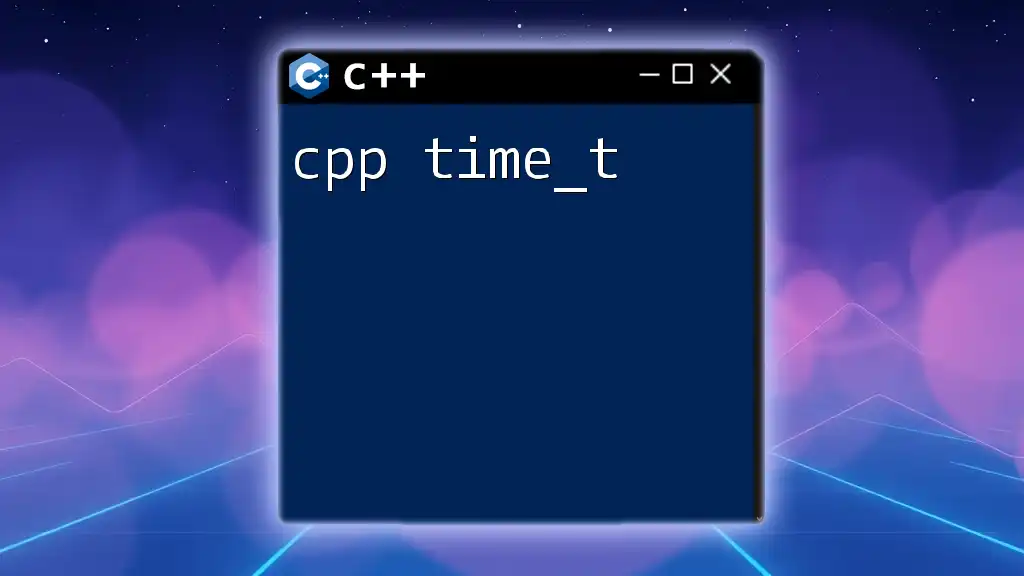
Working with `atoi`
Basic Usage of `atoi`
The most straightforward way to use `atoi` is to simply pass a string that represents an integer. Here’s a simple example:
#include <iostream>
#include <cstdlib> // Required for atoi
int main() {
const char* str = "12345";
int number = atoi(str);
std::cout << "The integer value is: " << number << std::endl;
return 0;
}
This code snippet declares a string representing the integer "12345", converts it using `atoi`, and then prints the output. The expected output will be:
The integer value is: 12345
Handling Edge Cases with `atoi`
Leading Whitespaces and Trailing Characters
`atoi` gracefully handles leading whitespaces, meaning that it will ignore these when converting a string. For example:
const char* str = " 6789abcd";
int number = atoi(str); // Output: 6789
Despite the trailing characters "abcd," `atoi` will return only the number parsed up to the first invalid character, resulting in 6789.
Strings that Don't Represent Valid Integers
If the string does not contain valid numeric characters, `atoi` will return 0. Consider the following example:
const char* strInvalid = "abc123";
int result = atoi(strInvalid); // Output: 0
In this case, because the string starts with non-numeric characters, the output will be 0. This behavior illustrates the ambiguity of error handling when using `atoi`.
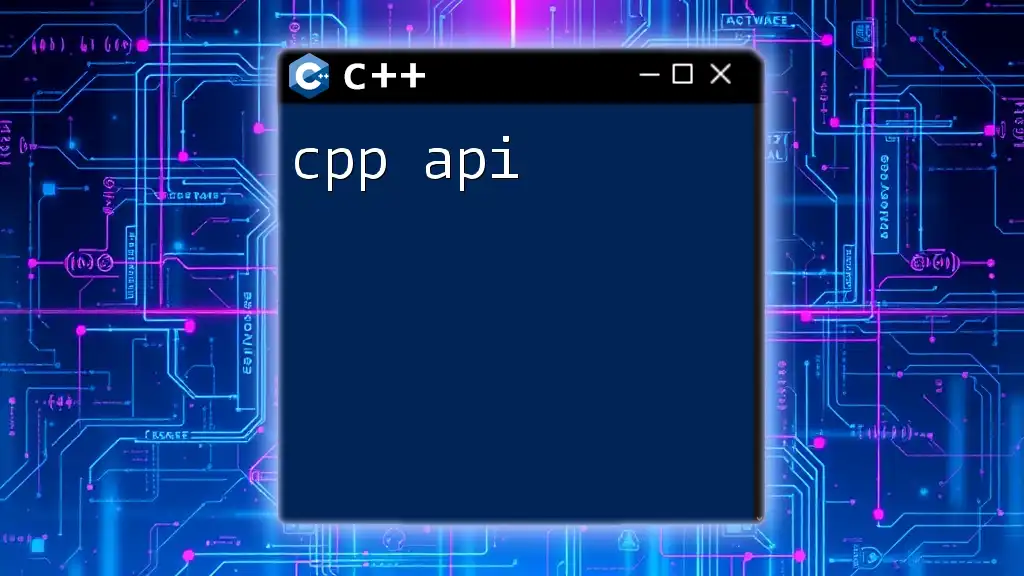
Alternatives to `atoi`
Using `stoi`
The `stoi` function, introduced in C++11, provides a modern alternative to `atoi`, offering improved error handling through exceptions. `stoi` syntax looks like this:
int stoi(const std::string &str, size_t *idx = 0, int base = 10);
Here’s an example showcasing its utility:
#include <iostream>
#include <string>
int main() {
std::string str = "12345";
try {
int number = std::stoi(str);
std::cout << "The integer value is: " << number << std::endl;
} catch (const std::invalid_argument& e) {
std::cout << "Invalid argument: " << e.what() << std::endl;
} catch (const std::out_of_range& e) {
std::cout << "Out of range: " << e.what() << std::endl;
}
return 0;
}
In this code snippet, if the string cannot be converted, a specific exception is thrown, giving you an opportunity to manage the error effectively.
When to Use `atoi` and When to Choose Alternatives
While `atoi` can still be used for its simplicity and speed in scenarios where you can guarantee valid input, `stoi` is generally preferred for its robust error handling. If your application requires rigorous input validation, `stoi` or other alternatives like `strtol` should be considered.
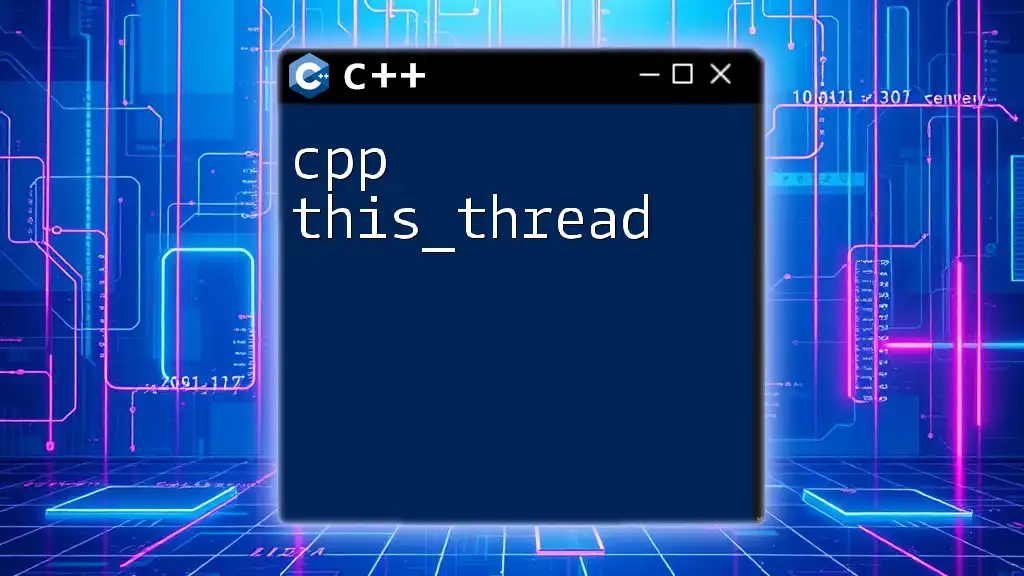
Best Practices for Using `atoi`
Always Validate Input
Before using `atoi`, it's crucial to validate the input. This can be done by checking the string’s contents before attempting to convert:
- Use regular expressions or utility functions to ensure that the input string is numeric before calling `atoi`.
Performance Considerations
In performance-sensitive applications, the choice of conversion function can be important. While `atoi` is generally very fast, the added overhead of exception handling in `stoi` is negligible in many real-world situations. However, always profile your code if performance is a concern.
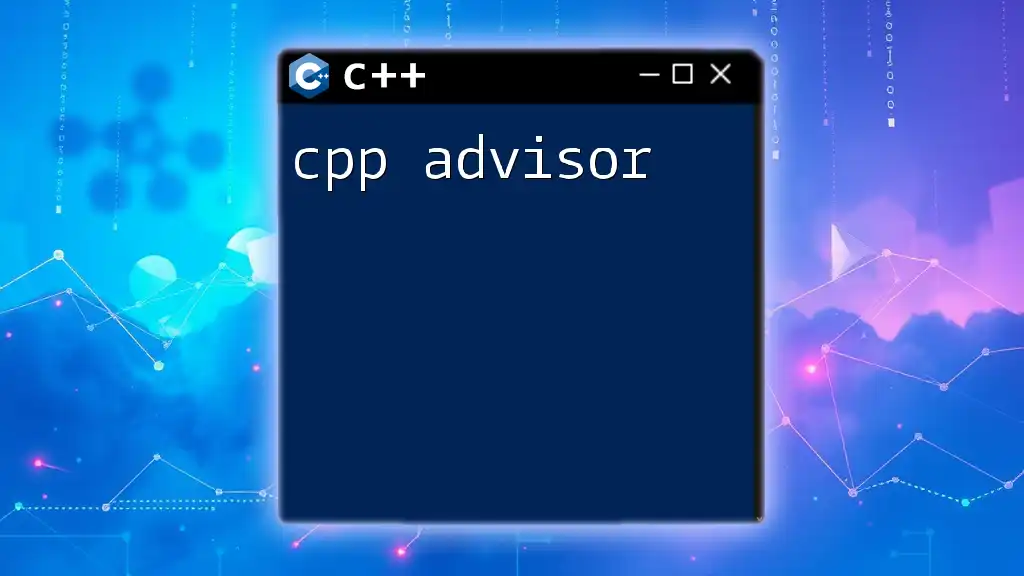
Conclusion
In this guide, we explored the `atoi` function in C++, covering its syntax, usage, error handling, and alternatives. While `atoi` remains a useful function for quick conversions, alternatives such as `stoi` offer enhanced error management and are generally recommended for newer codebases.
The world of string-to-integer conversion is vast, and understanding the tools available can significantly improve your coding practice. Engage with additional resources and practice using string conversion functions to become proficient in their usage!