To create a DLL (Dynamic Link Library) from C++ code, you define functions with `__declspec(dllexport)` and compile the code into a binary that can be used by other applications.
Here's an example of a simple C++ code snippet for creating a DLL:
// mylibrary.cpp
#include <iostream>
extern "C" {
__declspec(dllexport) void HelloWorld() {
std::cout << "Hello, World from the DLL!" << std::endl;
}
}
What is a DLL?
Dynamic Link Libraries (DLLs) are essential components of Windows software, allowing multiple applications to share functionality seamlessly. A DLL is a binary file containing code, data, and resources that can be used by various applications simultaneously. This not only helps in saving memory space but also ensures that updates to the library can be applied without needing to reinstall entire applications.
How DLLs Work
When a DLL is loaded into a process's memory space, its functions can be accessed, which allows for efficient code reuse. The calling application can refer to the DLL, invoking its functions as needed. This modularity simplifies software updates; if a library needs modification, only the DLL file needs to be altered without recompiling the entire application.
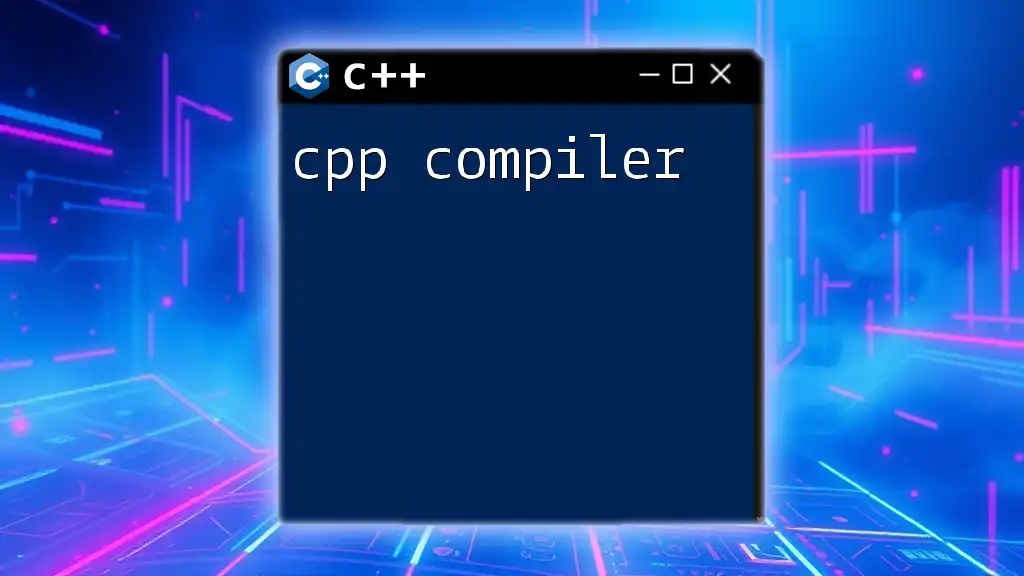
Creating a DLL with C++
Setting Up Your Environment
To start creating DLLs, you will need an appropriate C++ development environment. Visual Studio is highly recommended due to its robust tooling and intuitive interface. After installing, set up a new project specifically designed to create a DLL.
Writing Your First DLL
Step 1: Writing the Code
A simple DLL can be created by defining functions you want to expose. Consider the following example of a basic DLL that provides a greeting function:
// MyLibrary.cpp
#include <iostream>
#define EXPORT __declspec(dllexport)
extern "C" {
EXPORT void HelloWorld() {
std::cout << "Hello from DLL!" << std::endl;
}
}
In this example, we use `__declspec(dllexport)` to indicate which functions should be exposed outside the DLL. The `extern "C"` declaration prevents name mangling so that the function can be easily linked in other applications.
Step 2: Configuring the Project
Next, adjust your project’s settings to ensure it compiles as a DLL. In Visual Studio, access the project properties, navigate to Configuration Properties > General, and set Configuration Type to Dynamic Library (.dll). This ensures that when you build your project, it produces a .dll file instead of an executable.
Compiling the DLL
To compile your code, select the Build menu in Visual Studio and click Build Solution. If everything is set up correctly, you should see a `.dll` file generated in your project directory.
While compiling, you might encounter some errors. Common issues include forgetting to specify the correct configuration type or problems with your syntax. For any errors, double-check your code and make sure that all functions are correctly declared and defined.
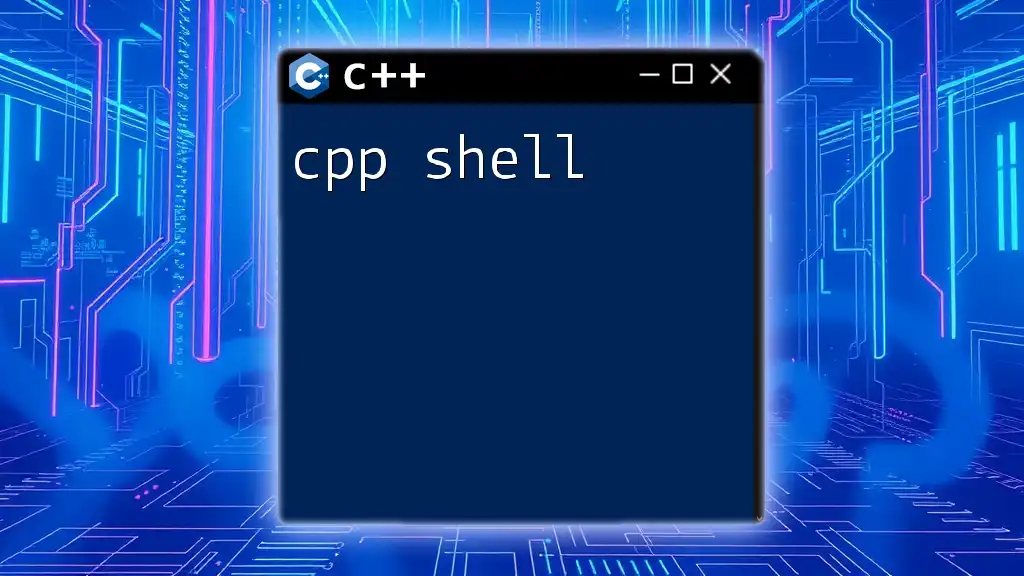
Using a DLL in a C++ Application
How to Link to Your DLL
To utilize the DLL you've created, you’ll need to link it to a new C++ application. Here’s how you can do that:
- Create a new project for your application in Visual Studio, selecting Console Application as the type.
- Include the DLL's header file in your main application file so that you can use its functions. For our previous example, the header file might look like:
// MyLibrary.h
#ifndef MY_LIBRARY_H
#define MY_LIBRARY_H
#ifdef MYLIBRARY_EXPORTS
#define EXPORT __declspec(dllexport)
#else
#define EXPORT __declspec(dllimport)
#endif
extern "C" {
EXPORT void HelloWorld();
}
#endif
- Then, in your `main` function, call the function from the DLL:
// MainApp.cpp
#include <iostream>
#include "MyLibrary.h" // Include the header file for the DLL
int main() {
HelloWorld(); // Calls the function from the DLL
return 0;
}
Managing Dependencies
When your application becomes more complex and requires multiple DLLs, it's essential to manage these dependencies effectively. Always ensure that the correct versions of each DLL are available in the System Path or the same directory as the executable to avoid runtime errors. Best practices include using versioning in your DLL names and organizing them in dedicated folders.
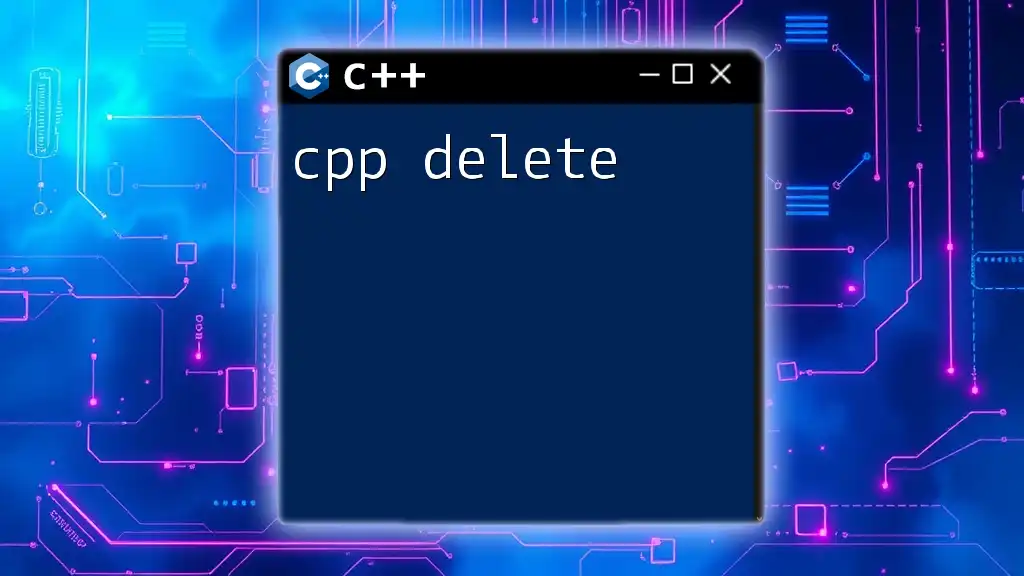
Common Use Cases for DLLs
Extending Functionality
DLLs offer a way to extend applications' functionality without recompilation. This is particularly useful for applications that need to add features over time or support plugins.
Code Reusability
One of the primary advantages of using DLLs is code reusability. Developers can encapsulate common functionalities in a DLL, allowing teams to share libraries across various projects.
Multilingual Applications
Developers can create language-specific DLLs for larger applications, facilitating the support of multiple programming languages. This approach improves maintainability by separating implementation details based on the language.
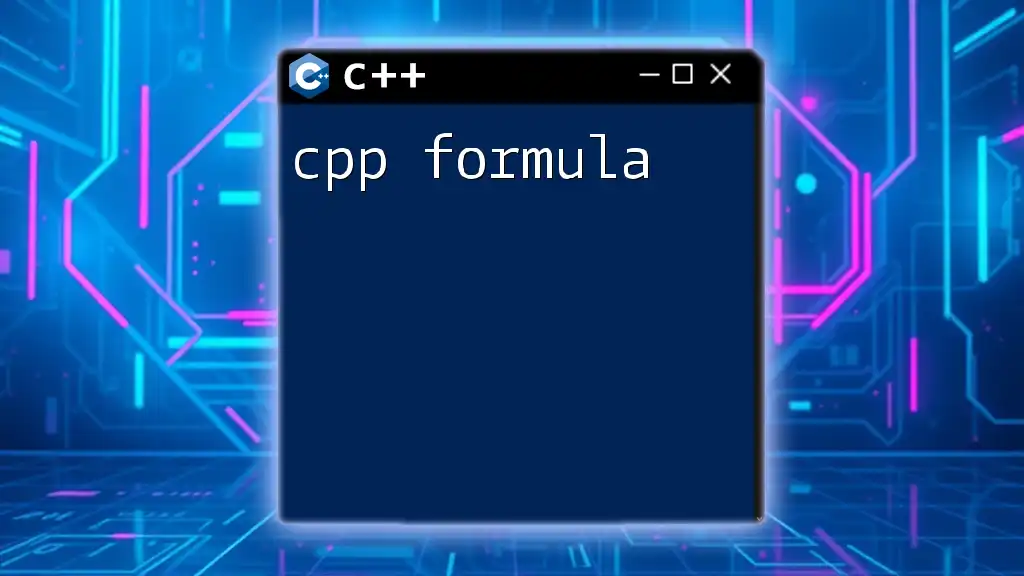
Best Practices for DLL Development
Design Considerations
Designing a DLL should focus on maintaining a clean interface and ensuring that exported functions are well documented. Use consistent naming conventions and retain backward compatibility whenever possible to avoid breaking existing code.
Error Handling in DLLs
Handle errors gracefully within your DLL functions. Make use of exceptions, logging, or error codes to provide feedback to users of your library. This aids in debugging and ensures that the calling application can manage errors effectively.
Security Concerns
Be cautious of DLL hijacking, where an attacker places a malicious DLL with the same name in the executable's directory. Protect applications by signing your DLLs and considering secure coding practices to mitigate vulnerabilities.
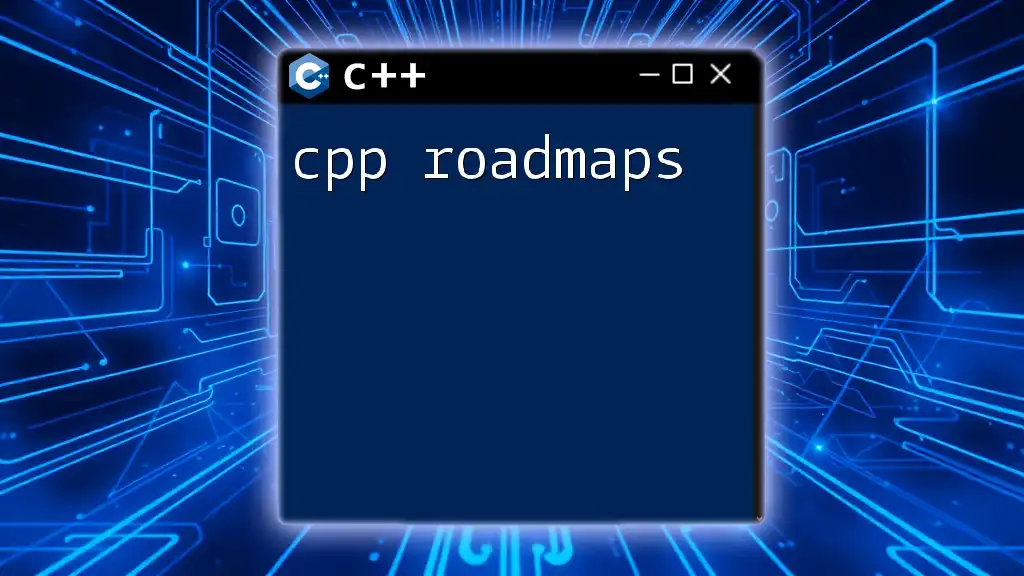
Debugging DLLs
Common Debugging Techniques
When debugging a DLL, process the same methodology as you would for any C++ application, but remember to ensure that the calling application is set to use the current build of the DLL. Utilize Visual Studio’s debugging tools to break and step through code, set breakpoints, and inspect variables.
Sample Debugging Scenario
Suppose you encounter an issue where the function isn't being recognized. Ensure that your DLL is being loaded correctly by checking the application’s references. Utilize debug output to trace problem areas, and confirm that the correct headers and libraries are linked.
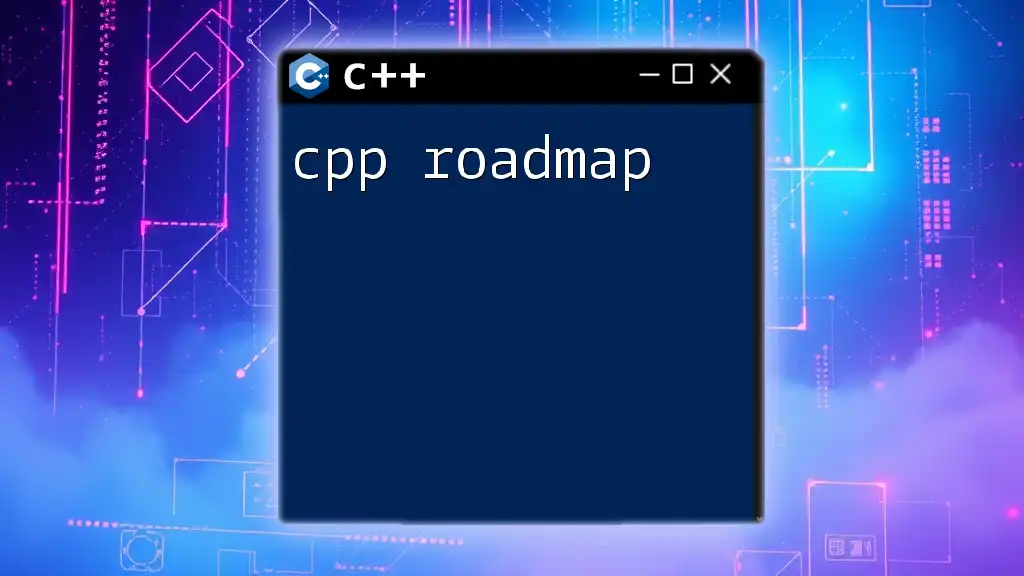
Conclusion
In summary, creating and using C++ DLLs enhances modularity in software development. By following the steps outlined in this guide, you can successfully create DLLs, utilize them in your applications, and take advantage of their many benefits. Remember to experiment and explore further, as working with DLLs presents an exciting opportunity to advance your C++ programming skills.
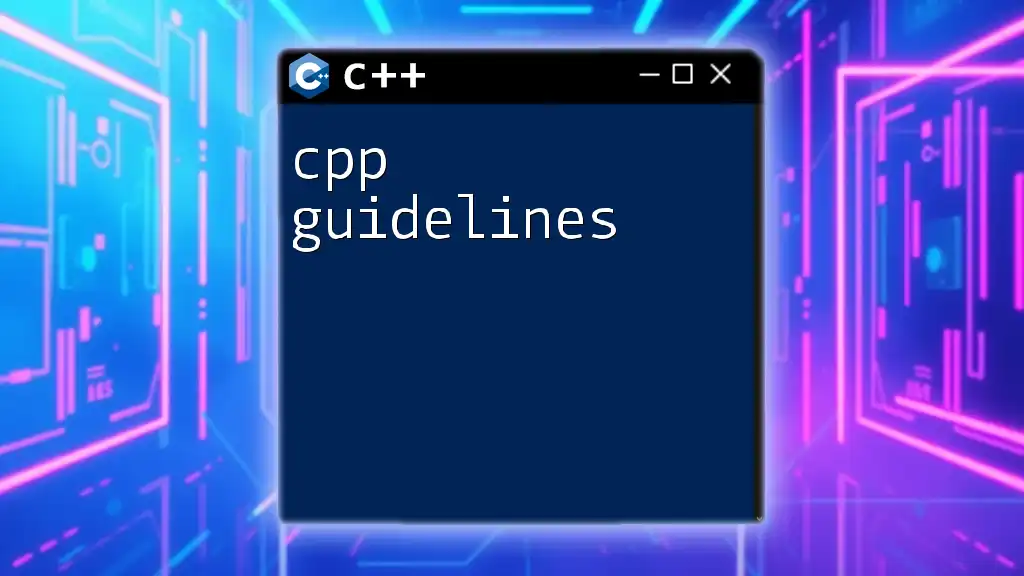
FAQs
What are the differences between static and dynamic linking?
Static linking includes all used library code into the executable at compile time, whereas dynamic linking connects libraries at runtime, which can save space and enable easier updates.
Can DLLs be used cross-platform?
While DLLs are specific to Windows, similar concepts exist in other operating systems, such as shared libraries in UNIX/Linux. Portability may require adjustments based on the target system.
How to handle memory allocation between a DLL and an application?
Always ensure that memory allocated in a DLL is freed by the same module. Mismanagement can lead to memory leaks or corruption. Use common memory management techniques through shared functions if necessary.