In C++, a boolean is a data type that can hold one of two values: true or false, often used in conditional statements and logic operations.
bool isEven(int number) {
return number % 2 == 0; // Returns true if the number is even, false otherwise
}
What is a Boolean in C++?
A Boolean is a fundamental data type in C++ that represents one of two states: true or false. This binary nature makes Booleans essential for controlling the flow of a program, particularly in decision-making processes.
The Role of Booleans in C++
In C++, Booleans play a crucial role in:
- Logical Operations: These are operations performed to yield true or false results. They help in evaluating conditions.
- Control Flow Statements: Booleans are vital for statements such as `if`, `while`, and `for`, allowing programmers to manage the execution of code based on certain conditions.
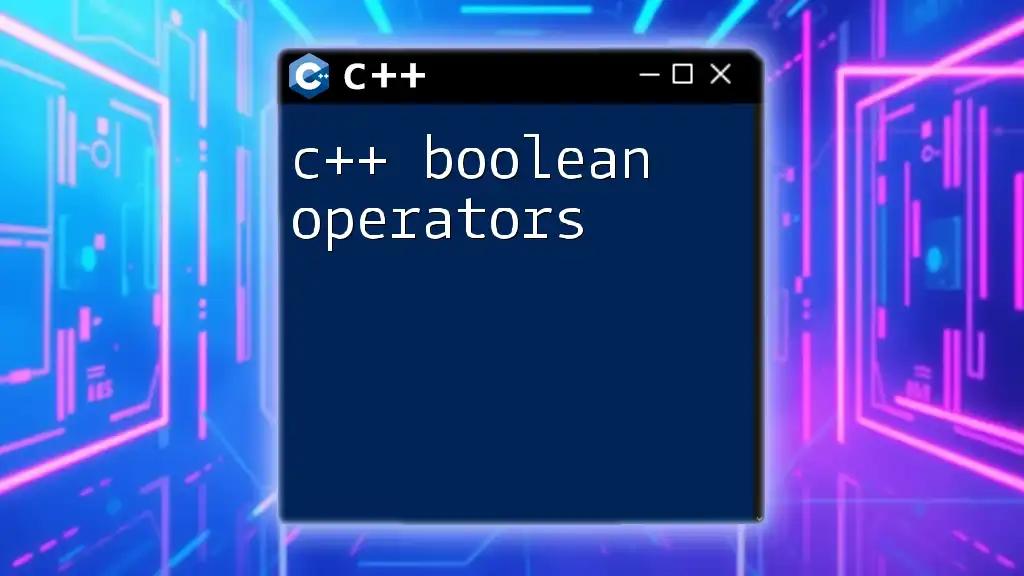
The Boolean Data Type in C++
Declaring Boolean Variables
To use Boolean values in your program, you need to declare Boolean variables. A Boolean variable can be initialized to either `true` or `false`.
Here’s an example:
bool isActive = true;
bool isComplete = false;
In this example, `isActive` is set to true, whereas `isComplete` is set to false. Proper initialization is essential as it defines the state of the variable right from its declaration.
Default Value of a Boolean
When declaring a Boolean variable without initializing it, the value is undefined, which can lead to unpredictable behavior in your program. It’s best practice to always initialize your Boolean variables to prevent any issues.
Consider the following code snippet:
bool myBool; // Undefined behavior; not recommended
Here, `myBool` is left uninitialized. Relying on its value would be dangerous, as it holds garbage data.
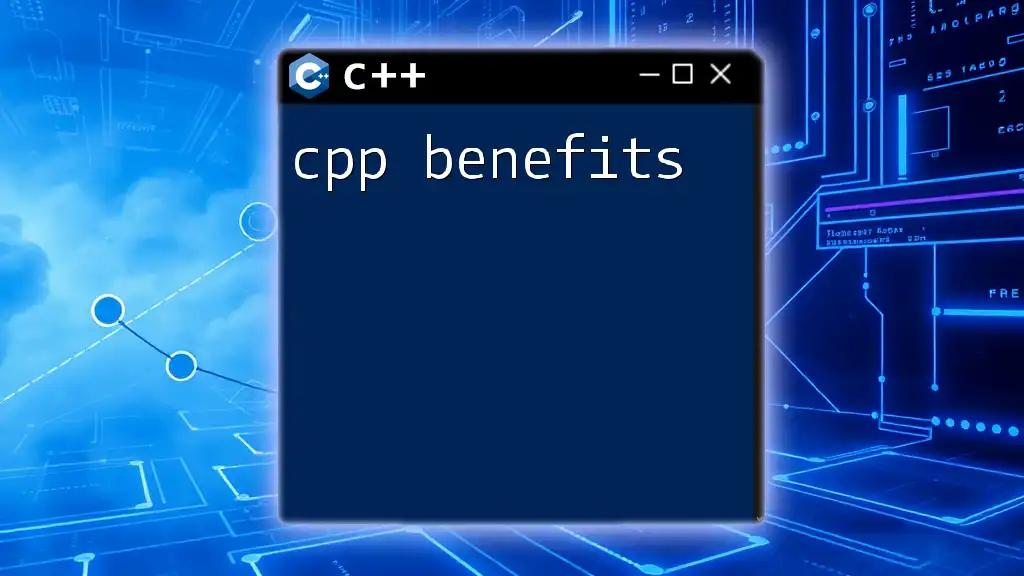
Operations with Booleans
Logical Operators
C++ provides several logical operators that operate on Boolean values:
- AND (`&&`): Returns true only if both operands are true.
- OR (`||`): Returns true if at least one of the operands is true.
- NOT (`!`): Inverts the value of the Boolean.
Here’s how they work in practice:
bool a = true;
bool b = false;
bool andResult = a && b; // false
bool orResult = a || b; // true
bool notResult = !a; // false
In this example, `andResult` evaluates to false because not both `a` and `b` are true, while `orResult` evaluates to true since `a` is true. The `notResult` inverts `a`, leading to false.
Comparison Operators
Comparison operators can also yield Boolean values. They are used to compare two values and determine their relational status:
- Equal to (`==`)
- Not equal to (`!=`)
- Greater than (`>`)
- Less than (`<`)
- Greater than or equal to (`>=`)
- Less than or equal to (`<=`)
For instance:
int x = 10;
int y = 20;
bool isEqual = (x == y); // false
bool isGreater = (x > y); // false
Here, `isEqual` evaluates to false because `x` and `y` are not equal, and `isGreater` also returns false since `x` is not greater than `y`.
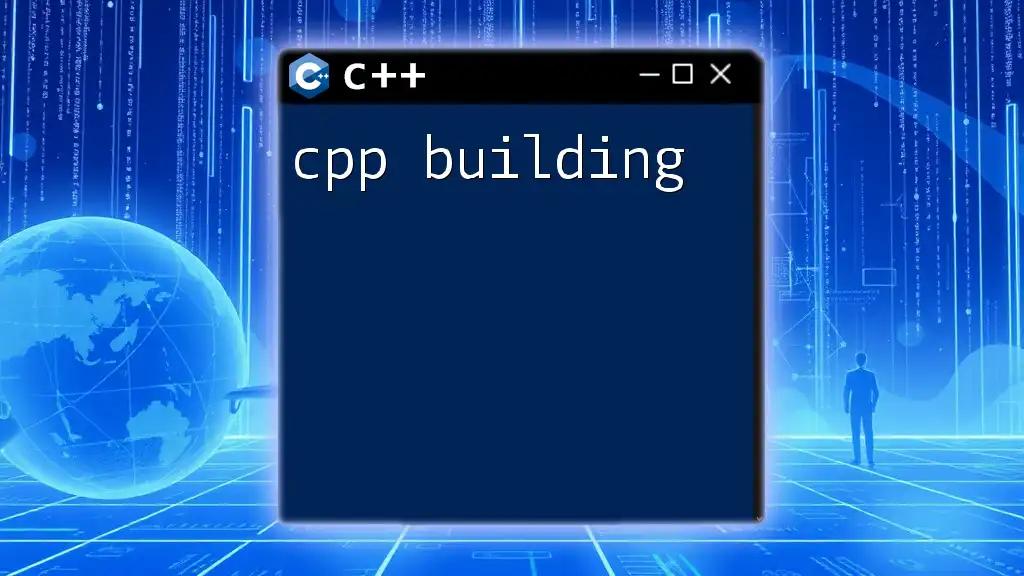
Common Use Cases for C++ Booleans
Control Flow Statements
Booleans are pivotal in control flow structures. For example, an `if` statement checks if a condition is true before executing a block of code:
if (isActive) {
cout << "The system is active." << endl;
}
In this snippet, the message is printed only when `isActive` is true. This showcases how conditions guide program execution.
Looping Constructs
Booleans also drive looping constructs such as `while` loops. The loop continues until the condition evaluates to false:
while (isComplete) {
// Perform actions
}
In this example, the loop will run as long as `isComplete` remains true. Once it changes to false, the loop will terminate.

Best Practices
Naming Conventions for Boolean Variables
Using clear and concise names for Boolean variables can significantly improve code readability. Opt for names that indicate a true/false condition. For instance, instead of naming a variable `x`, consider naming it `isConnected` to convey its purpose more clearly.
Avoiding Common Pitfalls
One common mistake with Boolean variables is in using assignment instead of comparison in conditional checks. For example:
// Potential pitfall
if (myBool = true) { // This assigns true, doesn't compare
// Incorrect logic
}
In this situation, rather than comparing `myBool` with `true`, it assigns `true` to `myBool`, leading to incorrect logic. Always use `==` for comparisons.

Conclusion
Understanding C++ Booleans is fundamental to effective programming. With their binary nature, they enable critical operations like logical operations and control flow. Keeping best practices in mind and avoiding common pitfalls can lead to clearer, more reliable code.
By practicing these concepts, you will be able to effectively leverage boolean logic in your C++ programming endeavors. Use this guide as a starting point to explore the powerful capabilities of Booleans in C++.