In "cpp building 4," we focus on leveraging advanced data structures such as vectors to efficiently manage collections of elements in C++.
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int number : numbers) {
std::cout << number << " ";
}
return 0;
}
Understanding C++ Building
What is C++ Building?
CPP building refers to the entire process of preparing a C++ source code to be executable, encompassing stages such as compilation and linking. It is an essential skill for anyone developing software in C++ because it ensures that the code is not only functional but also optimized for performance and resource management. In modern software development, understanding the intricacies of C++ building can dramatically improve the efficiency and reliability of your applications.
The Build Process Explained
The build process can be broken down into two primary functions — compilation and linking.
-
Compilation is the first step, where the source code is transformed into object code. During this phase, the compiler checks the syntax for errors, interprets the program logic, and generates machine code that will be executed.
-
Linking occurs after compilation. In this stage, the various object files are combined into a single executable. The linker resolves references between different files, ensuring that all components of your program can interact correctly.
The C++ build process can be further divided into several phases:
-
Preprocessing: The preprocessor handles directives like `#include` and `#define`, inserting the contents of included files and defining macros before the actual compilation takes place.
-
Compilation: As mentioned, this phase translates the preprocessed code into lower-level object code. It's the main responsibility of the compiler.
-
Assembly: The assembly phase converts the object code into machine code, which can be executed by the computer's processor.
-
Linking: Finally, the linking phase combines various modules into a final binary, resolving external dependencies as needed.
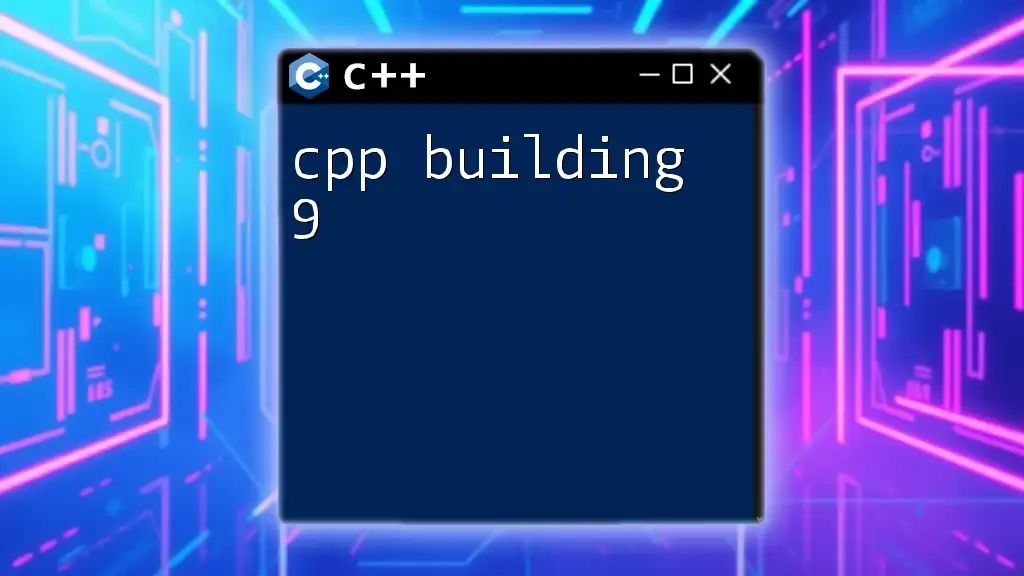
Essential Tools for C++ Building
C++ Compilers
Several compilers are widely used in the C++ programming community, each with its own strengths:
-
GCC (GNU Compiler Collection): A popular open-source compiler that supports multiple programming languages, renowned for its extensive feature set and optimization capabilities.
-
Clang: A compiler front end for the C family of languages, offering high performance, expressive diagnostics, and modular architecture.
-
Microsoft Visual C++: A premier compiler for Windows applications, tightly integrated with the Visual Studio IDE, renowned for its debugging tools and user-friendly interface.
When choosing a compiler, consider your target platform, the features and optimizations you need, and community support.
Build Systems
A build system manages the compilation and linking of code, automating the process and ensuring that dependencies are efficiently handled. Popular build systems include:
-
CMake: An open-source system that manages the build process across different platforms. CMake generates native makefiles and workspaces that can be used in multiple environments.
-
Make: A traditional tool that uses a file called `Makefile` to define how to compile and link applications. It's highly configurable and widely used for smaller projects.
-
Ninja: A small build system focused on speed. It is often used as a back end for larger systems like CMake.
Choosing the right build system is crucial as it significantly impacts your development workflow, especially in larger projects.
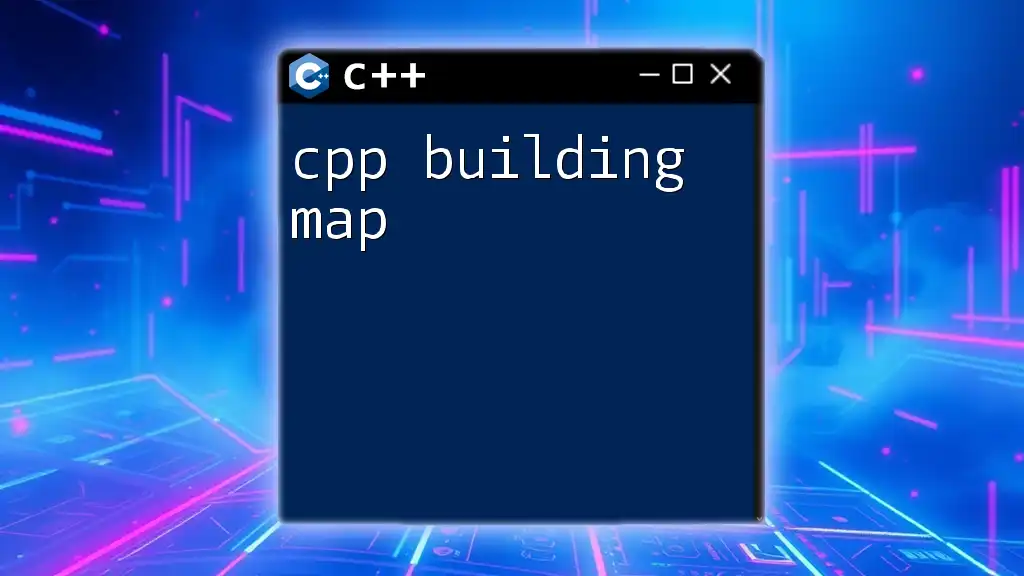
Practical Guide to C++ Building
Setting Up Your Development Environment
Establishing a solid C++ development environment is the first step towards successful programming. Here are key components to consider:
-
IDE Selection: Popular Integrated Development Environments such as Visual Studio, Code::Blocks, and CLion provide comprehensive tools for writing, testing, and debugging C++ code.
-
Setting Up Compiler Paths: Ensure that your compiler is included in the system’s PATH variable, allowing you to run it from any command line interface.
To set up an environment using GCC on a Unix-based system, you can follow these steps:
-
Install GCC:
sudo apt-get install build-essential
-
Verify the installation:
g++ --version
This setup allows you to compile your C++ programs directly from the terminal.
Writing Your First C++ Program
Once your environment is ready, you can write your first C++ program. Here’s a simple example:
#include <iostream>
int main() {
std::cout << "Hello, C++ Building!" << std::endl;
return 0;
}
To compile and run this program using GCC, follow these steps:
- Save the code in a file named `main.cpp`.
- Open the terminal, navigate to the directory containing your file, and run:
g++ main.cpp -o hello
- Execute your program:
./hello
If done correctly, you should see the output: `Hello, C++ Building!`
Building Larger Projects
Organizing Your Code
As your projects grow, it becomes critical to organize your code effectively. A well-structured hierarchy helps in debugging, enhances readability, and facilitates maintenance. Consider these practices:
- Directory Structure: Maintain separate folders for source files, header files, and libraries.
MyProject/
├── src/
│ └── main.cpp
├── include/
│ └── utils.h
└── lib/
└── mylib.a
-
Header Files vs. Source Files: Use header files for declarations and source files for implementations, promoting modularity.
-
Use of Namespaces: Namespace usage can help prevent naming collisions in larger projects.
Managing Dependencies
For larger projects, managing dependencies is vital. Dependencies often involve libraries that your application might use.
Utilizing a build system like CMake simplifies dependency management. Here's an example of a minimal `CMakeLists.txt` for a C++ project:
cmake_minimum_required(VERSION 3.10)
project(MyProject)
add_executable(MyExecutable main.cpp)
This CMake file specifies the minimum CMake version, names the project, and indicates which source files to compile.
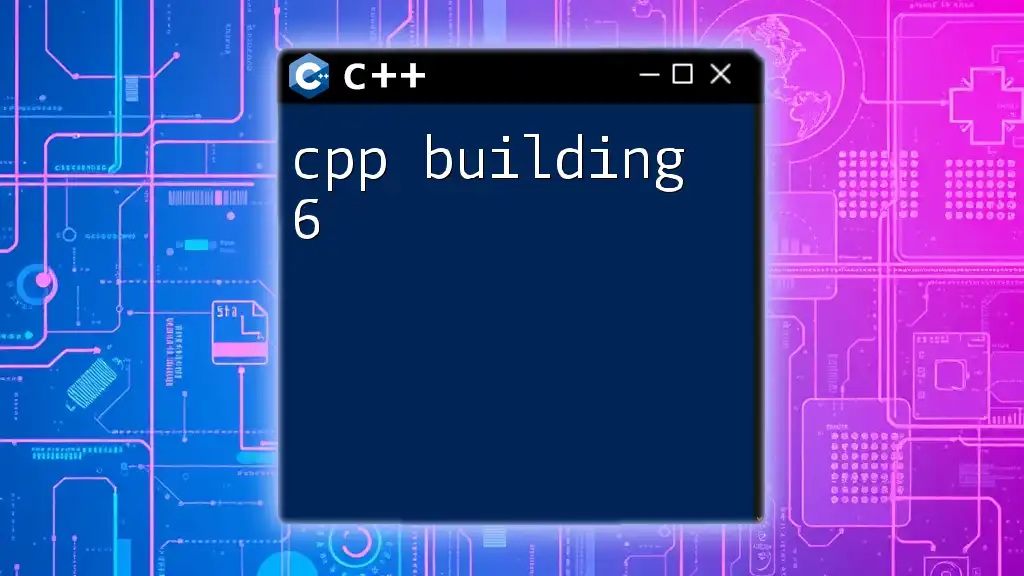
Advanced Topics in C++ Building
Debugging and Optimizing Your Build
Once your code is functional, focus on debugging and optimization. Some common build issues include:
-
Linker Errors: Often occur due to unresolved symbols. Ensure that every function definition has a corresponding declaration in a header file.
-
Compiler Warnings: Pay attention to warnings, as they can indicate potential issues that might lead to runtime errors.
To improve build performance, consider these strategies:
-
Build Configurations: Use `Debug` configurations for development and `Release` configurations for production to optimize speed and reduce file size.
-
Code Optimization Techniques: Utilize compiler flags for optimization. For example, using `-O2` with GCC can enhance performance:
g++ -O2 main.cpp -o optimized_program
Continuous Integration and Deployment (CI/CD)
CI/CD practices are becoming critical in software development, ensuring the integrity and availability of codebases. In the context of C++ building, settings for CI tools like Travis CI or GitHub Actions can automate the build process, making it easier to detect issues in real-time.
A simple example of a GitHub Actions configuration that triggers a build on every push might look like this:
name: C++ CI
on: [push]
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Set up GCC
run: sudo apt-get install g++
- name: Build
run: g++ -o hello main.cpp
This script sets up an Ubuntu environment, installs the required compiler, and compiles your C++ code each time there’s a new push to the repository.
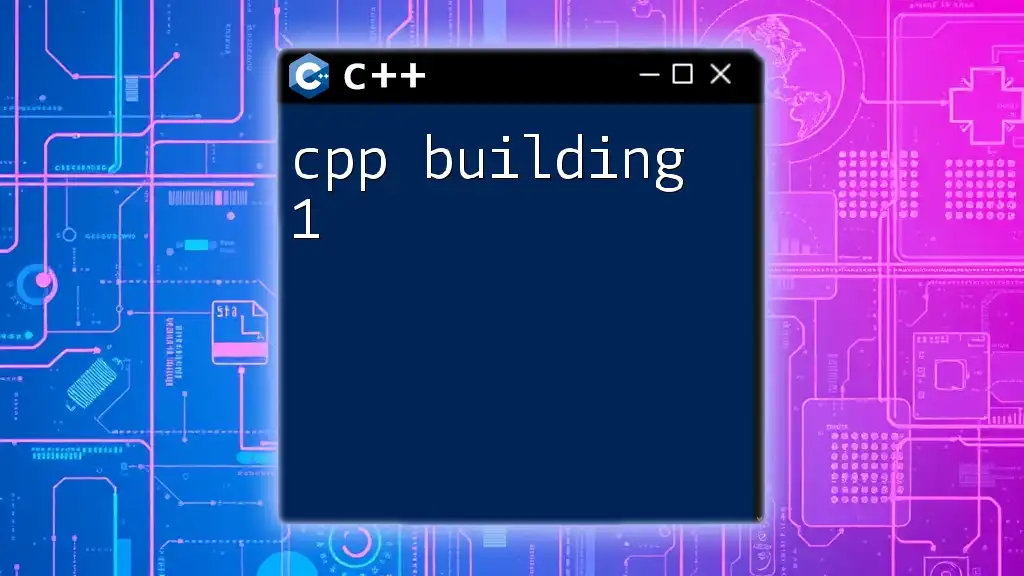
Final Thoughts
Key Takeaways
Mastering CPP building techniques is crucial for developing efficient and maintainable software. By understanding the build process, utilizing the right tools, and organizing code effectively, developers can enhance their skills and workflows.
Additional Resources
To continue your learning journey, consider exploring the following:
- Books: Recommended titles such as "Effective C++" by Scott Meyers.
- Online Tutorials: Websites like Codecademy or Udacity.
- Community Forums: Engage with C++ communities on Stack Overflow, Reddit, or specialized forums.
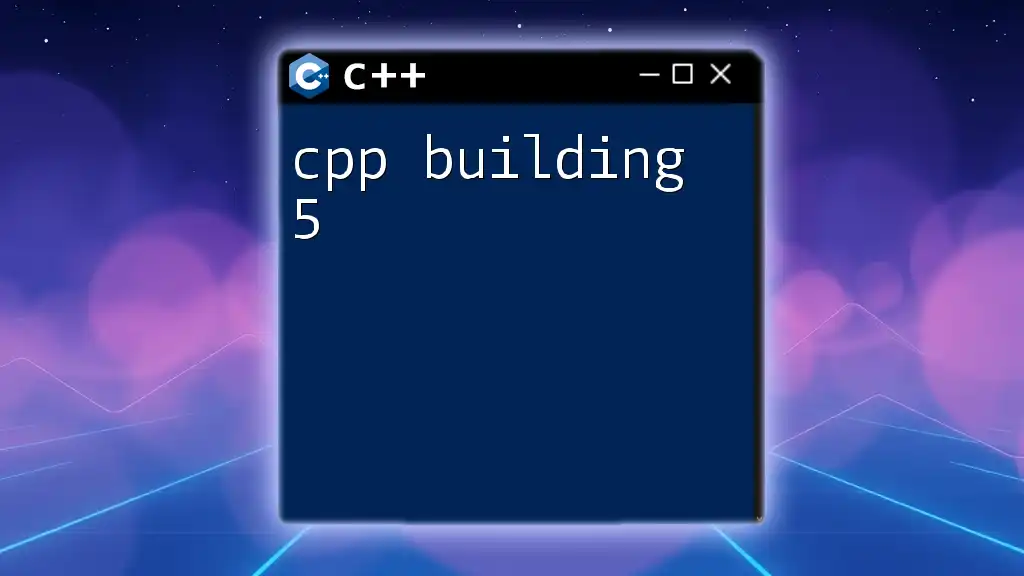
Conclusion
Embracing the C++ building process not only enriches your programming skills but also enhances your productivity. As you delve into cpp building 4, witness how a structured approach can pave the way for developing robust and efficient C++ applications. Join our platform for more resources and in-depth learning on mastering C++ commands!