"CPP building 66 refers to the process of compiling a C++ program using a build system, specifically focusing on setting up and managing project configurations efficiently."
Here’s a simple code snippet for a basic C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Overview of CPP Building 66
What is CPP Building 66?
CPP Building 66 refers to a concise framework of fundamental C++ commands that enhance the programming experience. This concept encompasses a curated list of commands and practices that allow developers to efficiently navigate the complexities of C++ programming. Designed to streamline the building process of C++ applications, CPP Building 66 has become a pivotal part of modern programming practices.
Why Learn CPP Building 66?
Understanding CPP Building 66 is essential for programmers, whether they are novices or seasoned developers. Learning these commands provides several advantages:
- Increased Productivity: Mastering CPP commands can significantly reduce the development time by streamlining repetitive tasks.
- Better Code Management: Familiarity with these commands leads to cleaner code and easier debugging.
- Enhanced Career Opportunities: Knowledge of CPP commands is attractive to employers, making developers more marketable.
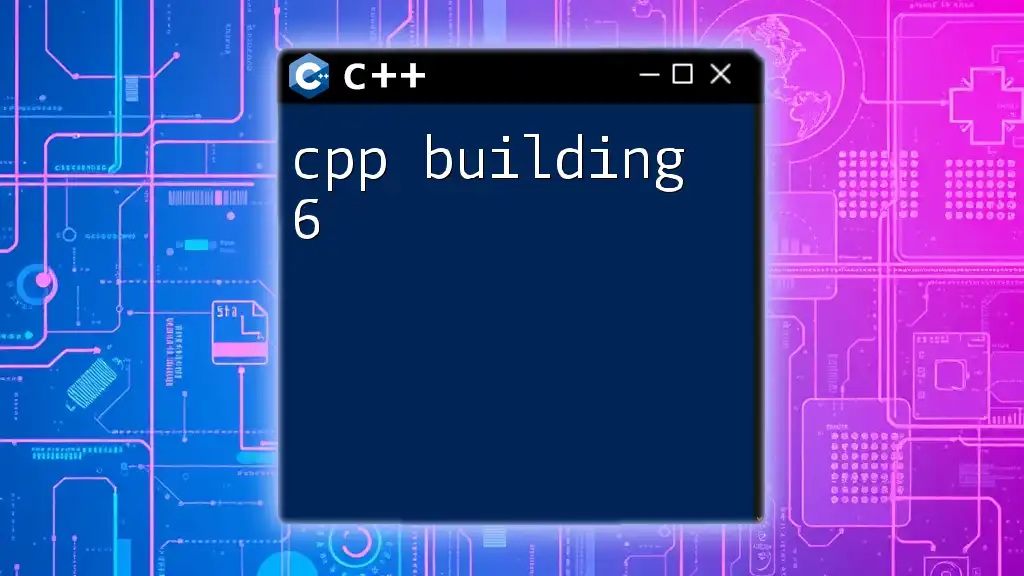
Getting Started with CPP Building 66
Prerequisites
Before diving into CPP Building 66, ensure you have a foundational understanding of C and C++. Familiarity with basic programming concepts, such as variables, loops, and functions, is essential. Additionally, you'll need the following tools:
- A C++ compiler (like g++)
- An Integrated Development Environment (IDE) like Visual Studio Code or Code::Blocks
Setting Up Your Development Environment
To effectively utilize CPP Building 66, you must set up your development environment. Here’s how to install the g++ compiler on various operating systems:
Installing g++
-
Windows:
- Download and install MinGW from [the MinGW website](http://www.mingw.org/).
- Add the `bin` directory (usually `C:\MinGW\bin`) to your system’s PATH.
-
macOS:
- Open Terminal and run:
xcode-select --install
-
Linux:
- Open the terminal and run:
sudo apt update sudo apt install g++
After installation, verify the setup by running the command:
g++ --version
This should display the version of g++ installed on your system.
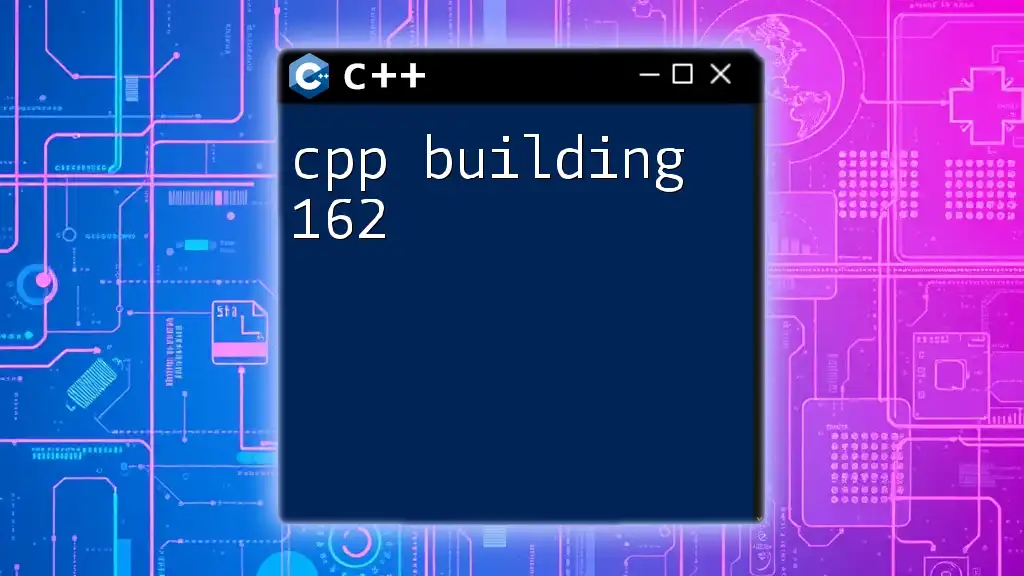
Understanding the Concepts of CPP Building 66
Key CPP Commands
Overview of Essential Commands
CPP Building 66 focuses on several essential C++ commands. Some of these include:
- `#include` - This command is used to include header files, enabling you to use libraries like `<iostream>` for input/output functionalities.
- `main()` - The entry point of every C++ program. The execution starts from the `main()` function.
Example of essential commands in a simple program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Advanced Commands
In CPP Building 66, advanced commands allow for more sophisticated programming techniques:
- `namespace` - This command helps avoid name conflicts by grouping related functionality under a unique identifier.
- `template` - A powerful feature for creating generic functions or classes.
Example of using a template function:
template <typename T>
T add(T a, T b) {
return a + b;
}
Building Projects with CPP Commands
Structure of a CPP Project
A typical CPP project comprises various components, including source files, headers, and resource files. Organizing your project structure is crucial for maintaining clarity. Here’s an example of a simple structure:
/MyProject
|-- src
| |-- main.cpp
|-- include
| |-- functions.h
|-- bin
|-- Makefile
Step-by-Step Project Building
Building a simple CPP project demonstrates how these commands come together. For instance, let’s create a "Hello World" program:
-
Create a file named `main.cpp` in the `src` directory.
-
Insert the following code:
#include <iostream> int main() { std::cout << "Hello, World!" << std::endl; return 0; }
-
Compile the program: Navigate to your project's `src` directory in the terminal and run:
g++ main.cpp -o HelloWorld
-
Run the program:
./HelloWorld
This program outputs "Hello, World!" to the console, showcasing a fundamental command in action.
Debugging and Testing Your Build
Debugging is essential for efficient coding. Using gdb, you can identify issues in your CPP project. Begin by compiling your code with debugging information:
g++ -g main.cpp -o HelloWorld
Then, run gdb:
gdb ./HelloWorld
You can set breakpoints, inspect variables, and step through the code, making it easier to catch bugs.
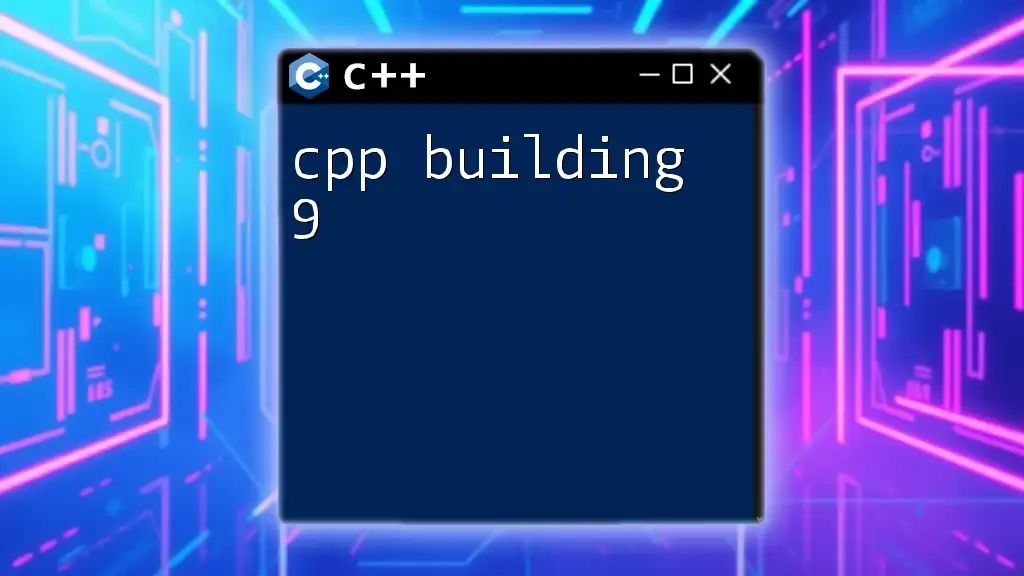
Practical Applications of CPP Building 66
Real-World Use Cases
CPP Building 66 is prevalent across various sectors, including:
- Game Development: Many game engines leverage C++ for speed and performance.
- Embedded Systems: C++ commands are crucial for low-level hardware manipulation.
Case Study: A Successful CPP Project
Examine a software application that utilized CPP Building 66 to streamline its codebase. For instance, a simplistic calculator application could demonstrate core functionalities such as addition, subtraction, and error handling.
Key components might be organized in distinct functions, allowing for a clear and maintainable structure.
#include <iostream>
double add(double a, double b) {
return a + b;
}
int main() {
std::cout << "Sum: " << add(5.0, 3.1) << std::endl;
return 0;
}
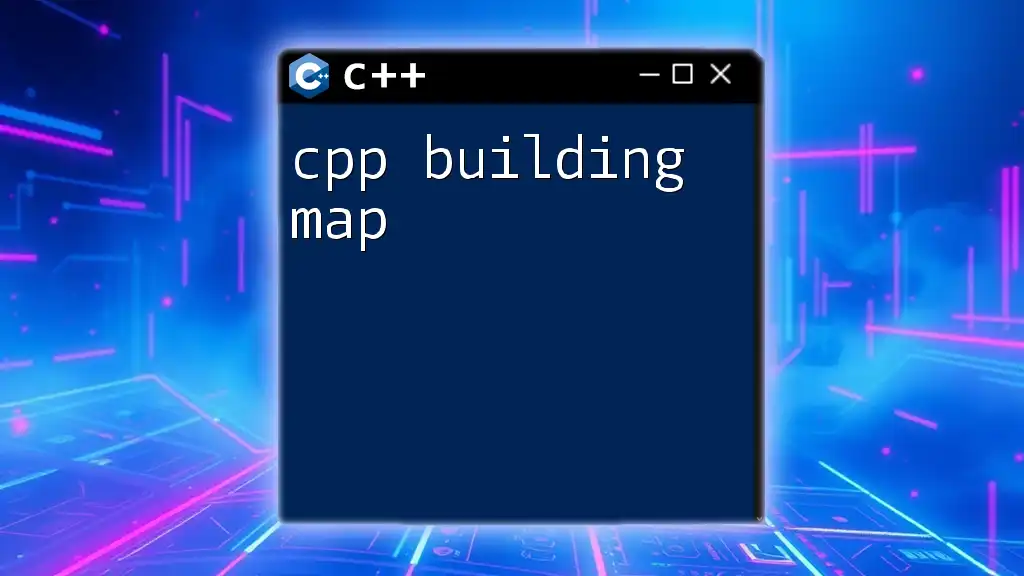
Common Mistakes and How to Avoid Them
Top Errors in CPP Building 66
Common pitfalls include forgetting to include necessary header files, leading to unresolved symbols, or not properly managing memory, which can result in leaks. Always compile your code frequently to catch such errors early.
Best Practices for CPP Building
To ensure efficient coding, adopt the following best practices:
- Use Meaningful Names: Variable and function names should accurately reflect their purpose.
- Comment Your Code: Clear comments help explain your logic, making your code easier to understand for others (and yourself).
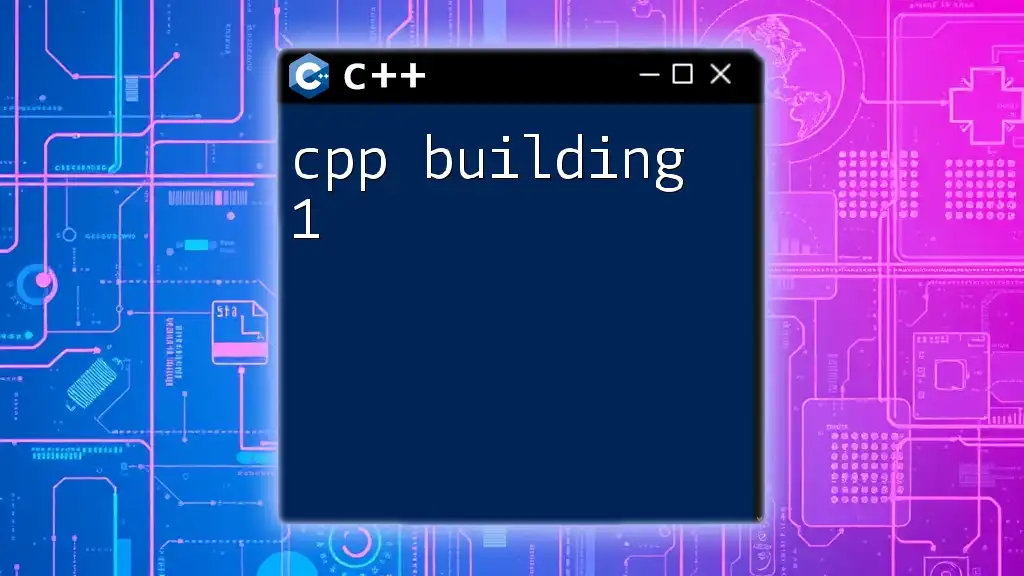
Resources for Further Learning
Recommended Books and Online Courses
To continue mastering CPP Building 66, consider the following resources:
- Books: "C++ Primer" by Stanley B. Lippman for deeper insights into core C++ concepts.
- Online Courses: Platforms like Coursera and Udemy offer comprehensive courses that include CPP Building 66 modules.
Community Forums and Support
Engaging with online communities, such as Stack Overflow or Reddit's r/cpp, allows you to ask questions, share knowledge, and learn from experienced programmers.
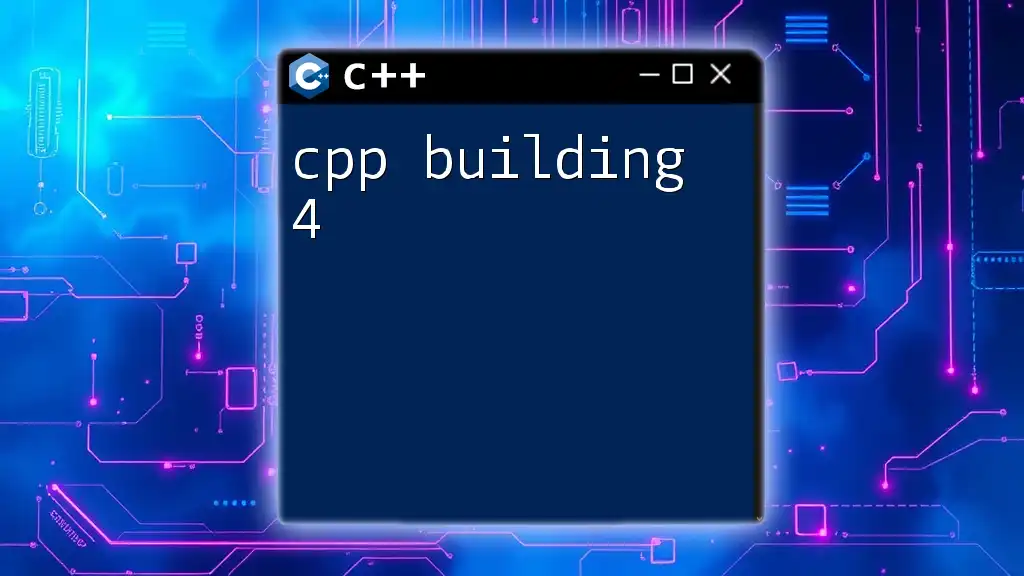
Conclusion
Recap of CPP Building 66
In summary, CPP Building 66 represents a structured approach to mastering C++ commands. Understanding these commands can dramatically influence your development skills and job prospects.
Call to Action
It's time to dive into the world of CPP Building 66! Start practicing the commands discussed, explore related resources, and become a proficient C++ programmer who can tackle complex projects with ease.