"C++ Building 6" focuses on using the C++ build system to compile and link programs efficiently, ensuring that developers can quickly manage their projects through simple commands.
Here’s a code snippet illustrating a basic compilation command:
g++ -o my_program main.cpp
Understanding C++ Building
What is C++ Building?
C++ building refers to the process of converting human-readable C++ code into machine-readable executable programs. This process is crucial in software development as it transforms the source code into a format that a computer can execute. The key components involved in building C++ applications include the compiler, linker, and build systems.
Compilation Process
Stages of Compilation
The compilation process can be broken down into three distinct phases:
-
Preprocessing: This is the first step where the preprocessor handles directives (like `#include` and `#define`). It processes source files to include header files, expand macros, and remove comments.
-
Compilation: The compiler translates the preprocessed code into assembly language, generating object code that is a low-level representation of your program.
-
Linking: The linker combines multiple object files and resolves external references to produce a final executable. It ensures that function calls and variable references are correctly mapped to their defined locations.
Code Snippet: A simple example of a C++ program
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Tools and Environments
Common C++ Build Tools
Several build tools facilitate the compilation and linking processes in C++. Among the most popular are:
-
CMake: A powerful tool that generates makefiles and project files for various environments. It excels in handling large projects with multiple dependencies.
-
Make: This traditional build system uses a Makefile to control the build process. It checks file timestamps and executes necessary commands to update the executable as needed.
Understanding when to use these tools is essential. For simple projects, a Makefile suffices, while CMake is preferable for larger, complex applications.
Development Environments
IDEs for C++ Development
Integrated Development Environments (IDEs) offer comprehensive tools to streamline the C++ development process. Some popular choices include:
-
Visual Studio: Ideal for Windows developers, it provides extensive debugging tools, a rich graphical interface, and powerful autocomplete functionality.
-
Code::Blocks: An open-source IDE that is highly customizable and lightweight, suitable for beginners and those who prefer simplicity.
These IDEs often include built-in support for building projects, making it easier for developers to compile their code without needing to rely entirely on the command line.

Setting Up Your C++ Environment
Installing Necessary Tools
To begin with C++ building, you need to install a C++ compiler and possibly an IDE. Here's how to install the GNU Compiler Collection (GCC) on various systems:
- For Windows: Install MinGW, which includes GCC as part of its package.
- For macOS: Use Homebrew and run the command `brew install gcc`.
- For Linux: Most distributions come with GCC pre-installed; if not, you can install it using the package manager (e.g., `sudo apt install build-essential`).
Configuring Your First Project
Creating a Basic C++ Project
Setting up a basic project structure can be essential for better organization.
- Create a main project folder.
- Inside it, create subfolders such as `src`, `include`, and `lib`.
Code Snippet: Directory setup example
/MyCPPProject
├── /src
│ └── main.cpp
├── /include
└── /lib
Build Configuration
It's also essential to understand build configurations—primarily Debug and Release. Debug configurations include extra information for debugging but may run slower due to this additional data. Release configurations optimize the code for performance, often removing debug symbols.
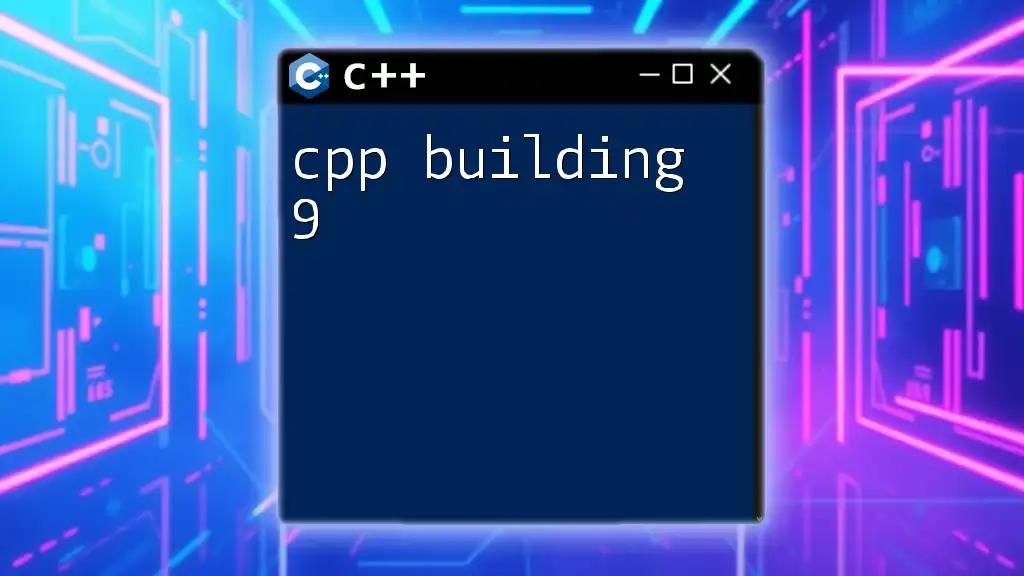
Building a C++ Project
Writing a Simple Application
Start by writing a simple application. Here’s an example, extending the `Hello World` idea into a basic calculator:
#include <iostream>
using namespace std;
int main() {
int a, b;
cout << "Enter two integers: ";
cin >> a >> b;
cout << "Sum: " << a + b << endl;
return 0;
}
Running the Build Process
To compile and link your program, you can use the command line. For a simple application using GCC, the command would look like this:
g++ src/main.cpp -o myCalculator
This command compiles `main.cpp` and produces an executable named `myCalculator`.
Troubleshooting Common Build Errors
Understanding Compile-Time Errors
It’s common to encounter compile-time errors. Here are a few typical ones:
- Syntax errors: Forgetting a semicolon or using the wrong syntax can halt the compilation process. The compiler’s error messages will indicate the issue's location.
- Undefined references: These occur when the compiler cannot find a function definition that was declared. Ensuring that all functions are defined before they are called resolves this.
Runtime Errors and Debugging
Building with Debugging in Mind
Debugging is an integral part of software development. Utilize built-in debugging tools provided by your IDE, or you can rely on command-line debugging tools like `gdb`.
Code Snippet: Example of common debugging commands
gdb myCalculator
break main
run
This will allow you to set breakpoints and step through your code, inspecting variables and flow control.
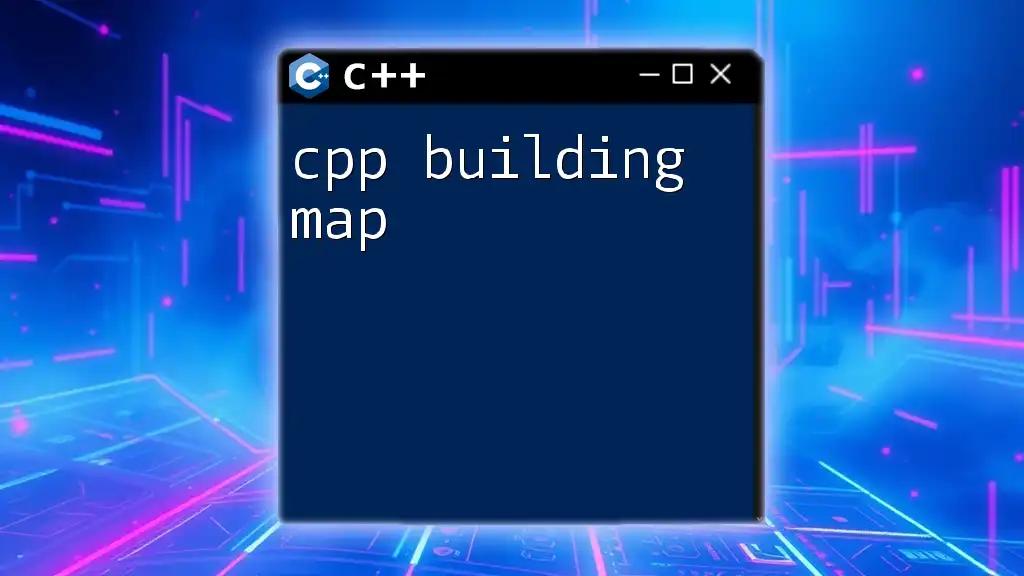
Advanced Building Techniques
Using Makefiles
Makefiles can automate the build process, especially for larger projects. A simple Makefile for our calculator might look like this:
CC=g++
CFLAGS=-Iinclude
OBJ=main.o
myCalculator: $(OBJ)
$(CC) -o $@ $^
%.o: %.cpp
$(CC) -c $< $(CFLAGS)
This example compiles `main.o` and links it to create the final executable with the command `make`.
CMake Basics
CMake for Efficient Builds
CMake is a widely adopted tool that enhances project management for C++ applications significantly. It generates build instructions for various platforms, ensuring smooth compilation across systems. A basic `CMakeLists.txt` file might look like this:
cmake_minimum_required(VERSION 3.0)
project(MyCPPProject)
add_executable(myCalculator src/main.cpp)
Continuous Integration
Setting Up CI/CD for C++ Projects
As projects grow, implementing Continuous Integration/Continuous Deployment (CI/CD) becomes essential. Tools like Travis CI and GitHub Actions provide automated building and testing functionalities.
These tools automatically run your build scripts every time changes are pushed to your repository, ensuring that code changes do not introduce new errors or break the build.
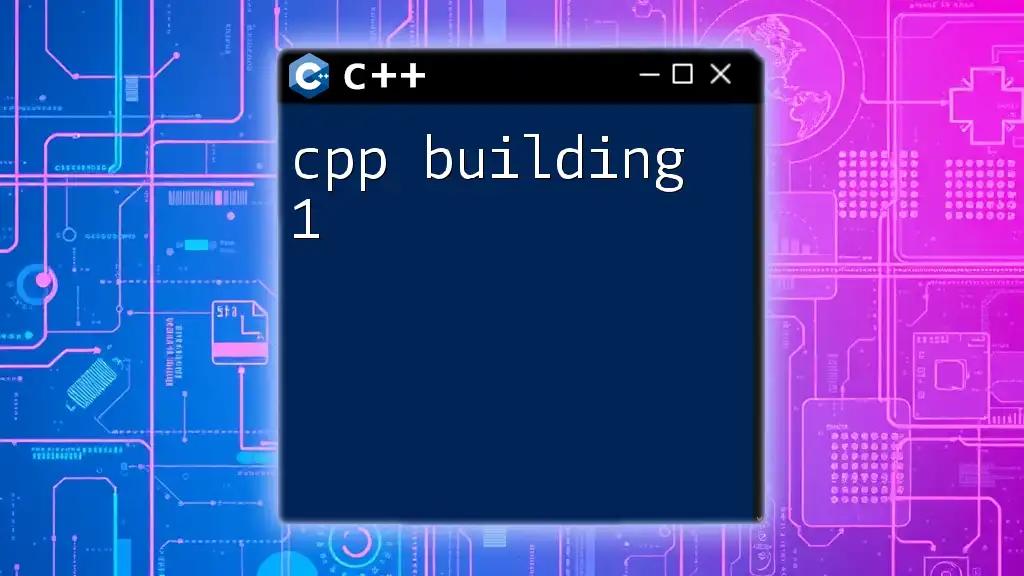
Conclusion
Understanding C++ building is crucial for any developer looking to create efficient and performant applications. By becoming familiar with the compilation process, utilizing build tools effectively, and embracing advanced techniques, you can streamline your development workflow and minimize compilation errors. Practice is key—the more you build, the more proficient you will become.
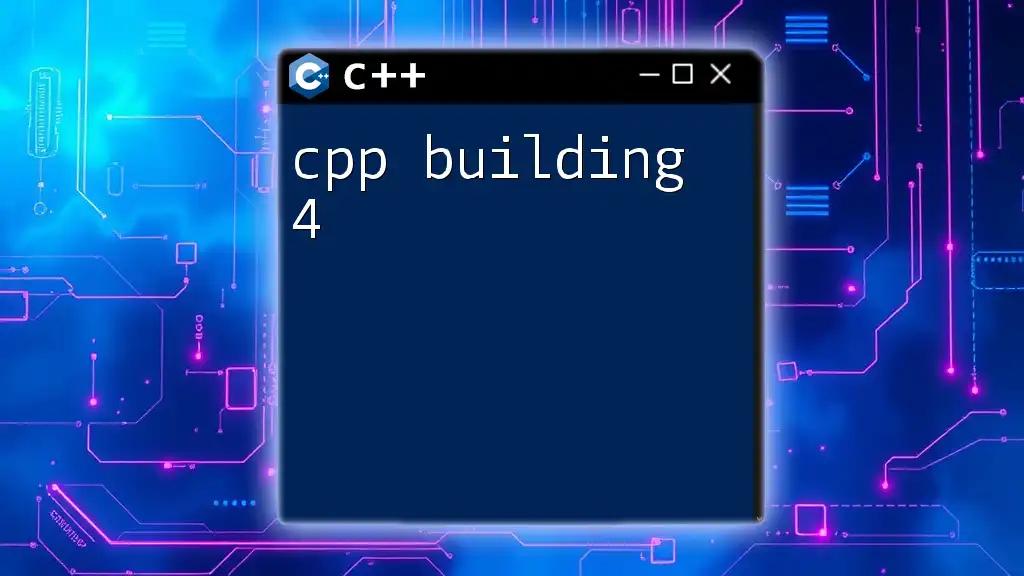
Additional Resources
For further learning and exploration, consider checking:
- Official C++ documentation and resources
- Libraries and frameworks like STL (Standard Template Library) and Boost for enhanced functionality in your projects.