"CPP building 15" refers to optimizing and efficiently structuring a C++ program by utilizing the latest features and best practices in C++15 for improved performance and readability.
Here's a code snippet demonstrating the use of a lambda expression, a feature introduced in C++11 and enhanced in C++14/15:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> nums = {1, 2, 3, 4, 5};
int sum = 0;
std::for_each(nums.begin(), nums.end(), [&sum](int n) {
sum += n;
});
std::cout << "Sum: " << sum << std::endl;
return 0;
}
Understanding the C++ Build Process
What Happens During the Build?
When you compile a C++ program, the build process undergoes several stages that transform your high-level C++ code into an executable program. Understanding these stages is crucial for troubleshooting and optimizing your build process:
-
Preprocessing: This is the first stage, where the preprocessor handles directives (commands) that start with `#`, such as `#include` and `#define`. It processes these directives and creates a modified source file.
-
Compilation: In this stage, the compiler translates the preprocessed code into assembly language. This conversion checks for syntax errors and generates assembly instructions.
-
Assembly: The assembly code is then transformed into machine code by an assembler, creating object files (.o or .obj) that contain machine-readable instructions.
-
Linking: Finally, the linker combines all the object files and resolves references to libraries and other object files to create the final executable.
Tools Used in C++ Building
Building a C++ project requires tools known as compilers and build systems. Some of the most common include:
-
g++: Part of the GNU Compiler Collection, g++ is widely used in many development environments.
-
clang: A compiler based on LLVM that provides fast compilation and excellent diagnostics.
-
Visual Studio: A powerful IDE for Windows, which includes a built-in C++ compiler.
Each tool serves a slightly different purpose and offers unique features, making the choice of toolchain an important aspect of your C++ development environment.
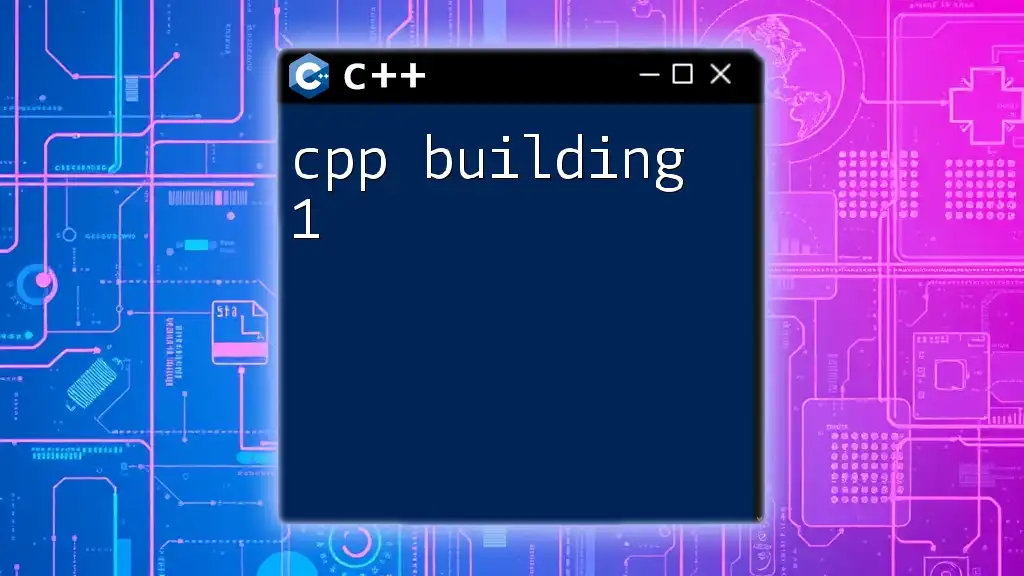
Setting Up Your C++ Build Environment
Installing C++ Compilers
Setting up a development environment begins with installing a C++ compiler. Here’s how you can install g++ on different operating systems:
-
Windows: Install MinGW or use WSL (Windows Subsystem for Linux). For MinGW:
- Download the MinGW installer from its official website.
- Select g++ during the installation process.
- Add the bin directory (e.g., `C:\MinGW\bin`) to your system PATH.
-
macOS: Use Homebrew to install g++:
brew install gcc
-
Linux: Install g++ using your package manager:
sudo apt install g++
Configuring Development Environment
Choosing the right IDE or text editor optimizes your workflow. Here are some popular options:
- Visual Studio: Excellent for Windows development, offering rich features for debugging and building.
- Code::Blocks: A free C++ IDE that is lightweight and highly configurable.
- Eclipse: With CDT (C/C++ Development Tooling) support, Eclipse is a versatile option.
For configurations, ensure that the paths to your compiler are set correctly in your IDE of choice, allowing seamless integration.
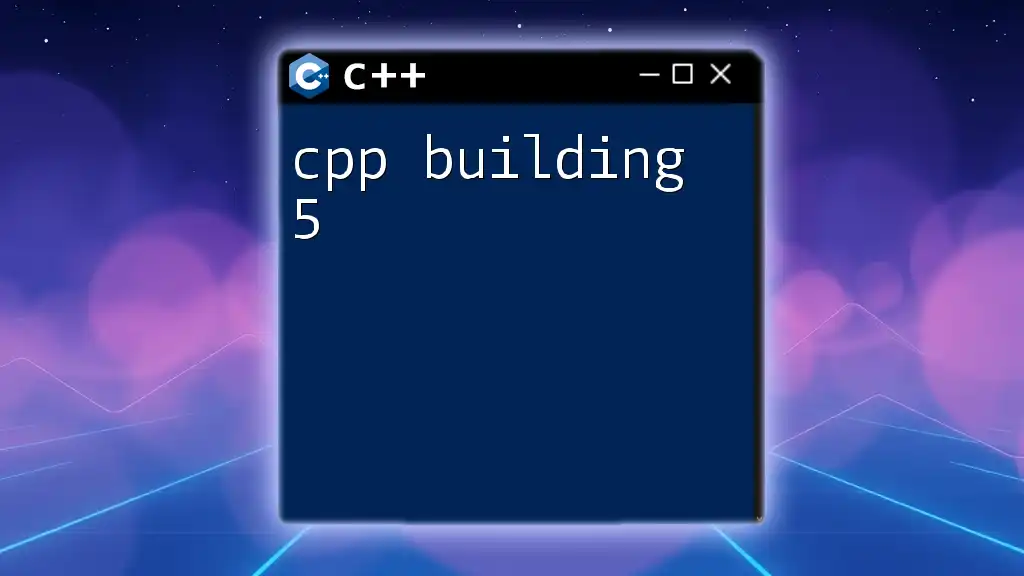
Key C++ Commands for Building Projects
Introduction to Basic Commands
C++ building involves several essential commands used frequently. Familiarizing yourself with the following commands can significantly improve your efficiency:
- g++: The primary command to compile C++ files.
- make: A build automation tool that builds projects based on Makefiles.
- cmake: A cross-platform build system that manages the generation of build files.
Using g++
The `g++` command is the cornerstone of compiling C++ programs. Here is the syntax:
g++ [options] [source files] -o [output file]
For example, if you have a simple C++ program saved as `hello.cpp`:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
You can compile it using:
g++ hello.cpp -o hello
After execution, run the program with:
./hello
Implementing Makefiles
A Makefile is a simple, yet powerful utility that automates the build process by defining rules for how to compile and link the program. Here’s a straightforward Makefile:
# Simple Makefile
all: main
main: main.o util.o
g++ -o main main.o util.o
%.o: %.cpp
g++ -c $<
clean:
rm -f *.o main
In this Makefile:
- `all` is the default target, which calls the `main` target.
- The rule `main: main.o util.o` specifies that to create `main`, it needs `main.o` and `util.o`.
- The use of `%.o: %.cpp` allows you to define how to compile `.cpp` files into `.o` files using pattern rules, while the `clean` target cleans up the build files.
Exploring CMake
CMake is another powerful build system that is more user-friendly for managing larger projects. Unlike Makefiles, CMake generates platform-specific build files such as Makefiles or Visual Studio project files. A simple `CMakeLists.txt` might look like:
cmake_minimum_required(VERSION 3.10)
project(ExampleProject)
add_executable(Example main.cpp)
This file specifies that an executable named `Example` should be built from `main.cpp`.
To build a project with CMake, follow these steps:
- Create a `build` directory within your project.
- Inside that directory, run:
cmake .. make
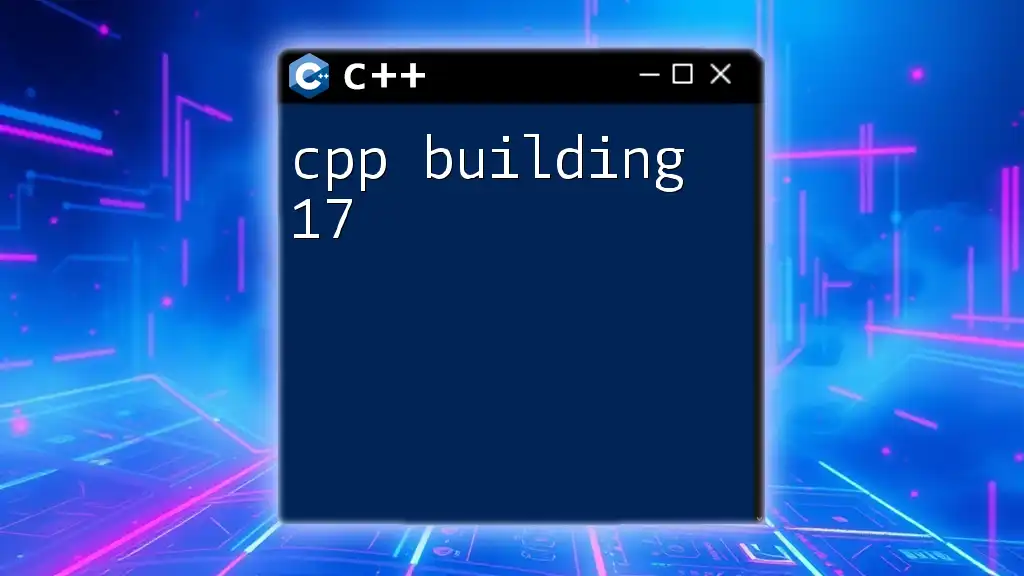
Advanced Tips for Efficient C++ Builds
Optimizing Build Performance
Optimizing your build process can significantly reduce development time. Here are a few techniques:
- Precompiled Headers: These headers are compiled once to improve compile times, particularly in large projects.
- Build Configurations: Learn to differentiate between Debug and Release builds to utilize optimization flags effectively. A Debug build includes extra diagnostic information, while a Release build optimizes for performance.
Handling Dependencies in C++
Managing dependencies is crucial in any sizable C++ project. Tools like vcpkg can simplify this process by automating the installation of libraries. It allows you to include libraries effortlessly, reducing manual installation errors. Here’s how to install a library:
vcpkg install [library_name]
Just link it to your project using the proper path in your build configuration.
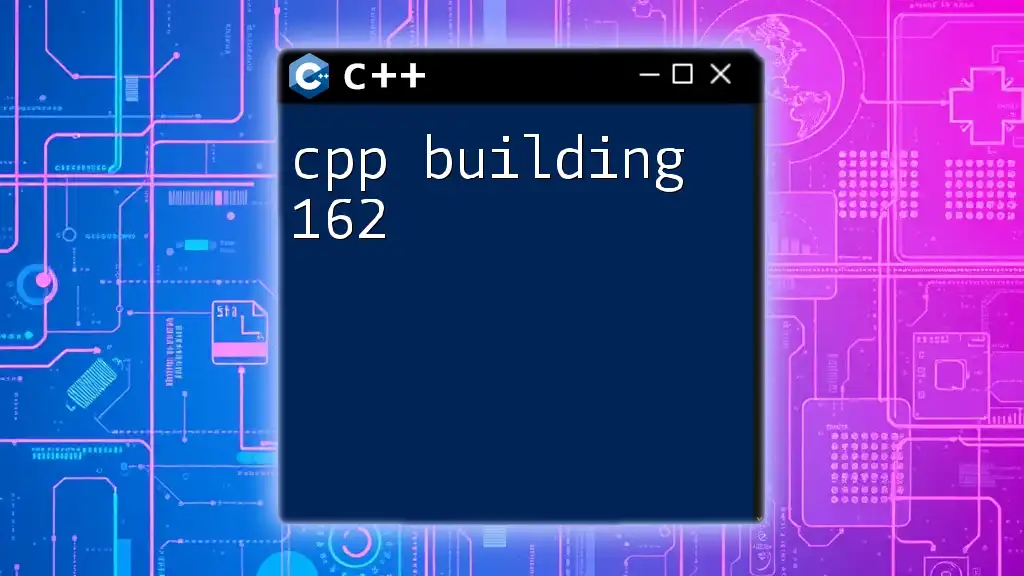
Troubleshooting Common Build Issues
Recognizing Build Errors
When building your project, you may encounter various errors. It's vital to understand these types:
- Syntax Errors: Typically caught during the compilation stage, indicating problems in your code's syntax.
- Linker Errors: Happen when you try to link an object file that can’t be found due to it either being absent or declared incorrectly.
Effective Debugging Techniques
Using tools like gdb can simplify the debugging process. Once your program compiles, run it under gdb to isolate the source of the issues.
gdb ./your_executable
Utilize commands like `run`, `break`, and `print` to analyze discrepancies in your program.
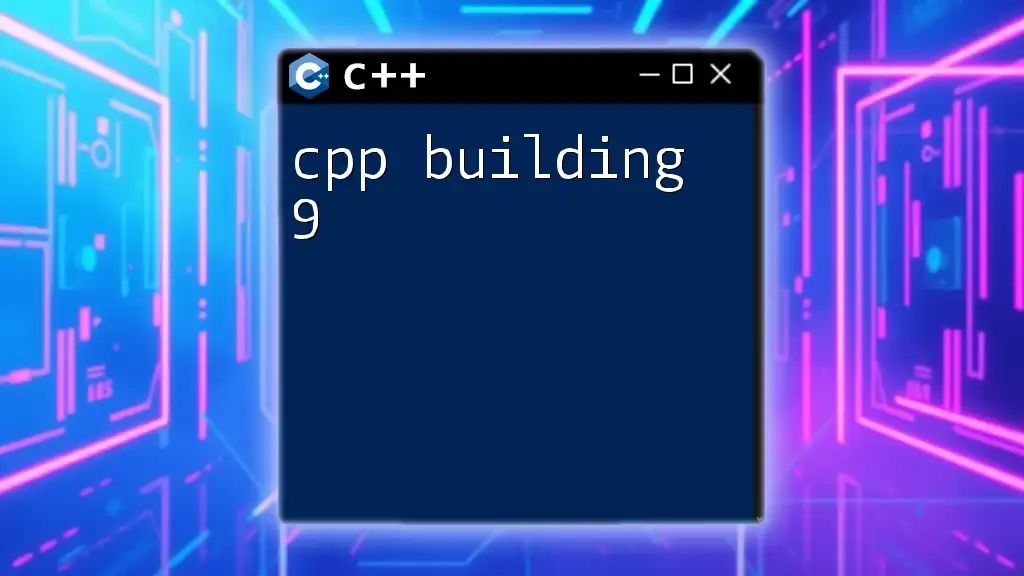
Conclusion
Understanding cpp building 15 and the intricacies of the build process is vital for any C++ developer. Mastery of the tools and techniques discussed in this guide will empower you to create efficient, high-quality C++ applications.
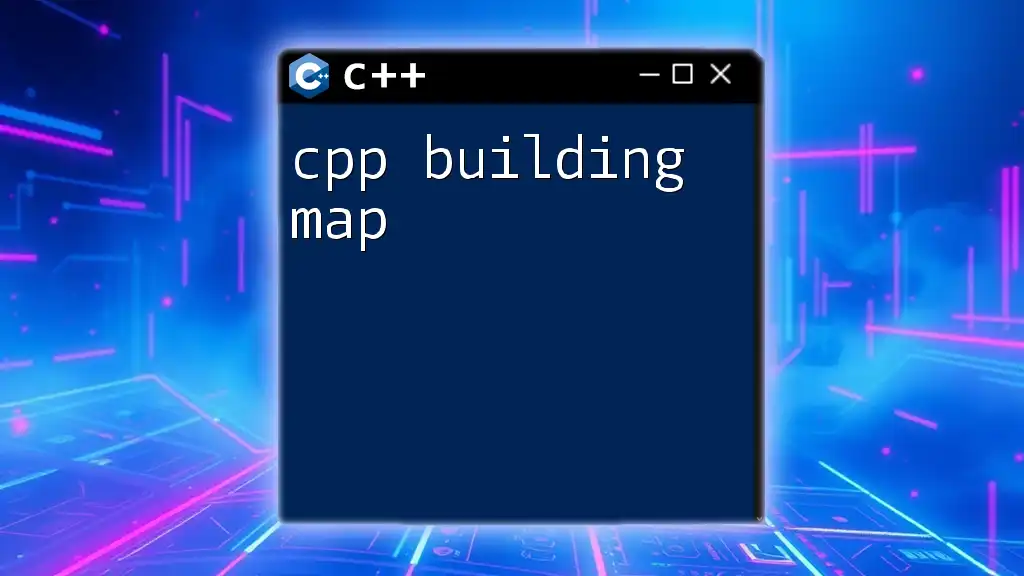
Call to Action
If you found this article helpful or want to share your experiences with C++ building, we encourage you to connect with us. Join our newsletter to keep informed about the latest updates, tips, and resources on enhancing your C++ skills!
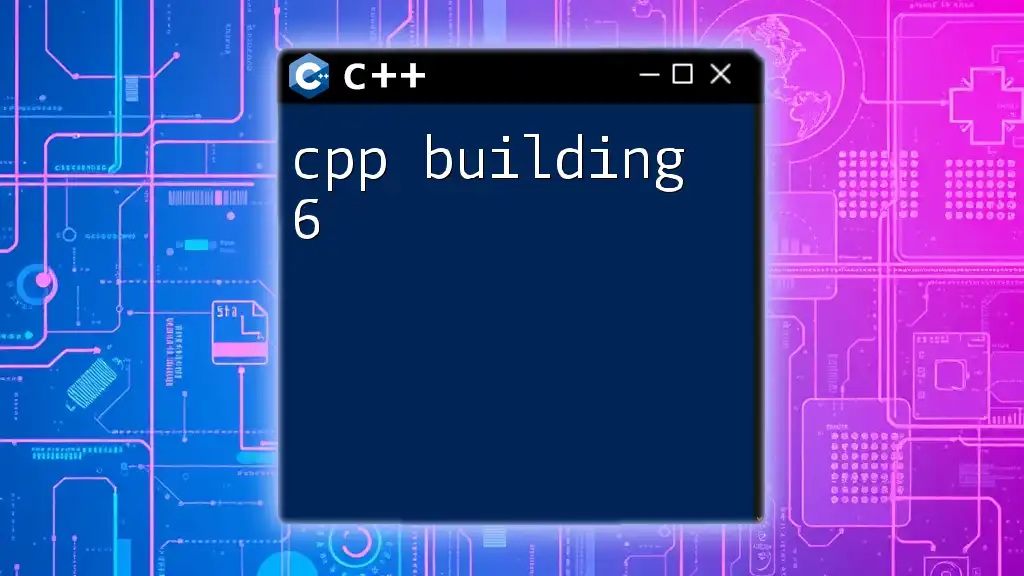
References
For further reading on C++ building and development, consider checking out these resources:
- Books on C++ programming and advanced C++ topics.
- Online courses that offer deeper dives into specific areas of C++ building and system design.