"CPP building 3" refers to the use of the C++ build system to compile and link multiple source files, enabling the creation of larger and more complex programs efficiently, as shown in the following code snippet:
// Sample main.cpp file
#include <iostream>
#include "functions.h"
int main() {
std::cout << greet("World") << std::endl;
return 0;
}
// Sample functions.cpp file
#include "functions.h"
std::string greet(const std::string& name) {
return "Hello, " + name + "!";
}
// Sample functions.h file
#ifndef FUNCTIONS_H
#define FUNCTIONS_H
#include <string>
std::string greet(const std::string& name);
#endif // FUNCTIONS_H
To build these files using `g++`, you would execute:
g++ main.cpp functions.cpp -o my_program
Understanding C++ Build Process
What is a Build Process?
The build process in C++ development is a series of steps that transform source code written by a programmer into executable code that a computer can run. This process is crucial, as it ensures that the program is compiled correctly, linked properly, and free of major errors. Understanding the key stages of the build process—compiling, linking, and loading—is essential for any programmer working with C++.
Tools Required for Building C++
To effectively build C++ applications, a few tools are necessary:
-
Compilers: Front-runners like GCC, Clang, and MSVC are widely used compilers that translate C++ code into machine language. Choosing the right compiler can influence performance and compatibility.
-
IDE Choices: Integrated Development Environments (IDEs) like Visual Studio and Code::Blocks provide user-friendly platforms that simplify coding, building, and debugging processes.
-
Build Systems: Tools like CMake and Makefile help manage and automate the build process, making it easier to compile large projects or those with multiple dependencies.
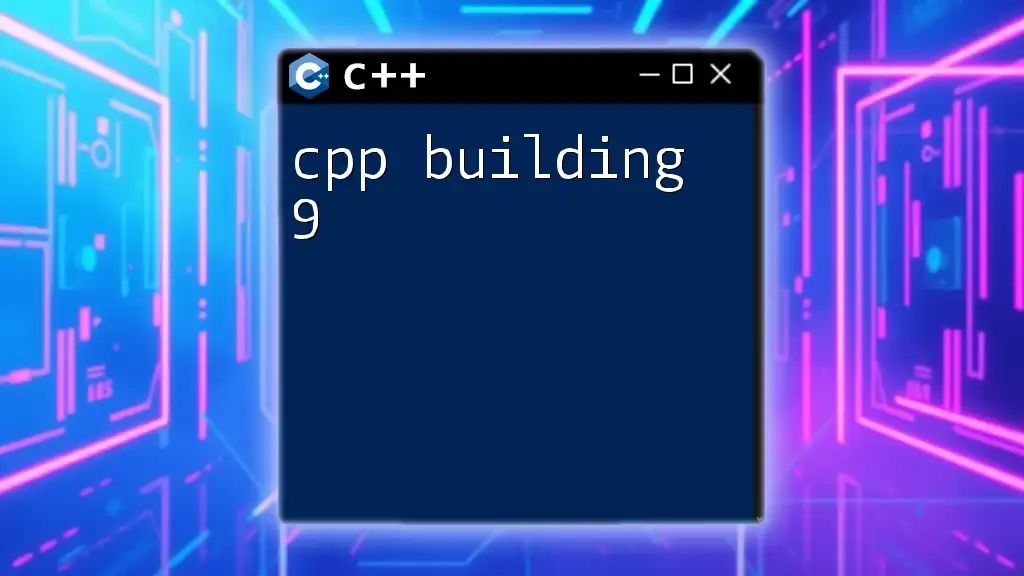
Key Concepts in C++ Building
Source Files and Object Files
Understanding the difference between source files and object files is fundamental in C++ building:
-
Source Files: These are files with a `.cpp` extension that contain the actual code written by developers. They need to be compiled to create executables.
-
Object Files: Once the compiler processes source files, it produces object files (`.o` or `.obj`). These files contain machine code, but they are not executable on their own until linked with other object files or libraries.
Linking Stages in C++
The linking stage is where object files are combined into a final executable. Here it is important to differentiate between two main types of linking:
-
Static Linking: This involves copying all the used library routines into the executable during the build. This results in larger executable files that don’t rely on external libraries at runtime.
Example of static linking command:
g++ -o myprogram main.o -static
-
Dynamic Linking: During dynamic linking, external libraries are referenced but not copied into the executable. This means the executable size is smaller, but it can fail at runtime if the required library isn’t available.
-
Linker Responsibilities: The linker handles addresses for the functions and variables defined across different object files, ensuring they can communicate seamlessly.
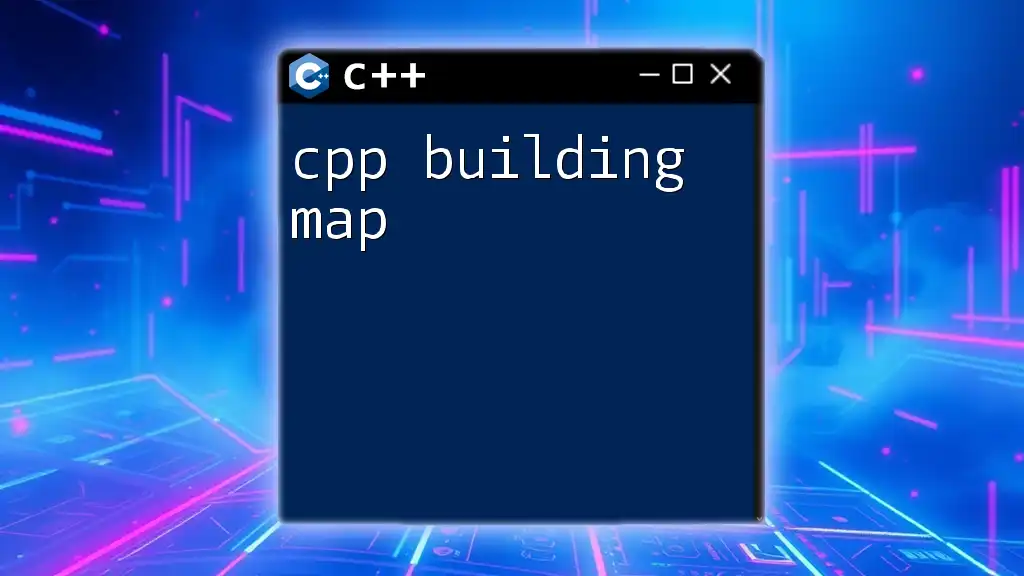
Step-by-Step Guide to Building your C++ Project
Setting Up Your Environment
-
Installing a Compiler: To get started, you need a C++ compiler. For example, installing GCC on different platforms is straightforward:
- Windows: You can use MinGW or install through WSL.
- macOS: Use Homebrew with the command:
brew install gcc
- Linux: Install via package management systems, like:
sudo apt install g++
-
Choosing an IDE: Select an IDE that you're comfortable with. Each IDE provides distinct features, so explore a few options to find the one that fits your style.
Writing Your First C++ Program
To illustrate how building works, let’s write a simple C++ program, the classic "Hello, World!" example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Next, you’ll compile and run this program. If using the command line, follow these steps:
- Open your terminal.
- Navigate to the directory containing your program file (e.g., `hello.cpp`).
- Compile the program using:
g++ -o hello hello.cpp
- Run the executable:
./hello
Structuring your Project
When building larger projects, organization is key. Following best practices for file organization can ease the development process:
- src/: Contains all your `.cpp` source files.
- include/: Stores the header files.
- lib/: Holds any external libraries your project depends on.
- bin/: This is where executable files can be placed after building.
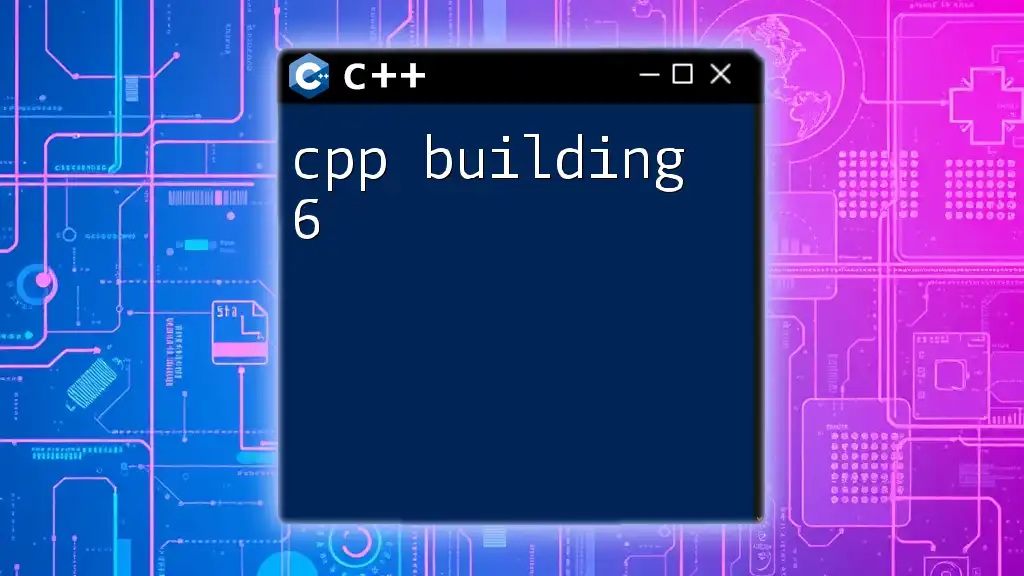
Advanced C++ Build Techniques
Using Makefile
A Makefile is a powerful utility for managing builds. It defines rules for how to build different parts of your project. Here’s a basic example:
CC = g++
CFLAGS = -I.
all: myprogram
myprogram: main.o utils.o
$(CC) -o myprogram main.o utils.o
main.o: main.cpp
$(CC) -c main.cpp $(CFLAGS)
utils.o: utils.cpp
$(CC) -c utils.cpp $(CFLAGS)
To run this Makefile, simply type:
make
Utilizing CMake for Building
CMake serves as an advanced build system that simplifies the management of complex builds. Here’s how to create a basic `CMakeLists.txt` file for your project:
cmake_minimum_required(VERSION 3.10)
project(MyProject)
set(CMAKE_CXX_STANDARD 11)
add_executable(myprogram main.cpp utils.cpp)
To build your project with CMake:
- Create a build directory:
mkdir build cd build
- Invoke CMake from within the build directory:
cmake ..
- Compile the project:
make
Debugging Your Build
No build process is free of errors. Understanding common build errors and how to address them is vital for a smooth development experience.
- Compilation Errors: Often occur due to syntax issues or undeclared variables.
- Linker Errors: Generally arise when there are unresolved references during the linking stage, such as missing functions or libraries.
To debug effectively, leverage debug flags in your compiler. For instance, compiling with the `-g` flag enables debugging information:
g++ -g -o myprogram main.cpp
Utilize debuggers like gdb to step through your code and identify problem areas.
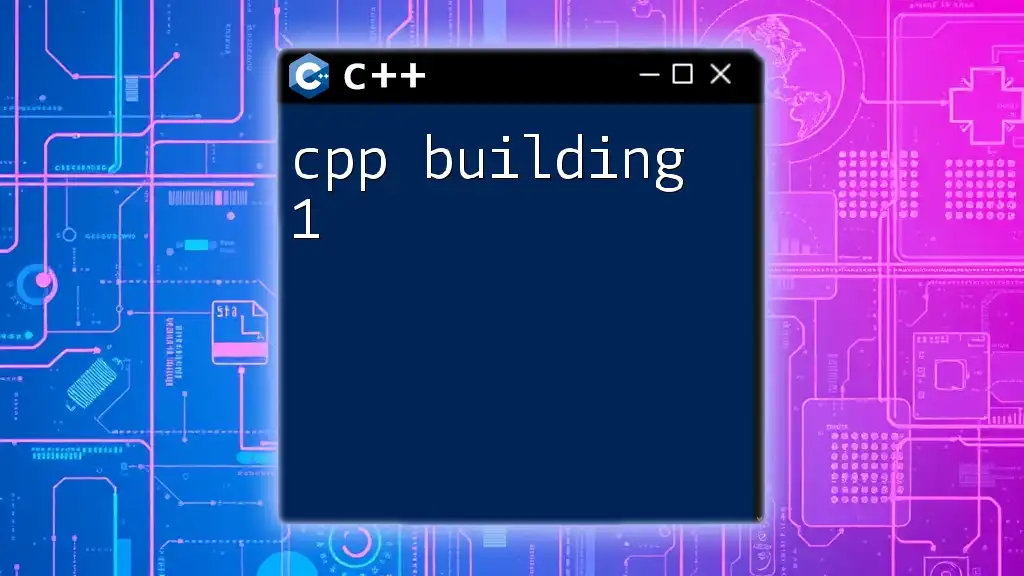
Conclusion
Understanding the intricacies of C++ building is essential for any developer looking to advance their skills. From grasping the build process to writing effective Makefiles and handling debugging, mastering these elements will significantly improve your proficiency in C++. Expand your learning through resources like books, online courses, and coding communities—all contributors to your journey in C++. Remember, experimentation is key; don’t hesitate to apply what you’ve learned in your own projects!