"CPP Building 5" refers to compiling and executing a basic C++ program that demonstrates the core concepts of C++ through a simple example.
Here’s a quick code snippet that showcases a basic C++ program which outputs "Hello, World!":
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding cpp: The Basics
What is C Preprocessor (cpp)?
The C preprocessor, commonly known as cpp, is a powerful tool used in C and C++ programming languages to process source code before compilation. It essentially prepares the code for the compiler by performing tasks such as macro expansion, file inclusion, and conditional compilation. The cpp operates on a line-by-line basis, making it a crucial step in the compilation process.
Why Use cpp?
Using cpp offers several advantages:
- Code Simplification: By defining macros, you can simplify complex expressions and repetitive code, thus enhancing maintainability.
- Conditional Compilation: With cpp, you can compile code selectively. This is particularly useful for platform-specific code or debugging.
- File Management: cpp allows you to include headers and other source files easily, fostering better organization of your codebase.
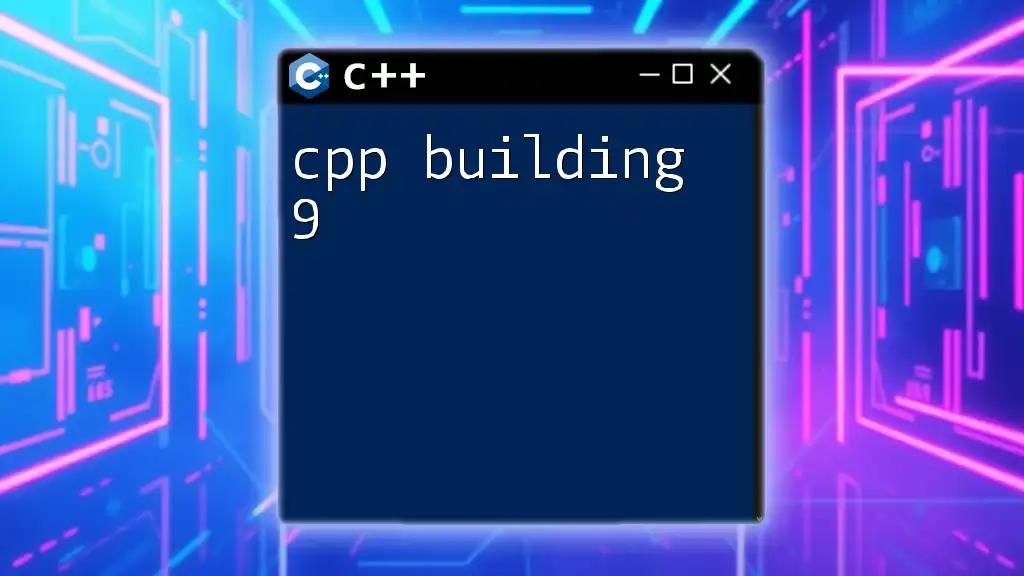
Exploring Building 5
What Does "Building 5" Mean?
"Building 5" refers to a set of practices and commands conducted within the cpp framework that enables efficient code preparation and compilation. This concept focuses on reducing complexity and streamlining the code build process, particularly in large projects.
Components of Building 5
Building 5 involves several essential components:
- Configuration Files: These files dictate how cpp should process the code, including settings for macro definitions and file inclusions.
- Preprocessed Files: The result of cpp processing, where all macros have been expanded and include files are merged.
- Linkers and Object Files: These are crucial for compiling the final executable, integrating all dependencies and source files.
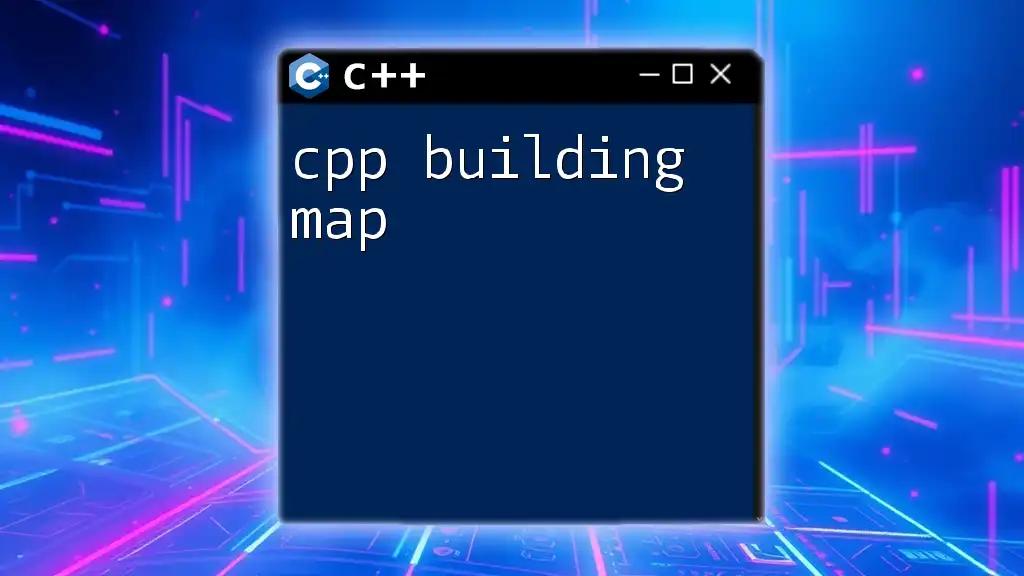
Setting Up Your Environment for Building 5
Required Software and Tools
To effectively apply cpp building techniques, you should have the necessary tools:
- A C/C++ Compiler: GCC (GNU Compiler Collection) or Clang are popular choices.
- Integrated Development Environment (IDE): Tools like Visual Studio, Code::Blocks, or any text editor with terminal capabilities will suffice.
Configuring Your Compiler for Building 5
Setting up your compiler for Building 5 involves a few straightforward steps. For instance, if you are using GCC on a Unix-based system, you can use the following command to compile a cpp file:
g++ -E my_file.cpp -o preprocessed_file.cpp
This command uses the `-E` flag to tell the compiler to run the cpp stage only, producing a preprocessed output file.
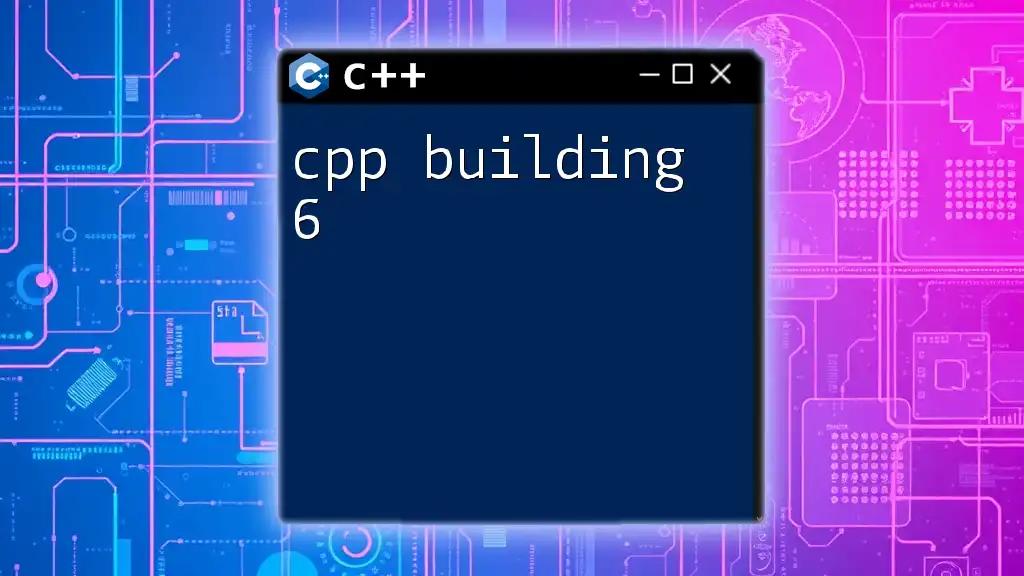
Core cpp Commands for Building 5
Macro Definition and Usage
Macros are fundamental in cpp, allowing you to define snippets of code that can be reused. You can define a simple macro using the `#define` directive:
#define SQUARE(x) ((x) * (x))
Using the above macro in your code becomes straightforward:
int area = SQUARE(5); // Equivalent to ((5) * (5))
The expanded code will replace `SQUARE(5)` with `((5) * (5))`, simplifying modifications and improving readability.
File Inclusion
To include external header files or other source files, cpp employs the `#include` directive. For example:
#include <iostream> // Standard library
#include "my_header.h" // Custom header
This command combines the contents of `my_header.h` into the current file, allowing access to its functions and variables.
Conditional Compilation
Conditional compilation allows sections of code to be compiled based on specific conditions. For instance:
#ifdef _DEBUG
std::cout << "Debugging Mode On" << std::endl;
#endif
This piece of code will only compile and run if `_DEBUG` is defined, which is particularly useful for toggling debugging-related code.
Compiler Optimizations
CPP also supports various compiler optimizations that can be enabled through flags at the command line. For example, the following command enables optimizations at different levels:
g++ -O2 my_file.cpp -o my_program
The `-O2` flag tells the compiler to perform a higher level of optimization, improving performance without significantly increasing compilation time.
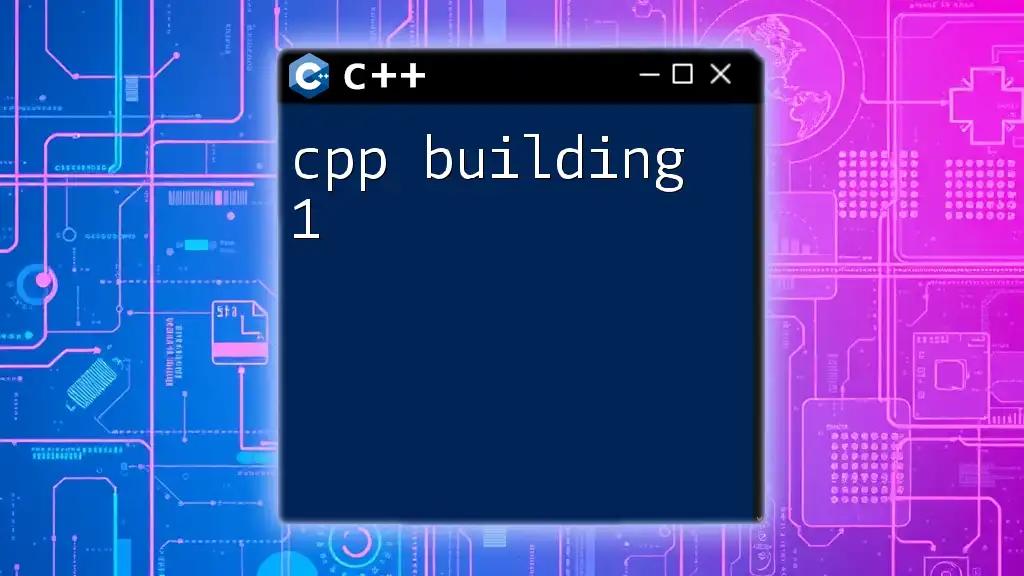
Common Pitfalls and Troubleshooting Tips
Frequently Encountered Issues
Even with the simplicity of cpp, common issues may arise, such as:
- Macro Re-definition: Redefining a macro without proper checks can lead to compiler errors.
- File Not Found Errors: Forgetting header file locations in include paths can cause compilation failures.
Simplified Troubleshooting Steps
To troubleshoot cpp issues:
- Check Macro Definitions: Ensure that macros are defined correctly and without conflict.
- Verify Include Paths: Make sure that the specified paths point to existing files.
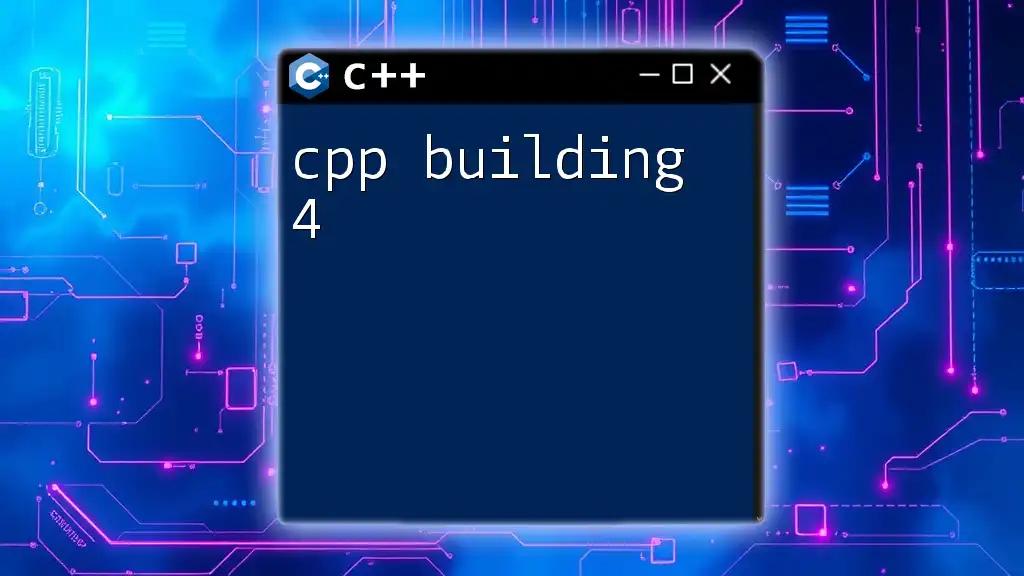
Advanced cpp Techniques for Building 5
Nested Macros and Complex Operations
Nested macros allow you to define one macro inside another. For example:
#define MULTIPLY(x, y) ((x) * (y))
#define SQUARE_AND_PRINT(x) do { std::cout << "Square: " << MULTIPLY(x, x) << std::endl; } while(0)
Using `SQUARE_AND_PRINT(5)` triggers the output of `Square: 25`.
Using cpp with External Libraries
Integrating external libraries like Boost requires a clear understanding of library paths and linking. A common command might look like:
g++ my_file.cpp -o my_program -I/path/to/boost -L/usr/local/lib -lboost_system
Here, `-I` adds include paths, `-L` specifies library directories, and `-l` links to the Boost system library.
Automating Builds with cpp
Automation tools such as Makefiles or CMake can significantly accelerate your Building 5 process. A simple `Makefile` example for cpp might look like:
all: my_program
my_program: my_file.o
g++ my_file.o -o my_program
my_file.o: my_file.cpp
g++ -c my_file.cpp
Running `make` in your terminal will compile and link your program automatically with a single command.
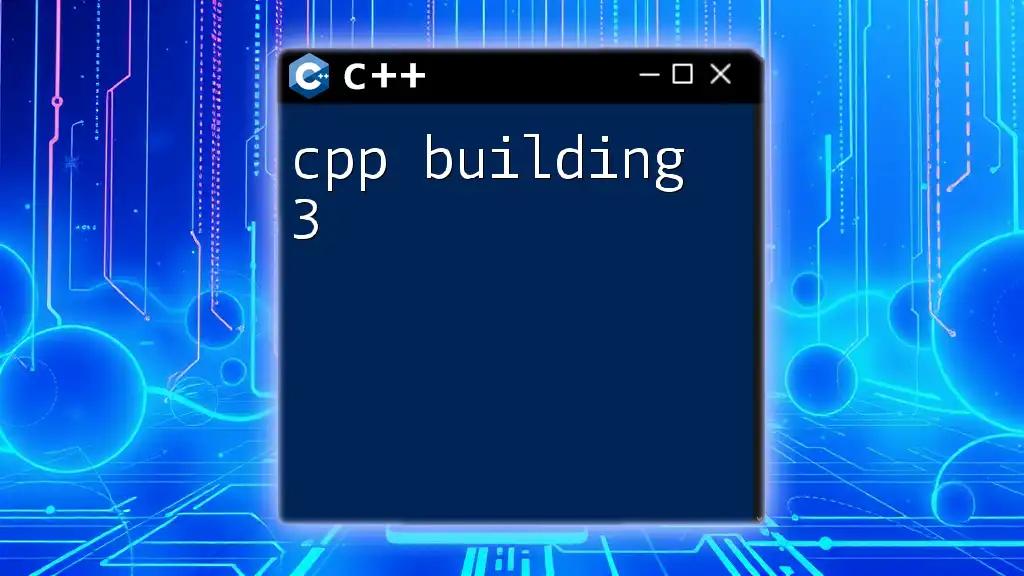
Conclusion
The cpp building process is essential for any C or C++ developer looking to streamline and optimize their code. Understanding these commands not only improves code maintainability but also enhances compilation performance. By mastering the practice of cpp building, particularly with the Building 5 framework, you can significantly contribute to a more efficient and organized coding environment.
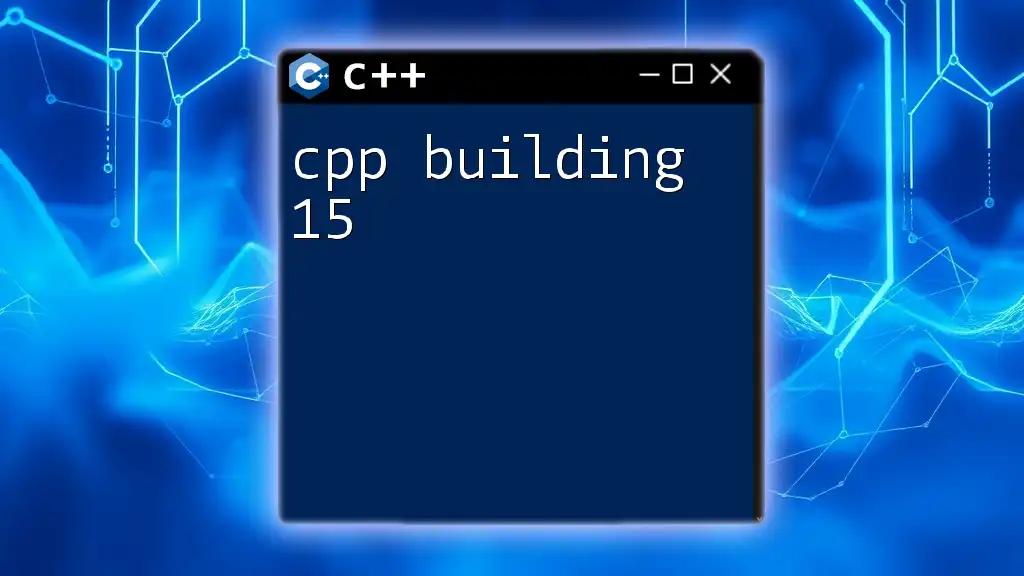
Call to Action
Dive deeper into cpp by enrolling in our courses or subscribing to our newsletter for ongoing tips and updates. Explore additional resources and tutorials that will elevate your cpp command skills and open up new programming possibilities!