"Building in C++ typically refers to the process of compiling, linking, and generating an executable from your C++ source code, and in this context, 'cpp building 7' could imply the seventh iteration or step in your building process."
Here's an example of a simple C++ build command using g++:
g++ -o my_program main.cpp
Setting Up the Environment
Choosing the Right IDE
Selecting the appropriate Integrated Development Environment (IDE) is crucial for mastering C++ commands and efficiently implementing cpp building 7 methodologies. Here are some popular IDEs that facilitate C++ development:
-
Visual Studio: This IDE is widely acclaimed for its robust features and user-friendly interface. It provides tools that significantly simplify the debugging process and offers seamless integration with the Microsoft ecosystem.
-
Code::Blocks: A versatile, open-source IDE that is lightweight and customizable. It's perfect for beginners looking to get started quickly without unnecessary complications.
-
CLion: Known for its intelligent code assistance capabilities, this JetBrains product supports CMake, making it suitable for complex C++ projects.
When installing any of these IDEs, follow the detailed installation steps provided on their official sites. For Visual Studio, ensure you include the C++ development workload during the initial setup. For Code::Blocks, installing a compatible compiler like MinGW is essential for the smooth execution of your C++ programs.
Compilers Overview
Understanding compilers is just as important as choosing the right IDE. The three leading C++ compilers are GCC, MSVC, and Clang.
-
GCC (GNU Compiler Collection): Known for its portability and adherence to the C++ standard. Providing excellent support for cross-platform development, it's a favorite among open-source programmers.
-
MSVC (Microsoft Visual C++): Best suited for Windows applications. It has strong debugging capabilities but can sometimes deviate from standard compliance.
-
Clang: Distinguished for its fast compilation speed and modern architecture. Clang emphasizes high-performance and is often preferred for developing performance-critical applications.
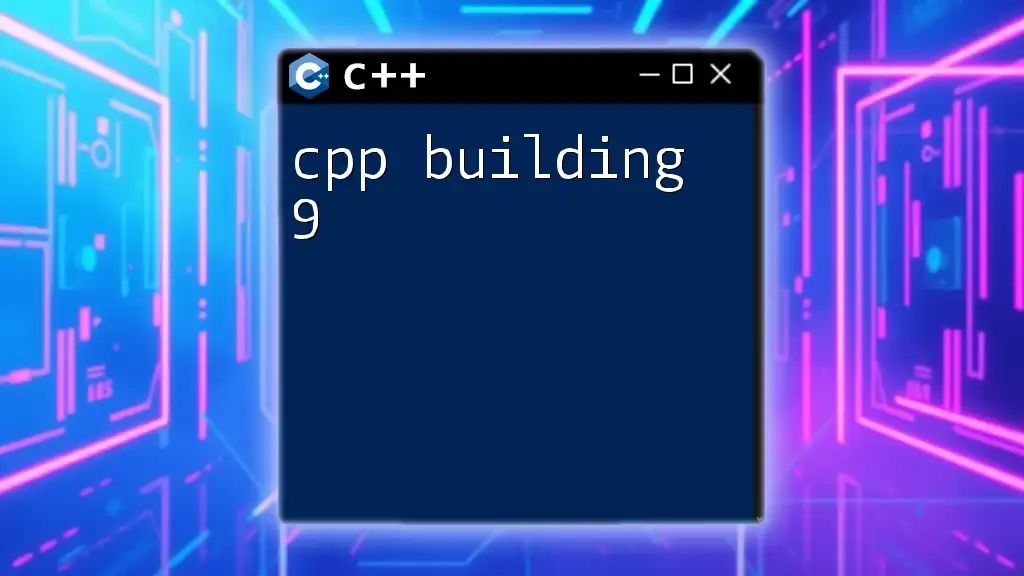
Understanding C++ Building Blocks
Core Concepts of C++
To effectively navigate cpp building 7, grasping core concepts is essential.
Variables and Data Types form the basis of every C++ program. C++ supports various data types such as `int`, `double`, `char`, and `bool`. Here's a quick example illustrating their implementation:
int age = 25;
double height = 5.9;
char initial = 'A';
bool isStudent = true;
By understanding how to declare and utilize these variables, you can control data within your applications efficiently.
Control Structures
Control structures guide the flow of a program based on specific conditions.
Conditional Statements are fundamental in determining how a program behaves under different scenarios. The following code snippet showcases how to use conditional statements effectively:
if (age > 18) {
cout << "Adult";
} else {
cout << "Minor";
}
Here, the output will vary based on the value of the `age` variable, demonstrating how to introduce branching logic into your programs.
Loops like `for` and `while` allow code blocks to be executed multiple times, streamlining repetitive tasks. Here's an example of a for loop in action:
for (int i = 0; i < 5; i++) {
cout << "Iteration " << i << endl;
}
This loop runs five times, showcasing how iterative processes work—essential for tasks like traversing arrays or executing repetitive algorithms.
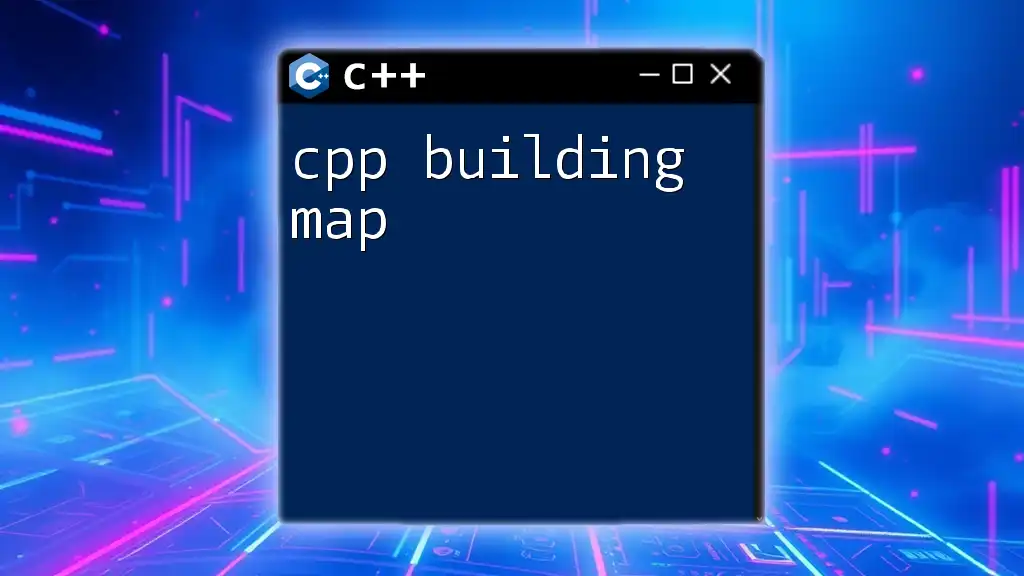
Building Classes and Objects
Introduction to Object-Oriented Programming
Object-Oriented Programming (OOP) is a paradigm that uses "objects" to model real-world entities. It allows for better organization and reusability of code.
Creating a Simple Class is a pivotal step in working with OOP in C++. In the following example, we define a class named `Car`, encapsulating its properties and behaviors:
class Car {
public:
Car(string name) : model(name) {}
void showModel() { cout << "Model: " << model << endl; }
private:
string model;
};
In this class, `Car` has a constructor for initializing its state and a method to display the model name. Understanding how to create and manipulate classes is crucial in cpp building 7 as it enhances code modularity.
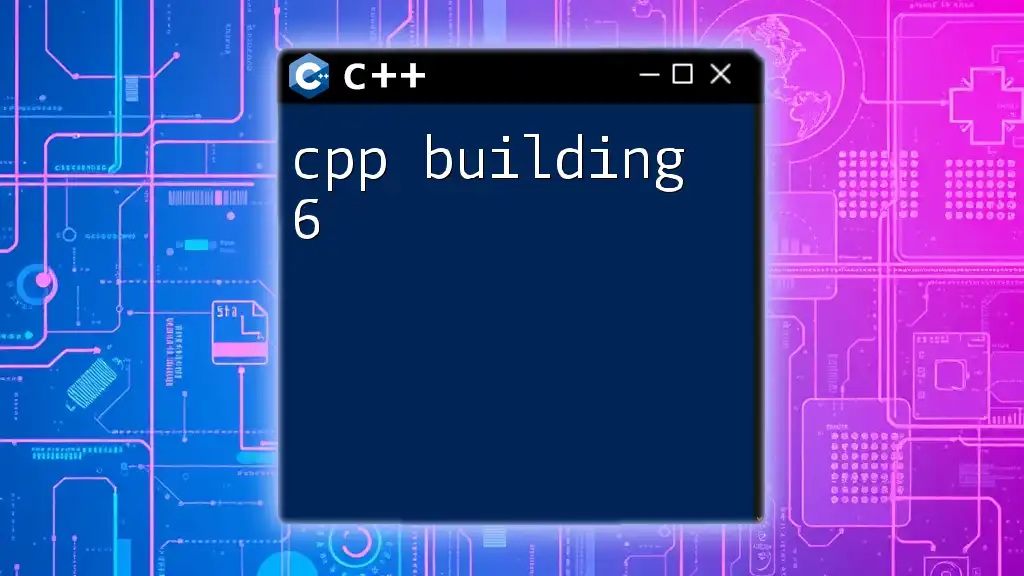
Mastering C++ Commands
Essential Cmds for Building in C++
C++ is rich in commands and functions, many of which come from standard libraries. It's vital to familiarize yourself with these to streamline your programming efforts.
Common Functions and Libraries allow developers to leverage prebuilt code to perform complex tasks without starting from scratch. For instance, the `cmath` library provides essential mathematical functions:
#include <cmath>
double squareRoot = sqrt(16);
In this example, `sqrt` computes the square root, illustrating how standard libraries can simplify calculations.
Advanced Commands for Building
To augment your programming skills, it's crucial to master templates and generics. Templates enable developers to create functions and classes that can operate on various data types, promoting reusability and flexibility. Consider this function template example:
template <typename T>
T add(T a, T b) {
return a + b;
}
In this template, `add` can take any data type, allowing it to perform addition on integers, doubles, or even user-defined types.
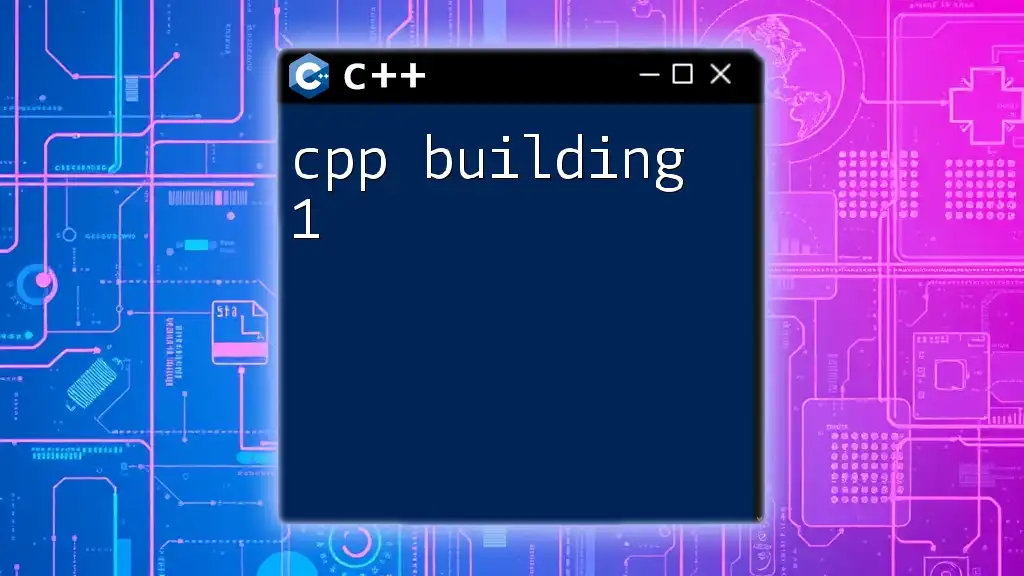
Debugging and Error Handling
Common Errors and How to Fix Them
When writing C++ code, developers often encounter errors. It's essential to recognize the difference between compilation errors and runtime errors.
Compilation Errors occur when the code is syntactically incorrect, preventing the code from being compiled. Common examples include misspelled keywords or missing semicolons.
Runtime Errors, on the other hand, manifest during execution. For instance, accessing an out-of-bounds index in an array can lead to runtime failures. Understanding these distinctions will help you debug effectively.
Debugging Tools
Each IDE generally comes equipped with its own debugging tools. Using breakpoints strategically allows you to pause program execution at critical points to inspect variable values and flow control. Mastering debugging tools can significantly streamline your development workflow.
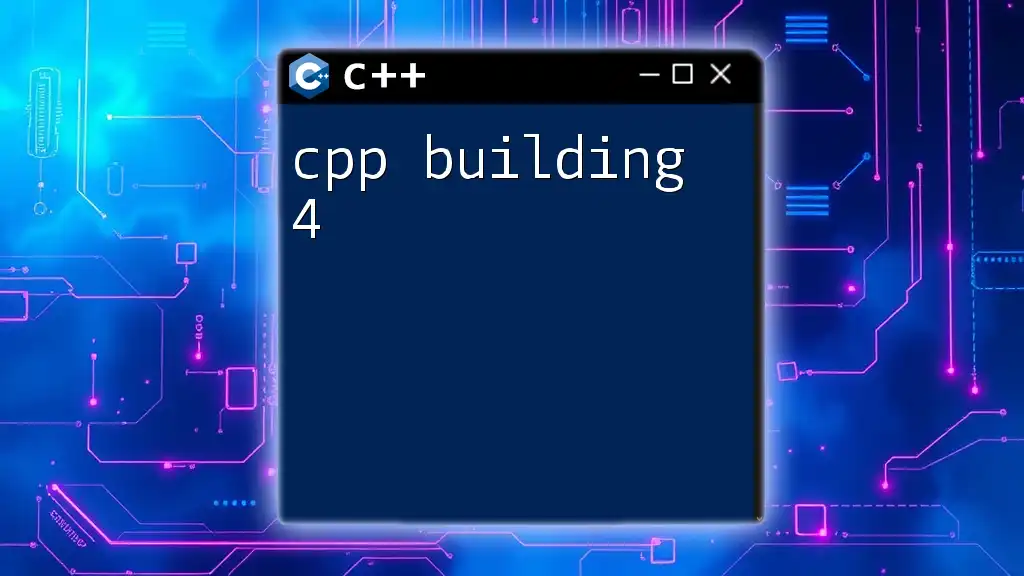
Best Practices for C++ Development
Writing Clean and Maintainable Code
Writing clear and organized code is vital for long-term project manageability. Adopting naming conventions—such as using camelCase for variables and PascalCase for classes—improves code readability.
Additionally, comments and documentation enhance understanding for yourself and others who may work on your code later. Clear comments can describe the purpose of complex functions or elaborate on algorithm logic, making collaboration easier.
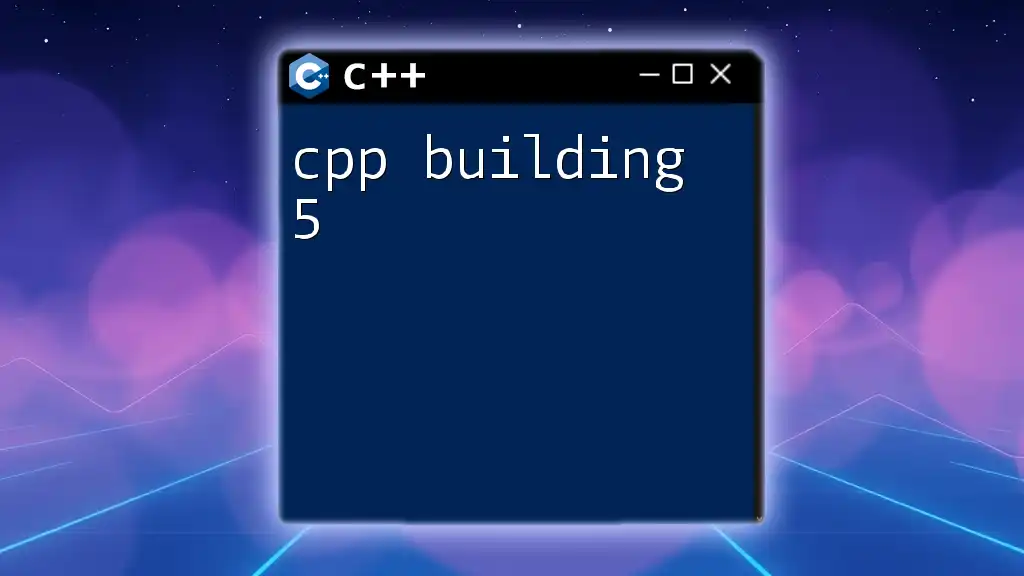
Conclusion
Mastering cpp building 7 involves not only learning commands but also understanding the underlying principles of C++. The journey requires dedication to continuous learning and practice.
Engage with the community, explore online resources, and commit to improving your skills. With time and effort, you'll not only understand C++ better but also appreciate its power in solving complex programming challenges.
Additional Resources
For further learning, check out online tutorials, forums, and recommended books that dive deeper into C++ programming concepts and practices. The journey may be intense, but the rewards of mastering cpp building 7 are well worth the effort!