C++ offers high-performance capabilities and fine-grained control over system resources, making it ideal for software development that demands efficiency and speed.
#include <iostream>
int main() {
std::cout << "Hello, C++ Benefits!" << std::endl;
return 0;
}
Key Benefits of C++
Versatility and Flexibility
Multi-Paradigm Language
C++ stands out as a multi-paradigm programming language, meaning it supports various programming styles such as procedural, object-oriented, and generic programming. This flexibility allows developers to choose the paradigm that best suits their project needs.
For example, consider the task of creating a simple shape representation in different paradigms:
// Procedural approach
struct Circle {
double radius;
};
void printArea(Circle c) {
std::cout << "Area: " << 3.14 * c.radius * c.radius << std::endl;
}
// Object-oriented approach
class Circle {
private:
double radius;
public:
Circle(double r) : radius(r) {}
double area() {
return 3.14 * radius * radius;
}
};
// Generic approach with templates
template<typename T>
double area(T shape) {
return 3.14 * shape.radius * shape.radius;
}
Platform Independence
C++ code is known for its platform independence, which means that it can be compiled and run on different operating systems with minimal changes. The compiler translates the code into machine-specific instructions, maximizing its portability.
Here is a simple C++ program that prints "Hello, World!", which can be compiled and executed on any system supporting C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Performance Efficiency
Low-Level Memory Manipulation
One of the major advantages of C++ is its ability to perform low-level memory manipulation. Pointers and references allow programmers direct access to memory addresses, leading to efficient memory management and performance optimizations.
For instance, using pointers in C++:
#include <iostream>
void updateValue(int* p) {
*p = 20;
}
int main() {
int value = 10;
updateValue(&value);
std::cout << "Updated Value: " << value << std::endl; // Output: 20
return 0;
}
Speed of Execution
C++ offers exceptional speed of execution by compiling closely to machine language, making it a preferred choice for time-sensitive applications. Benchmarks often demonstrate C++ running significantly faster than interpreted languages like Python or Java due to reduced overhead.
Strong Object-Oriented Features
Data Abstraction
C++ promotes data abstraction through encapsulation, allowing developers to hide data and expose only the necessary interface. This strengthens the integrity of the data and minimizes the risk of unintended interference.
A basic example illustrates the encapsulation of data:
class BankAccount {
private:
double balance;
public:
void deposit(double amount) {
balance += amount;
}
double getBalance() {
return balance;
}
};
Inheritance
C++ employs inheritance, a fundamental feature that allows developers to create new classes using existing ones. This fosters code reusability and facilitates polymorphism, enabling objects to be treated as instances of their parent class.
Here's a simple example of inheritance:
class Animal {
public:
void speak() {
std::cout << "Animal speaks!" << std::endl;
}
};
class Dog : public Animal {
public:
void bark() {
std::cout << "Dog barks!" << std::endl;
}
};
Dynamic Binding
C++ supports dynamic binding, allowing decision-making regarding method calls to occur at runtime, enhancing flexibility and extensibility.
Consider the example below, which uses virtual functions:
class Shape {
public:
virtual void draw() {
std::cout << "Drawing Shape" << std::endl;
}
};
class Circle : public Shape {
public:
void draw() override {
std::cout << "Drawing Circle" << std::endl;
}
};
void render(Shape* s) {
s->draw(); // Method call is determined at runtime.
}
Extensive Standard Library
Rich Set of Libraries
The C++ Standard Template Library (STL) provides a plethora of libraries that simplify various tasks such as algorithms and data structures. With containers (like vectors, lists, and maps) and algorithms readily available, developers can focus on solving complex problems rather than implementing common functionalities from scratch.
For example, here's a demonstration of `std::vector`:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
Customizable Code
C++ templates enable generic programming, allowing developers to write code that works with any data type. This feature promotes code efficiency and reduces redundancy.
Example of a simple template function:
template<typename T>
T add(T a, T b) {
return a + b;
}
int main() {
std::cout << add(5, 10) << std::endl; // Output: 15
std::cout << add(5.5, 2.5) << std::endl; // Output: 8.0
return 0;
}
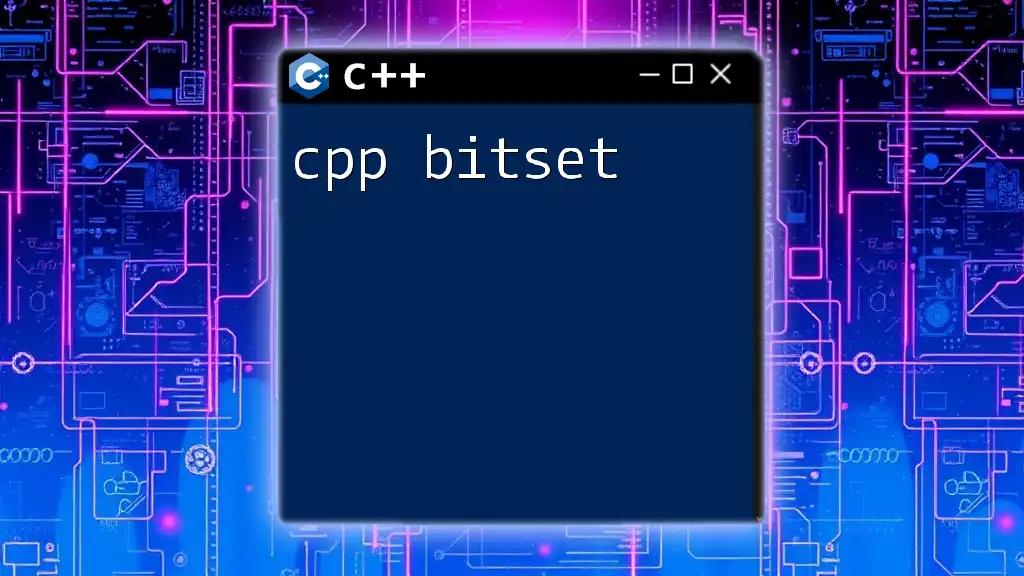
Industry Applications of C++
Game Development
C++ is a staple in game development, particularly in high-performance engines like Unreal Engine. The language's ability to handle complex graphics and real-time interactions makes it ideal for this domain.
A simple game loop in C++ might look like this:
while (gameRunning) {
handleInput();
updateGameLogic();
render();
}
Systems Programming
C++ is extensively used in systems programming, including operating systems and hardware interfaces. This capability arises from C++'s low-level memory manipulation and performance efficiency, which are crucial for systems that require tight resource control.
Here's an example illustrating basic driver functionalities:
void driverFunction() {
// Hardware interrupt handling
// Communicate with hardware through memory-mapped I/O
}
Real-time Systems
In areas like robotics and control systems, C++ excels due to its performance and real-time capabilities. The deterministic behavior and resource management afforded by C++ make it a perfect fit for such applications.
A simple control loop might be structured like this:
while (true) {
readSensors();
computeControlSignals();
applyControl();
}

Community and Ecosystem
Support and Resources
C++ has a robust ecosystem supported by a vast community. Numerous online forums, documentation resources, and educational platforms make it easier to learn and troubleshoot. Engaging with these communities fosters growth and development among C++ programmers.
Open Source Contributions
Many developers contribute to open source C++ projects, enhancing the language's functionality and accessibility. Joining these projects not only helps improve personal skills but allows programmers to give back to the community while working on meaningful initiatives.
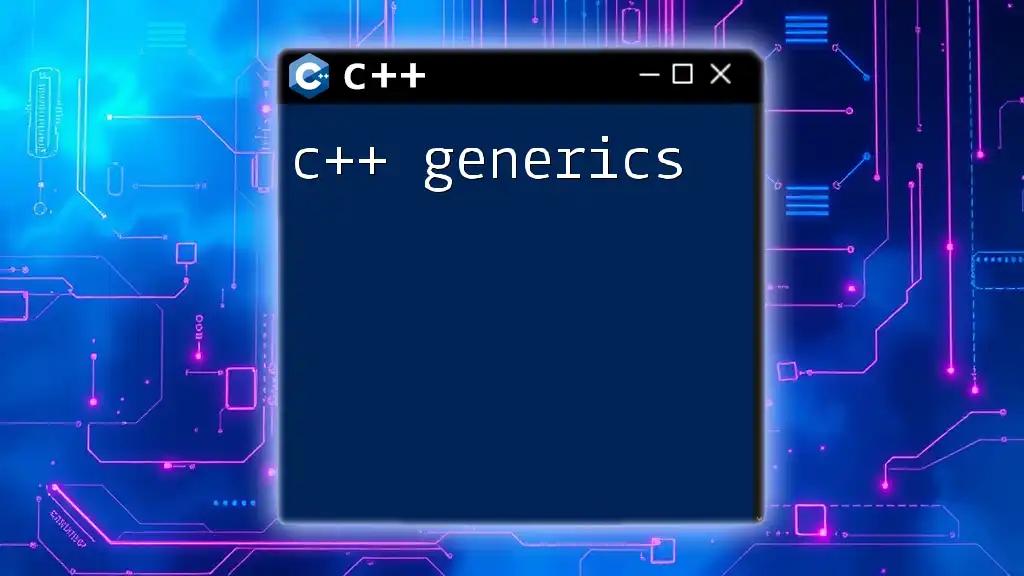
Conclusion
In summary, cpp benefits encompass versatility, performance efficiency, strong object-oriented capabilities, and a rich standard library, making C++ a preferred choice across various domains. Whether you are drawn to game development, systems programming, or real-time application development, learning C++ can pave the way for a successful career in programming.
So why wait? Start your C++ journey today and explore the numerous opportunities waiting for you in the tech world!
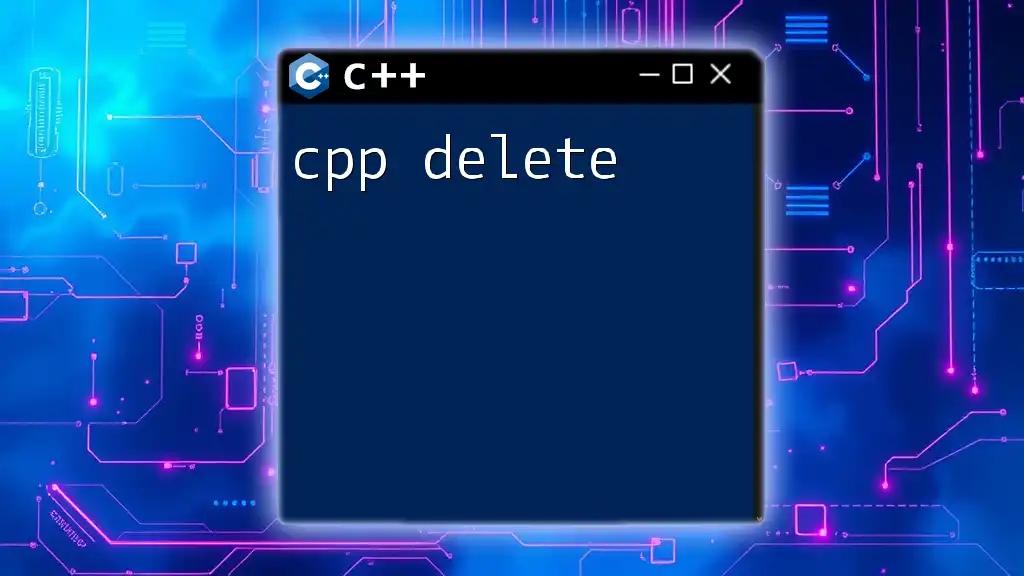
Additional Resources
For those looking to deepen their understanding of C++, a selection of books, tutorials, and courses is available. Engaging in coding challenges on various platforms can provide practical experience to complement theoretical knowledge.