C++ (or cpp) is a high-level programming language that supports object-oriented, procedural, and generic programming, making it versatile for various software development tasks.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is C++?
Understanding the Basics
C++ is a general-purpose programming language that was developed by Bjarne Stroustrup in the early 1980s as an enhancement to the C programming language. The primary goal was to add support for object-oriented programming (OOP), which allows for better organization, reusability, and scalability of code.
The evolution of C++ has made it a powerful tool for software development, offering developers the ability to create applications that range from simple scripts to complex systems. The essence of C++ lies in its strong efficiency and flexibility, making it ideal for high-performance applications.
Key Features of C++
C++ boasts several key features that set it apart and enhance its functionality:
-
Object-Oriented Programming: C++ is built around the concept of classes and objects, allowing developers to model real-world entities in software. This includes:
- Encapsulation: Bundling of data and methods that operate on the data.
- Inheritance: Creating new classes from existing ones for reusability.
- Polymorphism: Using functions in different forms.
-
Standard Template Library (STL): A powerful feature of C++, STL provides a rich set of data structures and algorithms. Developers can leverage templates to create generic classes and functions that can work with any data type.
-
Memory Management: C++ provides low-level memory manipulation capabilities, allowing for efficient memory use. This includes the use of:
- Pointers: Variables that store memory addresses, giving programmers direct access to memory.
- Dynamic memory allocation via `new` and `delete`, enabling flexible memory use based on program requirements.
-
Platform Independence: C++ code can be compiled on various platforms, making it versatile and widely applicable in software development.
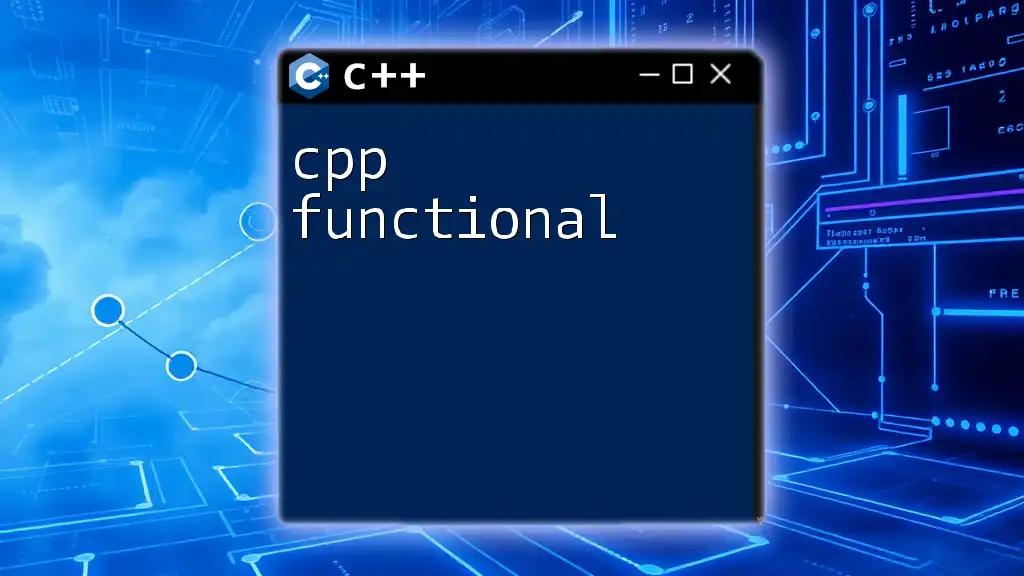
C++ Syntax Overview
Basic Syntax Structure
Understanding the syntax of C++ is crucial for any developer. The basic structure of a C++ program follows a simple format, and here’s a foundational example:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
In this example:
- `#include <iostream>` includes the input-output stream library.
- `using namespace std;` allows the use of standard functions without a prefix.
- `int main()` defines the main function where execution begins.
Data Types and Variables
C++ supports multiple data types, including:
-
Primitive Data Types:
- `int`: Used for integer values.
- `char`: Represents single characters.
- `float` and `double`: For floating-point numbers, with double offering higher precision.
-
User-Defined Data Types:
- `struct`: A way to group different data types together.
- `class`: An extension of struct that includes methods to operate on data.
- `union`: A memory-efficient structure that can store different data types in the same memory space.
- `enum`: A user-defined type where a variable can hold a set of constants.
Control Structures
Control structures guide the flow of the program based on conditions or repetitions. C++ offers various control structures:
-
Conditional Statements:
- `if`, `else`, and `switch` statements enable decision-making in code. Here’s a simple example using an `if` statement:
int x = 10; if (x > 0) { cout << "Positive number"; }
-
Loops: For repetitions, C++ uses `for`, `while`, and `do-while` loops, promoting efficient code execution:
for (int i = 0; i < 5; i++) { cout << "Iteration: " << i << endl; }
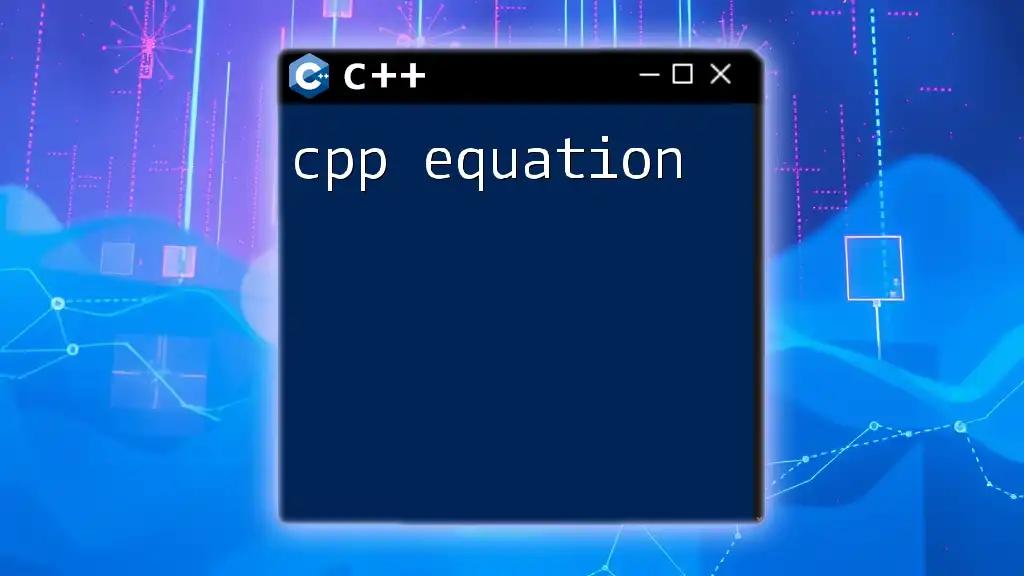
C++ Compilation Process
Understanding Compilation
The C++ compilation process transforms human-readable code into machine code through several steps:
- Preprocessing: Handling directives (e.g., `#include`) before actual compilation.
- Compiling: Translating source code into assembly code.
- Linking: Combining separate pieces of code and libraries into a single executable.
- Execution: Running the final executable program.
Role of Headers and Libraries
C++ programming heavily relies on libraries, and headers play a pivotal role. By including headers, developers can access predefined functions and classes. Commonly used libraries include:
- iostream: For input and output operations.
- vector, map: From the STL for convenient data handling.
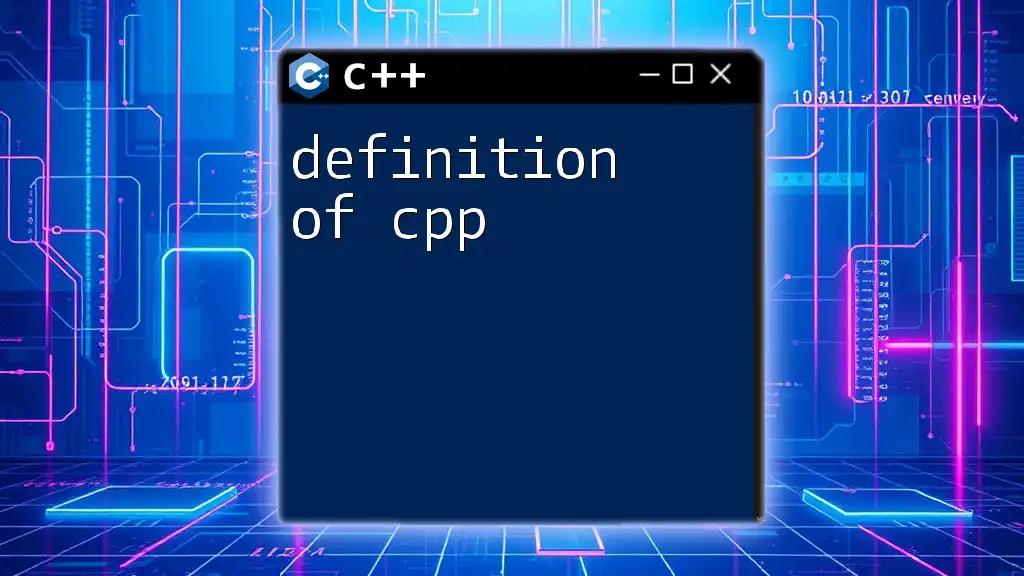
Comparing C++ with Other Programming Languages
C vs. C++
While C and C++ share a foundational syntax and similar language constructs, they differ significantly in terms of features. C is procedural-oriented, lacking several modern programming techniques found in C++. On the other hand, C++ includes support for OOP, making it more suited for large software applications where code organization is paramount.
C++ vs. Java
C++ and Java serve different purposes and utilize different paradigms. While both support OOP, they differ in memory management and syntax. C++ allows explicit memory management with pointers, whereas Java handles it through automatic garbage collection. This leads to differing performance characteristics and application suitability.
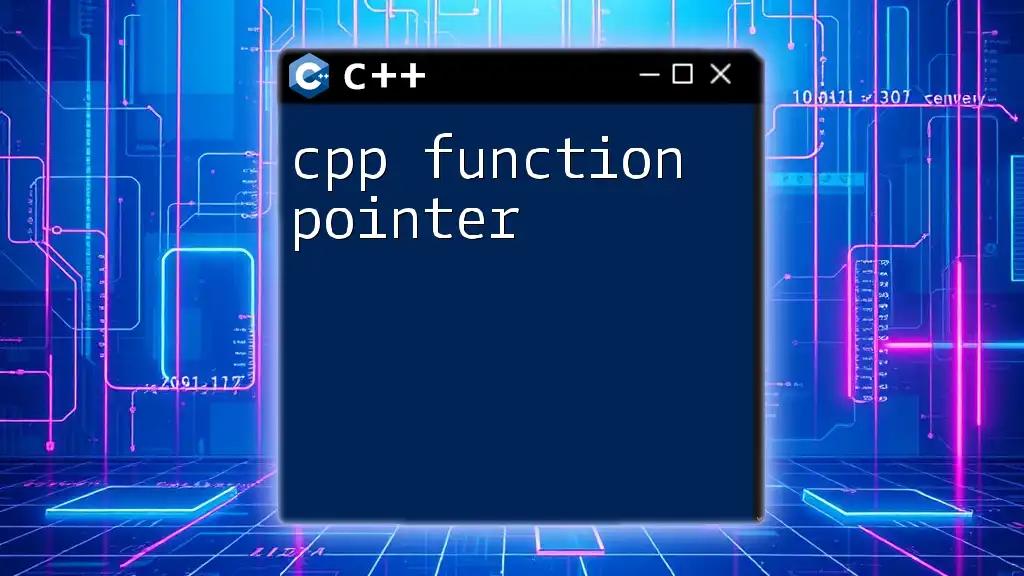
Practical Applications of C++
Where is C++ Used?
C++ is a versatile language excelling in various domains:
- Game Development: Many high-performance game engines are built in C++, facilitating real-time processing.
- Operating Systems: Core OS components and performance-critical applications often rely on C++.
- Embedded Systems: C++ efficiently supports programming for hardware devices with limited resources.
- High-Performance Applications: Applications requiring rapid data processing, such as finance and telecommunications, benefit from C++’s speed.
Examples of Popular C++ Applications
There are numerous notable applications built with C++. For instance:
- The Adobe suite (Illustrator, Photoshop)
- Major databases including MySQL and MongoDB
- Popular game engines such as Unreal Engine
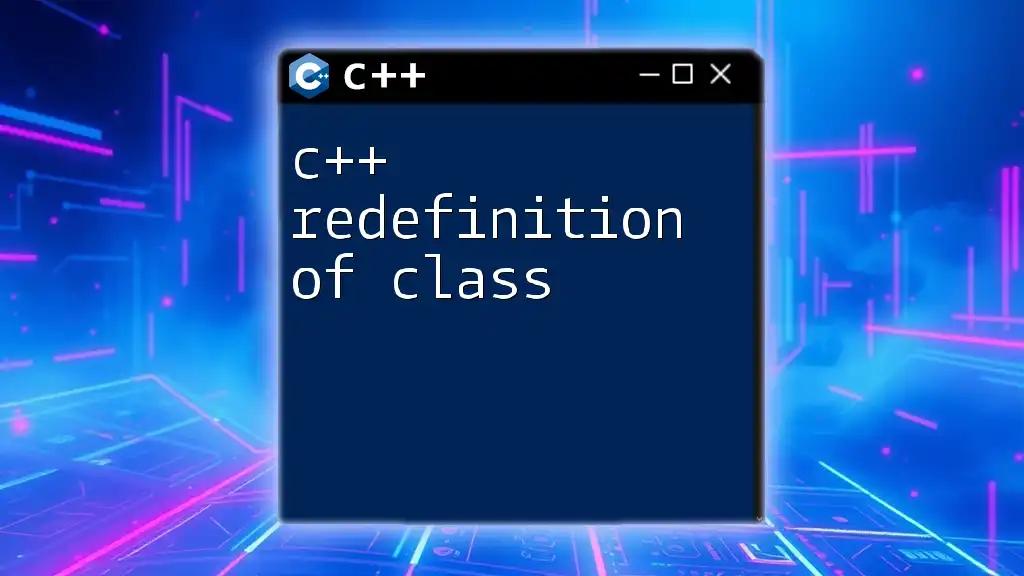
Conclusion
Understanding the cpp definition is foundational for anyone venturing into software development. C++'s unique features, flexibility, and performance make it a key language in numerous high-demand sectors. By mastering C++, developers can unlock advanced programming techniques and optimize their coding proficiency.
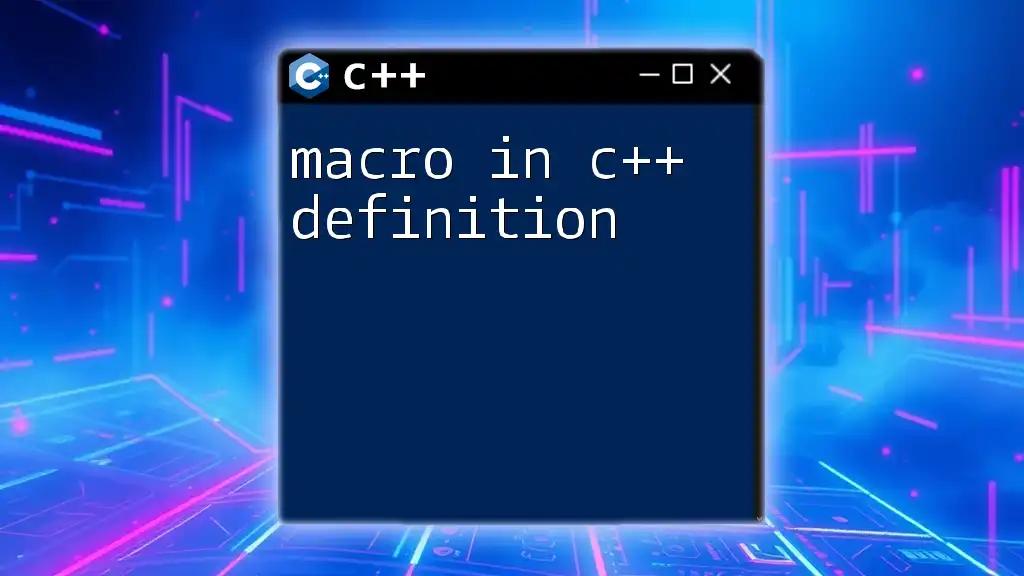
Call to Action
We encourage you to share your experiences with C++ in the comments below. Any questions or clarifications you seek will help guide your journey in mastering this powerful programming language. Don’t forget to subscribe to our platform for more actionable tutorials and tips on using C++ effectively!
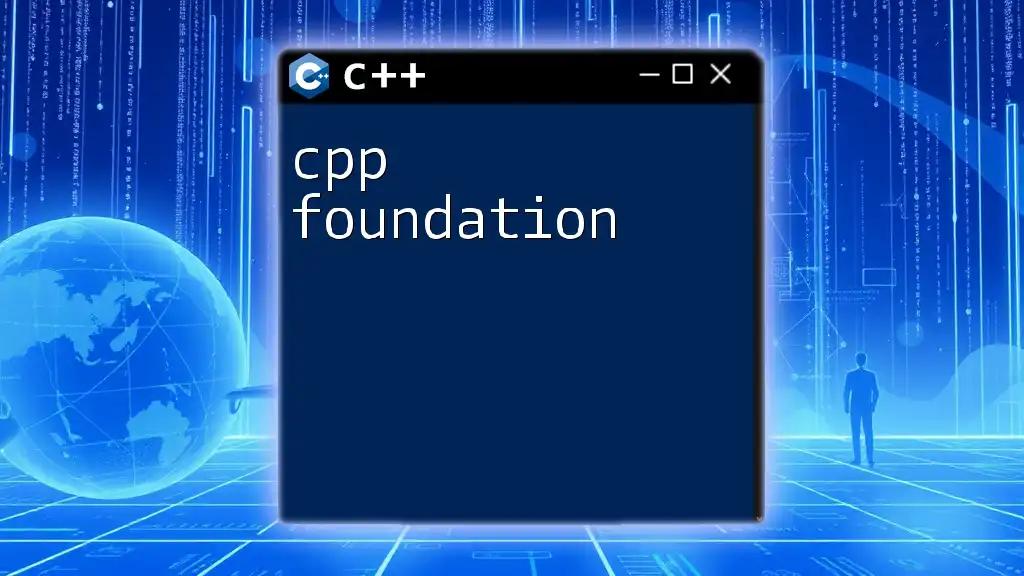
Additional Resources
For further exploration:
- Check the official C++ documentation for comprehensive guidelines.
- Engage with community forums to explore best practices and real-world applications.
- Seek online courses and interactive tutorials to deepen your understanding of C++.