C++ testing involves writing and executing tests to ensure that your code behaves as expected, typically using frameworks like Google Test for unit testing.
#include <gtest/gtest.h>
// Function to be tested
int add(int a, int b) {
return a + b;
}
// Test case for the add function
TEST(AddTest, PositiveNumbers) {
EXPECT_EQ(add(2, 3), 5);
EXPECT_EQ(add(10, 15), 25);
}
int main(int argc, char **argv) {
::testing::InitGoogleTest(&argc, argv);
return RUN_ALL_TESTS();
}
C++ Testing: A Comprehensive Guide
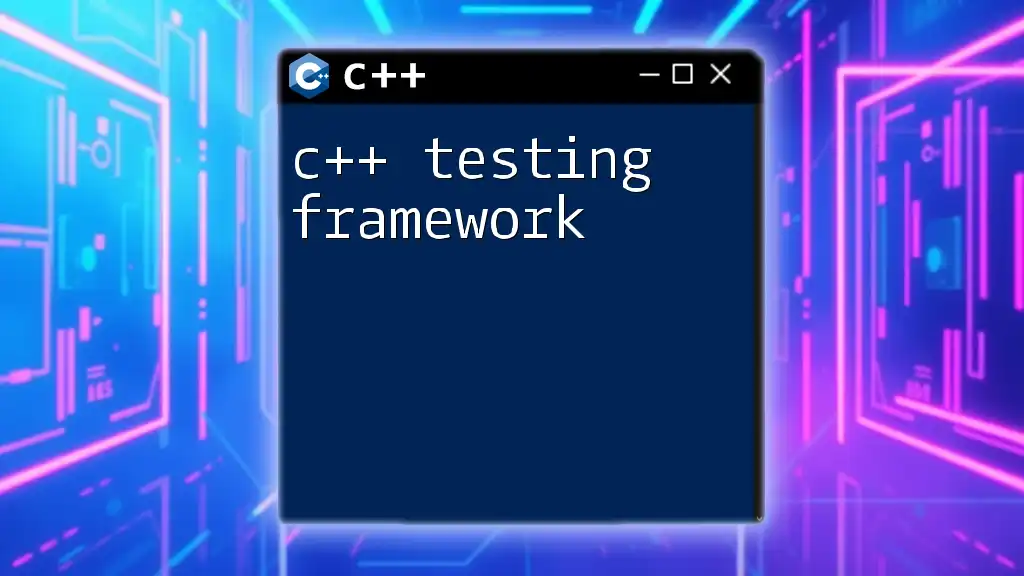
What is C++ Testing?
C++ testing refers to the process of executing and verifying the functionality of C++ code to ensure that it behaves as expected under various conditions. Testing is a critical aspect of software development because it helps identify defects and issues early in the development cycle, leading to more robust and reliable applications.
Benefits of Testing in C++ include:
- Improved Code Quality: Regular testing helps maintain high code quality.
- Facilitated Code Refactoring: Well-tested code can be refactored confidently, as the tests will catch any errors introduced by changes.
- Enhanced Collaboration: A clear testing strategy promotes better understanding and collaboration among team members.
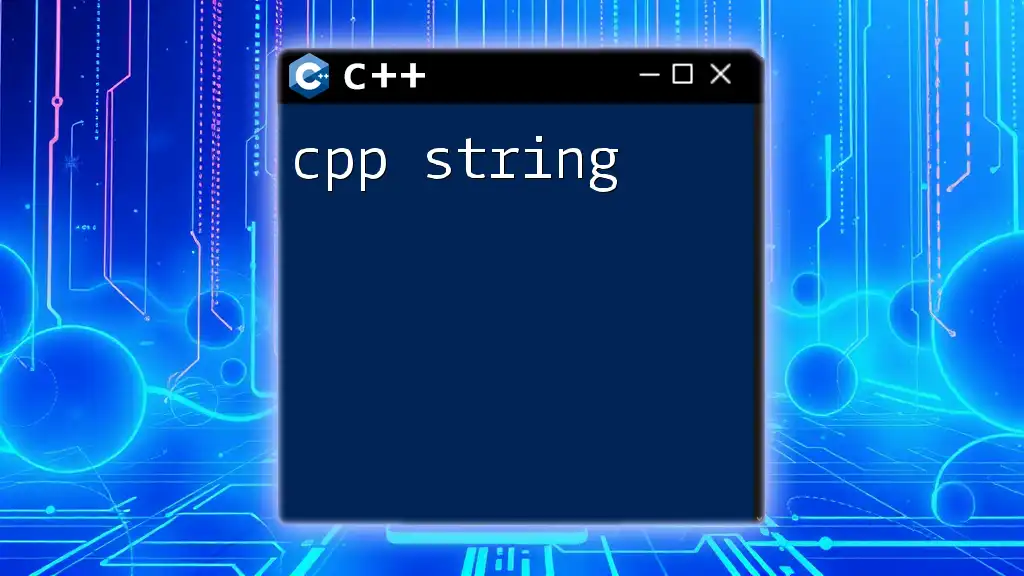
Types of Testing in Software Development
In the realm of software development, various types of testing can be employed to ensure code integrity:
Unit Testing
Focuses on individual components or functions to ensure they work correctly in isolation. It effectively tests small units of code, often resulting in faster identification of potential issues.
Integration Testing
Involves testing multiple components or systems together to ascertain that they work as intended when combined. Integration testing can unveil problems that unit testing might not capture.
System Testing
A comprehensive examination of the entire application to verify that it meets specified requirements. System testing accounts for all integrated components and checks the overall system behavior.
Acceptance Testing
Conducted to determine whether the software is ready for release. This testing is typically performed by end-users and validates that the software fulfills business requirements.
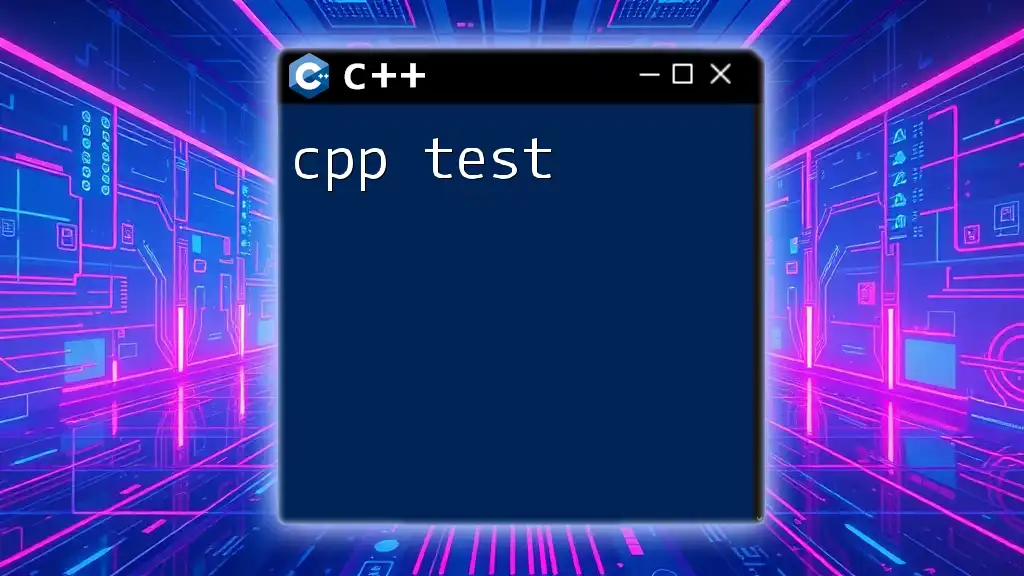
Getting Started with C++ Testing
Choosing a Testing Framework
Selecting the right testing framework is crucial for efficient C++ testing. Several popular frameworks are widely used:
- Google Test (gtest): A powerful and flexible C++ testing framework widely adopted in the industry.
- Catch2: Known for its ease of use and expressive syntax, Catch2 follows a Behavior-Driven Development (BDD) approach.
- Boost.Test: Part of the Boost libraries, this framework provides a rich set of functionalities but may require familiarity with the Boost ecosystem.
When choosing a framework, consider aspects such as simplicity, community support, and compatibility with your project structure.
Setting Up Your Testing Environment
Installation of Testing Frameworks
Step-by-Step Guide for Google Test:
- Dependencies: Ensure you have CMake and a C++ compiler installed on your machine.
- Installation: Clone the Google Test repository from GitHub and build it.
Here's a sample command to get started with Google Test:
git clone https://github.com/google/googletest.git
cd googletest
mkdir build; cd build
cmake ..
make
Make sure you have included the gtest library in your project’s include path in your IDE or CMake settings.
Basic File Structure for C++ Testing
Organizing your test files properly enhances maintainability. A common directory structure might look like:
/project_root
/src // Your C++ source files
/include // Header files
/tests // Your test files
test_sample.cpp // Tests for sample components
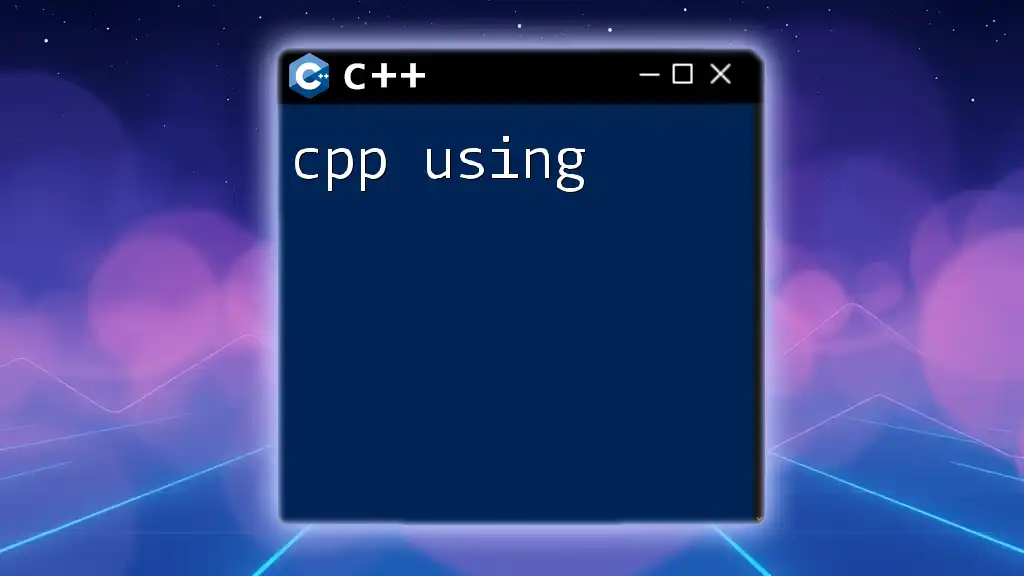
Writing Your First Test Case
Understanding Test Cases
A test case is a single scenario that checks a specific aspect of your code. Each test case generally consists of three parts: Arrange (set up the necessary objects and states), Act (execute the code you want to test), and Assert (check the result).
Example: Writing a Simple Test Using Google Test
Below is an example of a simple test case using Google Test, which verifies that a function returns the expected value:
#include <gtest/gtest.h>
#include "sample.h" // Assume this header defines the function we're testing.
TEST(SampleTest, FunctionReturnsCorrectValue) {
EXPECT_EQ(sampleFunction(2), 4);
}
In the example, `EXPECT_EQ` asserts that the result of `sampleFunction(2)` should equal `4`.
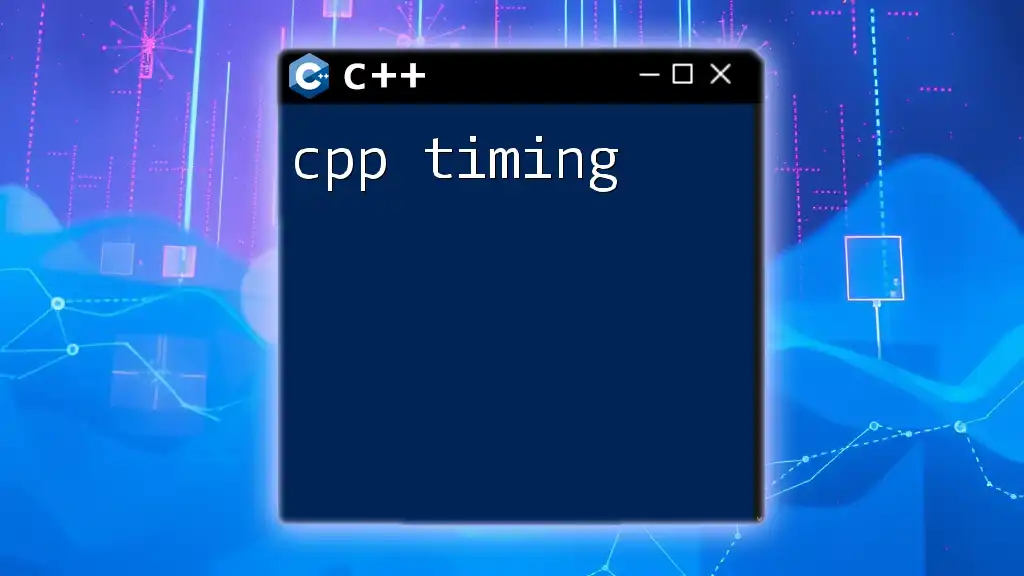
Running Your Tests
Executing Tests from the Command Line
To run your tests using Google Test, compile your tests along with the Google Test library in the command line. Then, execute the generated binary to see the results.
Example command to compile and run tests:
g++ -o runTests test_sample.cpp -lgtest -lpthread
./runTests
Using IDEs for Running Tests
Many Integrated Development Environments (IDEs) provide built-in support for running tests. For instance, in Visual Studio, the Test Explorer can help manage and run your tests effortlessly by detecting your Test Cases automatically.
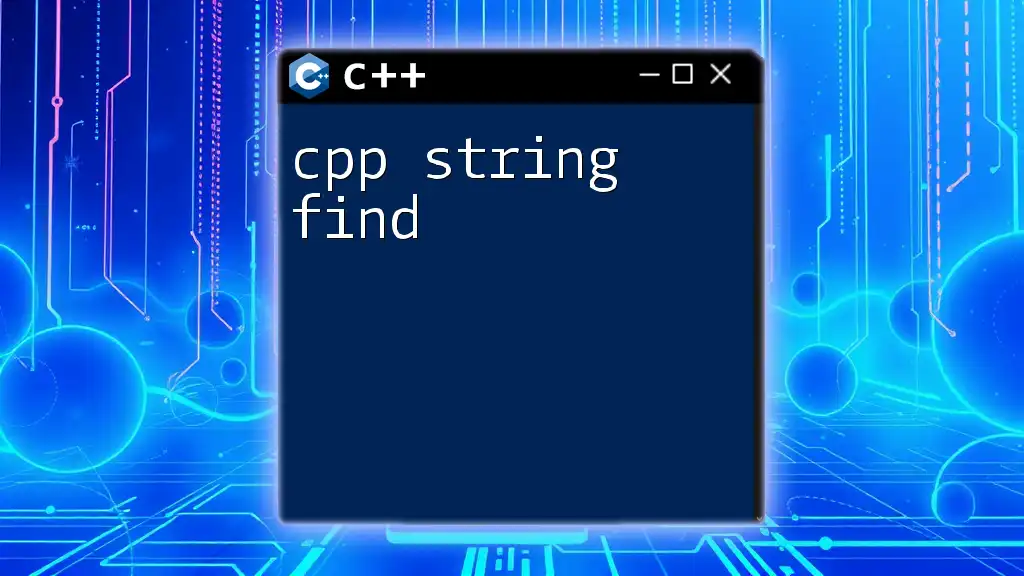
Common Testing Strategies
Unit Testing Best Practices
When writing unit tests, adhere to these principles for better results:
- Write Small, Isolated Tests: Each test should focus on one specific aspect.
- Keep Tests Independent: Ensure tests can run in any order and do not depend on each other’s outcomes.
- Maintain Readable Test Cases: Use descriptive names and comments to make it easier for others to understand the purpose of the tests.
Integration Testing
Integration testing evaluates how well multiple parts of your application work together. You can develop integration tests by:
- Combining modules that interact with each other.
- Checking for the fulfillment of business logic across integrated systems.
Here is an integration test example in pseudo code:
TEST(IntegrationTest, CombinedFunctionality) {
// Arrange
ComponentA a;
ComponentB b;
initializeComponents(a, b);
// Act
auto result = combinedFunction(a, b);
// Assert
EXPECT_TRUE(result.isSuccessful());
}
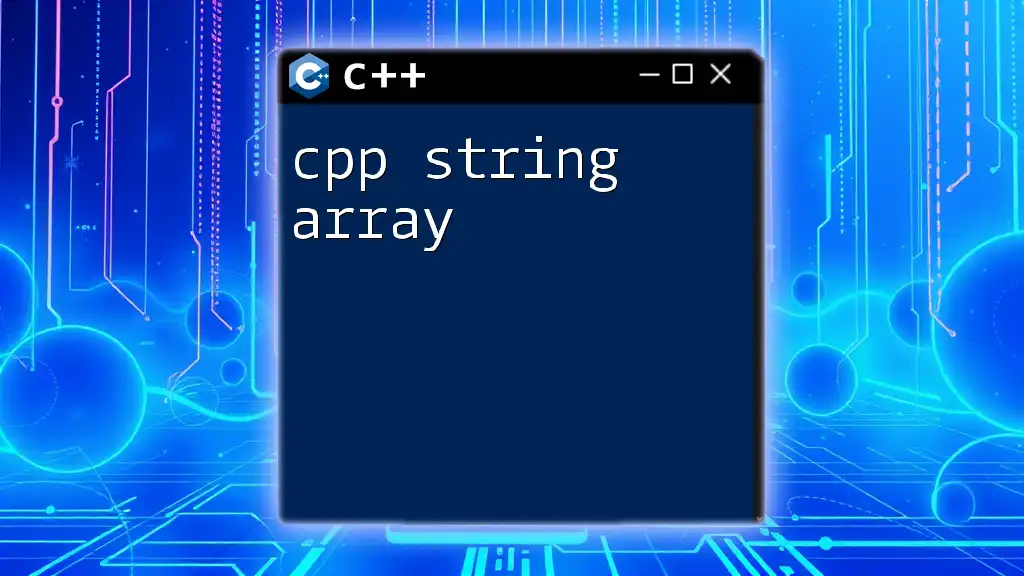
Mocking and Stubbing in C++
Mocking is a technique used during testing to simulate and verify the behavior of complex objects. It helps you isolate specific components or to handle interactions you do not want to perform during testing, such as database calls or network requests.
Using Google Mock with Google Test
Google Mock is an extension of Google Test that facilitates the creation of mock objects. A simple example illustrating its usage is shown below:
#include <gmock/gmock.h>
class MockClass {
public:
MOCK_METHOD(int, doSomething, (int), ());
};
TEST(MockClassTest, CallDoSomething) {
MockClass mock;
EXPECT_CALL(mock, doSomething(5)).WillOnce(Return(10));
ASSERT_EQ(mock.doSomething(5), 10);
}
In this example, `MockClass` simulates functionality, and the test verifies that the `doSomething` method behaves as expected when called with specific input.
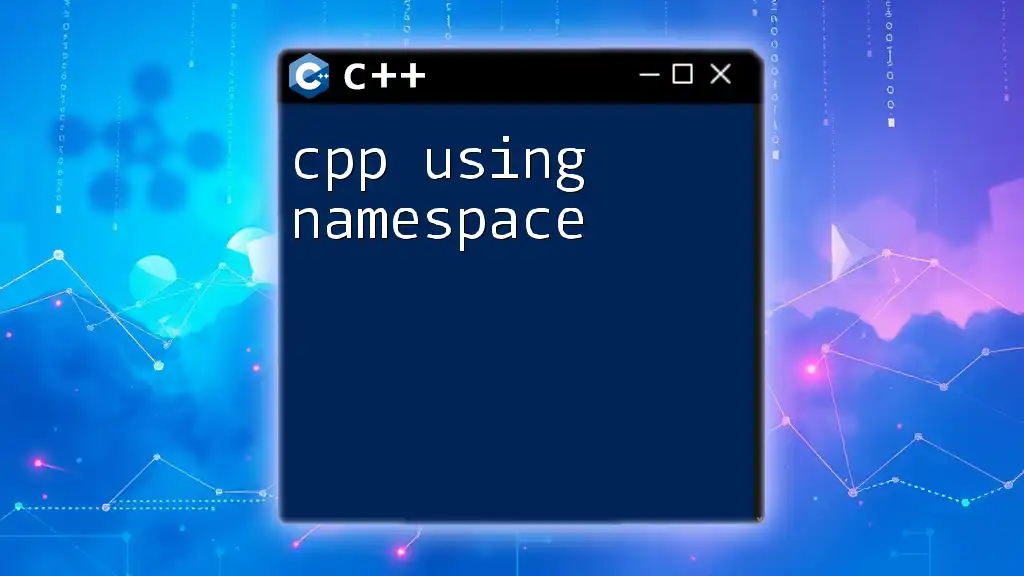
Continuous Integration and Automated Testing
Integrating C++ Tests with CI/CD
Continuous Integration and Continuous Deployment (CI/CD) frameworks allow for automatic testing of your application whenever changes are made. Examples of CI/CD tools suitable for C++ projects include Travis CI, Jenkins, and GitHub Actions.
To set up a CI pipeline, you can create a configuration file (like `.travis.yml` for Travis CI) that describes how to build and test your application automatically.
Setting Up a CI Pipeline for a C++ Project
A simple CI configuration might look like this:
language: cpp
compiler:
- gcc
script:
- mkdir build && cd build
- cmake ..
- make
- ./runTests
This configuration instructs the CI system to build the project and run tests, helping maintain code integrity.
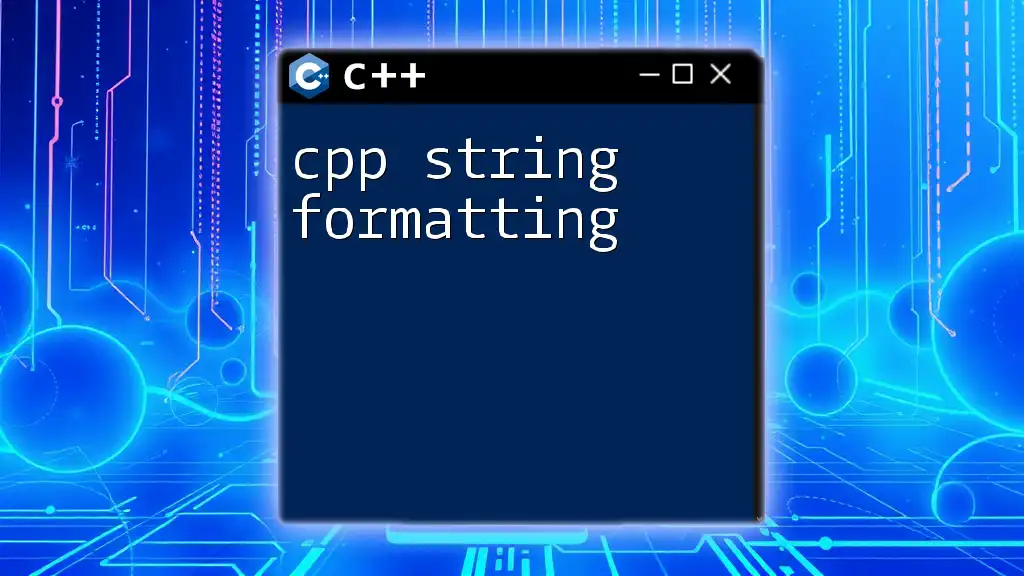
Troubleshooting Common Testing Issues
Test Failures and Debugging Techniques
When tests fail, it’s essential to analyze the output provided. Make sure to read test failure messages carefully, as they often indicate what went wrong. Utilize debugging tools like gdb to step through code and inspect variable states.
Maintaining Quality in Large Projects
In large projects, it's crucial to keep your tests relevant and updated. Strategically revisit and refactor tests when changes occur in your codebase to ensure they remain effective. Implement a policy for regular test reviews to maintain code quality.
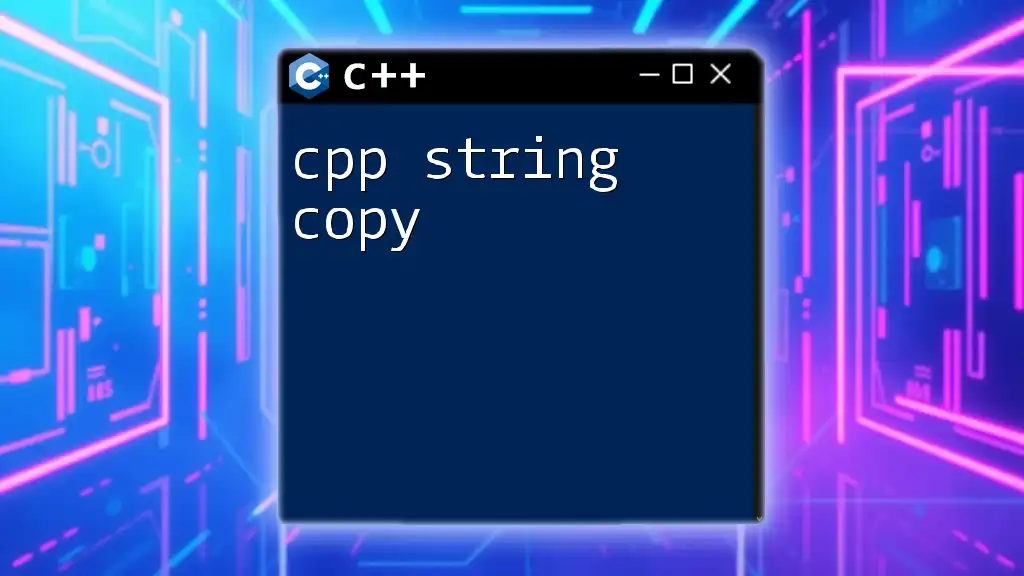
The Importance of Ongoing Testing
C++ testing should not be a one-off task but a continuous activity throughout the development lifecycle. Adopt a testing culture in your team to ensure high-quality software delivery.
Convincing stakeholders of the importance of robust testing processes will result in improved software reliability and client satisfaction.
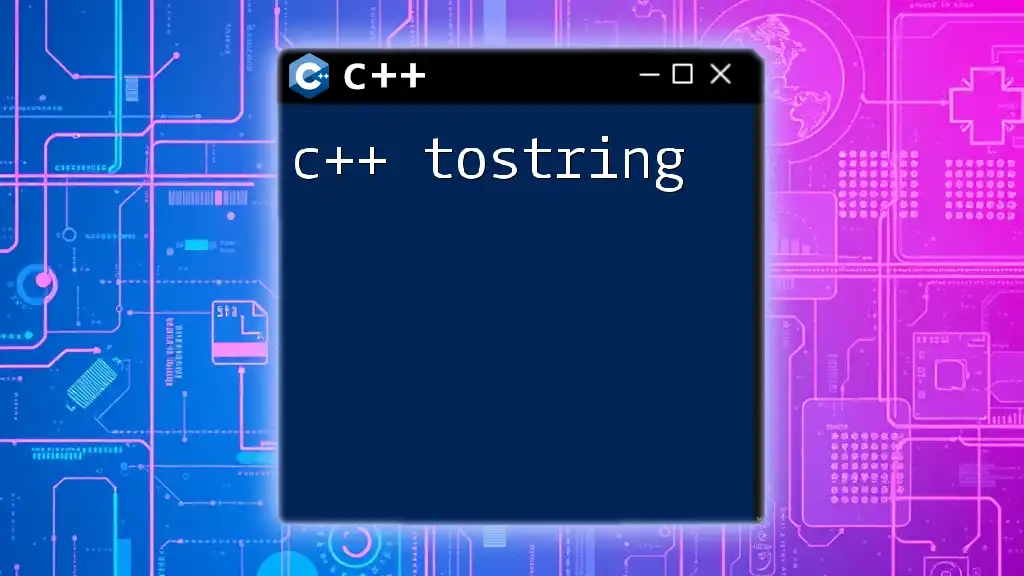
Additional Resources
To further enhance your C++ testing knowledge, consider exploring books and online courses focused on software testing. Join community forums and participate in discussions to stay updated with best practices and emerging trends in C++ testing.
By applying the principles and practices discussed in this guide, you can effectively leverage C++ testing to improve your development process and produce higher-quality applications.