The term "cpp bitmap" refers to a simple way to represent images using a grid of pixels, where each pixel's color is defined in a binary format, and can be manipulated using C++ commands.
Here's a code snippet demonstrating how to create a basic bitmap header in C++:
#include <iostream>
#include <fstream>
struct BitmapFileHeader {
uint16_t fileType = 0x4D42; // BM
uint32_t fileSize;
uint32_t reserved = 0;
uint32_t offsetData;
};
struct BitmapInfoHeader {
uint32_t size = 40; // Header size
int32_t width;
int32_t height;
uint16_t planes = 1;
uint16_t bitCount = 24; // 24-bit bitmap
uint32_t compression = 0;
uint32_t sizeImage;
int32_t xPixelsPerMeter = 0;
int32_t yPixelsPerMeter = 0;
uint32_t colorsUsed = 0;
uint32_t colorsImportant = 0;
};
int main() {
BitmapFileHeader bfh;
BitmapInfoHeader bih;
bih.width = 100; // Set the width of the bitmap
bih.height = 100; // Set the height of the bitmap
bfh.fileSize = sizeof(BitmapFileHeader) + sizeof(BitmapInfoHeader) + bih.width * bih.height * 3; // Calculate file size
bfh.offsetData = sizeof(BitmapFileHeader) + sizeof(BitmapInfoHeader);
std::ofstream file("image.bmp", std::ios::binary);
file.write(reinterpret_cast<char*>(&bfh), sizeof(bfh));
file.write(reinterpret_cast<char*>(&bih), sizeof(bih));
// Fill the image with white color
for (int i = 0; i < bih.width * bih.height; ++i) {
uint8_t color[3] = {255, 255, 255}; // RGB (White)
file.write(reinterpret_cast<char*>(color), sizeof(color));
}
file.close();
return 0;
}
Understanding Bitmaps
What is a Bitmap?
A bitmap, in the context of computer graphics, is a type of image file that represents a grid of pixels. Each pixel's color is defined, which collectively forms the complete image. The resolution of a bitmap is measured in width x height, with color depth indicating the number of bits used to represent each pixel's color.
Bitmaps are fundamentally different from vector graphics, which use geometric shapes to represent images. This distinction is significant, especially in applications where high resolution and detail are required.
Bitmap File Formats
Bitmaps can exist in various formats. The most common bitmap formats include:
- BMP: A raw image format that stores pixel data without compression, leading to larger file sizes but easier accessibility.
- PNG: Supports lossless compression, allowing for smaller file sizes while retaining quality.
- JPEG: Uses lossy compression, which results in smaller files at the cost of some image quality.
Each format has unique characteristics; thus, choosing the right one depends on the application requirements, such as color depth or file size limitations.
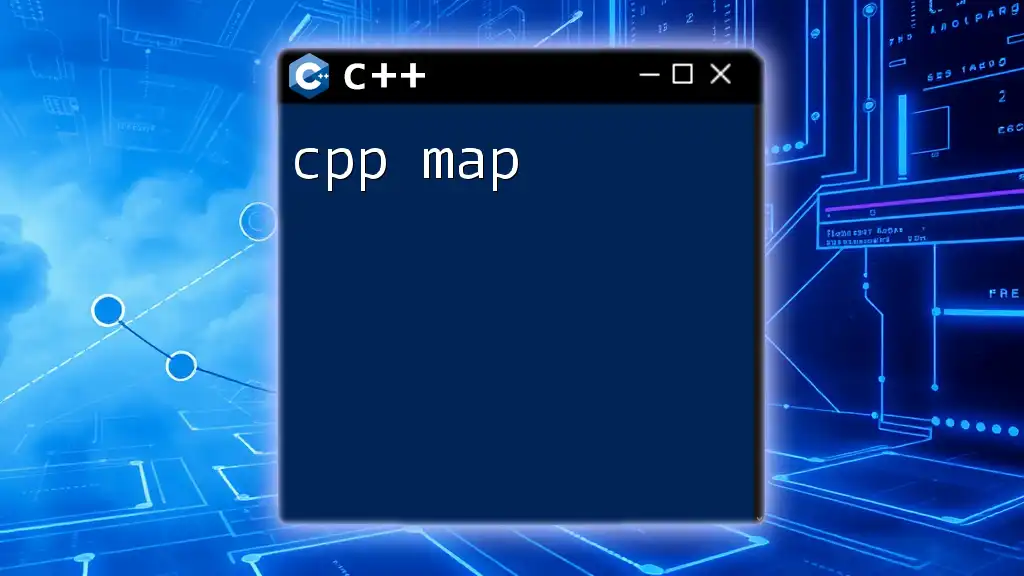
Working with C++ Bitmap Files
Loading and Displaying a Bitmap
When working with C++ bitmaps, libraries such as SDL (Simple DirectMedia Layer) or OpenGL can significantly simplify bitmap operations. Here’s a sample code snippet using SDL to load a bitmap:
#include <SDL.h>
#include <stdio.h>
void LoadBitmap(const char* filePath) {
SDL_Surface* surface = SDL_LoadBMP(filePath);
if (!surface) {
printf("Error loading bitmap: %s\n", SDL_GetError());
return; // Early exit if loading fails
}
// Additional code to display or manipulate the bitmap would go here
SDL_FreeSurface(surface); // Freeing the surface after usage
}
In this example, the `LoadBitmap` function loads a bitmap from a specified file path. If the loading is unsuccessful, an error message is printed.
Creating a Bitmap from Scratch
Creating a bitmap in C++ is straightforward. It involves defining pixel color and dimensions. Below is an example of how to create a simple 100x100 pixel bitmap filled with red:
void CreateBitmap(const char* filePath) {
const int width = 100;
const int height = 100;
SDL_Surface* surface = SDL_CreateRGBSurface(0, width, height, 32, 0, 0, 0, 0);
if (!surface) {
printf("Error creating surface: %s\n", SDL_GetError());
return; // Early exit if surface creation fails
}
// Fill the bitmap with a color
Uint32 color = SDL_MapRGB(surface->format, 255, 0, 0); // Red
SDL_FillRect(surface, NULL, color);
SDL_SaveBMP(surface, filePath); // Save the bitmap to a file
SDL_FreeSurface(surface); // Freeing the surface
}
This `CreateBitmap` function exemplifies creating a blank bitmap, filling it with a solid red color, and saving it to a file.
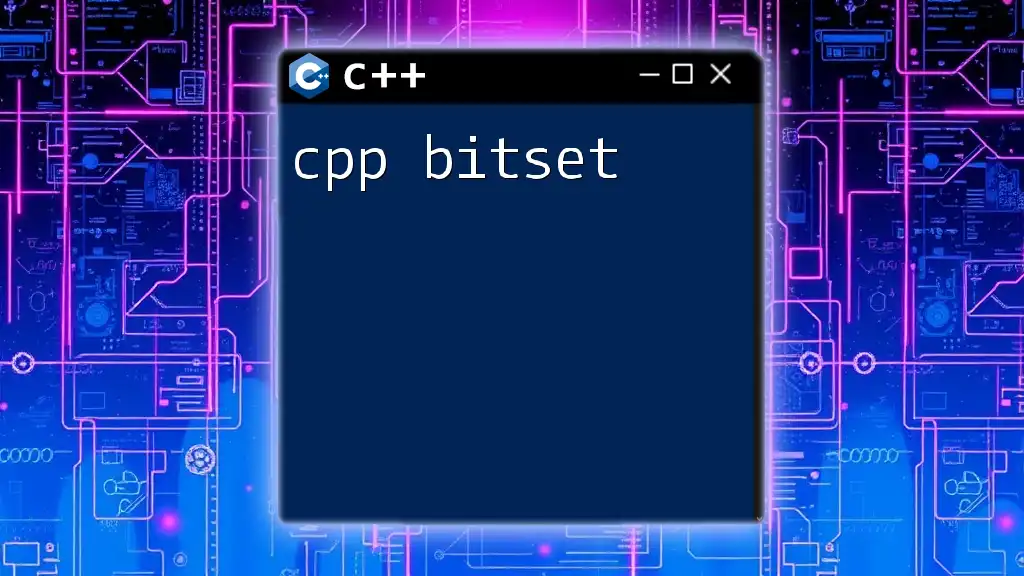
Manipulating Bitmap Data
Reading Bitmap Pixels
Accessing pixel data in C++ is facilitated by casting the bitmap surface to a pixel array. Here’s how you can read pixel values:
Uint32* pixels = (Uint32*)surface->pixels; // Casting surface pixels to a pixel array
Uint32 pixel = pixels[y * surface->w + x]; // Accessing pixel at (x, y)
In the snippet above, you can access any pixel value by calculating its position in the pixel array using its coordinates.
Modifying Bitmap Pixels
You can apply various techniques for pixel manipulation, such as color inversion and grayscale conversion. Here’s an example of converting a bitmap to grayscale:
void ConvertToGrayscale(SDL_Surface* surface) {
Uint32* pixels = (Uint32*)surface->pixels;
for (int y = 0; y < surface->h; ++y) {
for (int x = 0; x < surface->w; ++x) {
Uint32 pixel = pixels[y * surface->w + x];
Uint8 r, g, b;
SDL_GetRGB(pixel, surface->format, &r, &g, &b); // Getting RGB components
Uint8 gray = (r + g + b) / 3; // Calculating the grayscale value
pixels[y * surface->w + x] = SDL_MapRGB(surface->format, gray, gray, gray); // Setting new pixel value
}
}
}
This function iterates through each pixel, calculates its grayscale equivalent, and replaces the original pixel.
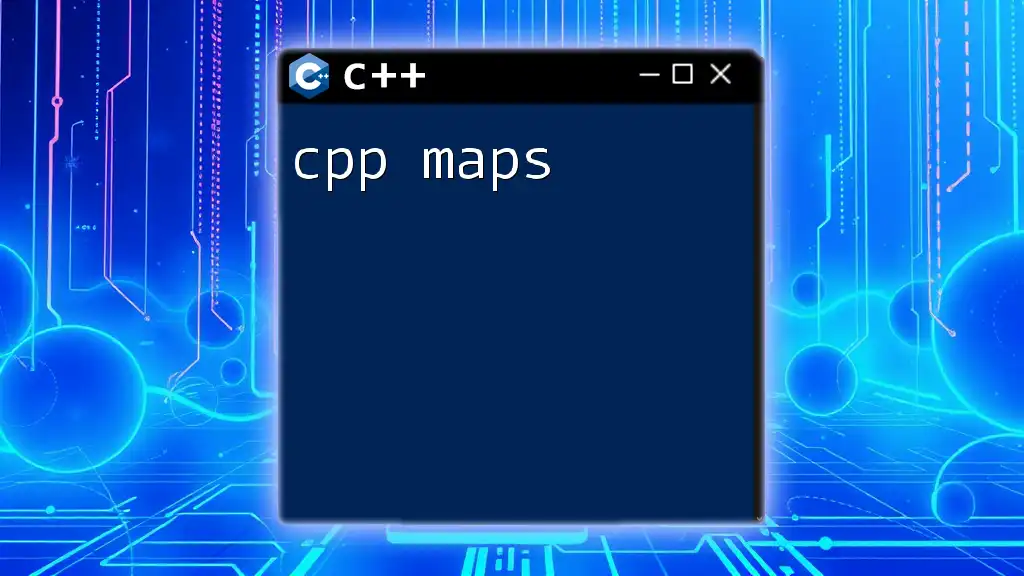
Advanced Bitmap Concepts
Bitmap Compression and Optimization
Managing bitmap size is important, especially for applications requiring efficient resource usage. Techniques such as lossless compression (e.g., PNG) preserve image quality while reducing file size, whereas lossy compression (e.g., JPEG) can result in a considerable reduction in quality.
Using libraries and tools that support these compression types can greatly aid in optimizing bitmap images for web or app usage.
Using Bitmaps in Graphics Applications
Integrating bitmaps into projects such as games involves loading and displaying them effectively. A common workflow might involve loading multiple bitmap images and rendering them during the game loop, allowing for dynamic interaction and visual updates.
For instance, if you wanted to display a loaded bitmap continuously, it might look something like this in SDL:
void GameLoop(SDL_Surface* screen) {
SDL_Surface* myBitmap = SDL_LoadBMP("myImage.bmp");
if (!myBitmap) {
printf("Error loading bitmap: %s\n", SDL_GetError());
return;
}
// Main game loop
while (true) {
// Handle events, update game state...
SDL_BlitSurface(myBitmap, NULL, screen, NULL);
SDL_Flip(screen); // Updating the display
}
SDL_FreeSurface(myBitmap); // Clean up after exit
}
In the `GameLoop`, the bitmap is continuously drawn to the screen, creating the illusion of motion or interactivity.
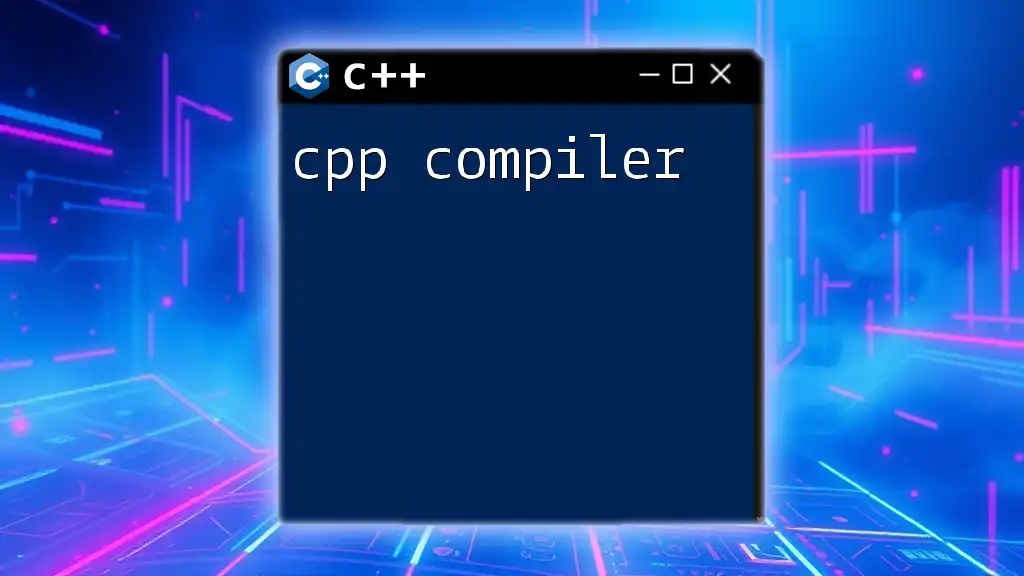
Conclusion
In this guide, we explored the fundamentals of C++ bitmaps, from basic definitions to practical applications in graphics programming. Understanding how to load, create, read, and manipulate bitmap files equips you with essential skills for any graphical application. With these skills, you can create graphics, games, and any visual elements that require precise pixel control.
By continuing to practice and experiment with these concepts, you'll unlock new creative possibilities in your C++ programming journey.
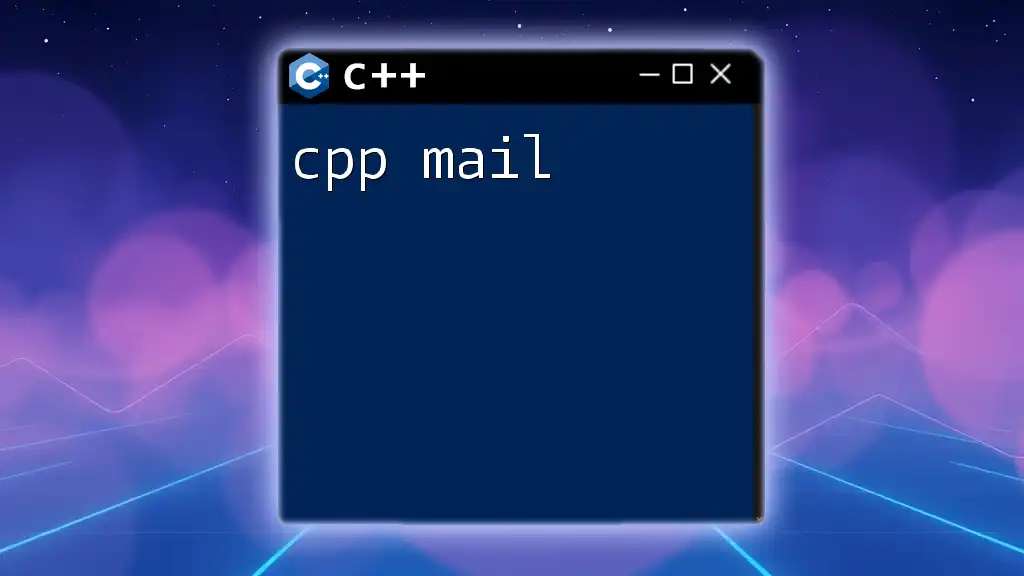
Further Reading
If you're eager to continue learning, consider diving deeper into the following resources:
- SDL Documentation for more detailed explanations on bitmap handling.
- Tutorials on OpenGL for more advanced graphics programming.
- Online communities and forums for support and shared knowledge on bitmap processing and graphics applications.