The C++ console is a command-line interface that allows users to interact with and execute C++ programs, typically for input and output operations.
Here's a simple code snippet demonstrating how to output "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Setting Up Your C++ Development Environment
Choosing the Right IDE
Selecting the right Integrated Development Environment (IDE) can significantly enhance your development experience with C++ console applications. Some popular options include:
- Visual Studio: Ideal for Windows users, it has powerful debugging tools and an intuitive interface.
- Code::Blocks: A free, open-source option that’s lightweight and versatile, suitable for beginners.
- Dev-C++: An older IDE but still beloved for its simplicity and ease of use.
To get started, simply download your chosen IDE from its official site and follow the installation instructions. Most IDEs come with built-in templates that make initializing a new C++ console project straightforward.
Setting Up Command Line Tools
For those keen on using the command line, it's crucial to install essential tools like GCC (GNU Compiler Collection) or Clang, which enable you to compile and run your C++ console applications directly from the terminal. Configuration involves setting environment variables to include your compiler in the system path, which allows executing commands from any directory in your command prompt.
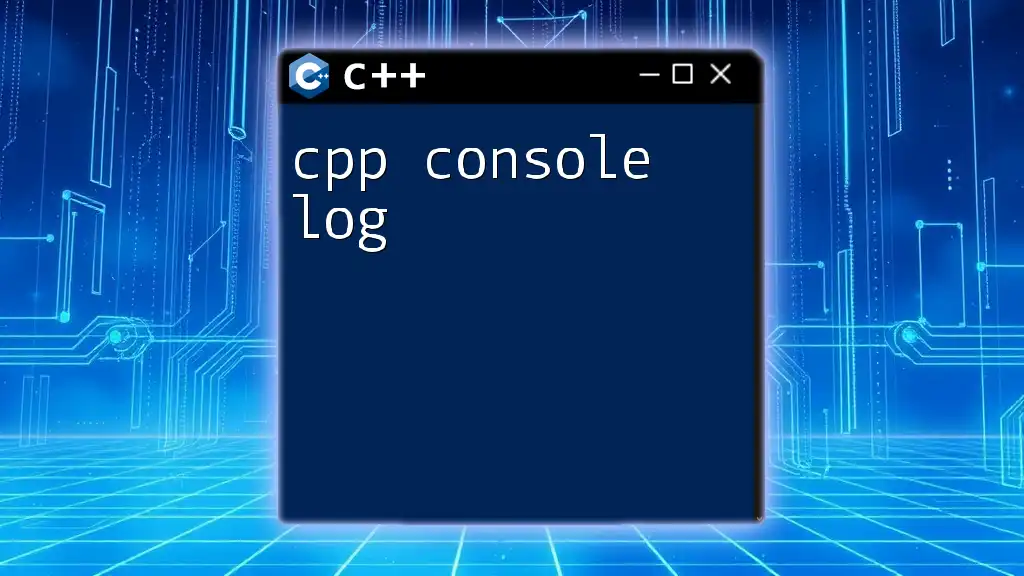
Understanding the Basics of C++ Console
What is a C++ Console Application?
A C++ console application is a text-based program that runs in a console or terminal window. Unlike graphical user interface (GUI) applications, C++ console applications interact with users through input and output text. This type of application is essential for learning the fundamentals of programming, as it emphasizes logical thinking and problem-solving without the complexities of graphical interfaces.
Essential C++ Libraries
C++ provides several libraries vital for building console applications. One of the most common is the iostream library, which allows for standard input and output. Using the #include directive, you can easily import this library at the beginning of your program:
#include <iostream>
Understanding how to utilize these libraries will lay a strong foundation for your programming skills.
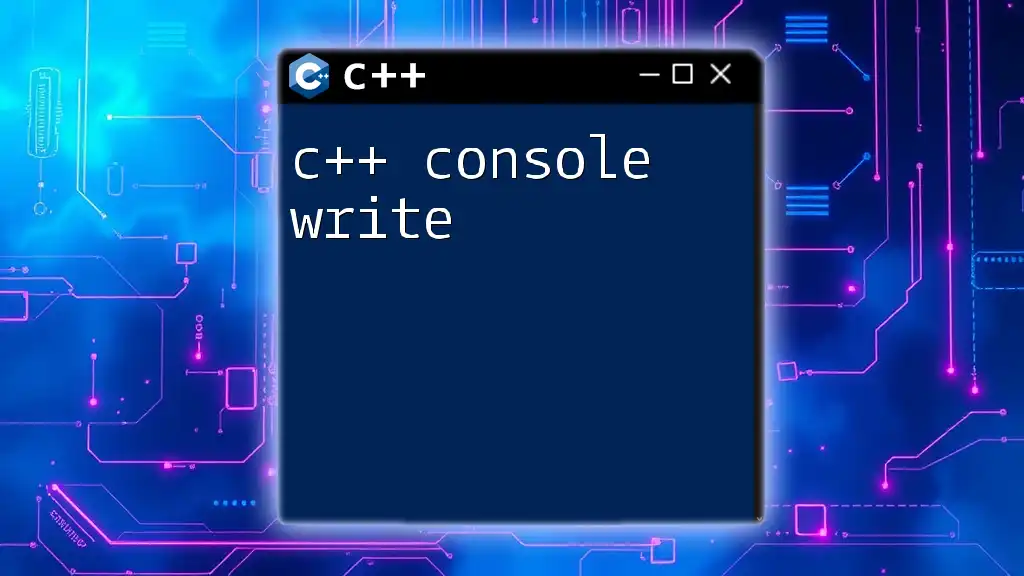
Writing Your First C++ Console Application
Basic Structure of a C++ Program
The backbone of any C++ console application is the main() function. This function is the starting point for program execution. Here’s a simple template:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this example, std::cout is used to send output to the console, and std::endl is employed to create a new line.
Compiling and Running a C++ Console Application
Compiling your program is a crucial step before execution. If you're using an IDE, you can often simply press a compile button. If you're working from the command line, navigate to your project directory and use a command such as:
g++ -o my_program my_program.cpp
This command compiles `my_program.cpp` into an executable called `my_program`. To run your application, use:
./my_program
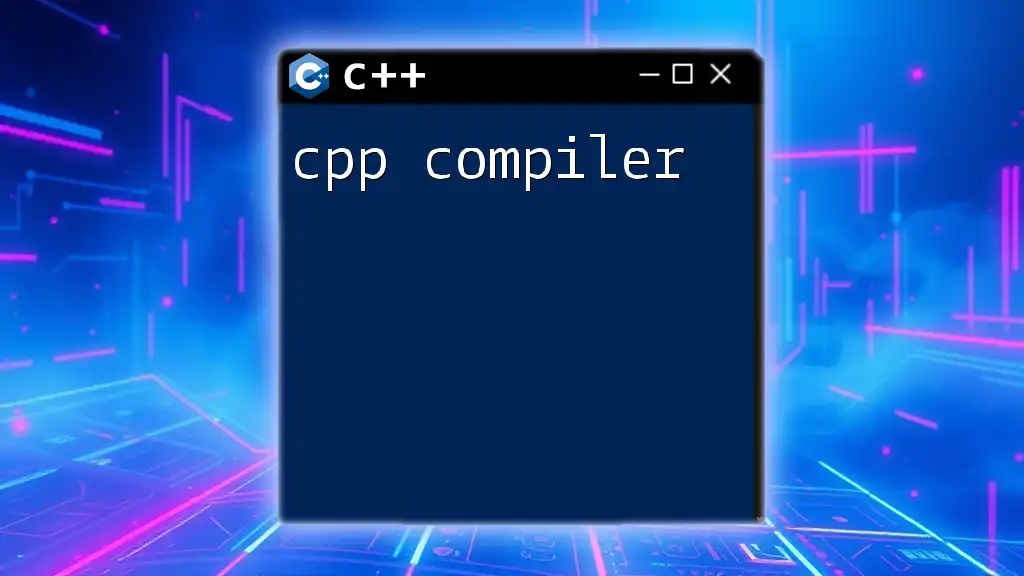
Input and Output in C++ Console
Using std::cout for Output
The std::cout statement is a fundamental way to output data to the console in C++. You can format your output using various operators. For example:
std::cout << "The answer is: " << 42 << std::endl;
This line outputs a string followed by an integer. Understanding these output streams will enable you to build more interactive applications.
Taking User Input with std::cin
Similarly, std::cin is used to obtain input from users. Here’s how you can read an integer:
int number;
std::cout << "Enter a number: ";
std::cin >> number;
This snippet prompts the user to enter a number and stores it in the variable number. Handling std::cin correctly helps you create interactive C++ console applications.
Validating User Input
Input validation ensures that the data received is appropriate for processing. To implement basic validation, you can add checks after user input. For instance, if the user inputs a string where a number is expected, use error handling techniques to repeat the prompt until valid input is received.
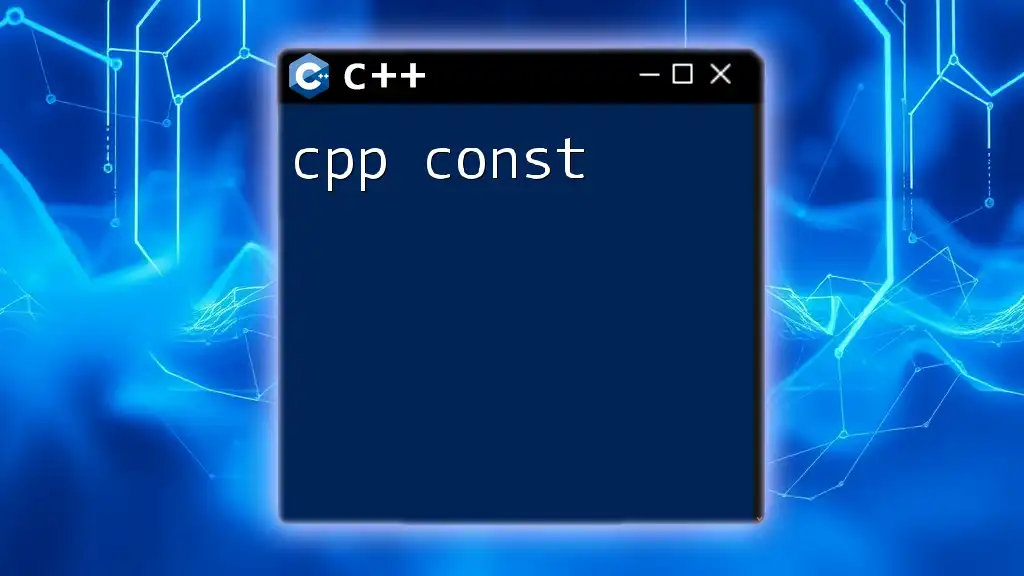
Advanced C++ Console Features
Using Command-Line Arguments
Command-line arguments allow your C++ console application to accept inputs when executed from the terminal. The main() function can be modified to accept arguments:
int main(int argc, char* argv[]) {
for(int i = 0; i < argc; i++) {
std::cout << "Argument " << i << ": " << argv[i] << std::endl;
}
return 0;
}
In this program, argc holds the count of arguments passed, while argv is an array of argument strings. This feature is particularly useful for building programs that require file names or flags during execution.
Error Handling in C++ Console Applications
Robust error handling is crucial for any application. C++ provides exceptions to catch runtime errors. Example:
try {
int num = std::stoi("abc");
} catch (const std::invalid_argument& e) {
std::cout << "Error: Invalid input!" << std::endl;
}
In this example, if std::stoi faces an invalid conversion, it throws an exception, which is caught and handled gracefully.
Creating Menus and Navigation in Console Applications
To enhance user experience, consider implementing menus in your applications. This promotes easy navigation and interaction. Here’s an example of a simple text-based menu:
void displayMenu() {
std::cout << "1. Option 1\n";
std::cout << "2. Option 2\n";
std::cout << "3. Exit\n";
}
This menu can be presented to the user, enabling them to choose actions based on their input, such as executing different functions or displaying information.
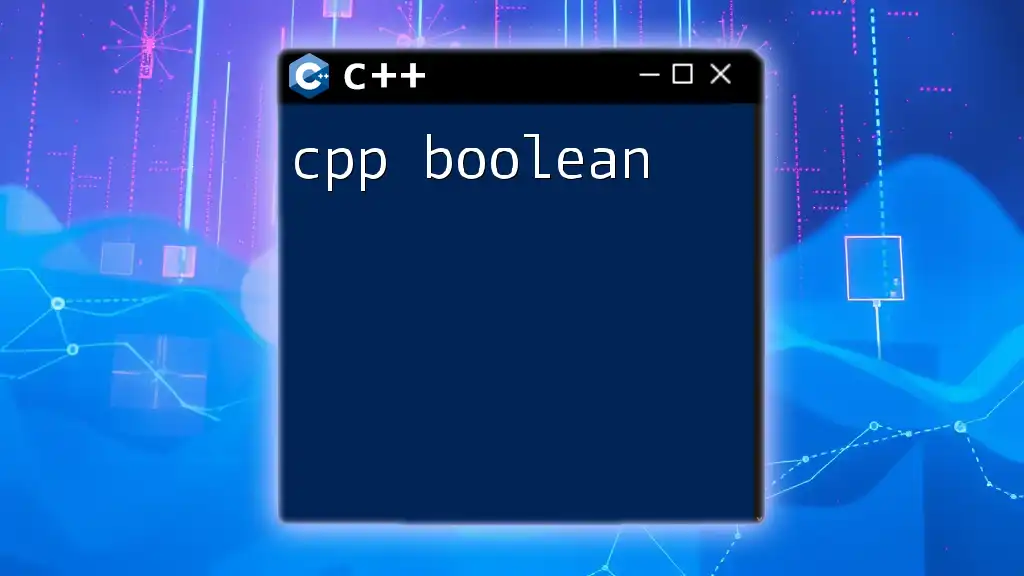
Best Practices for C++ Console Programming
Code Readability and Maintenance
Whether you're a beginner or an experienced developer, code readability is paramount. Use meaningful variable names, maintain consistent indentation, and comment your code where necessary. By following these practices, you enhance the maintainability of your C++ console applications, making them easier to understand and modify in the future.
Performance Optimization
Performance matters, especially in larger applications. To optimize your console programs, analyze algorithms for efficiency and be wary of using excessive loops or unnecessary computations. Profiling tools can help identify bottlenecks, allowing you to streamline your code for better performance.
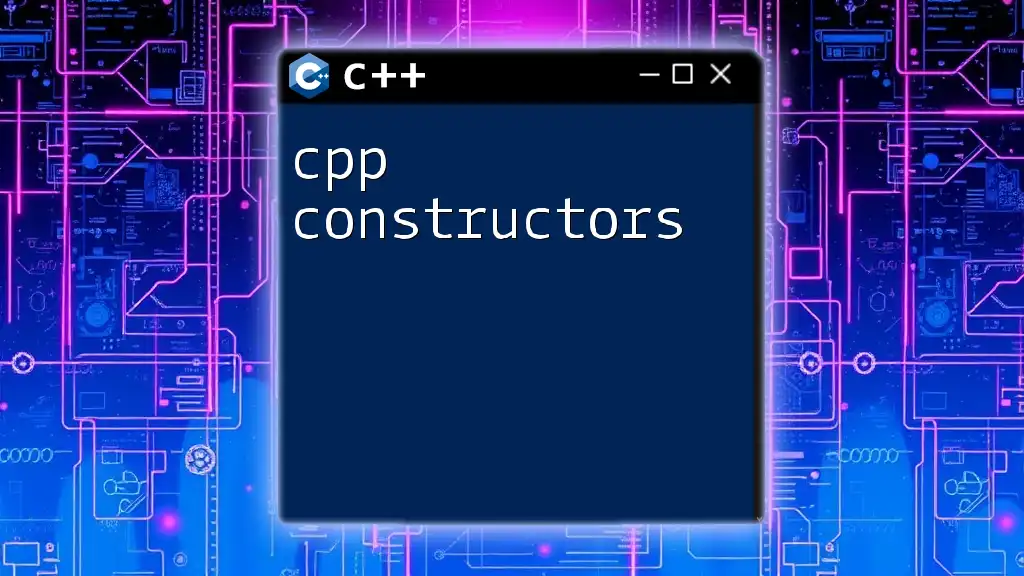
Conclusion
As explored, C++ console applications are fundamental to understanding programming concepts. From setting up your environment to writing complex applications, the knowledge gained equips you for further exploration of more advanced programming topics. Embrace the journey, experiment with different commands, and dive deeper into C++ as you grow your skills.
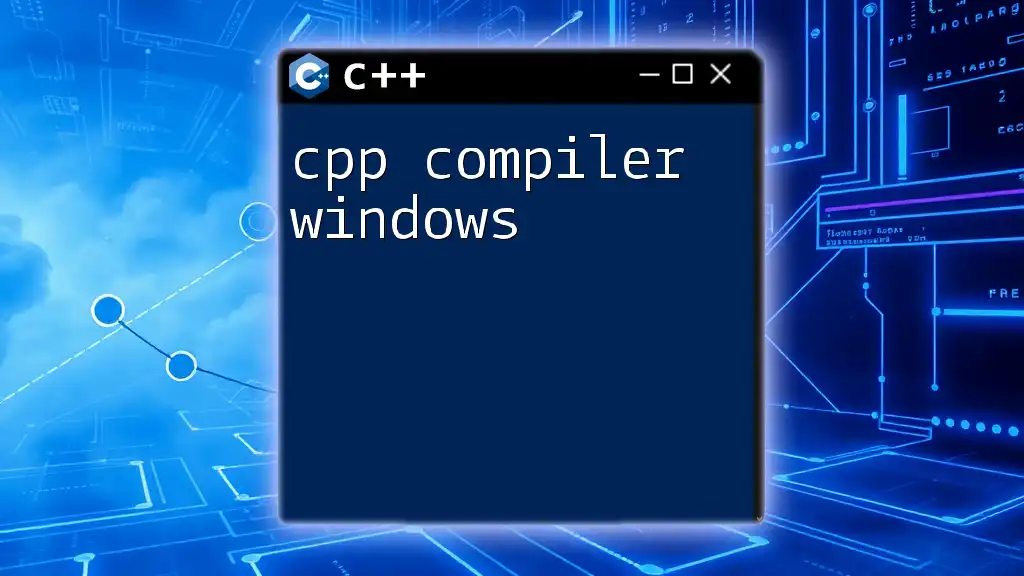
Additional Resources
For continued learning, consider exploring various resources, including books like "The C++ Programming Language" by Bjarne Stroustrup, online courses, and active communities. Engage in project-based learning through sample applications to solidify your understanding and practical expertise in C++ console programming. Happy coding!