CPP notes are concise, easy-to-follow reminders of essential C++ commands and syntax for quick reference.
Here's an example of a basic C++ program that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ Syntax
Basic Syntax Elements
C++ is a statically typed language, which means you must declare the type of every variable before using it. This helps in preventing type errors at compile time.
Data Types: Familiarize yourself with the common data types in C++. Here are the primary types you'll encounter:
- `int`: Represents integer values.
- `float`: Represents floating-point numbers, which allow for decimal values.
- `char`: Represents a single character.
Here’s how you can declare these data types:
int number = 10; // Integer
float pi = 3.14; // Float
char letter = 'A'; // Character
Variables and Constants: In C++, you can create variables to store data that can change, and constants for values that should remain fixed throughout the program.
To declare a constant, use the `const` keyword:
const int MAX_VALUE = 100; // Constant
int x = 5; // Variable
Control Structures
Control structures allow you to dictate the flow of your program. Understanding them is crucial for developing effective algorithms.
Conditional Statements: Use conditional statements to execute different sections of code based on certain conditions. The basic structure includes `if`, `else if`, and `else`.
For example:
if (x > 0) {
// Code to execute if x is positive
cout << "x is positive." << endl;
} else {
// Code to execute if x is not positive
cout << "x is not positive." << endl;
}
Loops: Loops are used to execute a block of code repeatedly. C++ provides several types of loops, including the `for` loop, `while` loop, and `do-while` loop.
Here's an example of a `for` loop:
for (int i = 0; i < 5; i++) {
cout << i << endl; // Print numbers from 0 to 4
}
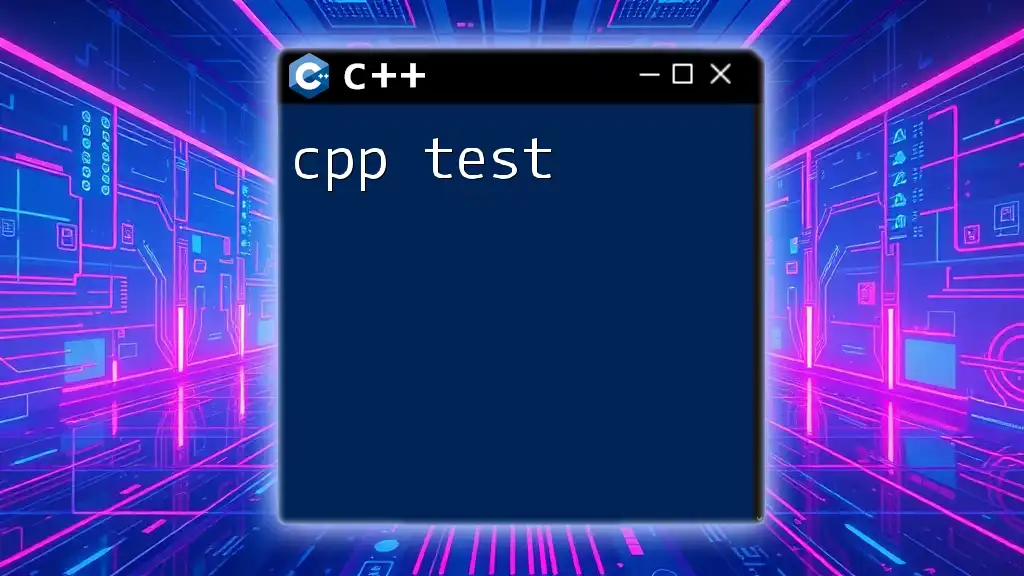
C++ Functions
Functions are fundamental building blocks in any C++ program. They allow you to encapsulate code for reuse and organization.
Function Definition and Declaration
You can define functions in C++ to perform specific tasks. A function can take parameters, perform operations, and return a result.
For example, here's a simple function to add two integers:
int add(int a, int b) {
return a + b; // Function that returns the sum of two integers
}
Function Overloading
C++ allows you to create multiple functions with the same name but different parameter lists. This is known as function overloading, and it enables you to use the same function name for different types of operations.
Here's an example with an overloaded function:
float add(float a, float b) {
return a + b; // This version works for floating-point numbers
}
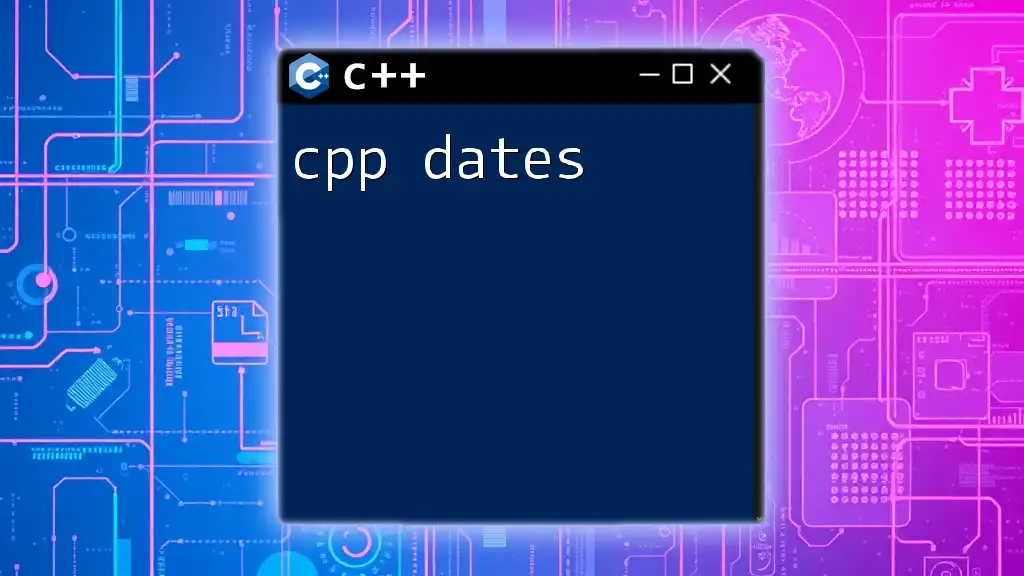
Object-Oriented Programming in C++
C++ is designed with a strong focus on Object-Oriented Programming (OOP), allowing you to create flexible and scalable software architectures.
Classes and Objects
A class is a blueprint for creating objects. It defines properties (attributes) and methods (functions) that the objects of that class can have.
Here’s a simple class definition:
class Car {
public:
string brand; // Attribute
void honk() { // Method
cout << "Honk!" << endl;
}
};
Inheritance and Polymorphism
Inheritance allows one class to inherit the properties and methods of another. This promotes code reusability and can simplify operations.
For instance:
class ElectricCar : public Car {
public:
void charge() {
cout << "Charging..." << endl;
}
};
Understanding Polymorphism: Polymorphism enables you to call derived class methods through base class pointers or references, allowing for dynamic method resolution.
Example of polymorphism through method overriding:
class Animal {
public:
virtual void sound() { // Virtual function
cout << "Animal sound" << endl;
}
};
class Dog : public Animal {
public:
void sound() override { // Overriding the base class method
cout << "Bark!" << endl;
}
};
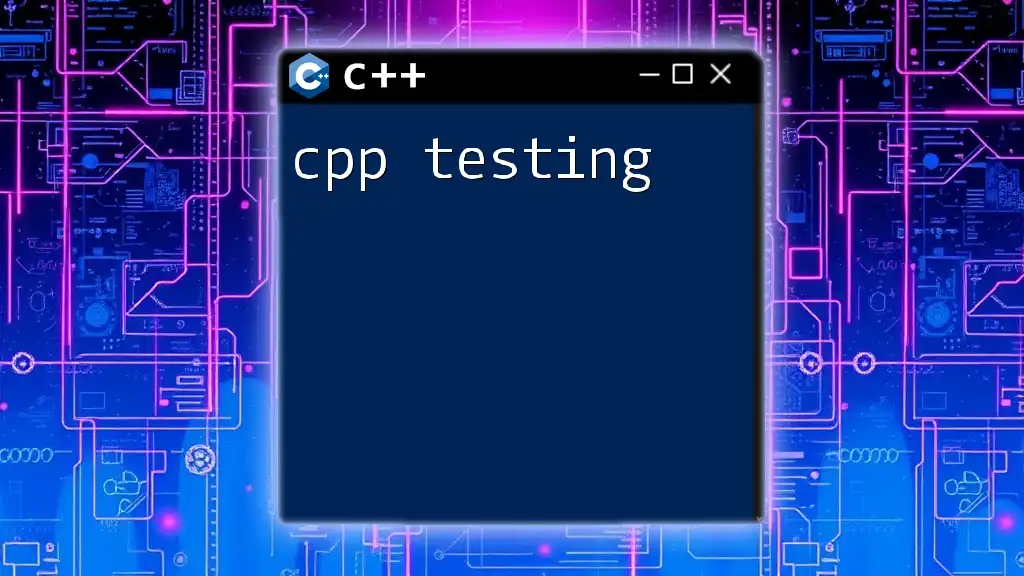
Standard Template Library (STL) Basics
The Standard Template Library (STL) is a powerful set of C++ template classes to manage collections of data, algorithms, and iterators.
Introduction to STL
The STL provides generic classes and functions, which means you can write code that works with any data type. This eliminates redundancy and improves code maintainability.
Common Containers
STL includes several container classes for storing collections of data. Understanding these will enhance your programming efficiency.
Vectors: Vectors are dynamic arrays that can resize themselves automatically when new items are added.
Example of using a vector:
#include <vector>
using namespace std;
vector<int> numbers = {1, 2, 3, 4, 5}; // Creating a vector of integers
Maps and Sets: Maps store key-value pairs, while sets store unique elements. Both provide efficient access and manipulation of data.
Here's an example of using a map:
#include <map>
using namespace std;
map<string, int> ages;
ages["Alice"] = 30; // Assigning age to the name 'Alice'
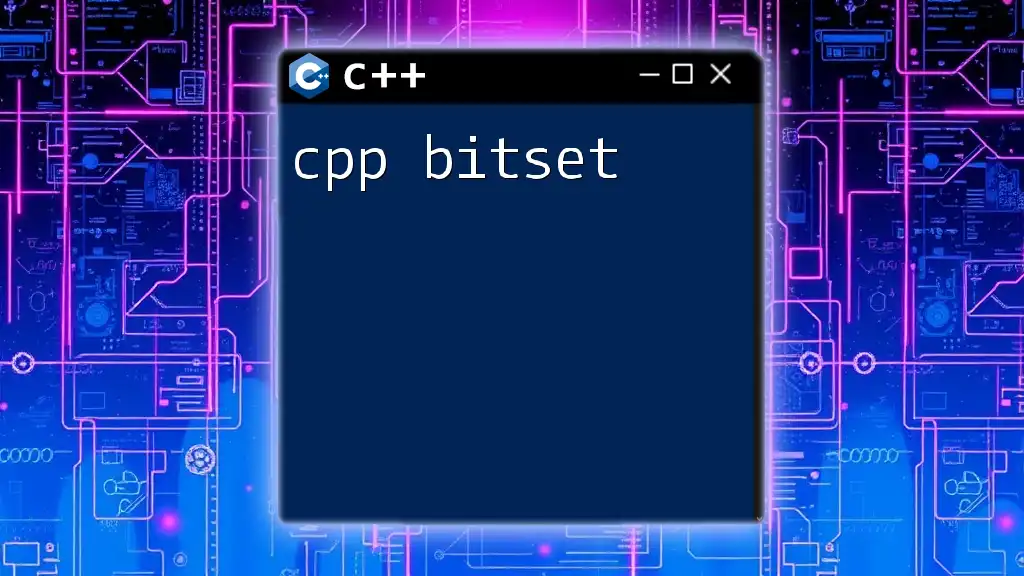
C++ Best Practices
Adhering to best practices is crucial in C++ programming. It ensures your code is clean, maintainable, and efficient.
Writing Readable Code
Readable code is essential for collaboration and long-term maintenance. Some tips include:
- Naming Conventions: Use descriptive names for variables and functions, adhering to standard naming conventions.
- Comments: Make effective use of comments to explain complex logic but avoid over-commenting obvious code.
- Indentation: Maintain consistent indentation to improve readability.
Debugging Techniques
Debugging is an integral part of the development process. Familiarize yourself with debugging tools and techniques to identify and fix issues efficiently.
Here’s a simple debugging message example:
#include <iostream>
using namespace std;
int main() {
int value = -1;
if (value < 0) {
cout << "Value is negative!" << endl; // Helpful debug message
}
return 0;
}
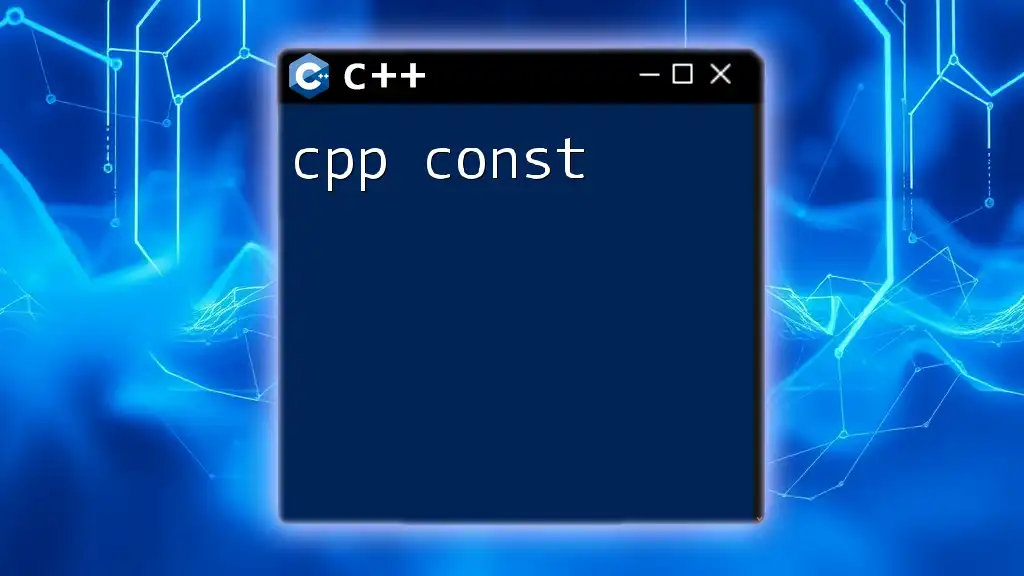
Conclusion
C++ notes are invaluable for organizing your coding knowledge efficiently. By mastering the syntax, functions, object-oriented features, and best practices, you’ll be well on your way to becoming proficient in C++. Emphasize practical application through projects and coding exercises to reinforce your learning.
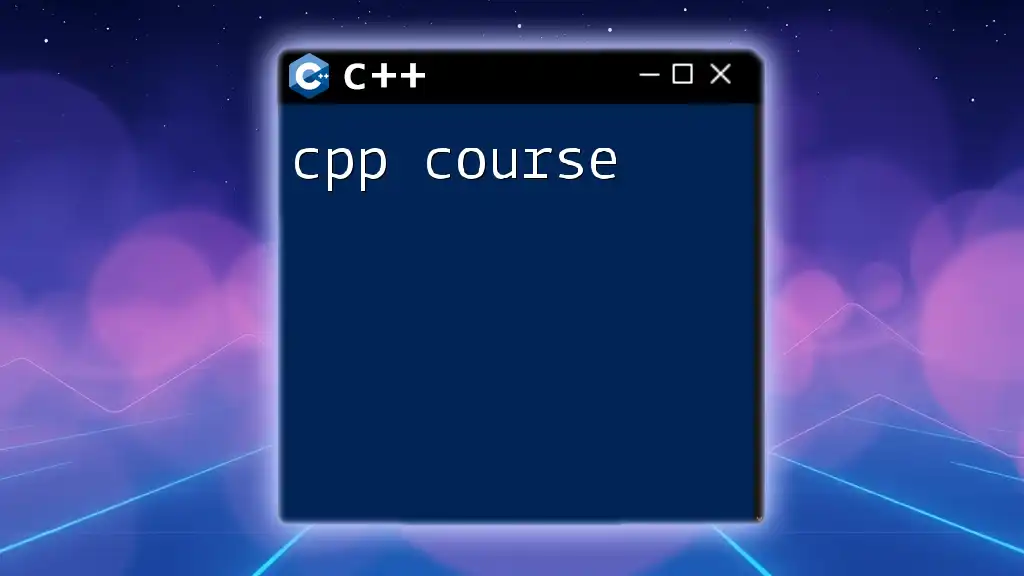
Additional Resources
For further learning, consider exploring recommended books and online courses that delve deeper into C++. Also, engage with communities and forums dedicated to C++ programming to expand your knowledge and seek assistance when needed.