The `strstr` function in C++ is used to locate the first occurrence of a substring within a string, returning a pointer to the beginning of the found substring or `nullptr` if it is not found.
Here’s a code snippet demonstrating its usage:
#include <iostream>
#include <cstring>
int main() {
const char* str = "Hello, world!";
const char* subStr = "world";
char* result = strstr(str, subStr);
if (result) {
std::cout << "Substring found at position: " << (result - str) << std::endl;
} else {
std::cout << "Substring not found." << std::endl;
}
return 0;
}
What is `strstr`?
The `strstr` function in C++ is a powerful tool for locating a substring within a larger string. It is defined in the C standard library, which means it is also available in C++. The primary purpose of `strstr` is to find the first occurrence of a specified substring, often referred to as the "needle," within another string, referred to as the "haystack."
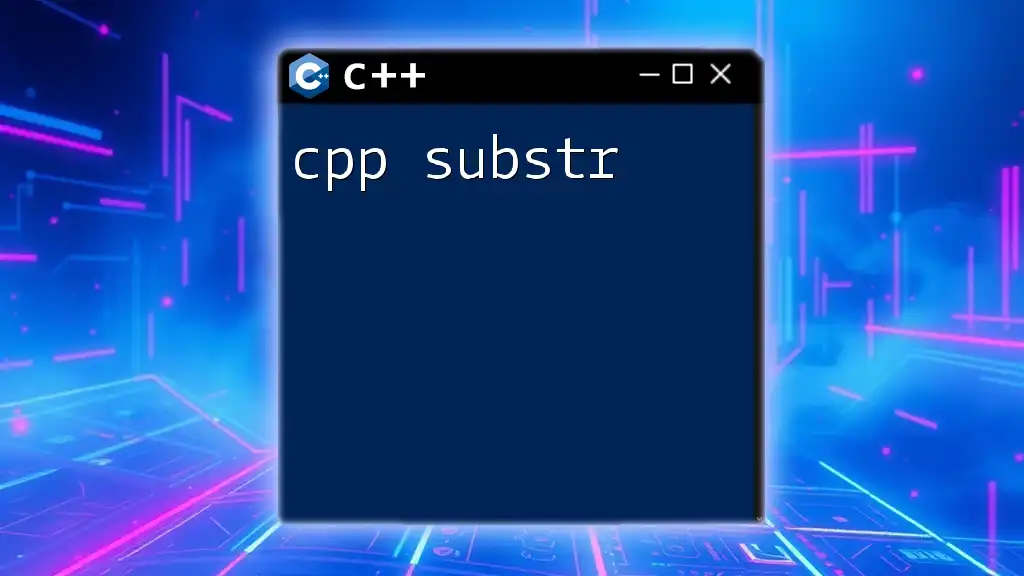
Why Use `strstr`?
Utilizing `strstr` can simplify many string manipulation tasks. Here are a few reasons to consider using it:
- Data Processing: In scenarios such as parsing text files or processing user input, being able to quickly find substrings is crucial.
- Performance: Depending on the implementation, `strstr` can provide efficient searching from within the C++ standard library.
- Convenience: It abstracts away the complexity of searching for substrings, allowing developers to focus on higher-order logic in their applications.
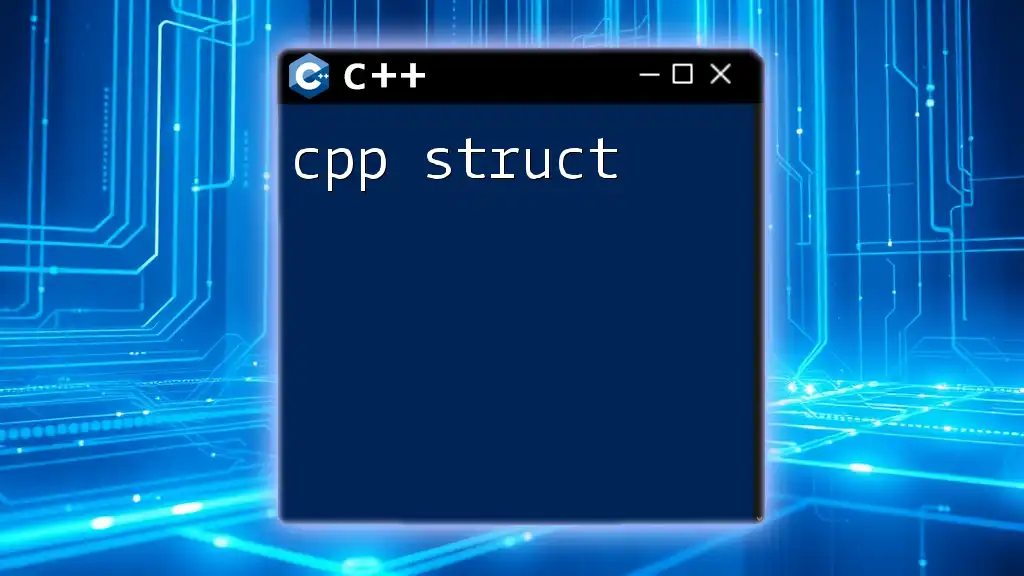
Understanding the Syntax of `strstr`
Basic Syntax of `strstr`
The function signature of `strstr` is as follows:
char* strstr(const char* haystack, const char* needle);
- Parameters:
- `haystack`: This is the string in which you want to search.
- `needle`: This is the substring you want to find within the haystack.
- Return Type: `strstr` returns a pointer to the first occurrence of the substring `needle` in `haystack`. If `needle` is not found, it returns a null pointer (`nullptr`).
Function Prototype
To use `strstr`, you should include the header file `<cstring>` or `<string.h>` at the beginning of your code. This ensures that the compiler recognizes the function.
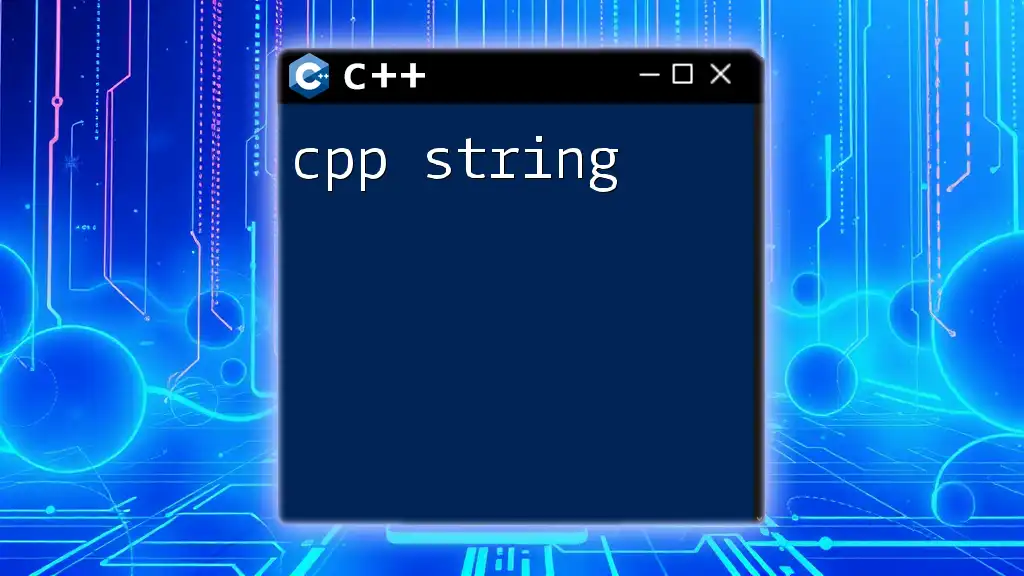
How `strstr` Works
Mechanism of String Searching
Internally, `strstr` uses algorithms to traverse the `haystack` and check for occurrences of the `needle`. It examines segments of the `haystack`, comparing them with the `needle`, until it either finds a match or reaches the end of the string.
Case Sensitivity in `strstr`
It's important to note that `strstr` is case-sensitive. For example, searching for `"hello"` will not match `"Hello"`. If case insensitivity is required, alternative methods or functions need to be implemented.
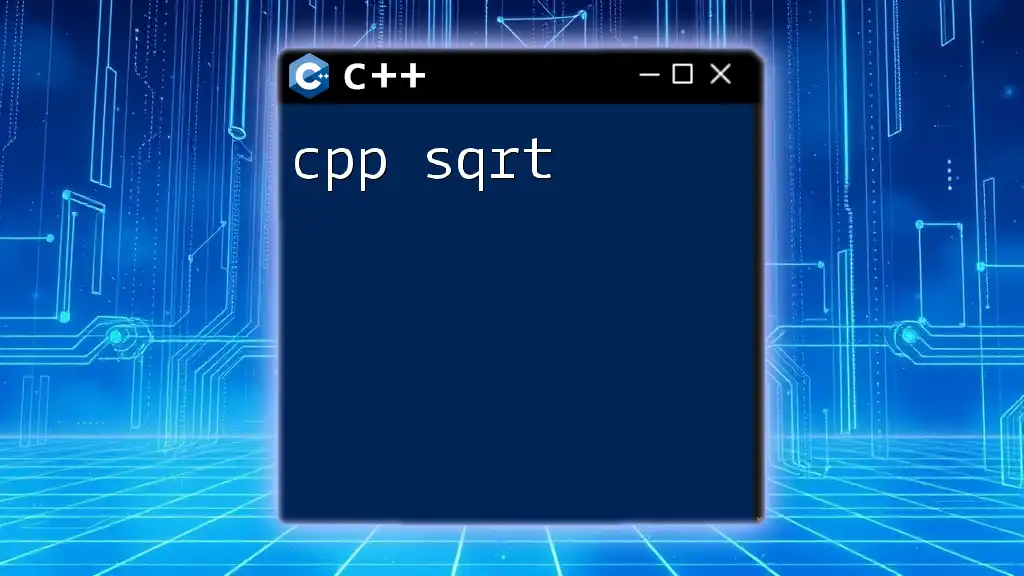
Using `strstr` with Examples
Example 1: Basic Usage
Here is a simple demonstration of using `strstr`:
#include <iostream>
#include <cstring>
int main() {
const char* str = "Hello World";
const char* substr = "World";
char* result = strstr(str, substr);
if (result) {
std::cout << "Substring found at position: " << (result - str) << std::endl;
} else {
std::cout << "Substring not found." << std::endl;
}
return 0;
}
In this example, we first declare a `haystack` and a `needle`. The `strstr` function attempts to locate `needle` within `haystack`. If found, it calculates and displays the position of the substring; otherwise, a message indicating that the substring was not found is displayed.
Example 2: When Substring is Not Present
Here's an example that illustrates what happens when the substring does not exist:
#include <iostream>
#include <cstring>
int main() {
const char* str = "Hello World";
const char* substr = "C++";
char* result = strstr(str, substr);
if (result) {
std::cout << "Substring found." << std::endl;
} else {
std::cout << "Substring not found." << std::endl;
}
return 0;
}
This example highlights that since `"C++"` is not part of `"Hello World"`, the output will indicate that the substring is not found.
Example 3: Using `strstr` in a Loop
We can also use `strstr` in a loop to find multiple occurrences of a substring:
#include <iostream>
#include <cstring>
int main() {
const char* str = "C++ is great. I love C++. C++ is fun.";
const char* substr = "C++";
char* result = (char*)str;
while ((result = strstr(result, substr)) != nullptr) {
std::cout << "Found C++ at position: " << (result - str) << std::endl;
result++; // Move to the next character for further searches
}
return 0;
}
In this code, we continue searching for `"C++"` in the original string and print the position of each occurrence. The use of `result++` ensures that we search for subsequent instances.
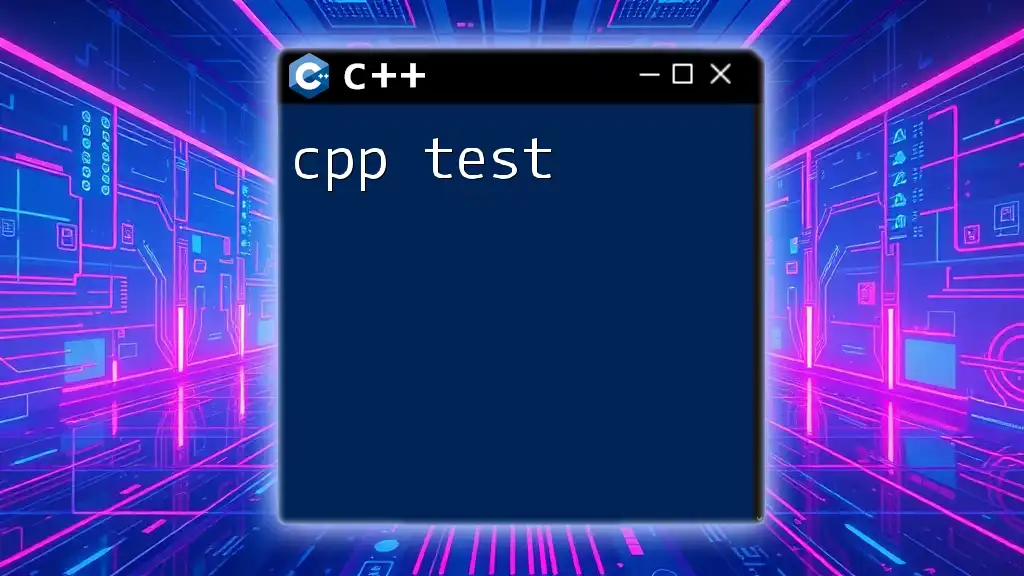
Common Pitfalls with `strstr`
Handling Null Pointers
When using the `strstr` function, it's essential to handle potential null pointers. If either `haystack` or `needle` is provided as a null pointer, the behavior of `strstr` is undefined, which may lead to crashes or unexpected behavior. Always validate input before calling `strstr`.
Performance Considerations
While `strstr` is generally efficient, performance can degrade with very large strings or when searching for complex patterns. For specific scenarios involving heavy string searching, consider alternate algorithms such as Boyer-Moore or Knuth-Morris-Pratt, especially when working with extensive datasets.
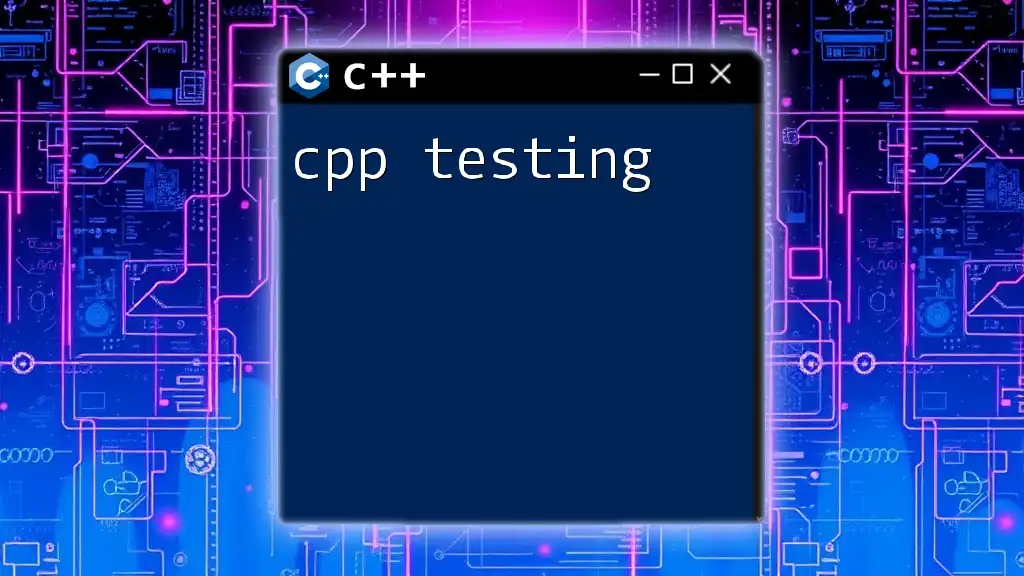
Advanced Topics in `strstr`
C++11 and Beyond Enhancements
With the advent of C++11 and later versions, you can leverage features like smart pointers and STL string classes. Instead of working with C-style strings, consider using `std::string` for safer memory management and enhanced functionality. You might use the `find` method of `std::string` as follows:
std::string str = "Hello World";
std::string substr = "World";
size_t pos = str.find(substr);
if (pos != std::string::npos) {
std::cout << "Substring found at position: " << pos << std::endl;
} else {
std::cout << "Substring not found." << std::endl;
}
Implementing Custom String Search Functions
There are scenarios where a custom substring search might be necessary. This could be for specific needs, such as case-insensitivity or advanced pattern matching. Here's a pseudo code example that outlines a basic substring search:
function custom_strstr(haystack, needle) {
for (each character in haystack) {
if (current character matches first character of needle) {
if (whole needle matches) {
return starting position
}
}
}
return null; // If no match found
}
This pseudo code illustrates how to approach building a custom search algorithm, adapting it as necessary based on your project's requirements.
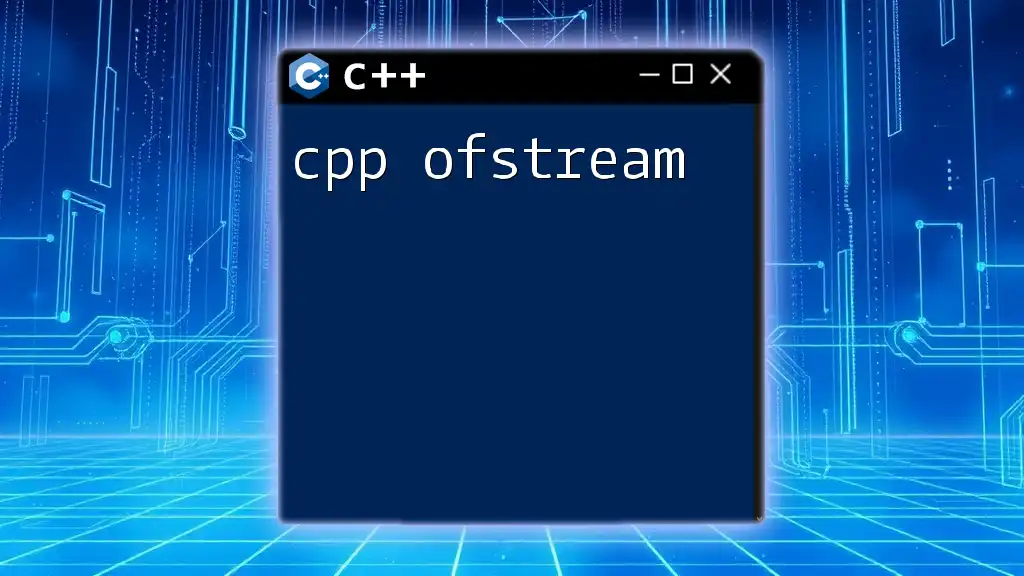
Conclusion
The `strstr` function serves a critical role in string manipulation for C++ developers. It simplifies the task of substring searching while offering a robust solution for many common problems. By leveraging `strstr` effectively—while being aware of its strengths, limitations, and potential pitfalls—you can improve the quality and efficiency of your string handling operations in C++.
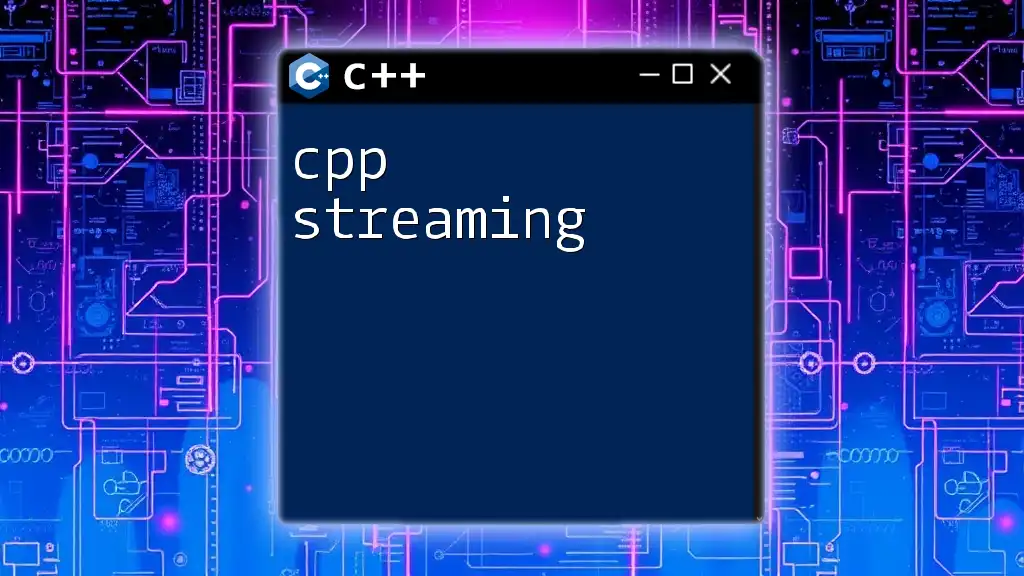
FAQs about `strstr`
What happens if the needle is an empty string?
When the `needle` is an empty string, `strstr` will always return a pointer to the beginning of the `haystack`.
Is `strstr` safe to use with user-generated input?
While `strstr` is safe with properly validated input, always ensure that inputs are checked for null values before passing them to the function to avoid undefined behavior.
How do I check if a substring appears multiple times?
You can use a loop with `strstr` to iterate through the `haystack`, incrementing the search pointer each time a match is found, as shown in the earlier `strstr` loop example.
By mastering `strstr`, you empower your C++ applications with a foundational tool for efficient substring searching and management—a skill that is invaluable in the developer's toolkit.