The C++ system call allows you to execute shell commands from within a C++ program, providing a way to interact with the operating system's command-line interface.
#include <cstdlib>
int main() {
system("ls -l"); // Execute the 'ls -l' command to list files in the current directory
return 0;
}
Understanding System Calls in C++
What is a System Call?
A system call is a fundamental interface between a program and the operating system. It provides a way for user programs to request services from the kernel without needing to understand the intricacies of the operating system's internal workings. System calls enable applications to perform functions such as reading and writing files, managing processes, and interacting with hardware.
In essence, system calls serve as a bridge for user-space programs to interact with the kernel-space, allowing higher-level programming languages like C++ to function effectively in a complex operating environment.
Why Use System Calls in C++?
Using system calls in C++ has several advantages. They provide direct access to the underlying system features, making them incredibly powerful for applications that require:
- Direct control over system resources.
- Enhanced performance by minimizing the overhead of higher-level abstractions.
- Greater efficiency for operations like I/O, as system calls can be more optimized than library functions.
Common scenarios where system calls are particularly beneficial include file management, network programming, and process control.

The Basics of C++ System Calls
How C++ Interfaces with System Calls
C++ leverages the capabilities of the C programming language, as many system calls originate from C libraries. The C++ Standard Library does not directly implement system calls but offers abstractions around them. Understanding how this interface works is crucial for effectively using system calls in C++.
Key System Call Categories
System calls in C++ can be categorized into several types:
- I/O Operations: System calls that handle input and output processes. Examples include `read()` and `write()`.
- Process Control: Involves creating and managing processes, with calls like `fork()` and `exec()`.
- Memory Management: These calls handle the allocation and deallocation of memory, such as `mmap()` and `brk()`.

Essential System Calls in C++
File Handling through System Calls
Opening and Closing Files with `open()` and `close()`
File operations are fundamental in programming, and system calls provide efficient mechanisms for file manipulation. The `open()` syscall is used to open a file and returns a file descriptor, while the `close()` syscall is used to close the opened file.
Here’s how you can use `open()` and `close()`:
#include <fcntl.h>
#include <unistd.h>
int main() {
int fd = open("example.txt", O_RDONLY);
if (fd == -1) {
// Handle error
}
// Use the file (perform operations using fd)
close(fd);
return 0;
}
In this example, `open()` attempts to open "example.txt" for reading. If the file descriptor returned is `-1`, it indicates an error occurred during the opening process.
Reading from Files using `read()`
To read data from a file, the `read()` system call is employed. It reads a specified number of bytes from the file descriptor into a buffer.
Here’s an example:
#include <fcntl.h>
#include <unistd.h>
#include <iostream>
int main() {
int fd = open("example.txt", O_RDONLY);
char buffer[128];
ssize_t bytesRead = read(fd, buffer, sizeof(buffer));
if (bytesRead >= 0) {
// Successfully read data; process buffer
} else {
// Handle read error
}
close(fd);
return 0;
}
This snippet illustrates how to read data into a `buffer` and handle potential errors from the `read()` call.
Creating and Managing Processes
Process Creation with `fork()`
Creating processes is a common task in system programming. The `fork()` system call creates a new process, known as a child process, that runs concurrently with the parent process.
Here’s a simple illustration:
#include <unistd.h>
#include <iostream>
int main() {
pid_t pid = fork();
if (pid == 0) {
std::cout << "Child process" << std::endl;
} else {
std::cout << "Parent process" << std::endl;
}
return 0;
}
In this example, the program checks if the `pid` returned from `fork()` is zero to determine whether it is executing in the child or parent process.
Executing New Programs using `exec()`
The `exec()` family of functions allows a process to execute a new program. This system call replaces the current process image with a new process image.
Here’s how to use `exec()`:
#include <unistd.h>
#include <iostream>
int main() {
char *args[] = {(char *)"/bin/ls", (char *)"-l", NULL};
execvp(args[0], args);
std::cerr << "Exec failed" << std::endl;
return -1;
}
In this example, the program attempts to execute the `ls -l` command. If the execution fails, it prints an error message.

Error Handling with System Calls
Understanding Return Values
When making system calls, it's essential to understand their return values. For most system calls, a return value of `-1` indicates an error occurred, while a non-negative value usually indicates success. Always checking these return values is crucial for robustness in your applications.
Using `errno` for Diagnostics
The `errno` variable is set by system calls and library functions in the event of an error to indicate what went wrong. Leveraging `errno` allows developers to implement effective error handling strategies.
Here's an example demonstrating how to use `errno`:
#include <fcntl.h>
#include <unistd.h>
#include <cerrno>
#include <iostream>
int main() {
int fd = open("nonexistent.txt", O_RDONLY);
if (fd == -1) {
std::cerr << "Error opening file: " << strerror(errno) << std::endl;
}
return 0;
}
This code tries to open a file that doesn't exist and uses `strerror(errno)` to print out a human-readable error message.

Best Practices for Using C++ System Calls
Writing Safe and Efficient Code
When using system calls, it is vital to adhere to best practices to avoid common pitfalls. Always validate the state of your program and handle potential errors gracefully. Performing operations in a manner that minimizes resource leaks—like forgetting to close file descriptors—can significantly improve both safety and performance.
Combining C++ Features with System Calls
C++ provides powerful abstractions like Standard Template Library (STL) containers and smart pointers. You can effectively combine these modern C++ features with system calls to create clean, efficient code. For instance, consider using `std::vector` for handling dynamic arrays in concert with `read()` to buffer file input.
By understanding the interplay between system calls and C++ abstractions, developers can optimize their code while maintaining readability and maintainability.
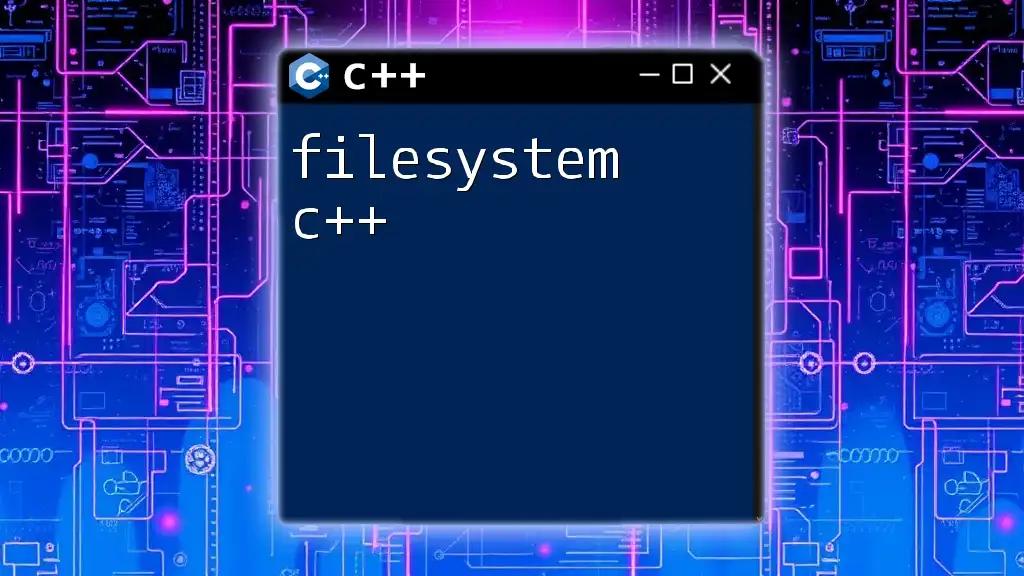
Conclusion
Recap of Key Points
In this comprehensive guide on cpp system calls, we explored the essential role that system calls play in enabling C++ programs to communicate effectively with the operating system. Understanding how to utilize these calls empowers developers with direct control over system resources, resulting in more performant and capable applications.
Further Learning Resources
For those eager to dive deeper, numerous books, websites, and online courses can further enhance your understanding of C++ and system programming. Engaging with community forums and discussion groups can also foster improvements in your C++ skills and troubleshooting abilities.