C++ type casting allows you to convert a variable from one data type to another, facilitating operations that require different type compatibility.
#include <iostream>
using namespace std;
int main() {
double pi = 3.14;
int intPi = static_cast<int>(pi); // Using static_cast for type casting
cout << "The integer value of pi is: " << intPi << endl; // Output: 3
return 0;
}
What is cpp type casting?
Type casting in C++ is the process of converting one data type into another. This can be particularly useful when you need to perform operations on different types of data. By employing cpp type casting, you can ensure that your operations behave as expected, avoid data loss, and optimize memory management.
Why Type Casting is Important in C++
Understanding and utilizing cpp type casting is crucial for several reasons:
- Enables operations across different data types, which enhances the flexibility of your code.
- Helps maintain data integrity, especially when dealing with arithmetic operations or function parameters.
- Optimizes memory usage by selecting appropriate data types for variables based on context and requirements.
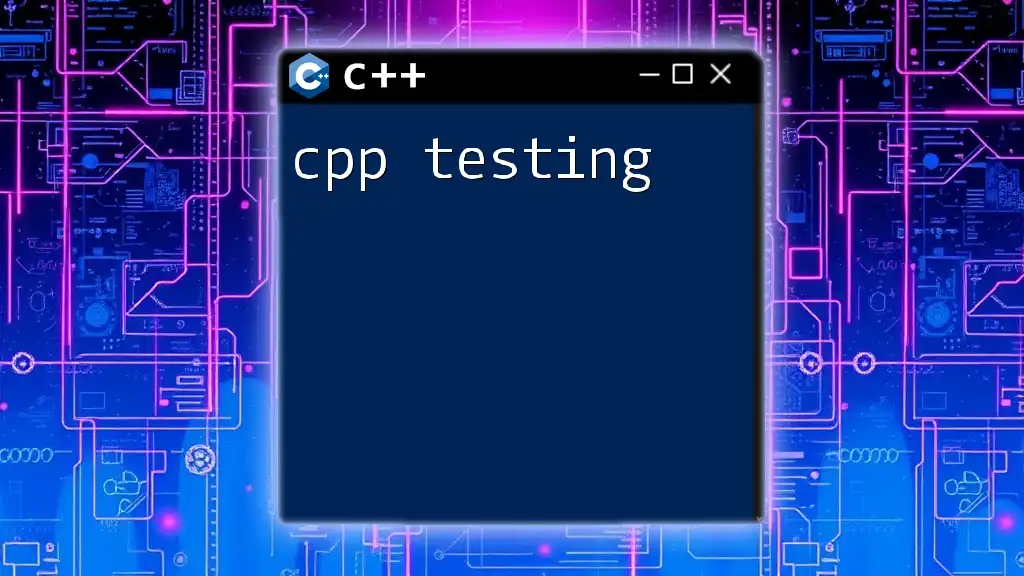
Types of Type Casting in C++
Overview of Casting Types in C++
C++ offers multiple casting types to facilitate the conversion of data types. Each type serves a specific purpose, and understanding them can significantly improve your programming practices.
static_cast
Definition and Purpose
`static_cast` is a versatile casting operator that allows for safe and explicit conversions between compatible types. It's especially useful for conversions that don’t involve runtime checks.
Syntax and Use Cases
float fValue = 3.14;
int iValue = static_cast<int>(fValue);
In this example, the floating-point value is explicitly converted to an integer, truncating any decimal.
Examples and Applications
-
Type Conversion from Float to Int: This use case is common when you want to discard the decimal part and keep only the whole number for further processing.
-
Pointer Conversions: You can convert pointers to base class types safely:
class Base {};
class Derived : public Base {};
Derived* derived = new Derived();
Base* base = static_cast<Base*>(derived);
dynamic_cast
Definition and Purpose
`dynamic_cast` is employed for safe downcasting, especially when working with polymorphism. It checks the type at runtime and ensures the conversion is valid.
Syntax and Use Cases
Base* basePtr = new Derived();
Derived* derivedPtr = dynamic_cast<Derived*>(basePtr);
if (derivedPtr) {
// Safe to use derivedPtr
}
Examples and Applications
-
Safe Downcasting: This is crucial in class hierarchies. If `basePtr` doesn't actually point to a `Derived` object, `dynamic_cast` will return a `nullptr`, preventing runtime errors.
-
Handling Polymorphism: When working with virtual methods in derived classes, `dynamic_cast` lets you invoke the correct methods based on the object's actual type.
const_cast
Definition and Purpose
`const_cast` allows you to change the constness of a variable. This is particularly useful when interfacing with legacy APIs that expect non-const pointers.
Syntax and Use Cases
const int* ptr = new const int(42);
int* modifiablePtr = const_cast<int*>(ptr);
Examples and Applications
-
Modifying Const-Correctness: If you need to pass a pointer to a function that expects a non-const pointer, `const_cast` enables this. However, use this with caution, as modifying the object pointed to can lead to undefined behavior if it was originally declared as const.
-
Interfacing Legacy Code: When adapting older C libraries or APIs, `const_cast` can facilitate smoother integration.
reinterpret_cast
Definition and Purpose
`reinterpret_cast` performs low-level type conversions, allowing you to treat a data type as another unrelated type. It’s powerful but should be used sparingly due to the potential for undefined behavior.
Syntax and Use Cases
int* pInt = new int(42);
char* pChar = reinterpret_cast<char*>(pInt);
Examples and Applications
-
Pointer Manipulation: This cast is often used in system programming or when working with hardware-level operations.
-
Converting Between Unrelated Pointer Types: This cast allows for experimentation with memory layouts but should be approached with caution to avoid misinterpretations of data.
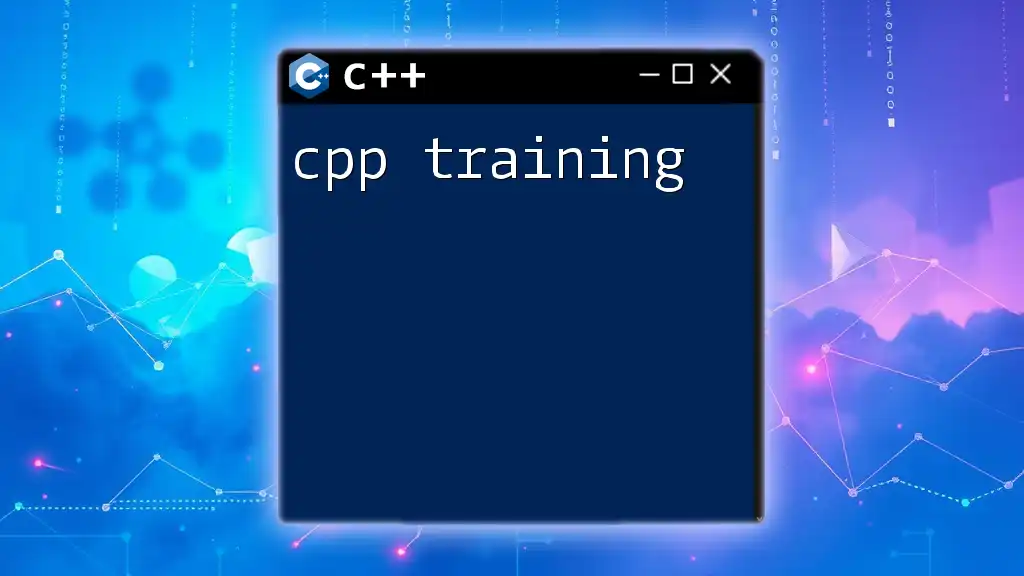
Safe vs. Unsafe Casting in C++
Identifying Safe Casts
Safe casts are those that preserve type integrity and involve minimal risk. `static_cast` and `dynamic_cast` are typically considered safe options. They ensure that the conversion can occur without the potential for type mismatches or data corruption.
Risks Associated with Unsafe Casts
Unsafe casts, notably those performed with `reinterpret_cast`, can lead to significant issues. This type of cast should be reserved for scenarios where you fully understand the implications and can manage the risks involved.
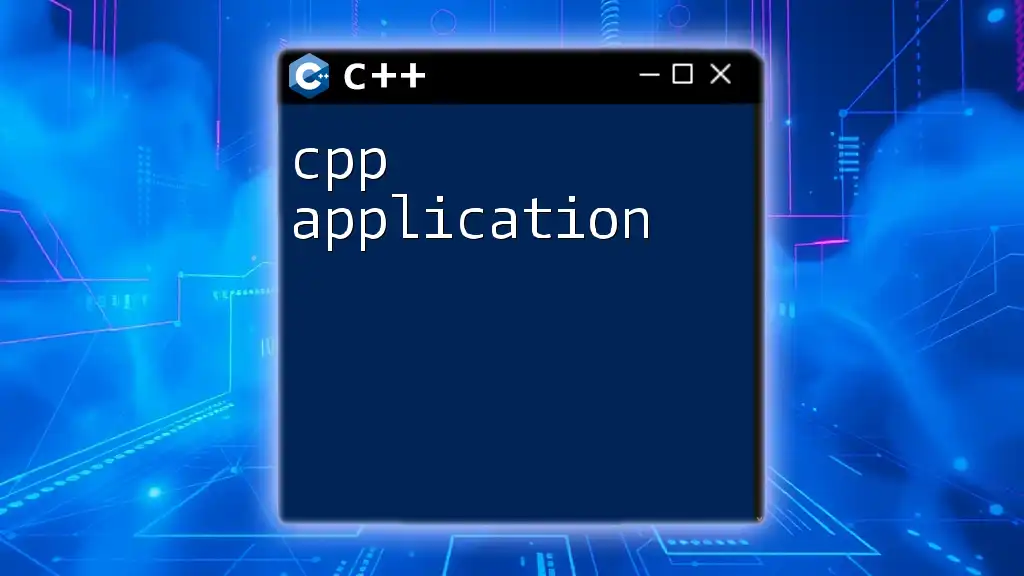
Best Practices for Type Casting in C++
When working with cpp type casting, adhere to these best practices to maintain code clarity and safety:
-
Prefer `static_cast` and `dynamic_cast`: Always use the most appropriate cast for the situation to improve readability and safety.
-
Limit the Use of `reinterpret_cast`: Avoid using `reinterpret_cast` unless absolutely necessary. It can lead to intricate bugs that are challenging to diagnose.
-
Focus on Design: Aim to minimize the need for type casting by designing classes and interfaces that leverage polymorphism and templates effectively.
When to Avoid Casting
It’s often better to avoid casting altogether when you can redesign your code to work with a single type, reducing confusion and potential bugs. Explicit casting should be a last resort, deployed only when necessary and beneficial.
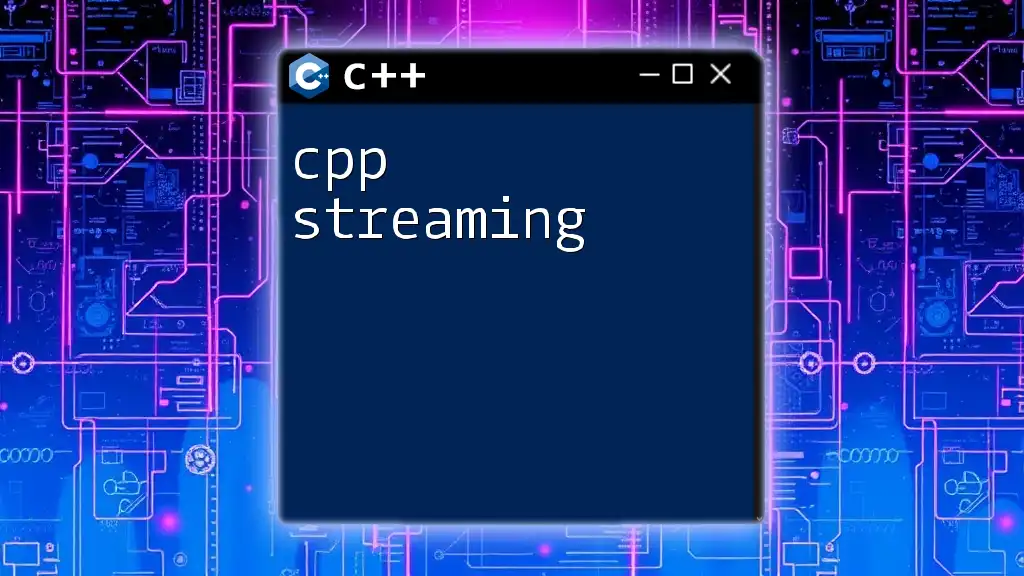
Conclusion
In your journey of mastering cpp type casting, understanding the various casting types—`static_cast`, `dynamic_cast`, `const_cast`, and `reinterpret_cast`—is fundamental. By grasping when and how to use each cast effectively, you can enhance your programming skills and write robust, maintainable code. Embracing type safety helps ensure that your applications run smoothly, providing a better experience for users and easier maintenance for developers. As you continue to learn, explore advanced C++ functionalities and watch your competence grow!
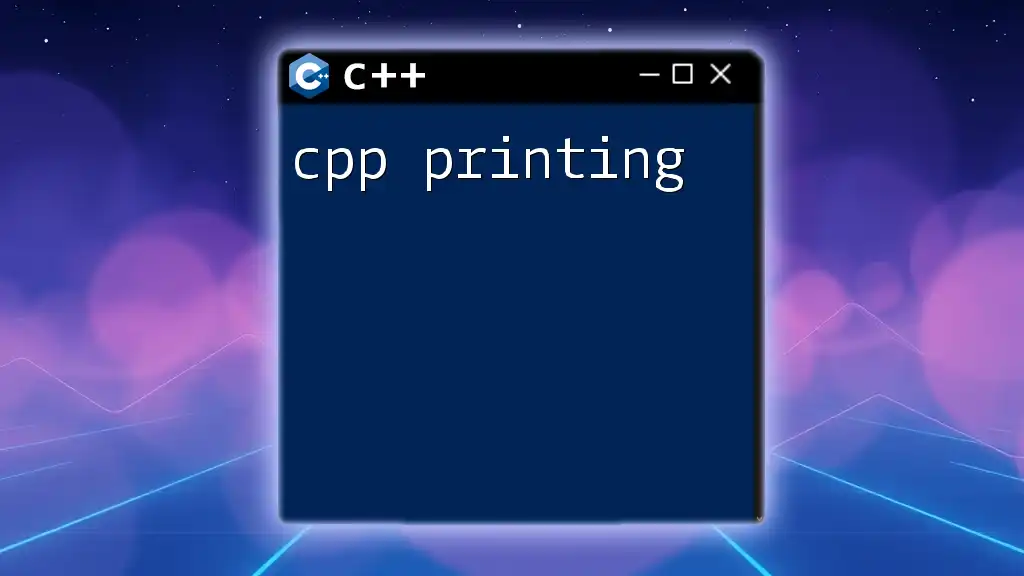
Code Snippets Appendix
This section includes complete programs showcasing each type of cast discussed, allowing for practical, hands-on learning. Feel free to compile and run these examples to solidify your understanding of cpp type casting.