CPP timing refers to measuring the execution time of code snippets in C++, which helps optimize performance. Here’s a simple example demonstrating how to measure the time taken to execute a piece of code using the `<chrono>` library:
#include <iostream>
#include <chrono>
int main() {
auto start = std::chrono::high_resolution_clock::now();
// Code to time (e.g., a simple loop)
for (volatile int i = 0; i < 1000000; ++i); // volatile to prevent optimization
auto end = std::chrono::high_resolution_clock::now();
std::chrono::duration<double> duration = end - start;
std::cout << "Time taken: " << duration.count() << " seconds\n";
return 0;
}
Understanding C++ Timing
What is C++ Timing?
C++ timing refers to the measurement of code execution duration within C++ programs. It is crucial for performance-sensitive applications where understanding how long specific operations take can greatly impact overall efficiency. Precise timing can help optimize code, allowing developers to identify bottlenecks and streamline processes.
Use Cases for C++ Timing
There are various scenarios where cpp timing plays an important role:
- Performance Measurement: Timing can help determine how long portions of code take to execute, enabling developers to refine algorithms and improve application performance.
- Benchmarking Algorithms: By measuring the execution time of different algorithms under the same conditions, developers can make informed decisions about which algorithms to use for optimal performance.
- Event Timing in Games and Real-time Systems: Timing is essential for synchronizing events in game development or real-time applications, where even small delays can significantly affect user experience.
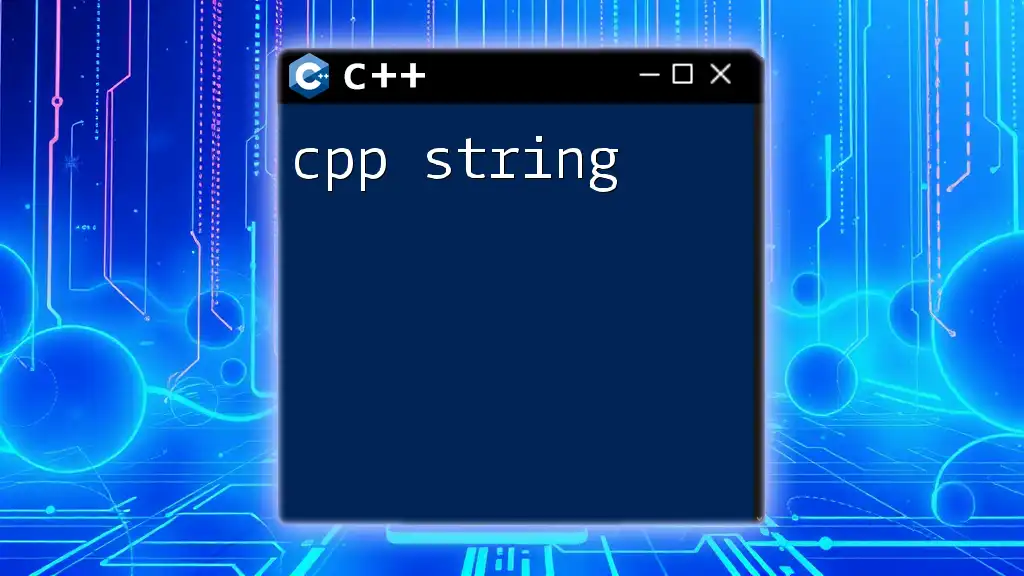
Tools and Libraries for Timing in C++
The `<chrono>` Library
The C++ standard library provides the `<chrono>` library, which is a powerful tool for handling time-related tasks.
Key Components:
- Clock: Represents the passage of time and provides various types of clocks such as `system_clock`, `steady_clock`, and `high_resolution_clock`.
- Duration: Represents a span of time and can be expressed in various units (e.g., seconds, milliseconds, microseconds).
- Time Point: Represents a specific point in time, which can be used for calculations and comparisons.
Alternative Libraries
While `<chrono>` is widely used, other libraries can complement or enhance timing functionalities:
-
Boost.Chrono: This extension of the C++ standard library offers similar features with additional flexibility and functionality. It is recommended for more complex timing operations or when working within the Boost framework.
-
GTest Timing Utilities: Google Test provides tools specifically designed to measure timing within unit tests. This is particularly useful for verifying the performance of functions against expected benchmarks.
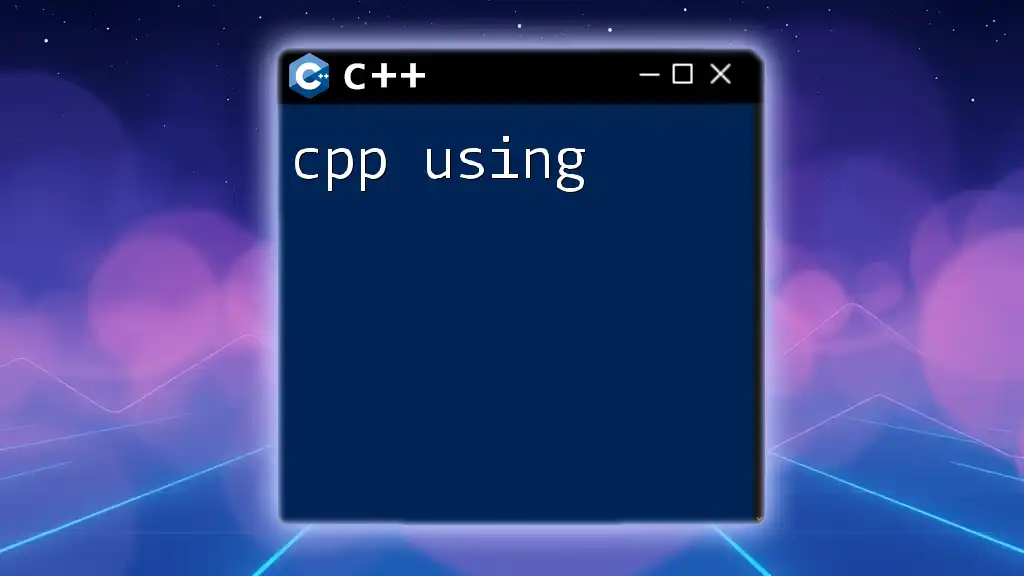
Getting Started with Timing Code
Basics of Timing
To begin using `cpp timing`, you must include the `<chrono>` header in your C++ program. This makes all the timing functionalities accessible:
#include <chrono>
Simple Timing Example
A basic timing example illustrates how to measure execution duration. By capturing the start and end times, the duration of the operations in between can be calculated effectively:
auto start = std::chrono::high_resolution_clock::now();
// Code to be measured
auto end = std::chrono::high_resolution_clock::now();
std::chrono::duration<double> duration = end - start;
std::cout << "Execution Time: " << duration.count() << " seconds." << std::endl;
This code snippet uses the high-resolution clock for more precise measurements, displaying the execution time in seconds.
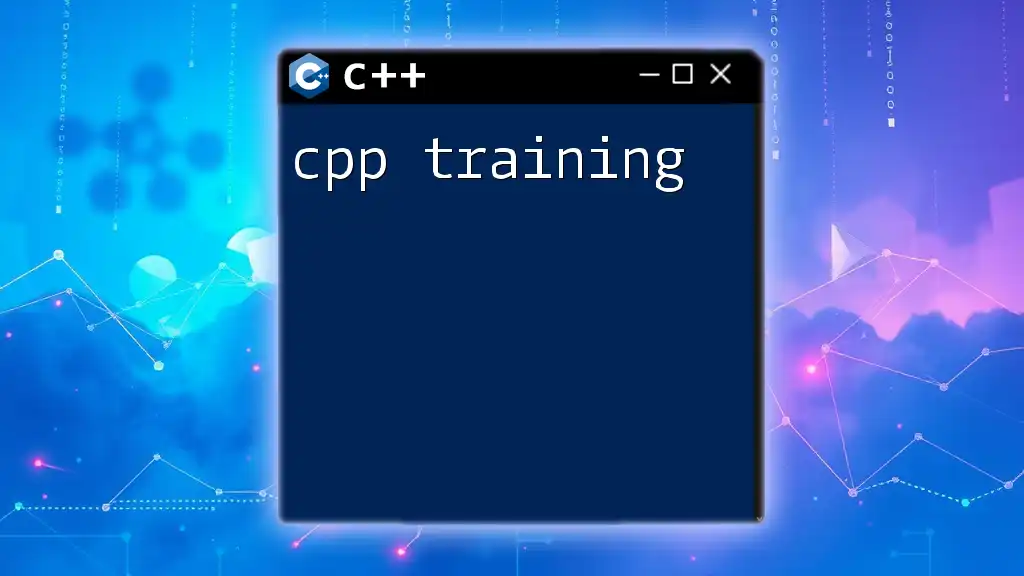
Advanced Timing Techniques
Measuring Function Execution Time
To streamline timing measurements, you can create a utility function that wraps the desired code:
template <typename Func>
void measure_time(Func f) {
auto start = std::chrono::high_resolution_clock::now();
f();
auto end = std::chrono::high_resolution_clock::now();
std::chrono::duration<double> duration = end - start;
std::cout << "Function Execution Time: " << duration.count() << " seconds." << std::endl;
}
This utility allows for easy timing of any function passed to it, without repeating the timing logic.
Comparing Code Snippets
Measuring the execution time of different implementations can provide valuable insights:
measure_time([] {
// Code Snippet A
});
measure_time([] {
// Code Snippet B
});
This approach enables developers to compare various algorithms directly, helping inform decisions based on empirical data.
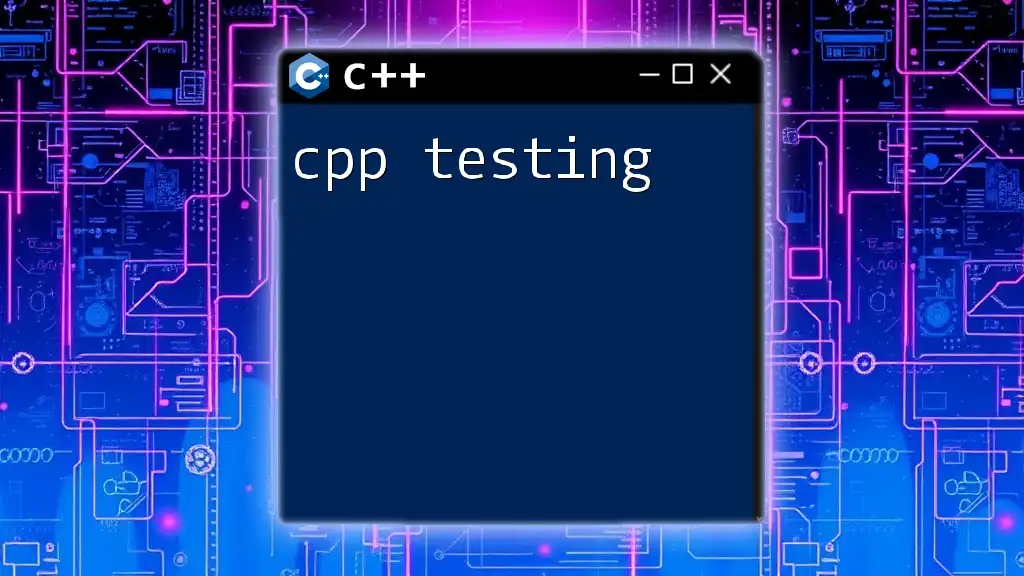
Handling Timing Precision and Performance
Best Practices for Accurate Timing
To ensure accurate timing results, consider these best practices:
- Perform Repeated Measurements: Running tests multiple times and averaging the results can minimize anomalies caused by system load or other factors.
- Avoid Compiler Optimizations: Sometimes, the compiler may optimize away code that it perceives as unnecessary. You can prevent this by including certain operations (such as dummy operations) in your timed sections.
- Use High-Resolution Clocks: The `high_resolution_clock` provides the most precise time measurements available, making it ideal for performance-sensitive tasks.
To reliably introduce some delay, you might consider using:
std::this_thread::sleep_for(std::chrono::microseconds(1));
Performance Overhead Considerations
While timing is crucial, it’s important to keep in mind the potential performance overhead of measurement. Excessive timing calls can disrupt the very performance you are trying to measure; hence, aim to minimize the impact of your timing code.
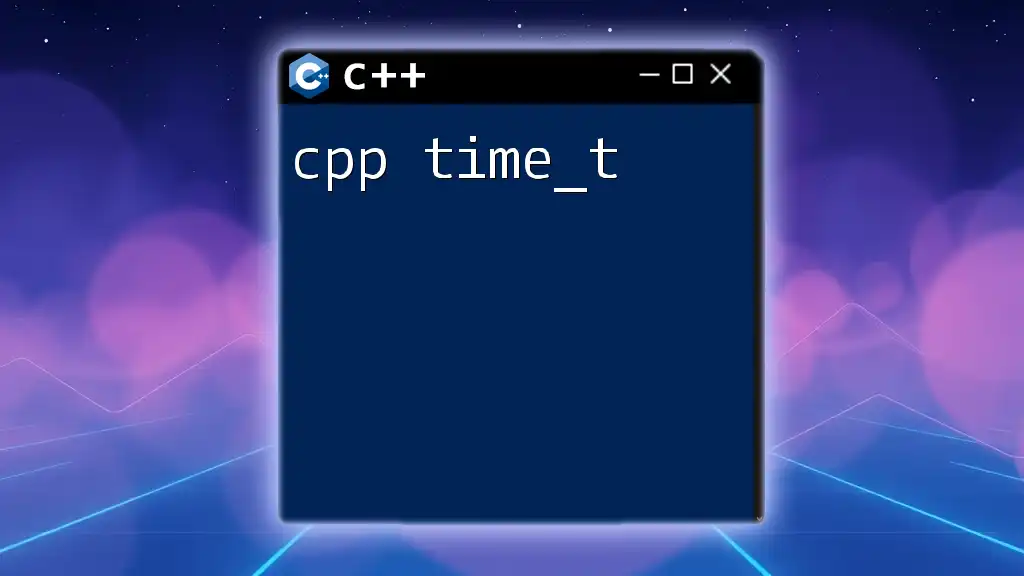
Real-World Applications
Timing in Game Development
In game development, managing time accurately is essential for delivering a smooth player experience. Game engines often rely on precise frame timings to handle animations and physics.
For example:
auto frame_start = std::chrono::high_resolution_clock::now();
// Game update logic
auto frame_end = std::chrono::high_resolution_clock::now();
This simple measurement can help developers ensure that the game is running at the intended frame rate.
Timing in Data Processing
In data processing applications, timing can inform developers about batch processing times or the efficiency of various data manipulation techniques. By timing different algorithms, you can make informed adjustments to enhance throughput and performance.
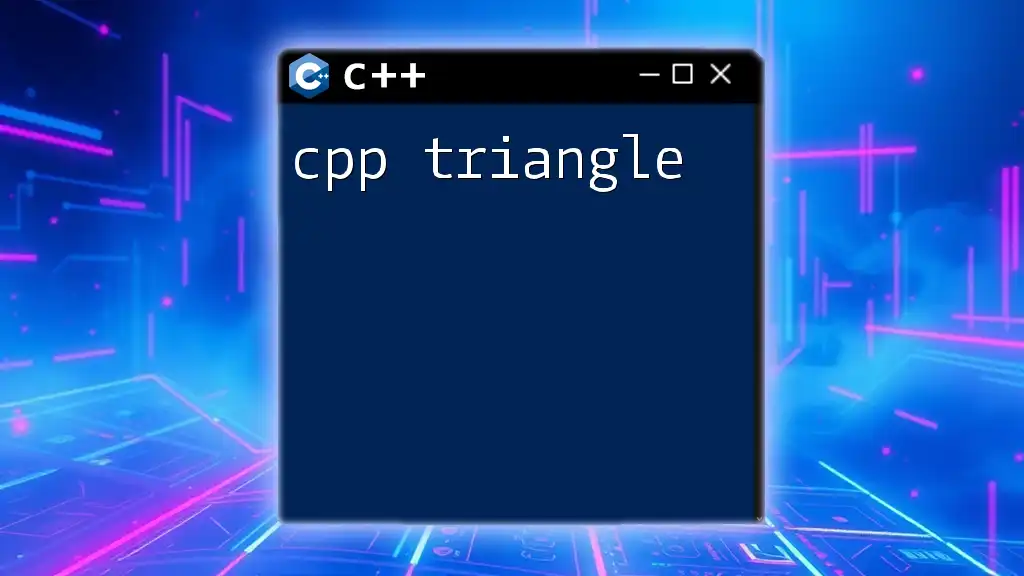
Conclusion
Recap of Key Takeaways
Understanding cpp timing is essential for optimizing C++ applications and ensuring efficient performance. Employing the right tools and libraries, writing reusable timing code, and adhering to best practices can significantly improve your programming outcomes.
Final Thoughts
Experimenting with timing in real projects will deepen your understanding of C++ performance characteristics. Embrace the opportunity to explore various timing techniques, libraries, and real-world applications to improve your coding efficiency and effectiveness.