"CPP WiFi" refers to the use of C++ programming to manage WiFi connections and related functionalities, enabling developers to create applications that can connect, disconnect, or perform operations on WiFi networks.
Here's a simple example code snippet for scanning available WiFi networks using C++:
#include <WiFi.h>
void setup() {
Serial.begin(115200);
WiFi.mode(WIFI_STA);
Serial.println("Scanning for WiFi networks...");
int n = WiFi.scanNetworks();
Serial.println("Scan complete.");
for (int i = 0; i < n; i++) {
Serial.print(i + 1);
Serial.print(": ");
Serial.println(WiFi.SSID(i));
delay(10);
}
}
void loop() {
// Do nothing here
}
Understanding WiFi in C++
What is WiFi?
WiFi, short for Wireless Fidelity, is a technology that allows electronic devices to connect to a wireless LAN (Local Area Network) primarily using radio waves. It has become an essential component of modern computing, enabling functionalities like internet browsing, communication, and data exchange without the need for wired connections. Integrating WiFi capabilities into applications can significantly enhance user experience, making devices more mobile and versatile.
Overview of C++ in Network Programming
C++ is widely used in developing software applications due to its performance and control over system resources. In network programming, C++ provides unique capabilities to interact with various networking protocols and manage WiFi functionalities. Key libraries employed for WiFi interaction in C++ include Boost.Asio, Qt Network, and several others. Each library brings its strengths, facilitating tasks such as asynchronous I/O operations, seamless UI integration, and robust connectivity management.
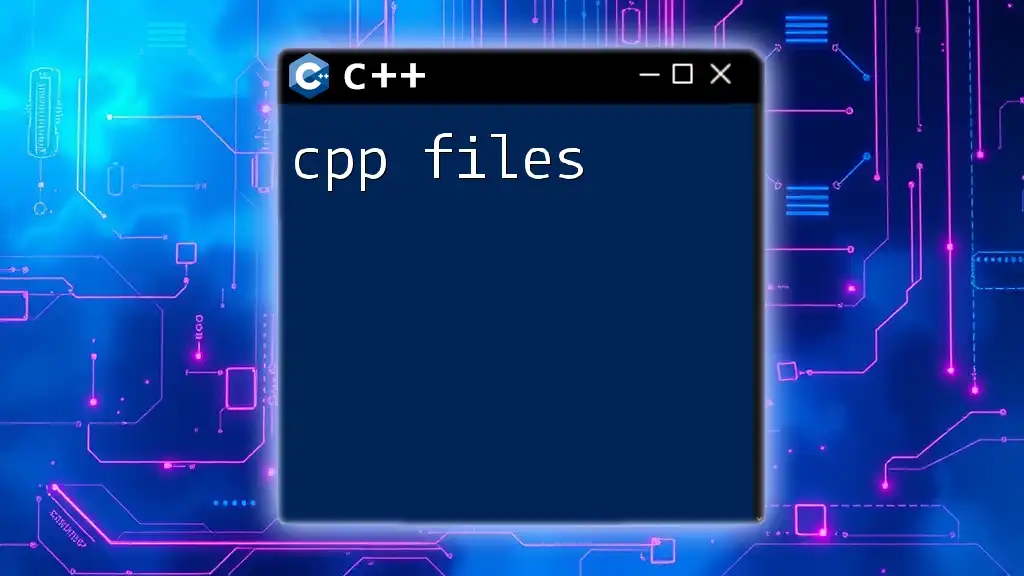
Setting Up Your C++ Environment for WiFi Programming
Required Libraries and Tools
To effectively work with C++ and WiFi, you will need specific libraries:
- Boost.Asio: A cross-platform C++ library that provides a consistent asynchronous I/O model. Perfect for network programming, it simplifies socket programming and resource management.
- Qt Network: Part of the Qt framework, this library offers a variety of functionalities for handling network communications, including support for TCP and UDP protocols.
- Other Libraries: You might also explore libraries like POCO for ease of access to network functionalities and libcurl for handling data transfers over various protocols.
Installing Dependencies
To set up your environment, follow these steps to install the necessary libraries:
-
Install Boost.Asio:
- For Mac, use Homebrew: `brew install boost`
- For Linux, use your package manager, e.g., `sudo apt-get install libboost-all-dev`
- For Windows, download the binaries from [Boost's website](https://www.boost.org/) and set up in your C++ environment.
-
Install Qt:
- Download from [Qt's website](https://www.qt.io/download) and follow the installation instructions.
- Make sure to select the 'Qt Network' component during the installation process.
-
Validate the installation with simple `#include` statements in your code:
#include <boost/asio.hpp>
// or
#include <QtNetwork>
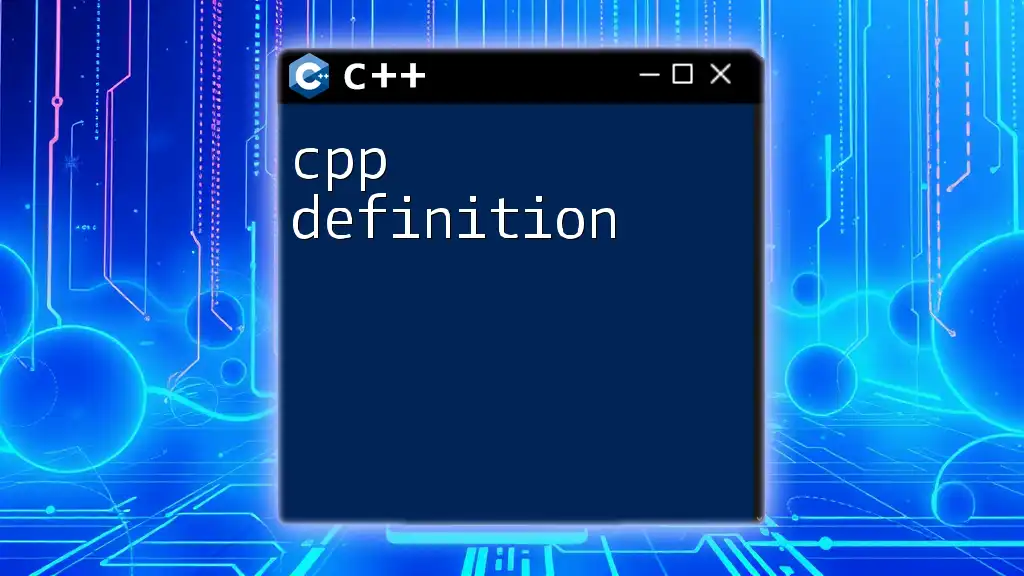
Connecting to a WiFi Network
Scanning Available Networks
To connect to a WiFi network, you first need to scan for available networks. This can be accomplished using libraries such as Boost or Qt.
Here’s how you can scan for networks using Qt:
#include <QNetworkConfigurationManager>
QNetworkConfigurationManager manager;
foreach (const QNetworkConfiguration &config, manager.allConfigurations(QNetworkConfiguration::Active)) {
qDebug() << "Available networks: " << config.name();
}
This code snippet initializes a network configuration manager and prints the names of available networks.
Connecting to a WiFi Network
Once you have the list of available networks, connecting to one involves specifying the SSID and password, if applicable. Here’s an example of connecting to a WiFi network using `WPA2` security via a command-line utility that can also be executed from a C++ program:
#include <cstdlib>
void connectToWifi(const std::string& ssid, const std::string& password) {
std::string command = "nmcli dev wifi connect '" + ssid + "' password '" + password + "'";
system(command.c_str());
}
// Usage
connectToWifi("MyNetwork", "MyPassword");
In this example, we use `nmcli`, which is a command-line interface for NetworkManager. You can customize it based on the API or the tool you’re using for network operations.
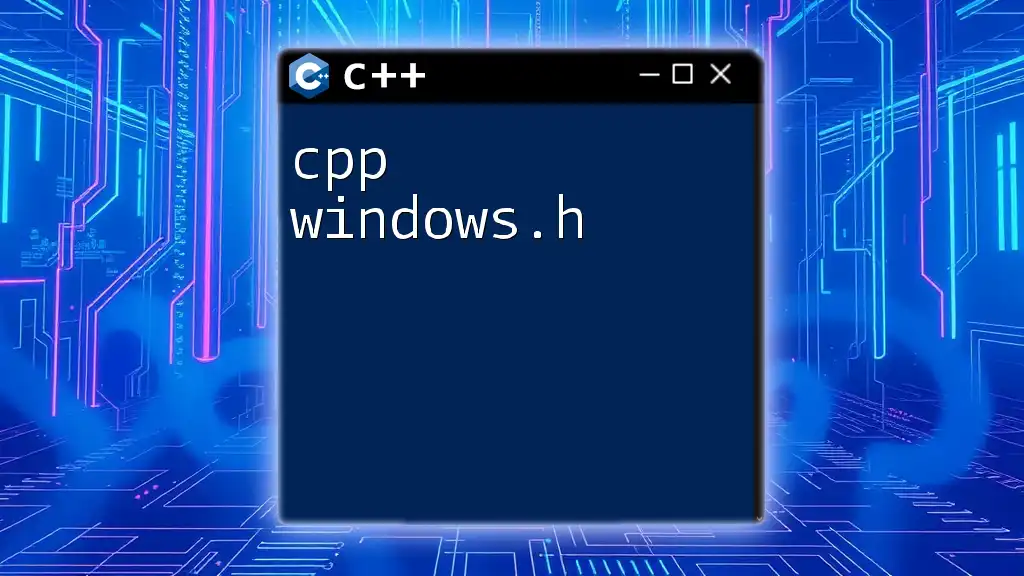
Managing WiFi Network Settings
Retrieving Network Information
Retrieving the current network information such as SSID, IP address, and signal strength is critical for managing network connections effectively. Here’s how you can fetch and display network settings:
#include <QNetworkInterface>
foreach (const QNetworkInterface &interface, QNetworkInterface::allInterfaces()) {
if (interface.flags() & QNetworkInterface::IsLoopBack) continue;
qDebug() << "Network Interface: " << interface.humanReadableName();
qDebug() << "IP Address: " << interface.addressEntries().first().ip();
}
This code snippet filters out the loopback interface and retrieves IP address information from other interfaces, displaying it in the debug output.
Disconnecting from a Network
Disconnecting from a WiFi network can also be done using system commands or library functions. A typical method involves invoking `nmcli` as follows:
void disconnectFromWifi() {
system("nmcli device disconnect wlan0");
}
// Usage
disconnectFromWifi();
This effectively disconnects the specified wireless interface from the network, allowing your application to manage WiFi connections programmatically.
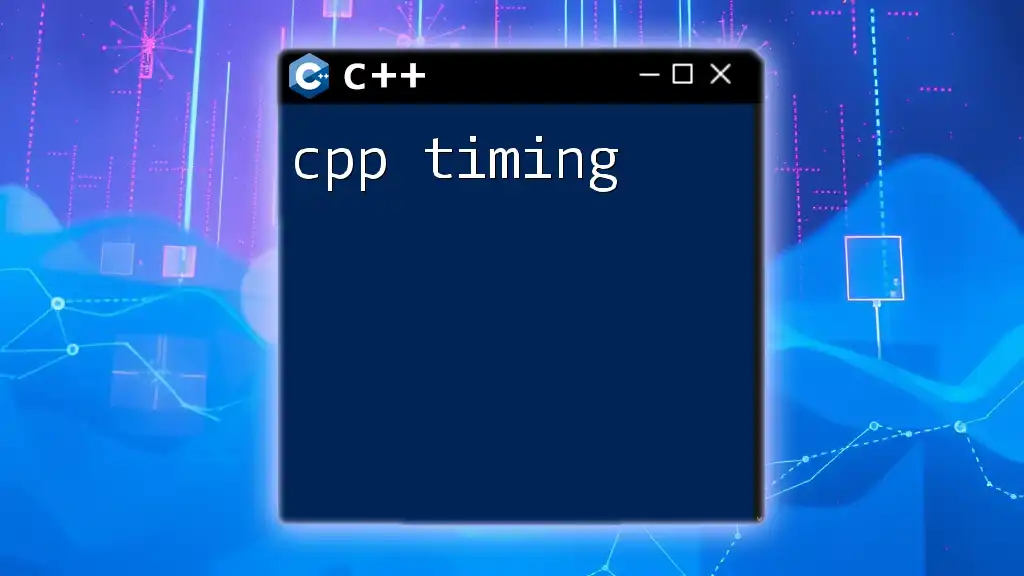
Error Handling in C++ WiFi Applications
Common Errors and Troubleshooting
When working with C++ and WiFi, several common errors may arise, such as:
- Connection Timeouts: Often caused by poor signal strength or incorrect credentials.
- Module Not Found: This usually indicates that the required library is not properly installed.
Implementing Effective Error Handling
Error handling is crucial in network programming. Utilizing exceptions and error handling strategies can enhance your application's robustness. Here is an example of how to manage exceptions while connecting:
try {
connectToWifi("MyNetwork", "MyPassword");
} catch (const std::exception &e) {
std::cerr << "An error occurred: " << e.what() << std::endl;
}
This snippet captures exceptions thrown during the connection process, allowing you to respond appropriately and maintain application stability.
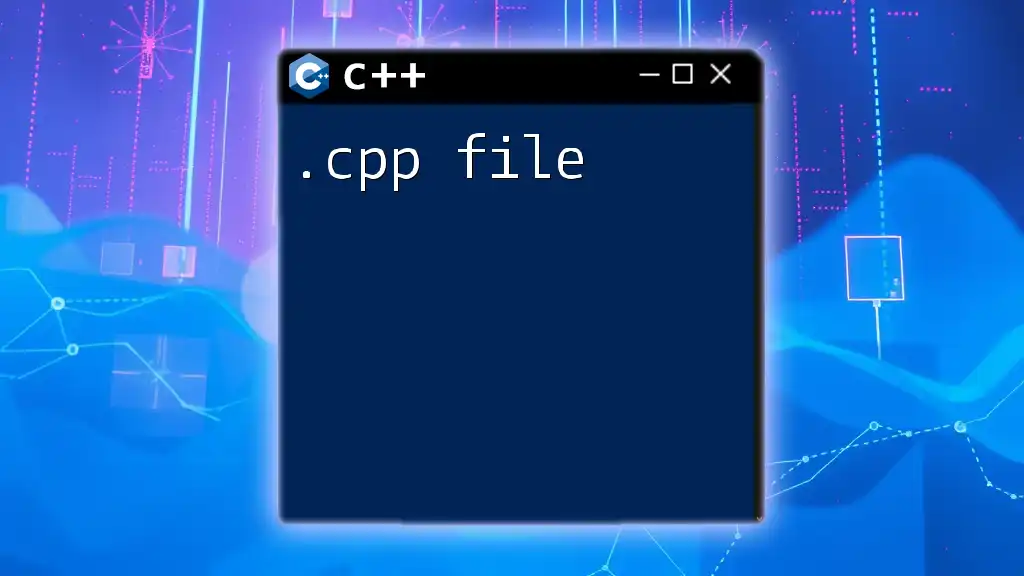
Advanced Topics in C++ WiFi Programming
Implementing Security Protocols
Integrating security measures in your WiFi applications is fundamental. Utilizing WPA2 or WPA3 standards ensures that data transmitted over the network is encrypted and secure. Make sure the libraries you use support secure connections and implement required authentication protocols.
Creating a WiFi Hotspot
Creating a personal WiFi hotspot programmatically using C++ can be a challenging task, typically involving system networking commands or specialized libraries. Here is a basic outline of how you might accomplish this using `nmcli`:
void createHotspot(const std::string& ssid, const std::string& password) {
std::string command = "nmcli dev wifi hotspot ifname wlan0 ssid '" + ssid + "' password '" + password + "'";
system(command.c_str());
}
// Usage
createHotspot("MyHotspot", "HotspotPassword");
This function initializes a WiFi hotspot using the specified SSID and password.
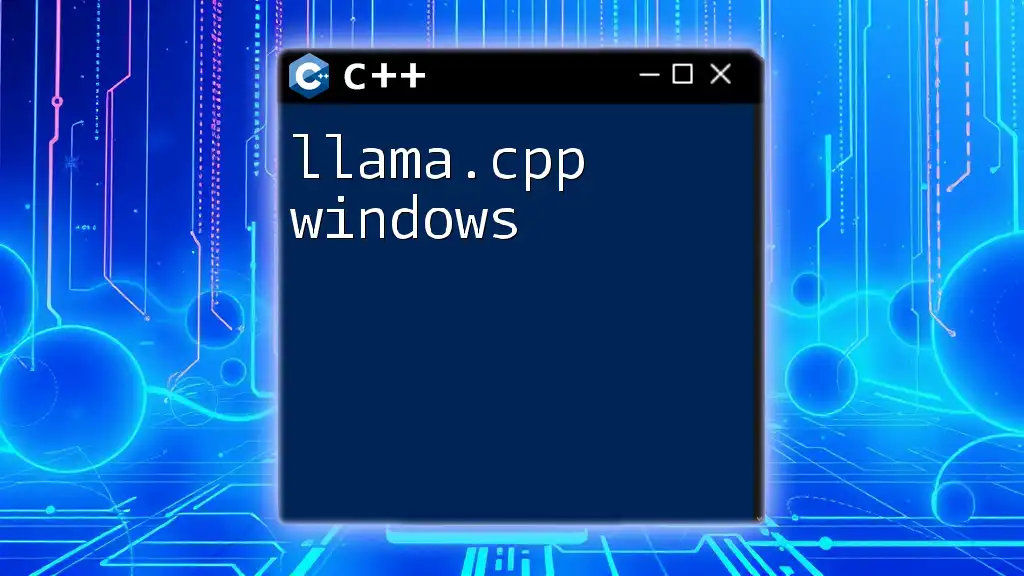
Use Cases of C++ in WiFi-Connected Applications
IoT Applications
C++ is frequently employed in developing IoT devices due to its efficiency and performance advantages. These devices can leverage WiFi connectivity for remote monitoring, control, and automation. For instance, you might create a C++ application that interfaces with sensors and transmits data via WiFi for real-time analysis.
Desktop and Mobile Applications
C++ can significantly enhance user experience in desktop and mobile applications where WiFi connectivity is pivotal. Applications that handle file transfers, streaming, or remote access all benefit from efficient network operations achieved through well-structured C++ code.
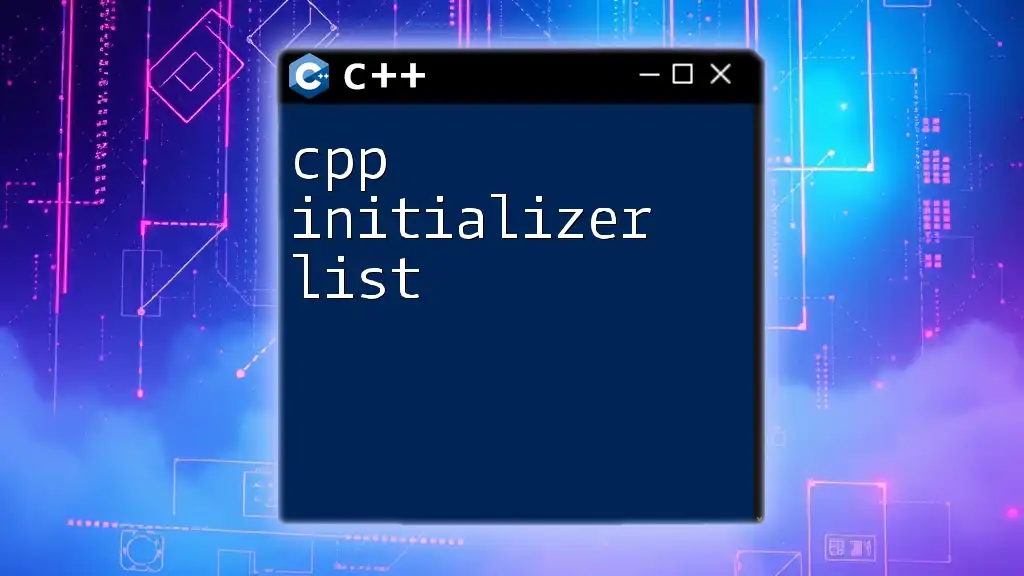
Conclusion
Throughout this guide, we've explored the essential aspects of using C++ for managing WiFi connections, starting from scanning available networks to connecting, managing settings, and handling errors effectively. By familiarizing yourself with these commands and libraries, you can develop robust applications that utilize WiFi to its fullest potential.
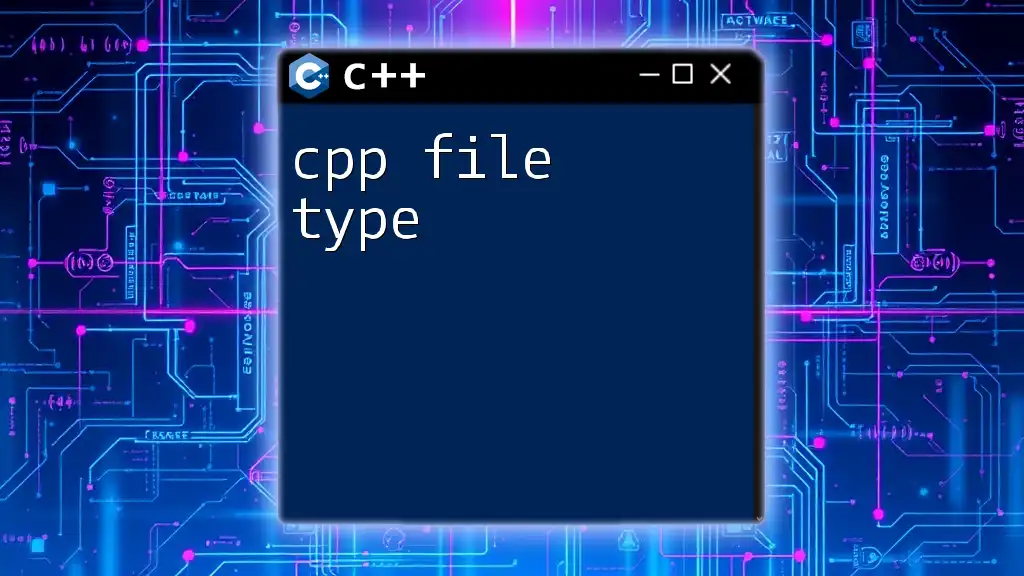
Additional Resources
For those interested in continuing their education in C++ and network programming, consider the following resources:
- Online platforms like Coursera, Udacity, or Pluralsight offer relevant courses.
- Books such as "C++ Network Programming" can provide deeper insights.
- Always refer to the official documentation of libraries like Boost and Qt for comprehensive guidance.