The `windows.h` header file in C++ provides the necessary declarations for Windows API functions, allowing programmers to create and manage windows, handle messages, and utilize various system features.
Here's an example code snippet demonstrating how to create a simple Windows application using `windows.h`:
#include <windows.h>
LRESULT CALLBACK WindowProc(HWND hwnd, UINT uMsg, WPARAM wParam, LPARAM lParam) {
switch (uMsg) {
case WM_DESTROY:
PostQuitMessage(0);
return 0;
case WM_PAINT:
{
PAINTSTRUCT ps;
HDC hdc = BeginPaint(hwnd, &ps);
FillRect(hdc, &ps.rcPaint, (HBRUSH)(COLOR_WINDOW + 1));
EndPaint(hwnd, &ps);
}
return 0;
}
return DefWindowProc(hwnd, uMsg, wParam, lParam);
}
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE, LPSTR, int nShowCmd) {
const char CLASS_NAME[] = "Sample Window Class";
WNDCLASS wc = {};
wc.lpfnWndProc = WindowProc;
wc.hInstance = hInstance;
wc.lpszClassName = CLASS_NAME;
RegisterClass(&wc);
HWND hwnd = CreateWindowEx(0, CLASS_NAME, "Hello Windows", WS_OVERLAPPEDWINDOW,
CW_USEDEFAULT, CW_USEDEFAULT, CW_USEDEFAULT, CW_USEDEFAULT,
NULL, NULL, hInstance, NULL);
ShowWindow(hwnd, nShowCmd);
MSG msg;
while (GetMessage(&msg, NULL, 0, 0)) {
TranslateMessage(&msg);
DispatchMessage(&msg);
}
return 0;
}
Understanding `windows.h`
What is `windows.h`?
The `windows.h` header file is a fundamental component of the Windows API, which provides a rich set of functions, data types, and constants that allow developers to create applications that can interact with the Windows operating system at a high level. This header is essential for any application that requires functionalities tied to Windows, such as GUI creation, file handling, or system-level programming.
Why Use `windows.h`?
Using `windows.h` allows you to harness the full power of Windows OS functionalities. This means you can:
- Create and manage windows for your applications.
- Handle user input from keyboard and mouse actions.
- Create multi-threaded applications, allowing for more efficient processing.
- Access system resources such as files, memory, and other operating system components.
Common use cases include GUI applications, background services, and games that need to interact with the operating system.
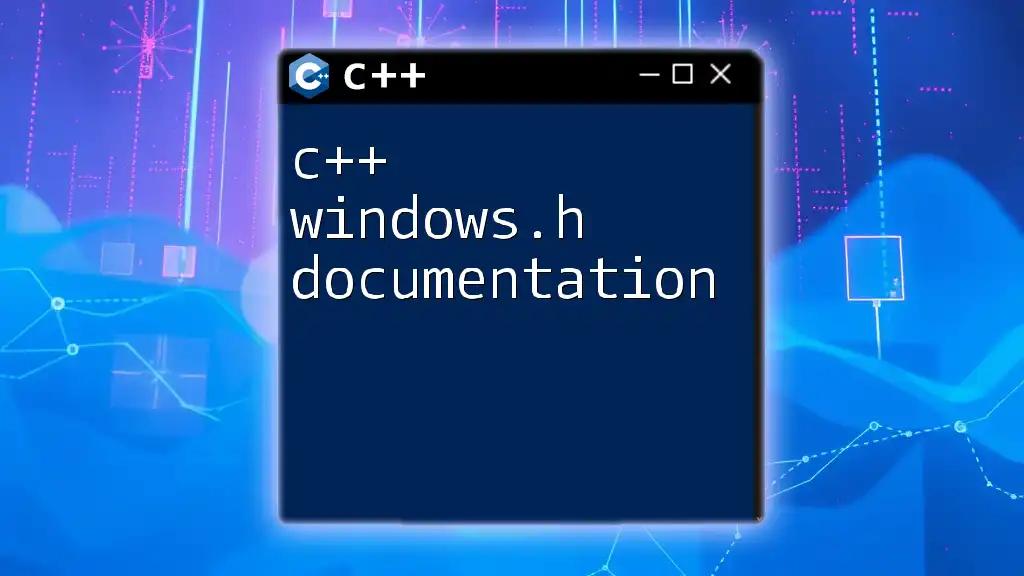
Setting Up Your Development Environment
Choosing the Right Compiler
When working with `cpp windows.h`, it's vital to choose the right development environment. Popular options include:
- Visual Studio: A feature-rich IDE with comprehensive debugging tools. It's widely accepted in the industry for Windows development.
- MinGW: A minimalist option that provides a GCC-based environment for Windows programming. This is suitable for those who prefer lightweight setups.
Configuring the Project
Once a compiler is chosen, you can create a new C++ project. Ensure that you include the `windows.h` header at the top of your source file to utilize its functions and features:
#include <windows.h>
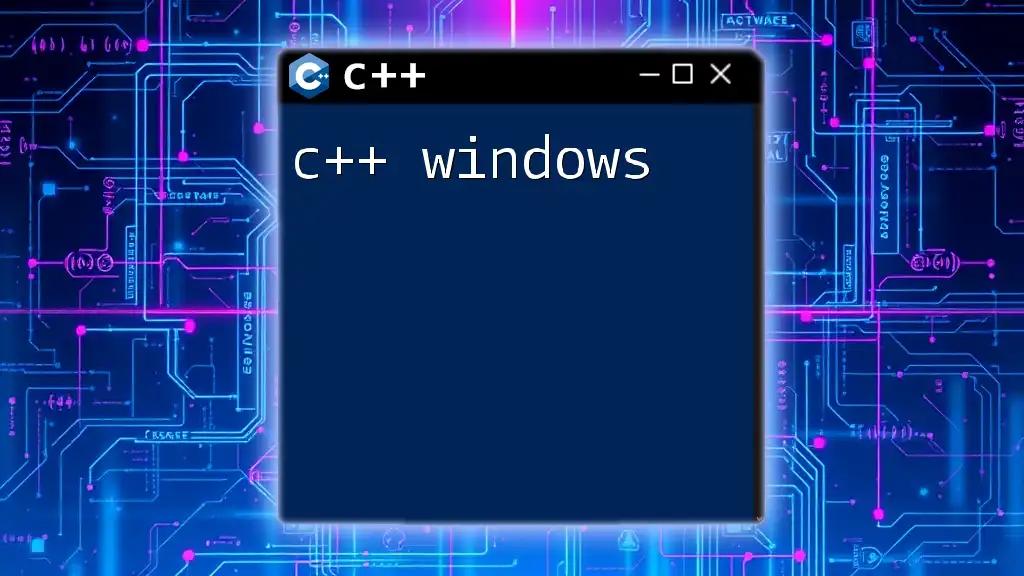
Core Components of `windows.h`
Data Types and Constants
Common Data Types
- HANDLE: This opaque data type represents a reference to an object. It is crucial for various Windows API functions.
- DWORD: This is an unsigned 32-bit integer, typically used to represent various bits of information, such as error codes or process status.
- LPCTSTR: A pointer to a constant string. This data type is used across many API functions to handle string inputs.
Constants and Macros in `windows.h`
Constants such as WIN32_API, NULL, TRUE, and FALSE are often utilized throughout your code, serving as standard values and enhancing readability.
Structures Defined in `windows.h`
Overview of Key Structures
- MSG: A structure that holds message information. Essential for handling events in the message loop.
- WNDCLASS: This structure defines the window class properties. It allows you to specify how your windows will behave and appear.

Functions Provided by `windows.h`
Creating Windows
The Message Loop
Understanding the message loop is critical for any GUI application because it processes all incoming messages from the operating system. The message loop typically looks like this:
MSG msg;
while (GetMessage(&msg, NULL, 0, 0)) {
TranslateMessage(&msg);
DispatchMessage(&msg);
}
In this loop:
- `GetMessage` retrieves messages from the queue.
- `TranslateMessage` translates keyboard messages into character messages.
- `DispatchMessage` sends the message to the window procedure.
Handling Windows Messages
Understanding Windows Messages
Windows messages are notifications sent to applications regarding events, such as user input or system requests. Each message has a unique identifier and carries information about the event.
Example of Handling Messages
To process these messages, you define a window procedure that handles different types of messages. Here’s an example:
LRESULT CALLBACK WindowProc(HWND hwnd, UINT uMsg, WPARAM wParam, LPARAM lParam) {
switch (uMsg) {
case WM_DESTROY:
PostQuitMessage(0);
return 0;
case WM_PAINT:
// Handle window painting
break;
// Additional cases for other messages
}
return DefWindowProc(hwnd, uMsg, wParam, lParam);
}
This function will manage various messages such as window destruction and painting, enabling you to create responsive applications.
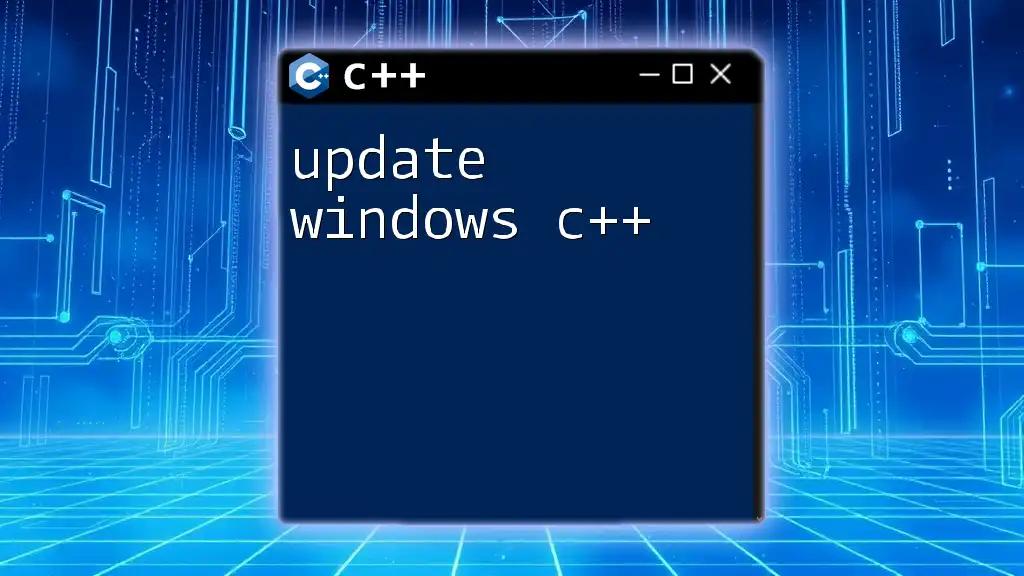
Working with the Windows API
Creating and Managing Windows
Window Creation
Creating a window is one of the first steps in building a GUI application. The `CreateWindowEx` function is used for this purpose. Here's its basic structure followed by an example:
HWND hwnd = CreateWindowEx(
0,
CLASS_NAME,
"Sample Window",
WS_OVERLAPPEDWINDOW,
CW_USEDEFAULT, CW_USEDEFAULT, CW_USEDEFAULT, CW_USEDEFAULT,
NULL, NULL, hInstance, NULL
);
In this code:
- `CLASS_NAME` is the name of the window class.
- The window style and dimensions are specified, allowing you to customize the appearance and behavior of your window.
Interacting with Windows
Basic User Input
To read user input, you can use `GetMessage()`, which retrieves messages from the application’s message queue. Below is a simple input handling demonstration:
while(GetMessage(&msg, NULL, 0, 0)) {
TranslateMessage(&msg);
DispatchMessage(&msg);
}
This snippet continuously checks for user activity and processes the messages accordingly.

Advanced Topics
Multi-threading with `windows.h`
Understanding Threads
Threads allow applications to perform multiple tasks simultaneously. Using the Windows API, you can create threads to improve the performance of your application.
To create a thread, use the `CreateThread` function, as shown in this example:
DWORD WINAPI MyThreadFunction(LPVOID lpParam) {
// Thread code here
return 0;
}
This function will run in a separate thread, allowing your program to execute concurrent operations.
Working with System Resources
Memory Management
Efficient memory management is vital for any application. Windows API provides functions like `HeapAlloc` and `GlobalAlloc` for memory allocation. Using them, you can allocate and free memory dynamically:
HANDLE hHeap = GetProcessHeap();
LPVOID lpvMemory = HeapAlloc(hHeap, HEAP_ZERO_MEMORY, size);
This snippet allocates memory from the process heap, ready for your application’s use.
File Operations
To interact with files, you can use functions such as `CreateFile`, `ReadFile`, and `WriteFile`. Here’s a basic code example for opening a file and reading its content:
HANDLE hFile = CreateFile("example.txt", GENERIC_READ, 0, NULL, OPEN_EXISTING, FILE_ATTRIBUTE_NORMAL, NULL);
if (hFile != INVALID_HANDLE_VALUE) {
DWORD bytesRead;
char buffer[100];
ReadFile(hFile, buffer, sizeof(buffer), &bytesRead, NULL);
CloseHandle(hFile);
}
This example demonstrates how to open a file and read its content into a buffer while managing errors appropriately.
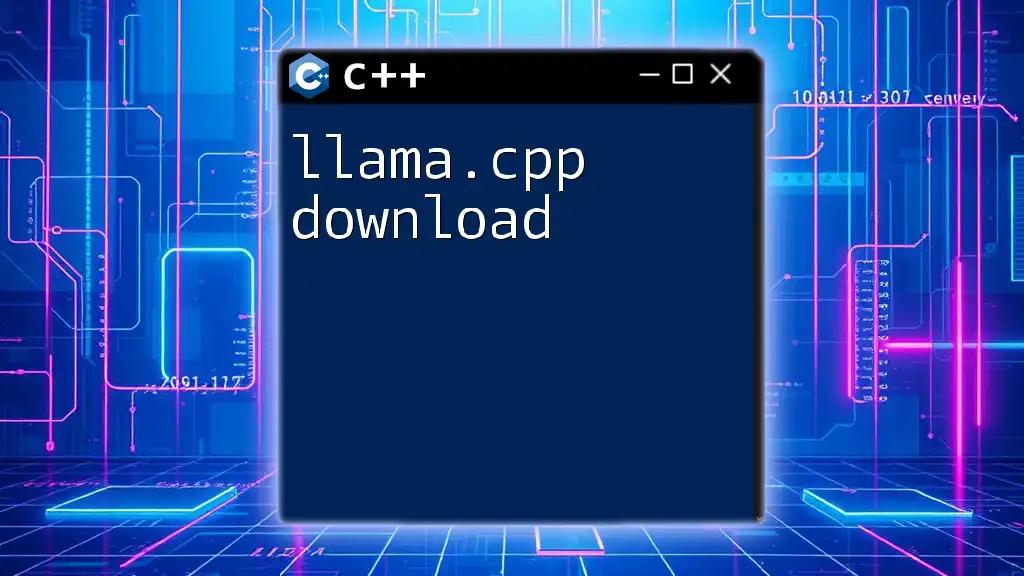
Conclusion
Summary of Key Points
In this guide, we've explored the breadth of functionalities offered by `windows.h`. You learned about core data types, constants, structures, and essential functions for creating and managing windows and input handling. Moreover, we delved into advanced topics like multi-threading and file operations, providing a solid foundation for developing Windows applications using C++.
Further Reading and Resources
To expand your knowledge further, consider referring to:
- Books on Windows API Programming
- Online tutorials and documentation such as Microsoft’s official documentation on the Windows API.
Encouragement to Practise
Practice makes perfect! The best way to become proficient in using `windows.h` is through hands-on experience. Start building small applications, incrementally adding more complexity as you feel comfortable with the API.

FAQs
Common Questions About `windows.h`
What is the purpose of `windows.h`?
`windows.h` serves as the gateway for developers to access the numerous functionalities of the Windows API, enabling them to create robust applications that interface with the operating system.
How do I handle errors in Windows API?
Error handling can be done through the use of `GetLastError()` to retrieve error codes, allowing you to implement error handling routines in your code.
Can I use `windows.h` in cross-platform applications?
`windows.h` is specific to Windows, so if you're looking to develop cross-platform applications, consider using libraries like Qt or wxWidgets that abstract away the platform-specific details.