To update a Windows C++ application, you can use the `Windows API` to check for and install available updates, ensuring that your software runs efficiently and securely.
Here’s a simple code snippet to check for updates:
#include <windows.h>
#include <iostream>
void checkForUpdates() {
// Simulate update checking process
std::cout << "Checking for updates..." << std::endl;
// Here you would implement the logic to check for updates
// e.g., making a network call to your update server.
std::cout << "No updates available." << std::endl;
}
int main() {
checkForUpdates();
return 0;
}
Why You Need to Update Windows C++
Understanding the importance of keeping your Windows C++ updated is crucial for maintaining a smooth and efficient development experience. Updates bring a series of performance improvements, security patches, and compatibility enhancements necessary for leveraging the full capabilities of modern C++.
The Role of Updates in C++
Performance improvements are not just a side benefit; they directly affect how efficiently your applications run. Each update can bring optimizations that enhance compilation speeds and execution times, ultimately leading to better user experiences.
Security patches are another vital reason for updates. The development community continuously discovers vulnerabilities, and timely updates help safeguard against these threats. An outdated C++ environment can expose you to security risks, allowing potential attacks to exploit known flaws.
Finally, as new libraries and tools are developed, keeping your C++ environment updated ensures that you can integrate these resources seamlessly. Without them, you might miss out on important features and advancements that can significantly enhance your projects.
Common Issues Caused by Outdated C++ Versions
Operating with an outdated C++ version can lead to numerous challenges, including:
-
Bugs and performance degradation: Over time, older versions can exhibit performance issues not seen in newer iterations due to advancements in technology.
-
Security vulnerabilities: As mentioned, old versions often lack the latest patches that protect your applications from exploits.
-
Difficulty integrating with modern C++ standards: New C++ features may not be supported in older versions, which can hinder your ability to implement modern coding practices.
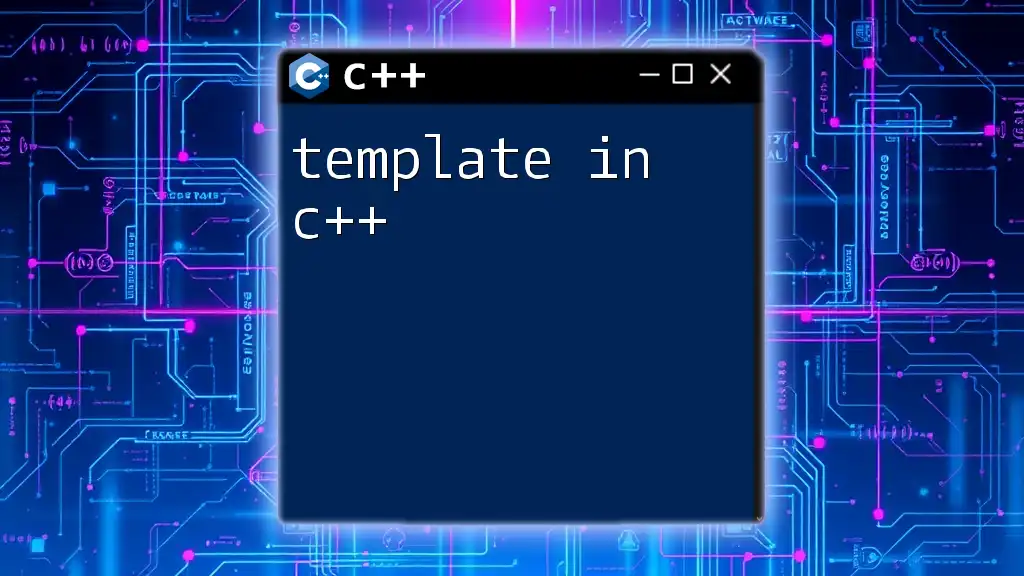
Understanding Visual C++
Before diving into the update process, it is essential to understand what Microsoft Visual C++ is and why it is integral to Windows development.
What is Microsoft Visual C++?
Microsoft Visual C++ (often referred to as Visual C++) is a powerful tool used for developing applications designed to run on Windows. Its primary features include an extensive IDE, a robust compiler, and a rich library of pre-built functions that simplify the coding process.
Components of Visual C++
Visual C++ Compiler: This component is responsible for translating your code into executable programs. With each update, you'll often find enhancements to the compiler that yield better optimization, more robust error handling, and support for newer C++ standards.
Visual C++ Libraries: Libraries contain pre-written code that developers can utilize to perform common tasks without having to write from scratch. Familiarizing yourself with these libraries and their updates can save valuable time and effort in your projects.
Visual C++ IDE (Integrated Development Environment): The IDE is your primary working environment, offering numerous features that facilitate easier coding, debugging, and project management. Regular updates to the IDE can provide new tools and enhance existing functionalities, making your development experience smoother.
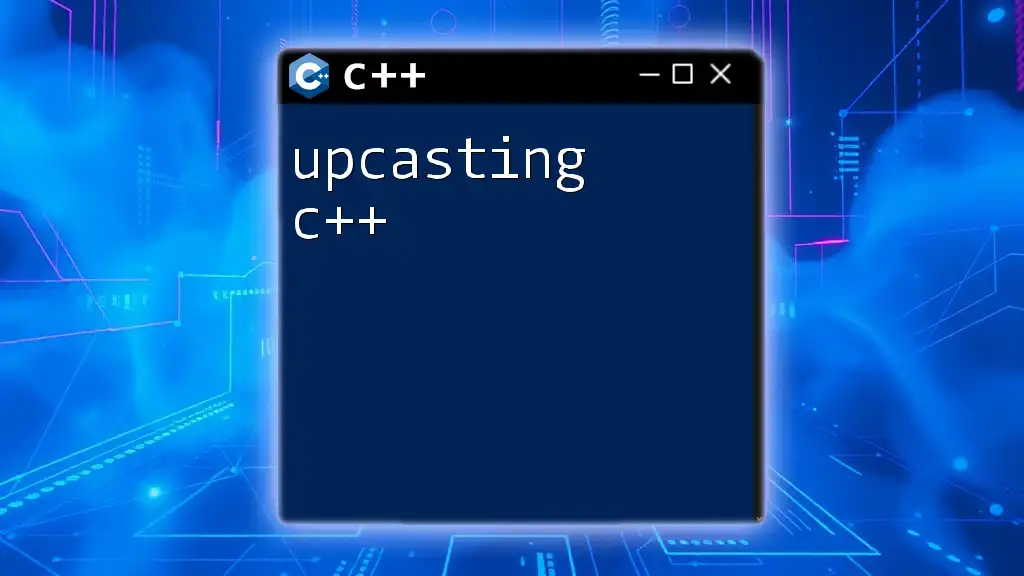
How to Upgrade Visual C++
Upgrading Visual C++ can seem daunting, but following a structured approach ensures a seamless transition.
Preparing for the Upgrade
Before upgrading, check your current Visual C++ version by navigating to the Help menu in your IDE and selecting "About Microsoft Visual Studio." Ensuring you know your version helps confirm that you are upgrading correctly.
Backing up your projects is crucial. Making a backup allows you to revert to the previous state if anything goes wrong during or after the update.
It's also important to read release notes carefully. Each update comes with its unique features and potential breaking changes that could affect your ongoing projects.
Step-by-Step Guide to Upgrade Visual C++
Using Visual Studio Installer
- Open the Visual Studio Installer from your Start menu.
- Locate the version of Visual Studio currently installed and click on the "Modify" button.
- In the installation screen, select the appropriate version and components you want to install.
- Finalize the installation settings and click "Update."
For example, to run the installer from the command line, you may use:
vs_installer.exe update --version 16.11
Manual Installation of Microsoft Visual C++ Redistributable
Sometimes, you might need to manually install the Visual C++ Redistributable. Navigate to Microsoft's official website, find the latest version, and download it. Keep in mind that redistributables are essential for running applications built with Visual C++, as they contain necessary libraries.
After downloading, install it using this command:
msiexec /i vcredist_x64.exe
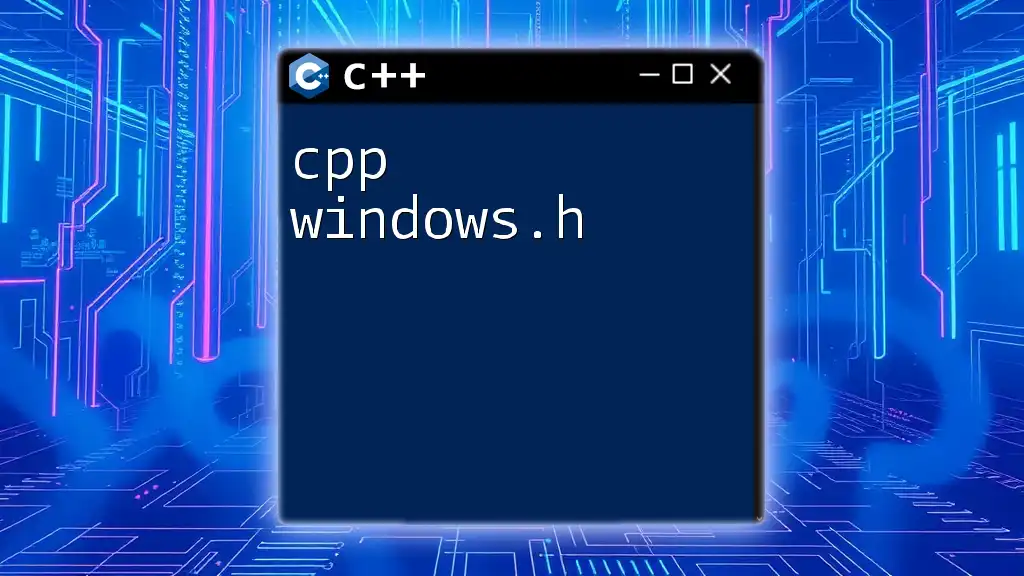
How to Perform a C++ Update
Once you’ve upgraded Visual C++, ensure your projects are also updated appropriately.
Steps for Updating C++ Projects
After the upgrade:
-
Open each project in Visual Studio and review the project properties. Make adjustments to reflect the updated version. This often involves changing the platform toolset to the latest version.
-
Update your dependencies for any libraries you utilize. This may involve downloading updates from their respective repositories or reinstalling them via NuGet.
For example, in your project settings, you might need to change compiler settings as follows:
#include <iostream>
// Ensure you're using the latest features available in your updated Visual C++
using namespace std;
int main() {
cout << "Hello, updated Visual C++ World!" << endl;
return 0;
}
Once you've updated your project settings, compile and test the project. This is critical to ensure everything works correctly with the new version.
Common Problems and Their Solutions
After an update, you might encounter some issues:
Compilation errors can arise. If so, check the error logs to diagnose problems accurately. Often, adjusting project configurations or modifying code according to updated standards will resolve these issues.
Compatibility issues with third-party libraries can also occur. Always check for updates from these vendors to ensure smooth operation. The code might require changes to accommodate new versions of libraries you are using.
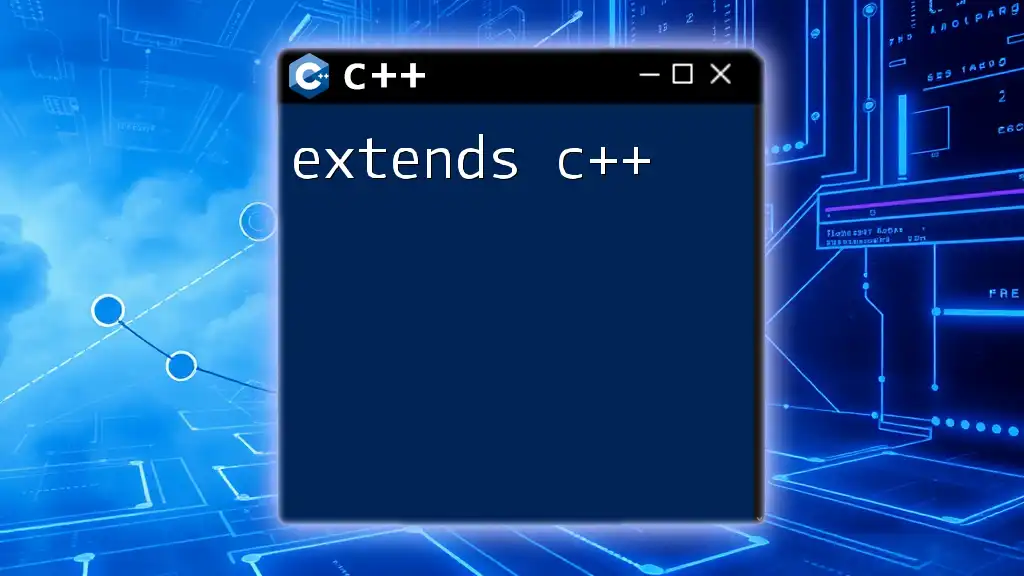
Best Practices for Maintaining Updated C++ Environments
Regularly Check for Updates
To maintain an efficient and secure development environment, prioritize regularly checking for updates. This can be automated through specific tools or reminders so you won’t miss out on essential updates.
Testing and Verification Post-Update
Thorough testing is crucial after any upgrade. Implement comprehensive testing methods for your applications. This includes unit tests, integration tests, and performance assessments to confirm that all functionalities are working as intended following the update.
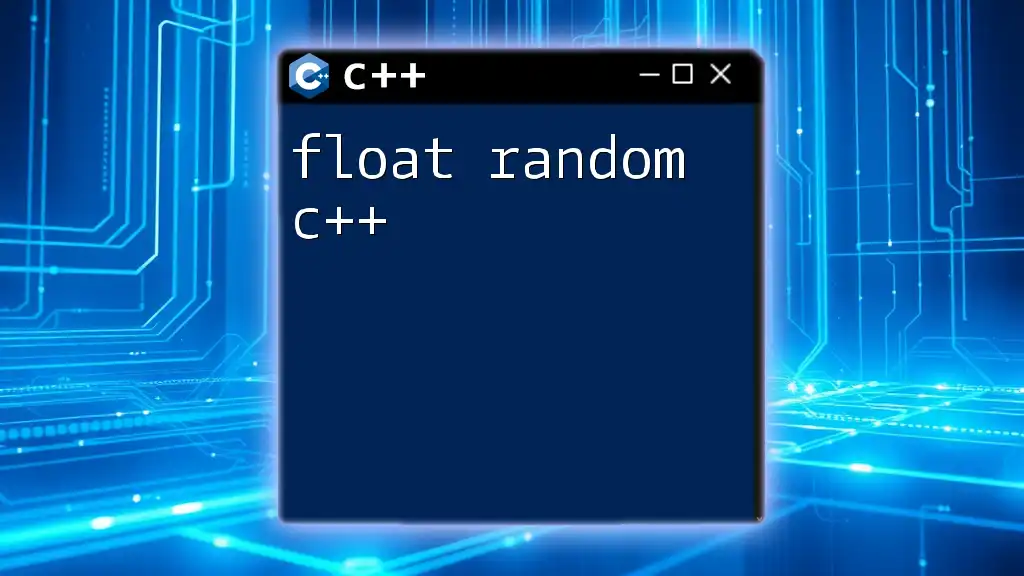
Conclusion
In summation, keeping your Windows C++ updated is essential for optimal performance, coding security, and enjoying modern coding standards. By following the steps outlined above, you can ensure your development environment remains robust and flexible enough to meet the demands of contemporary software development. Embrace the updates and start making your applications more competitive today!