To set up a Windows development environment for C++, you need to install a compiler like MinGW or Visual Studio, set up your PATH variable, and create a simple "Hello World" program to test the setup.
Here's a basic example of a C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ Development Environment
What is a Development Environment?
A development environment is a collection of tools, libraries, and settings that programmers use to write, test, and debug code efficiently. It includes Integrated Development Environments (IDEs), compilers, debuggers, and essential libraries that make coding smoother and more productive.
Components of a Development Environment
- IDE: An environment where developers can write code, navigate, and execute programs.
- Compiler: Translates human-readable code into machine language that the computer understands.
- Debugger: Helps find issues in code and allows stepping through code line-by-line.
- Libraries: Pre-written code that can be reused, saving time and effort.
Why Choose Windows for C++ Development?
Choosing Windows for C++ development comes with distinct advantages such as:
- Software Compatibility: Many applications are designed primarily for Windows, making it the go-to environment for various development projects.
- Ease of Use: The Windows interface is familiar to many users, lowering the learning curve for new developers.
- Community Support: A robust community means access to an array of resources, tutorials, and troubleshooting help.
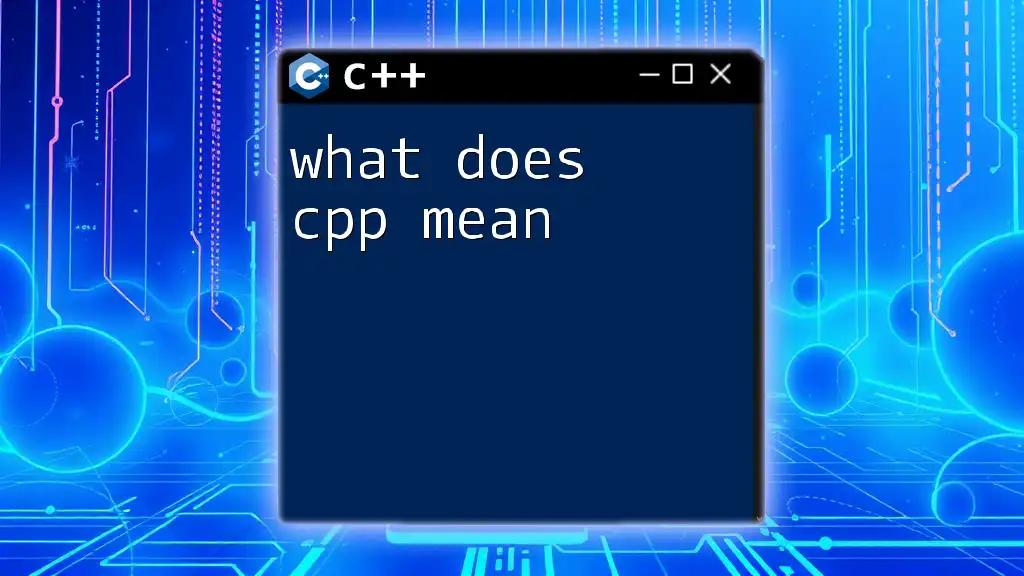
Prerequisites
Minimum System Requirements
Before setting up your environment, ensure your system meets the:
- Operating System: Windows 10 or later preferred for optimal support.
- Processor: Dual-core or higher recommended for efficient compilation.
- RAM: Minimum 4GB, but 8GB is ideal.
- Disk Space: At least 5GB free for installation and additional libraries.
Basic Knowledge Requirements
A fundamental understanding of programming concepts is necessary to make the most of your development environment. Familiarity with command-line interfaces will also aid in navigating tasks efficiently.
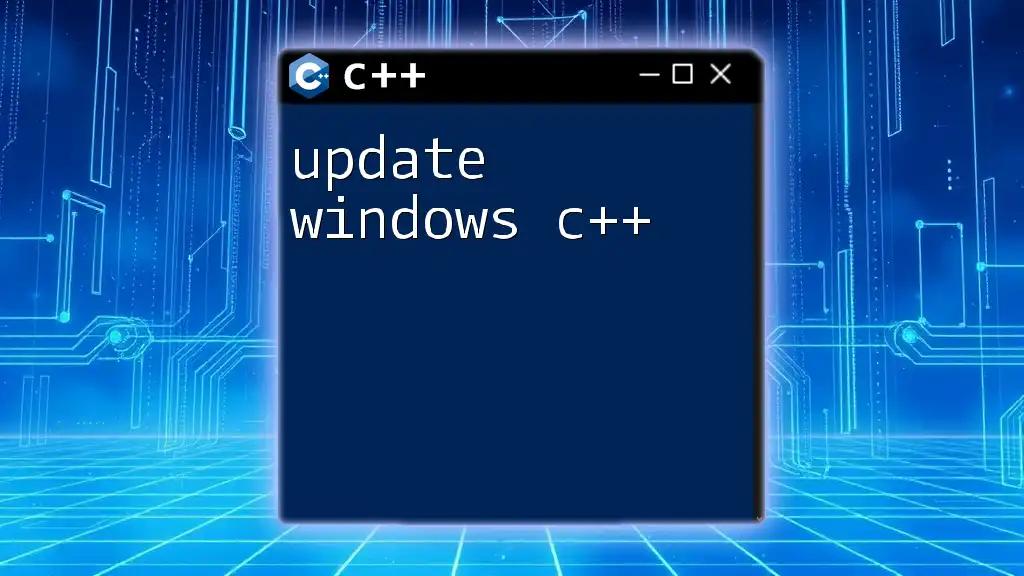
Choosing the Right Tools
Recommended IDEs for C++
Selecting the right IDE is crucial for enhancing your programming experience. Here are some popular options:
-
Microsoft Visual Studio: A powerful IDE with extensive features. It provides robust debugging tools and integrated Git support, essential for modern development.
- Installation Guide: Download from the official site, run the installer, and choose the Desktop development with C++ workload.
-
Code::Blocks: A lightweight, open-source IDE, perfect for beginners. Code::Blocks supports multiple compilers, allowing flexibility.
- Installation Process: Download the installer and follow guided steps to set up the IDE, ensuring the built-in MinGW compiler option is selected.
-
CLion: A premium IDE tailored for professional developers. It offers a rich feature set, including smart code completion and on-the-fly analysis.
- Installation Steps: Obtain CLion from JetBrains, download the installer, and follow the prompts to set it up.
Essential Compilers
The choice of compiler directly impacts your development experience:
-
MSVC (Microsoft C++ Compiler): Included with Visual Studio, offering access to the latest C++ standards and optimized performance. Install with your Visual Studio setup.
-
MinGW (Minimalist GNU for Windows): A popular choice for cross-platform development. It provides essential Unix-like tools and is easy to set up.
- Setup Guide: Download and run the installer. Ensure to add MinGW/bin to your system PATH for command-line usage.
Debugging Tools
Debugging is an integral part of C++ development that helps identify and fix errors swiftly. Two popular debugging tools are:
- GDB (GNU Debugger): A powerful command-line debugger for MinGW that allows inspecting variables and controlling program execution.
- Visual Studio Debugger: Integrated within Visual Studio, offering breakpoints, watch windows, and a visual representation of call stacks.
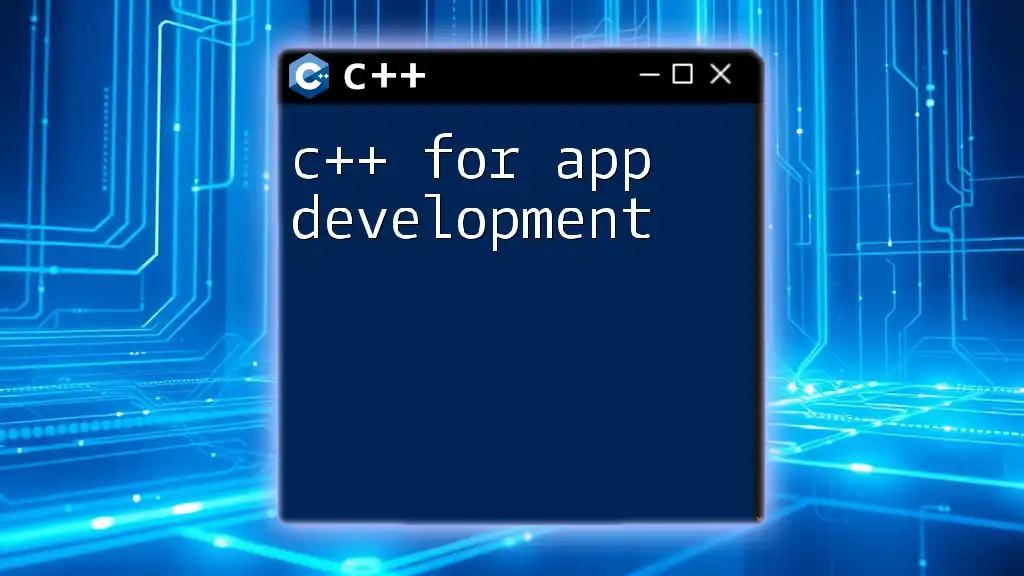
Installing the Development Environment
Step-by-Step Installation Guide
Installing Microsoft Visual Studio
- Navigate to the [Visual Studio website](https://visualstudio.microsoft.com/).
- Download the installer and run it.
- On the installer screen, select Desktop development with C++ to install necessary components including the MSVC compiler.
- Follow installation prompts, complete the setup, and open Visual Studio.
Installing Code::Blocks
- Visit the [Code::Blocks website](http://www.codeblocks.org/).
- Download the binary release that includes MinGW.
- Launch the installer and follow the prompts.
- Once installed, create a new C++ project to ensure everything functions correctly.
Installing MinGW Compiler
- Download the MinGW installation manager from the [MinGW website](http://www.mingw.org/).
- Follow the prompts in the installer, ensuring to include mingw32-base and mingw32-gcc-g++ packages.
- After installation, configure your environment variables:
- Right-click on This PC > Properties > Advanced system settings > Environment Variables.
- Under System Variables, find Path and append the path to your MinGW bin folder (usually C:\MinGW\bin).
Setting Up Environment Variables
Setting up environment variables is essential for enabling command-line access to your installed tools. The steps include:
- Open the System Properties (Right-click This PC > Properties).
- Select Advanced system settings > Environment Variables.
- Edit the Path variable to include paths for MSVC and MinGW compilers, ensuring they are separated by semicolons.
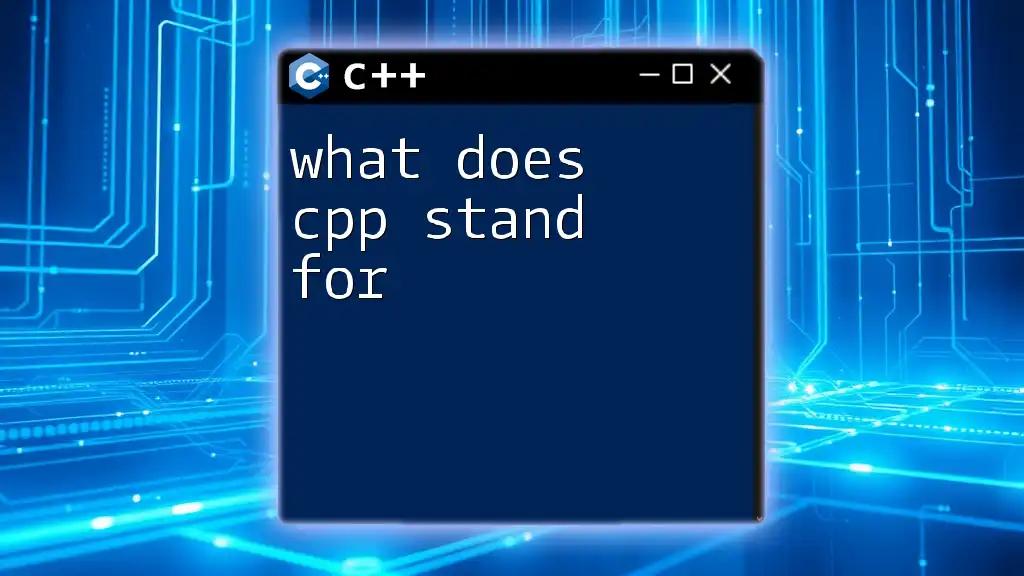
Testing Your Setup
Writing Your First C++ Program
To ensure your development environment is working correctly, write a simple "Hello, World!" program.
#include <iostream> // Include the iostream library
using namespace std;
int main() { // Main function
cout << "Hello, World!" << endl; // Output to console
return 0; // Indicate successful program termination
}
How to Compile and Run:
- In Visual Studio: Create a new C++ project, add your code, and hit CTRL + F5.
- In Code::Blocks: Create a new project, paste the code, then click on the build button and run it.
Common Issues and Troubleshooting
Even with a correctly set up environment, beginners may encounter issues such as:
- Compiler not found errors: Revisit your PATH settings to make sure they include the compiler directories.
- Linker errors: These often indicate missing libraries, so ensure all required libraries are properly linked and set in your project settings.
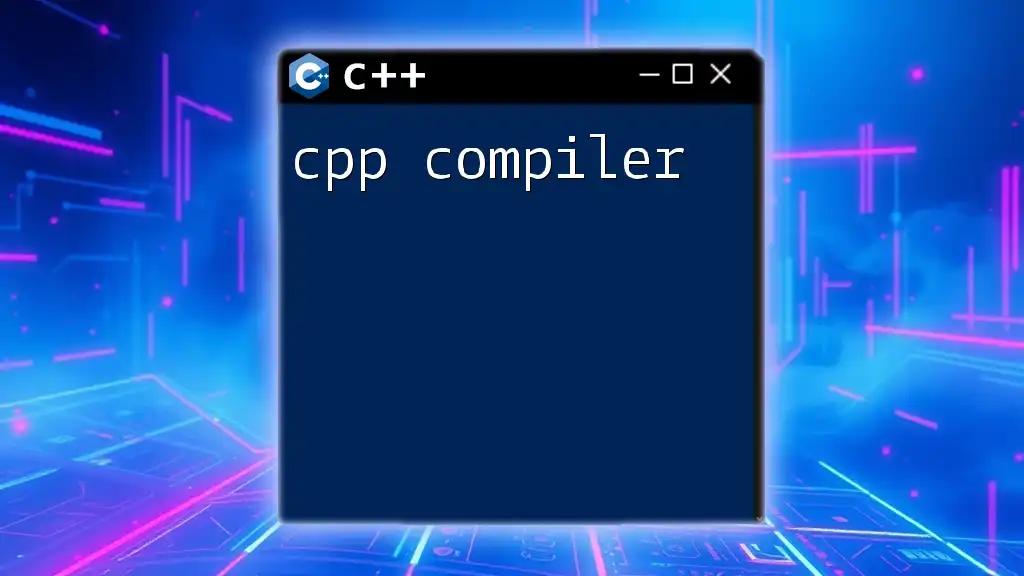
Additional Considerations
Version Control
Incorporating version control into your development process is crucial for managing changes and collaboration. Git is the recommended tool for version control.
- Setup Guide: Install Git from the [official site](https://git-scm.com/). After installation, use Git Bash to initialize a repository for your C++ projects.
C++ Libraries to Consider
Using libraries can speed up development and introduce powerful capabilities:
- Boost: Provides free, peer-reviewed C++ libraries that extend functionality.
- STL (Standard Template Library): A powerful set of C++ template classes that implement generic algorithms and data structures.
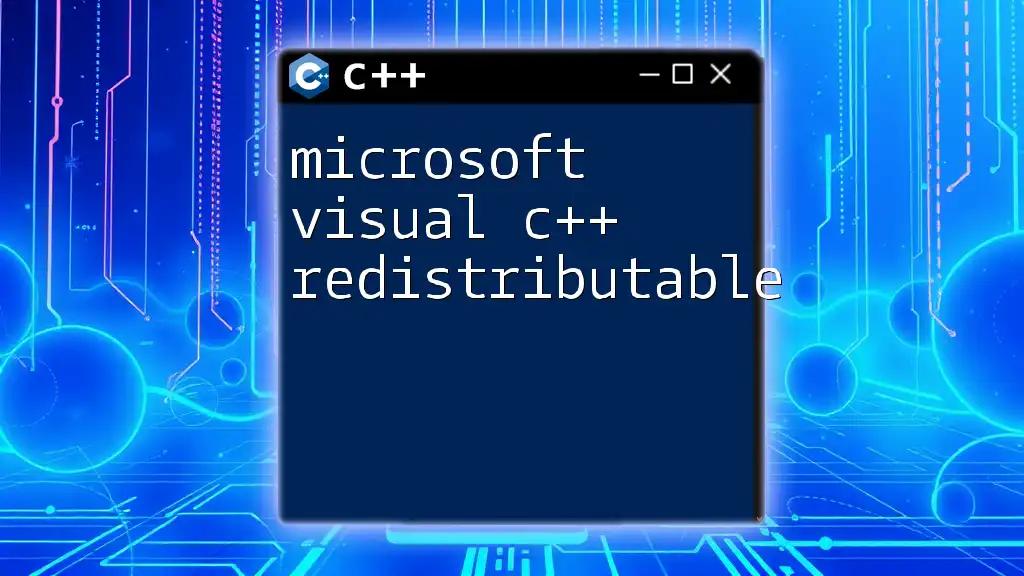
Conclusion
A well-set development environment is the foundation of efficient C++ programming. By following the steps outlined above, you will set yourself up for success, allowing you to focus on what really matters—writing great code. Consider exploring project ideas and additional resources to further enhance your skills.
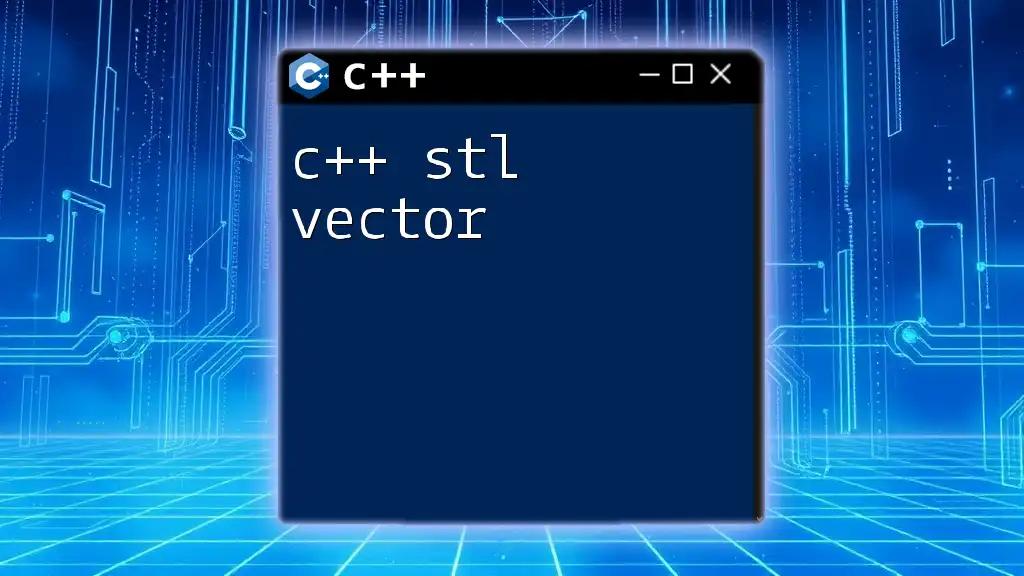
Resources
For further reading, check the documentation for Visual Studio, Code::Blocks, MinGW, Git, and C++ libraries.
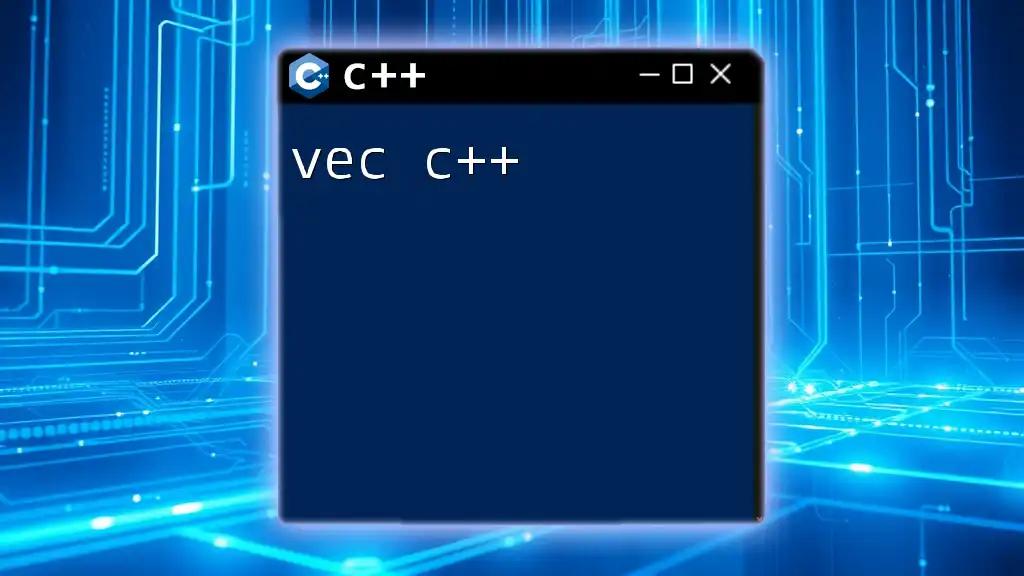
Call to Action
We invite you to share your setup experiences or any challenges you faced while configuring your development environment. Your story could help others in their journey. Don't forget to follow our blog for more quick and concise C++ tutorials!