CPP stands for C Preprocessor, which is a tool that processes C (and C++) source code before compilation, handling directives such as macros and file inclusions. Here’s a simple code snippet to illustrate a basic use of a macro:
#define SQUARE(x) ((x) * (x))
#include <iostream>
int main() {
int number = 5;
std::cout << "The square of " << number << " is " << SQUARE(number) << std::endl;
return 0;
}
What is CPP in Programming?
Definition of CPP
CPP primarily stands for C++, an advanced programming language developed as an extension of the C programming language. Created by Bjarne Stroustrup in the early 1980s, C++ incorporates various features that enhance programming efficiency, notably object-oriented programming.
Core Features of C++
C++ introduces several powerful features that are key to its popularity and versatility:
-
Object-Oriented Programming (OOP): One of the most significant aspects of C++ is its incorporation of OOP principles, including:
- Encapsulation: Bundling the data and the methods that operate on that data within one unit, or class.
- Inheritance: Enabling new classes (derived classes) to inherit properties and behavior (methods) from existing classes (base classes).
- Polymorphism: Allowing entities to take on multiple forms, significantly enhancing flexibility and reusability in programming.
-
Rich Standard Library: The Standard Template Library (STL) offers a robust set of functions and classes that simplify complex programming tasks. It includes:
- Algorithms, such as searching and sorting.
- Containers, such as vectors, lists, and maps, which facilitate data organization and manipulation.
-
Compatibility with C: C++ provides a high degree of compatibility with C, which means that most C programs can be used as C++ programs with minimal modifications. This is particularly beneficial for code reuse and maintaining legacy systems.
Code Snippet
To illustrate a basic C++ program, consider the following example, which displays a simple greeting to the user:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
This code showcases the basic syntax of C++: it includes the necessary input-output library and defines the main function, which is the starting point of any C++ program. The `std::cout` command is used to output text to the console, demonstrating how straightforward C++ can be.
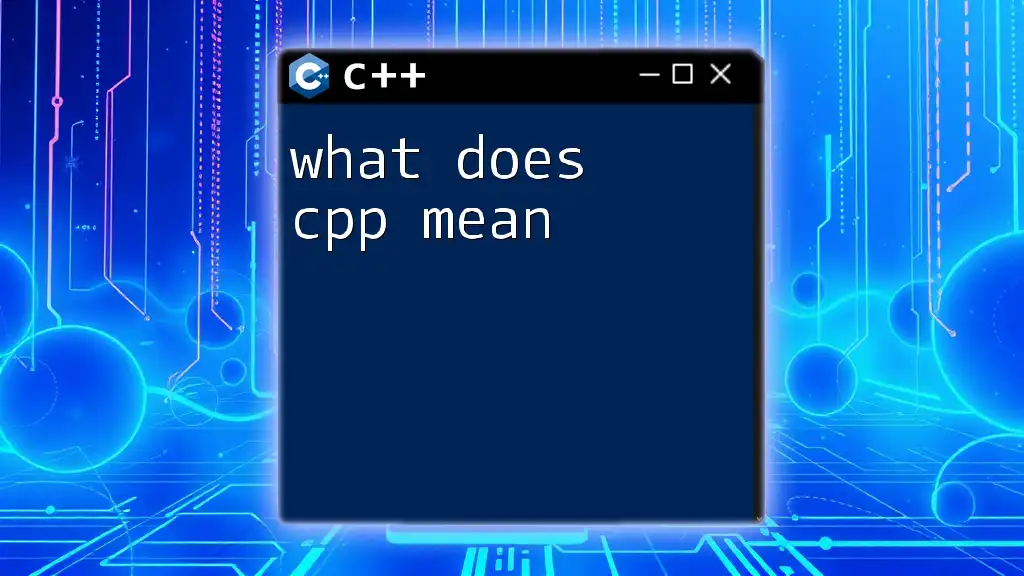
CPP Acronym Meaning in Different Contexts
C++: The Programming Language
As previously discussed, C++ is renowned for its ability to provide high performance and efficiency in executing programs. This is paramount in areas such as game development and software requiring real-time processing, where execution speed is critical.
Other Meanings of CPP
Beyond the realm of programming languages, CPP can denote various concepts:
-
C PreProcessor: The C PreProcessor prepares source code for compilation. It processes directives (commands) before actual compilation begins. Key functionalities include:
- File inclusion: Allowing code to be modular with the `#include` directive.
- Macros: Implementing portable code using pre-defined parameters and conditions.
-
C++ Programming Paradigms: C++ supports multiple programming paradigms, including procedural, object-oriented, and functional programming. This versatility results in a broader range of applications and styles, accommodating different programmer preferences and project requirements.
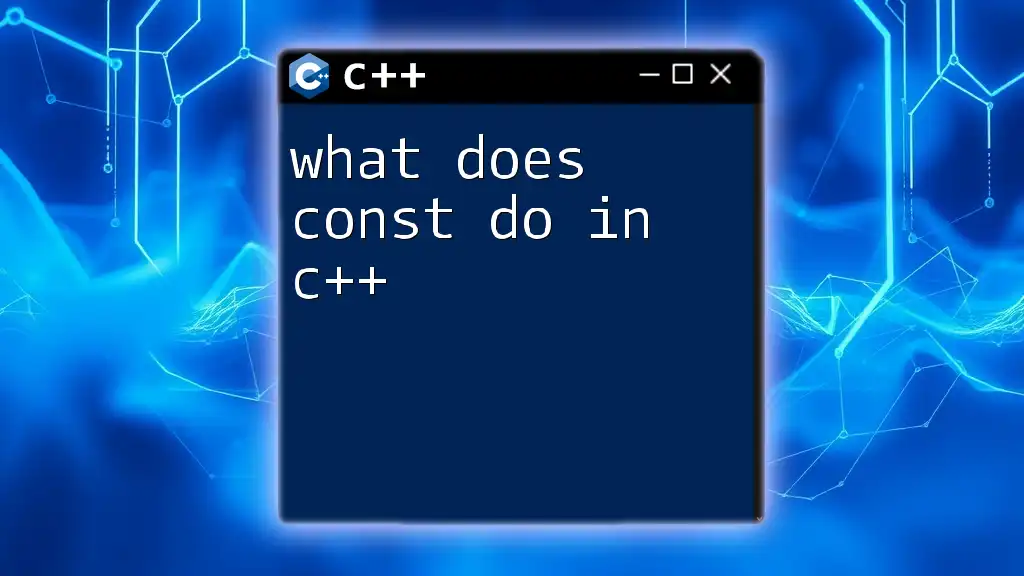
Practical Applications of C++
Industries Utilizing C++
The applications of C++ are extensive and deeply ingrained in several critical sectors:
-
Software Development: C++ is foundational in developing software applications, including high-performance games, where real-time rendering is essential.
-
Financial Sector: C++ shines in high-frequency trading algorithms and quantitative finance, where speed and efficiency directly correlate to profitability. Financial applications benefit from C++'s performance in managing large data sets and complex calculations.
-
Embedded Systems: Industries such as automotive and IoT often utilize C++ for programming embedded systems. Its alignment with hardware capabilities allows developers to create sophisticated applications that interact directly with devices.
C++ Libraries and Frameworks
Numerous libraries bolster the functionality of C++, providing features that expedite development:
-
Boost: Widely regarded as a comprehensive collection of peer-reviewed portable libraries, Boost provides solutions for tasks such as:
- Smart pointers for memory management.
- Multi-threading capabilities for concurrent programming.
-
Qt: A powerful framework for developing GUI applications, Qt simplifies the design of interfaces and boosts productivity. It supports both desktop and mobile application development.
-
OpenCV: Renowned for its application in computer vision, OpenCV is crucial in scenarios ranging from image processing to real-time video analysis. It underscores C++'s role in cutting-edge technology.
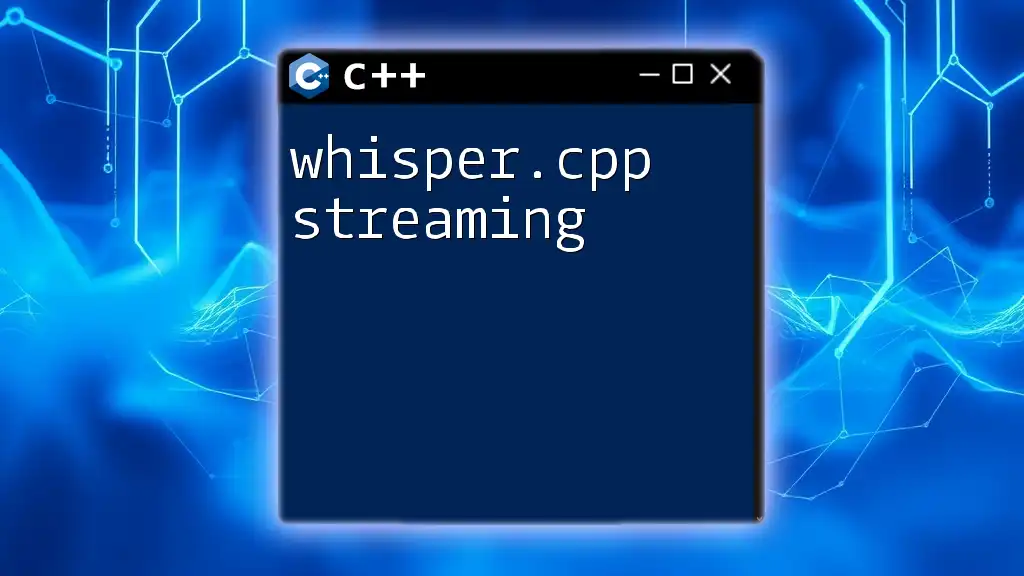
Conclusion
Summary of CPP
In summary, when asking "what does CPP stand for?", the most direct answer is the programming language C++. However, CPP encompasses various meanings within the programming landscape, including the C PreProcessor and broader programming paradigms. Understanding C++ is pivotal for anyone looking to venture into software development, offering capabilities that are essential for creating efficient, versatile programs.
Finding Resources to Learn CPP
For those eager to grasp the intricacies of C++, numerous online courses, coding boot camps, and books are available to help learners navigate this complex yet rewarding language. Moreover, as a company dedicated to teaching CPP commands in a quick, short, and concise manner, we offer tailored resources to enhance your learning experience and proficiency in C++.
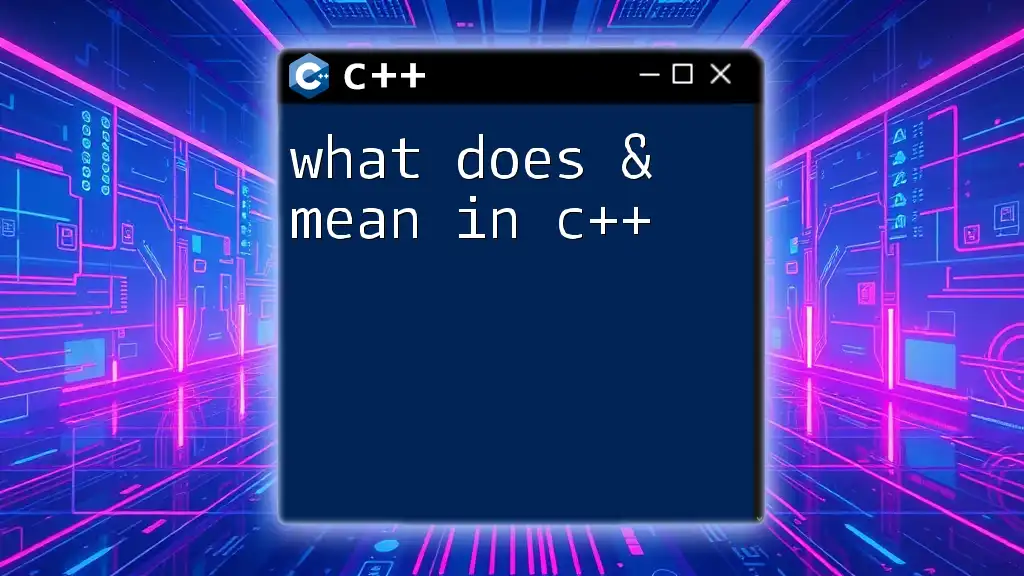
Call to Action
We invite you to subscribe for further articles and tutorials on C++ and related topics. Share your experiences with C++ and what specific aspects you wish to learn more about, as we strive to create content that resonates with the programming community.