In C++, `char` is a built-in data type used to represent a single character, typically occupying 1 byte of memory.
char letter = 'A';
What is a Char in C++?
A char in C++ is a fundamental data type that stores a single character. This could be any letter, digit, or symbol. For example, the character 'A' is represented as `char letter = 'A';`.
Char vs. Other Data Types
Compared to other data types, the char data type stands out in its specificity. Below are some distinctions:
- int: Used for integer numbers, commonly occupies 4 bytes.
- float: Suitable for floating-point numbers, usually takes 4 bytes or more.
- string: A collection of characters, not limited to single character storage.
Understanding char is essential because many advanced areas like strings, character encoding, and even input/output operations in C++ build on this fundamental data type.
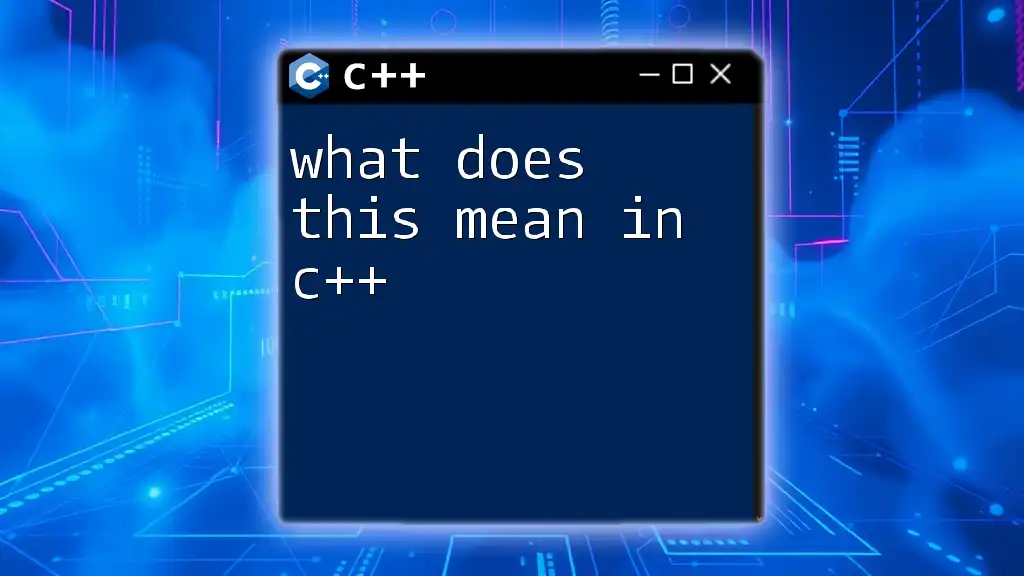
How to Declare a Char in C++
Declaring a char in C++ is straightforward. The syntax is simple and follows the structure:
char letter = 'A';
Examples of Char Declarations
Here are several examples that illustrate how to declare char variables:
char initial = 'Z';
char digit = '3';
char specialChar = '#';
This versatility allows you to store various types of characters using a single data type.
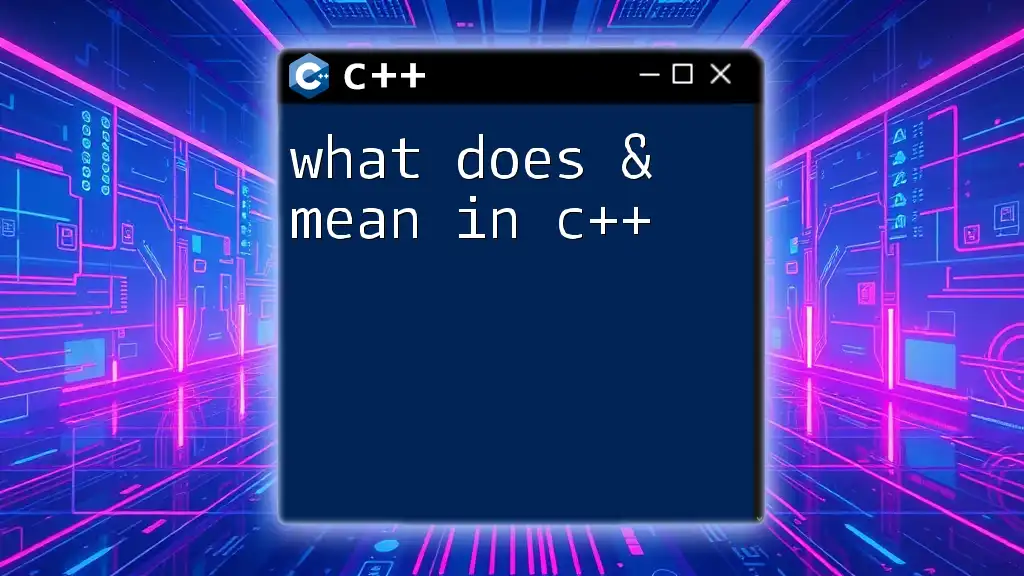
Characteristics of Char in C++
Size and Memory Allocation
A char generally occupies 1 byte of memory. This small size makes it essential for memory management, especially when working with larger datasets.
Char Range
Chars are fundamentally linked to the ASCII character set. In C++, a char can hold values from:
- -128 to 127 (for signed char)
- 0 to 255 (for unsigned char)
This range is crucial for understanding how characters are represented in memory.
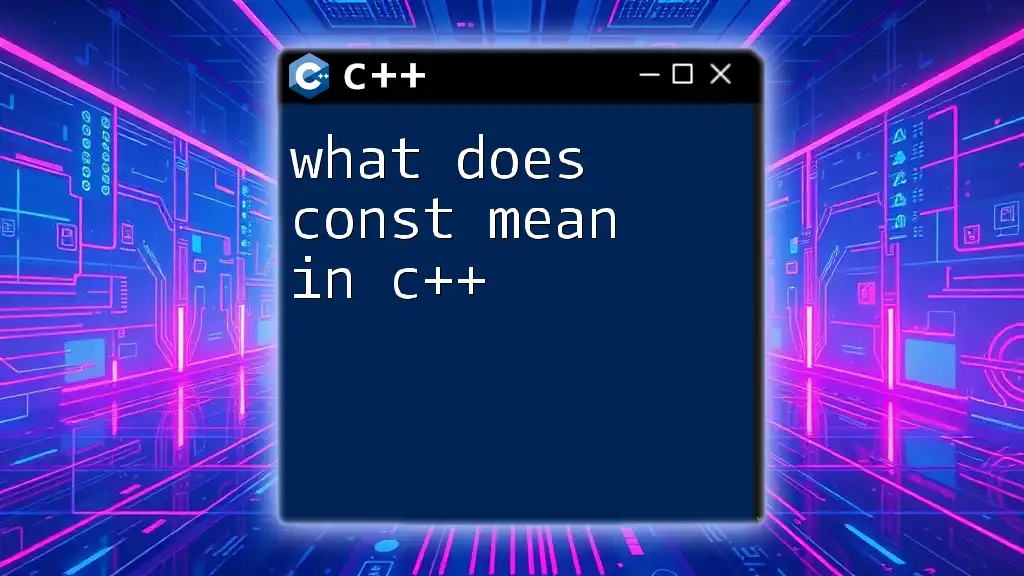
Using Char in C++ Programs
Basic Char Operations
Char variables can be assigned, compared, and printed just like other variables. Below is a quick example of basic operations with char:
char a = 'A';
char b = 'B';
if (a < b) {
cout << "A comes before B";
}
Char and Arrays
Chars can also be used to create character arrays, effectively creating strings. For instance:
char name[10] = "Alice";
cout << name; // Outputs "Alice"
This method of using character arrays is foundational for string manipulation in C++.
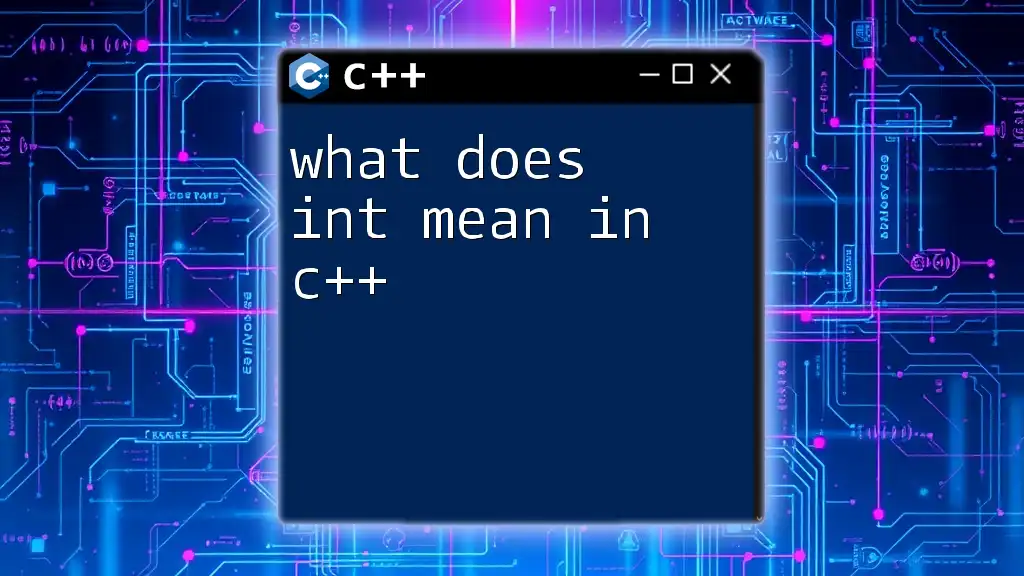
Advanced Char Concepts
Char in Input/Output
In C++, the `cin` and `cout` functions can handle char variables efficiently. Here’s a simple example of taking char input from the user:
char inputChar;
cout << "Enter a character: ";
cin >> inputChar;
This functionality allows for more interactive programs.
Escape Sequences
Escape sequences represent special characters in a string, such as new lines and tabs. For example:
cout << "This is a newline character: \n";
Such sequences enhance how we format output in C++ effectively.
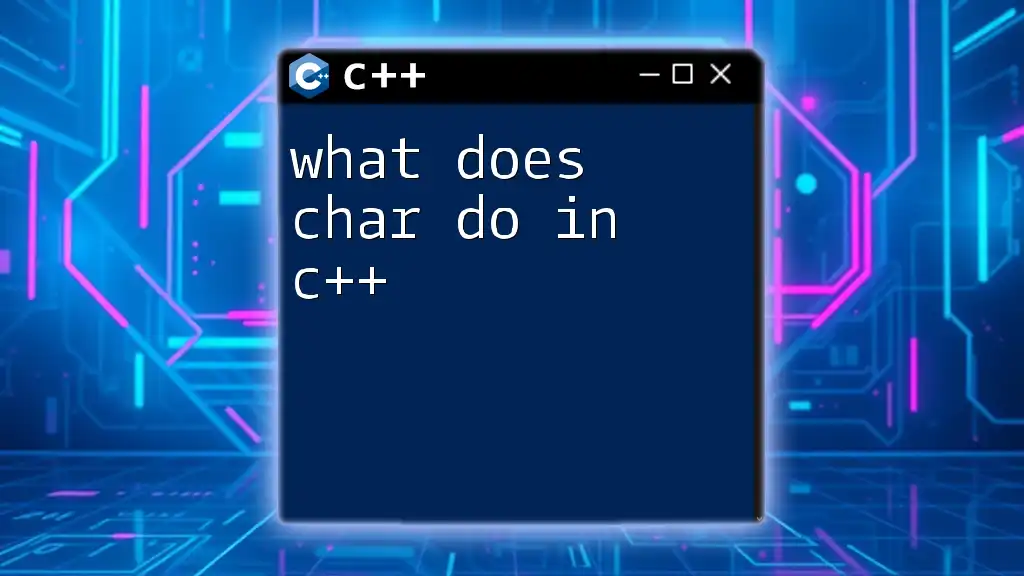
Conversion Between Char and Other Types
Char to Int and Back
An important feature of chars is their ability to represent ASCII values. You can easily convert a char to its integer ASCII value as shown below:
char c = 'A';
int ascii = c; // ascii now holds the value 65
Conversely, converting back from an integer to a char is equally straightforward:
int asciiValue = 66;
char convertedChar = (char)asciiValue; // convertedChar now holds 'B'
String to Char and Vice-Versa
Converting between strings and char arrays is another fundamental concept:
std::string str = "Hello";
const char* charPtr = str.c_str();
In this example, the C++ string is converted to a C-style string (char pointer), which can be useful for certain functions that accept char pointers.
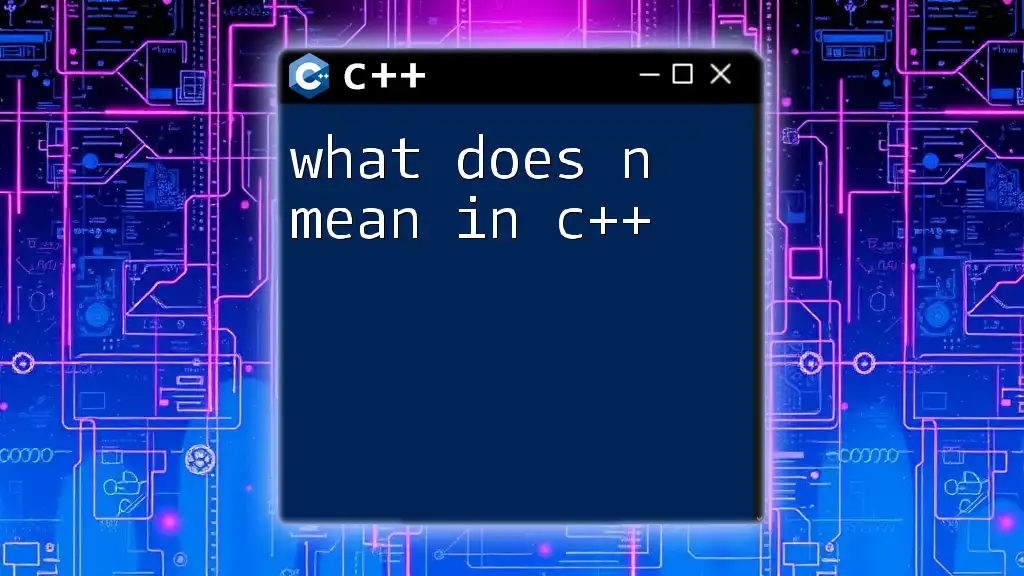
Common Mistakes When Using Char
Misusing Quotes
A common error is confusing single and double quotes. In C++, single quotes (`'`) are used for characters, while double quotes (`"`) are for strings:
char a = 'A'; // Correct: single character
char* b = "A"; // Incorrect: this is treated as a C-style string
Null Terminator
When dealing with character arrays (strings), one must remember the null terminator (`\0`), which is used to signify the end of the string in C++. Forgetting to include the null terminator can lead to undefined behavior.
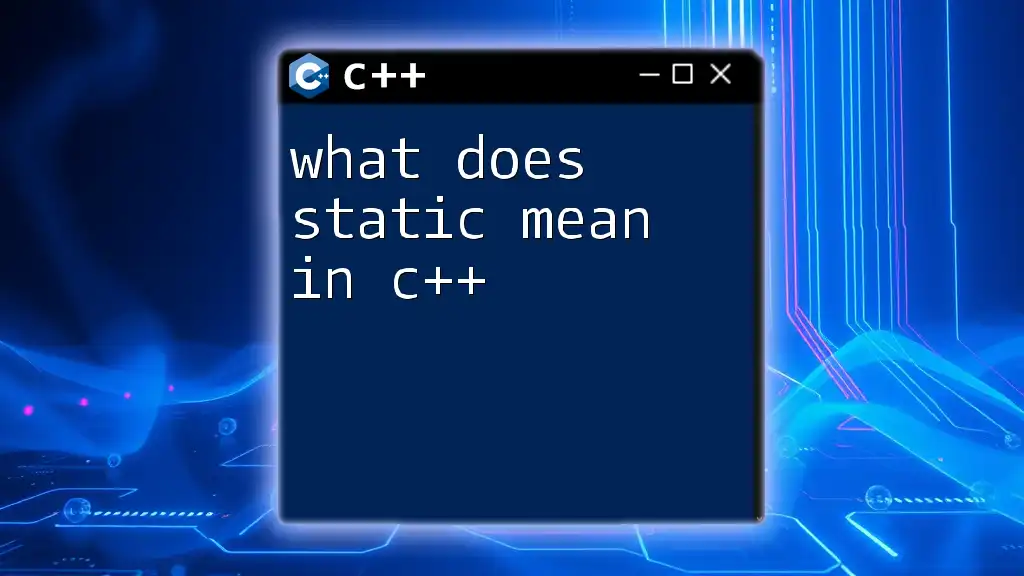
Conclusion
Understanding what does char mean in C++ is fundamental to effective programming in the language. The char data type is versatile, easy to use, and forms a building block for more complex data types like strings. Mastering the char type not only enhances your coding skill but also enriches your understanding of memory management and data representation in C++.
As you embark on your C++ journey, practice creating and manipulating char variables and experiment with their various capabilities to solidify your knowledge. With practice, you will find that the power of the char data type opens up a world of possibilities in software development.
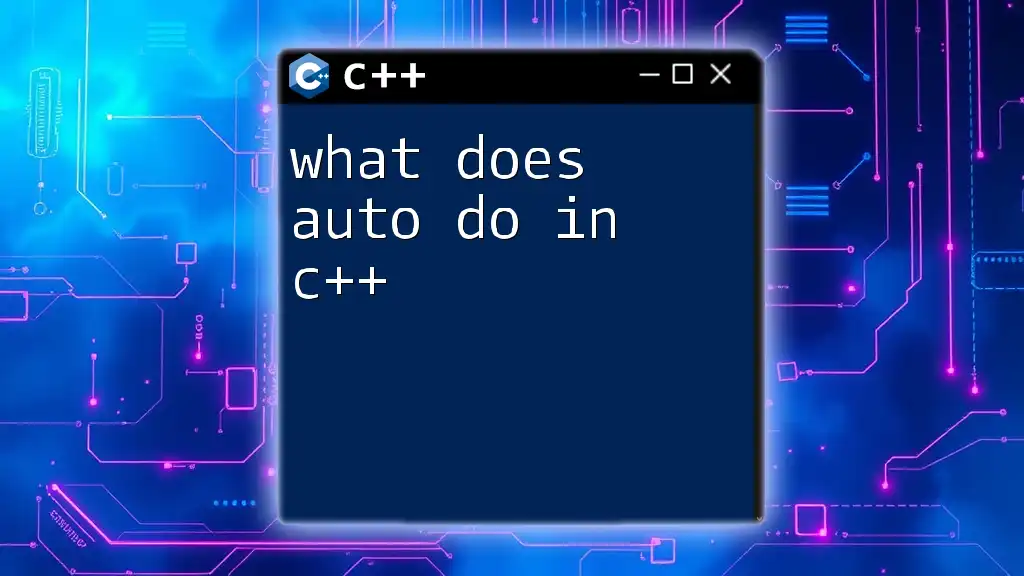
References and Further Reading
For a deeper dive into the char data type and its applications in C++, consider exploring official C++ documentation, online courses, and programming books that focus on data structures and algorithms.