In C++, the `this` pointer refers to the object for which a member function is called, allowing access to the object's attributes and methods within that function.
Here's a simple example of its usage:
#include <iostream>
class MyClass {
public:
void display() {
std::cout << "Object address: " << this << std::endl;
}
};
int main() {
MyClass obj;
obj.display();
return 0;
}
Understanding C++ Syntax
What is Syntax in C++?
In C++, syntax refers to the set of rules that define the combinations of symbols and keywords that are considered to be correctly structured programs. Arguably, it forms the backbone of your coding experience. Understanding these rules is crucial for writing valid C++ code. Any deviation from this syntax can lead to compiler errors, making your program unreadable and non-functional.
Common Syntax Elements
- Keywords: These are reserved words that have special meanings in C++. Examples include `int`, `return`, and `class`.
- Operators: Operators in C++ perform operations on variables and values. These include mathematical operators like `+` and `-`, as well as logical operators.
- Identifiers: Identifiers refer to names given to elements such as variables, functions, and classes. They must be unique within their scope.
- Literals: These represent fixed values in your code, like integers, floating-point numbers, and strings.
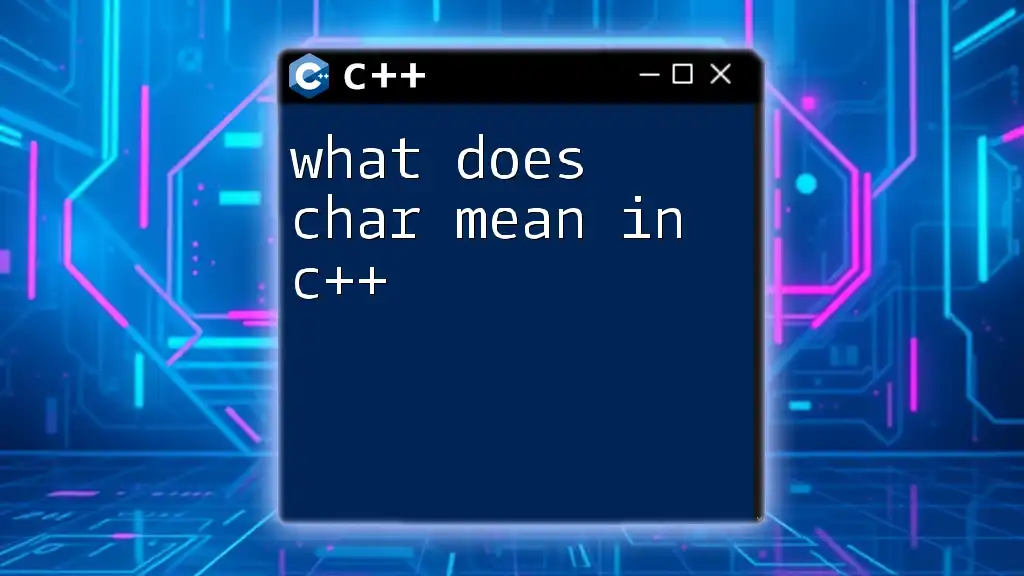
Common C++ Commands and Their Meanings
What Does `#include` Mean?
The `#include` directive is a preprocessor command that allows you to include the contents of a file or library within your code. It's almost like telling the compiler, "Hey, I need this code to function properly."
For example, the following code snippet includes the input-output stream library:
#include <iostream>
This line of code gives you access to basic functionalities like input via `std::cin` and output via `std::cout`.
What Does `main()` Mean?
The `main` function is the entry point of any C++ program. Every C++ application starts executing from this function. It's essential to define this function in your code, as it is where your program will begin execution.
Here's a simple example:
int main() {
return 0;
}
In this example, `return 0;` indicates successful program completion to the operating system.
What Does `cout` Mean?
`cout` is an output stream used to display information on the console. It is part of the standard library and requires the `iostream` header file for its definition.
Here's how you might use it:
std::cout << "Hello, World!" << std::endl;
In this code snippet, `std::cout` prints "Hello, World!" to the console, and `std::endl` inserts a newline character, moving the cursor to the next line.
What Does `cin` Mean?
While `cout` displays output, `cin` helps you take input from the user via standard input (usually the keyboard). This command also requires the `iostream` library.
For example:
int age;
std::cout << "Enter your age: ";
std::cin >> age;
This code prompts the user to enter their age and stores that input in the variable `age`.
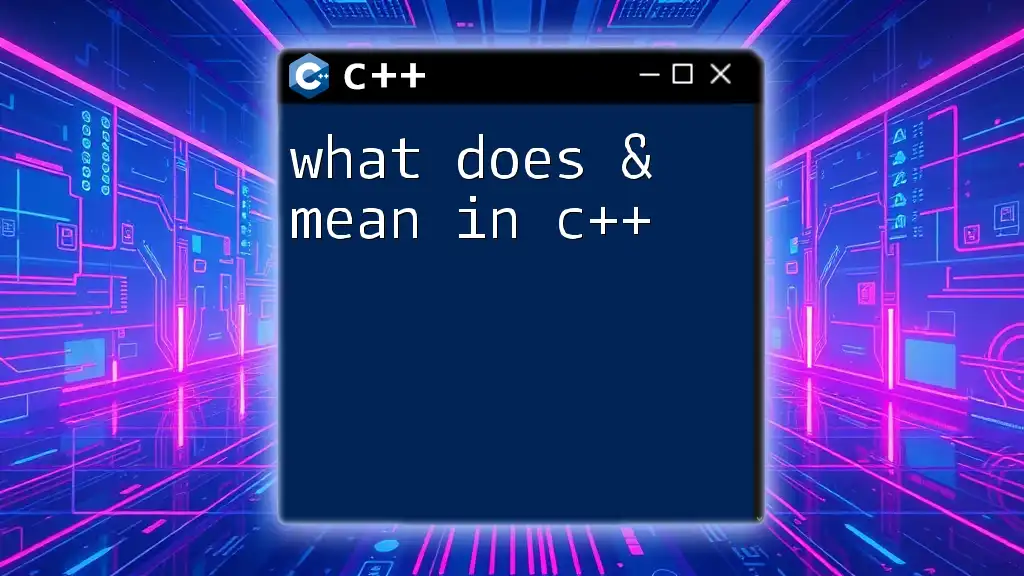
Common Terms and Phrases in C++
Object-Oriented Concepts
What Does `class` Mean?
In C++, a class is a blueprint for creating objects. It encapsulates data and functions that manipulate that data into one single unit. This allows for better organization and reusability of code.
Here's a simple class definition:
class Car {
public:
void drive() {
std::cout << "The car is driving." << std::endl;
}
};
In this example, the class `Car` has a public method `drive`, which when called, will execute the code inside its definition.
What Does `public`, `private`, and `protected` Mean?
These are access specifiers that determine how members of a class can be accessed.
- public: Members can be accessed from outside the class.
- private: Members can only be accessed within the class itself, ensuring data encapsulation.
- protected: Members can be accessed in the class itself and in derived classes.
Here’s an example:
class Person {
private:
std::string name;
public:
void setName(std::string n) {
name = n;
}
};
In this code, the member variable `name` is private and cannot be accessed directly from outside the class.
Understanding Functions
What Does `void` Mean?
The `void` keyword indicates that a function does not return any value. When a function is declared as `void`, it simply performs its operations without sending any information back to the place where it was called.
For instance:
void displayMessage() {
std::cout << "Hello from a void function!" << std::endl;
}
In this example, `displayMessage` performs an action (printing a message) but doesn’t return any value.
What Does `return` Mean?
The `return` statement serves two main purposes: to exit a function and to provide a value back to the caller.
When used in a non-void function, it communicates the outcome of the function.
Example:
int add(int a, int b) {
return a + b;
}
This `add` function takes two integers, adds them, and returns the result. The integer returned can be used elsewhere in the program.
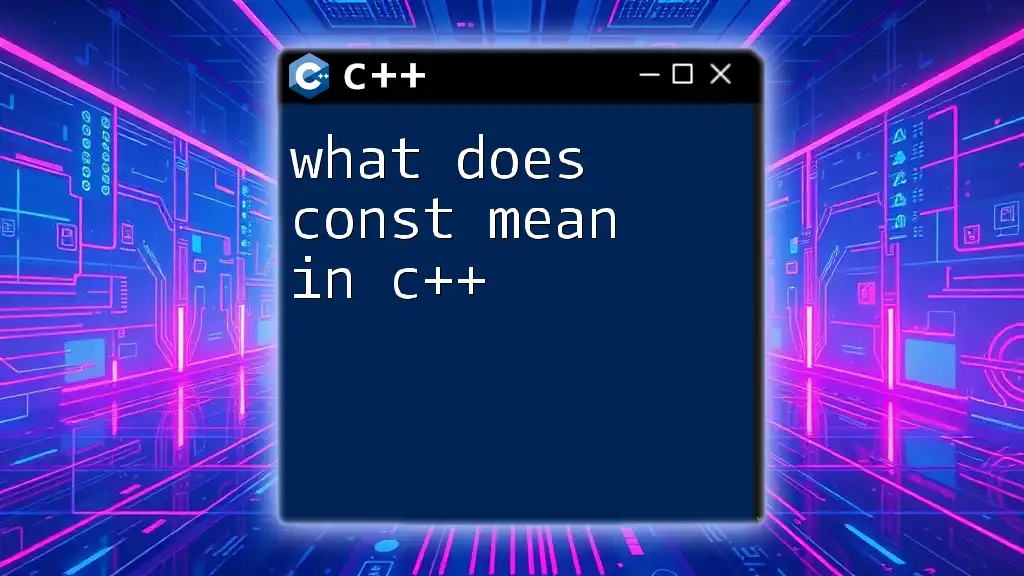
Debugging Common Misinterpretations
Common Errors and Their Meanings
What Does an `Undefined Reference` Error Mean?
This error typically arises during the linking stage of compilation when the code references a function that the compiler cannot find. It often indicates that you have declared a function but forgot to define it.
Consider the following:
void myFunction(); // Declared but no definition
To fix this error, ensure that every declared function has a corresponding definition.
What Does a `Segmentation Fault` Mean?
A segmentation fault occurs when your program tries to access a memory segment that it is not allowed to access. Common examples include dereferencing null or invalid pointers.
Here is an example:
int* ptr = nullptr;
*ptr = 5; // This line causes a segmentation fault
In this code, attempting to assign a value to a null pointer leads to this runtime error. Always ensure pointers are properly initialized before use.
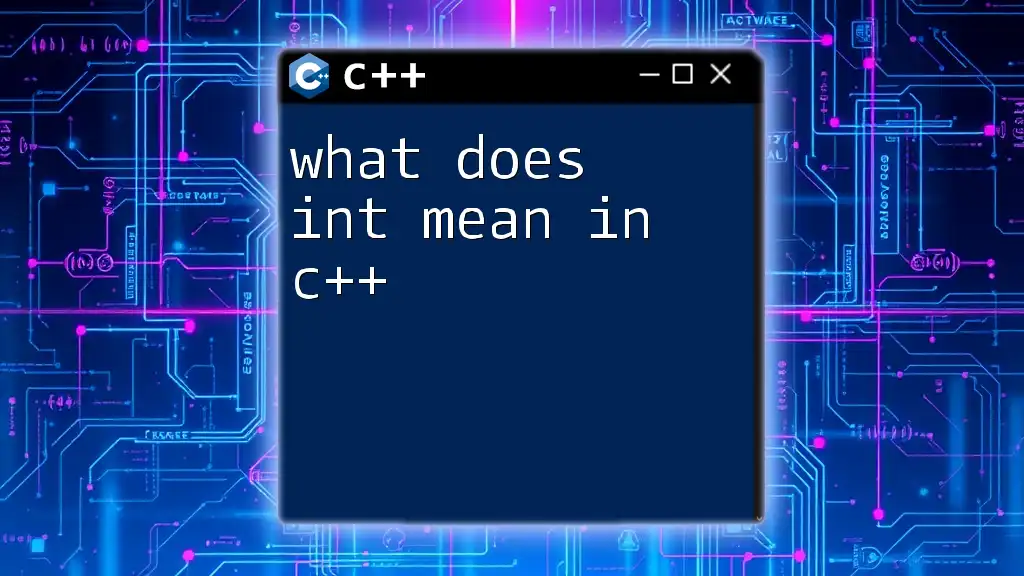
FAQs about C++ Commands and Terminology
-
What does `namespace` mean?
A namespace is a declarative region that provides a scope to the identifiers (the names of types, functions, variables, etc.) inside it. Using `namespace std;` allows access to the `std` library without prefixing commands with `std::`. -
What is the purpose of `const`?
The `const` keyword signifies that a variable’s value cannot be modified after its initialization, enforcing immutability. -
What is a template?
Templates are a feature of C++ that allows you to create functions or classes that work with any data type.
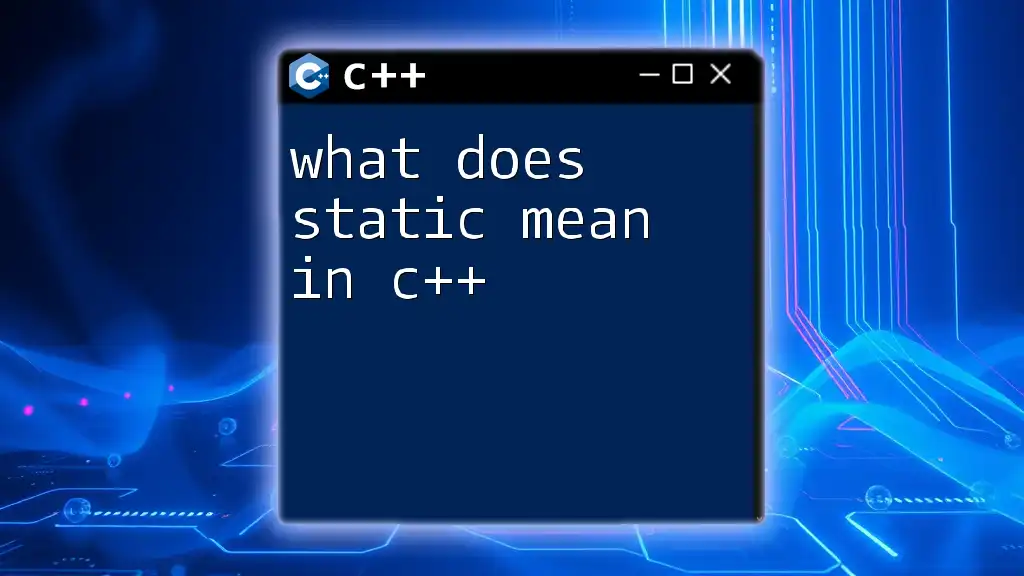
Conclusion
Throughout this article, we've explored various C++ commands and terminology that are vital for understanding the language. By breaking down statements like `#include`, `cout`, and `main`, we have built a clearer picture of what these concepts mean. As you continue to practice and expand your C++ skills, remember that every command comes with its own significance and utility.
By diving deeper into C++, you will unlock its full potential, making you a more effective programmer. For additional learning, consider joining our community for further studies and resources tailored specifically to help you succeed in C++.