In C++, the `static` keyword extends the lifetime of a variable to the duration of the program, meaning it retains its value between function calls and is visible only within the scope it is defined.
Here’s a code snippet demonstrating the use of static:
#include <iostream>
void increment() {
static int count = 0; // Static variable
count++;
std::cout << "Count: " << count << std::endl;
}
int main() {
increment(); // Output: Count: 1
increment(); // Output: Count: 2
increment(); // Output: Count: 3
return 0;
}
Understanding the Keyword `static`
The `static` keyword in C++ serves multiple purposes, fundamentally altering the way variables and functions are utilized. At its core, `static` indicates that the variable or function has a persistent state that remains in memory across function calls or is limited in scope to the file or class where it is declared.
Different Contexts for `static`
Static Local Variables
Static local variables are initialized only once and retain their value even after the function has completed execution. This leads to behavior that differs noticeably from regular local variables, which are destroyed once the function exits.
Example Code Snippet:
void function() {
static int count = 0; // This variable retains value between function calls
count++;
std::cout << count << std::endl;
}
In the example above, each time `function` is called, the static variable `count` does not reset to zero. Instead, it increments based on the number of function calls, allowing you to track how many times the function has been invoked.
Static Global Variables
A static global variable, in contrast, is confined to the file in which it is declared, preventing other files from accessing it. This encapsulation is particularly useful in larger projects to avoid naming conflicts and unintended interactions between different file scopes.
Example Code Snippet:
static int globalCount = 0; // Accessible only within this file
Here, `globalCount` is a private global variable. Any code in other files will be unable to access or modify this variable, promoting better data encapsulation.
Static Member Variables
Static member variables in classes are shared across all instances of that class. They differ from regular member variables in that they are not tied to a specific instance but are instead class-oriented.
Example Code Snippet:
class MyClass {
public:
static int objCount; // Class-level variable shared among objects
};
int MyClass::objCount = 0; // Definition outside the class
As shown, `objCount` keeps track of how many instances of `MyClass` have been created, giving developers a simple way to monitor object creation without needing to instantiate an object for access.
Static Member Functions
Similar to static member variables, static member functions belong to the class itself rather than any individual object. They can be called without creating an instance of the class, making them useful for utility functions.
Example Code Snippet:
class MyClass {
public:
static void displayCount() {
std::cout << "Total objects: " << objCount << std::endl;
}
};
In this case, you can call `MyClass::displayCount()` even if no `MyClass` object exists, allowing operations that do not rely on instance-specific data.
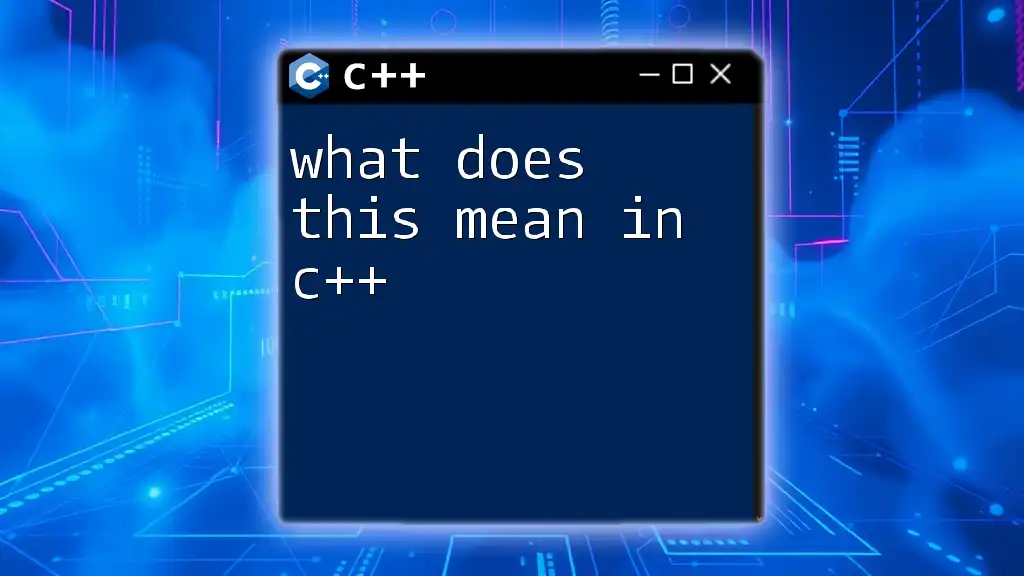
What Does Static Do in C++?
Memory Management with Static
The memory management characteristics of static variables are crucial for understanding C++. Static variables are allocated in a special memory segment, distinct from automatic (stack) and dynamic (heap) allocation.
When a program runs, a static variable is created once and persists for the lifetime of the program, allowing it to retain its value between function calls or access throughout the program (for static globals).
Scope and Lifetime of Static Variables
The scope defines where a variable can be accessed from, while its lifetime determines how long it exists in memory. Static variables have a lifetime that lasts for the entire program execution but may have limited scope, depending on whether they are declared globally or locally within functions or classes.
Key Consideration: A static variable initialized within a function can still be accessed only within that function, but it will live as long as the program does, unlike a typical local variable that is destroyed at the end of the function's execution.
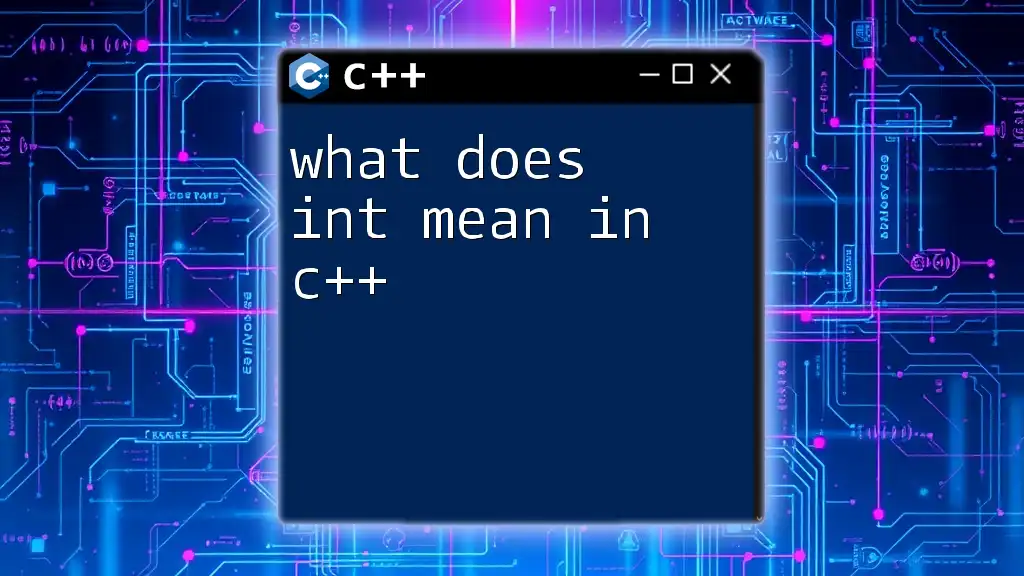
Advantages of Using Static
Reduced Memory Usage: Static variables consume memory only once during the program's lifetime, as they do not need re-initialization each time a function is called.
Data Sharing Across Instances: Static member variables allow shared state among multiple instances of a class, making them invaluable for counting or keeping track of objects.
Enhanced Performance: Because static variables and functions can be accessed without instance creation, they often lead to improved performance due to reduced overhead in object management.
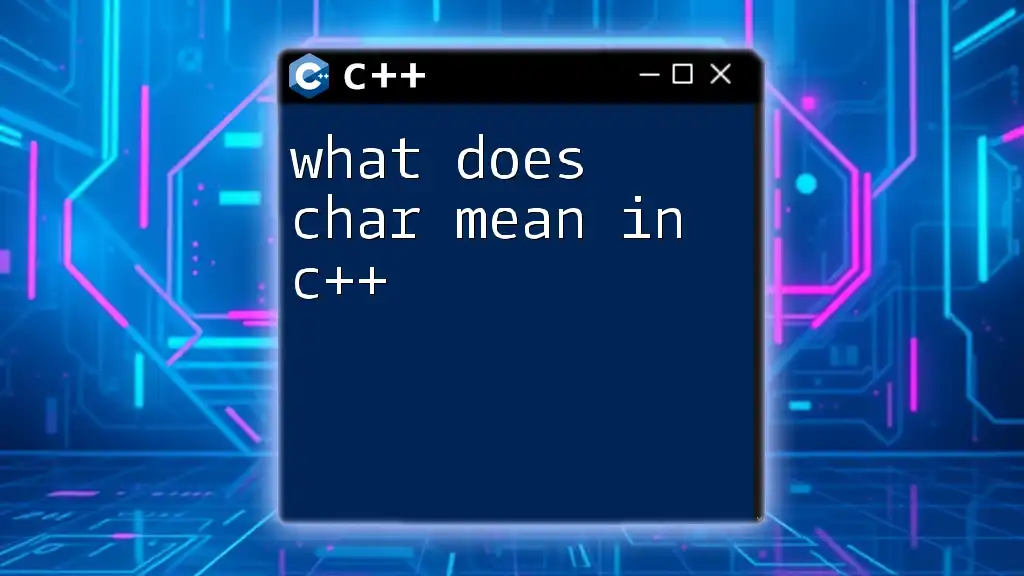
Disadvantages of Using Static
Global State Management: While static variables can be beneficial, they can introduce complications in multi-threaded environments. A shared static variable may produce race conditions, where multiple threads access and change its value simultaneously.
Limited Flexibility: Once static variables are declared, they cannot change their storage duration. This can limit flexibility, making it challenging to use them in contexts where dynamic behavior is preferred.
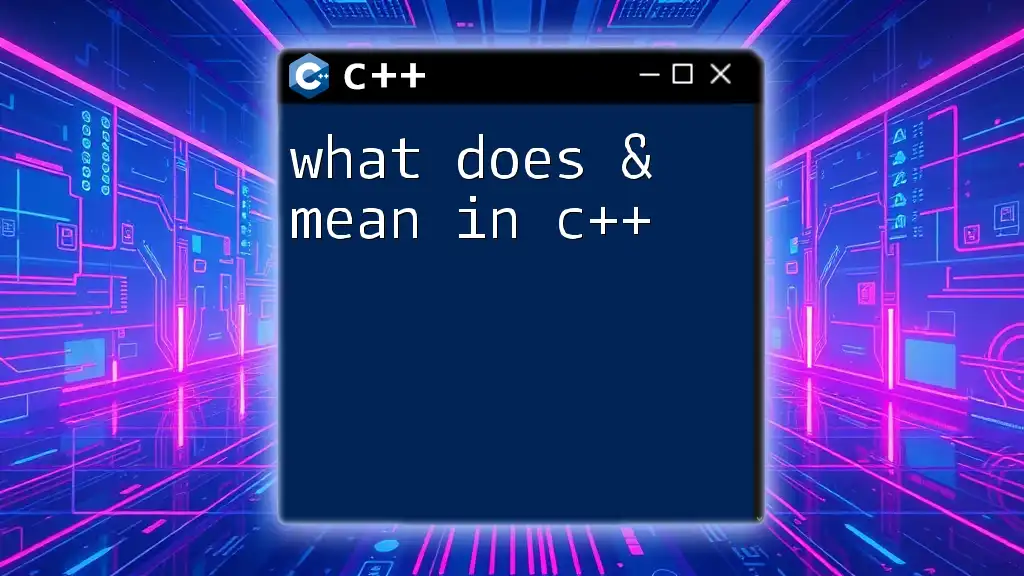
Practical Use Cases of Static in C++
C++ developers employ static variables and functions in various scenarios, such as tracking the number of instances created from a class or creating singleton patterns where only one instance of a class is needed.
Example Scenario: Counting Object Instantiations
Consider the following example demonstrating multiple use cases:
#include <iostream>
class Counter {
public:
static int count; // Static member variable
Counter() { count++; }
static void displayCount() {
std::cout << "Current count: " << count << std::endl;
}
};
int Counter::count = 0;
int main() {
Counter c1, c2;
Counter::displayCount(); // Shows total objects created
return 0;
}
In this code, each time a new `Counter` object is created, the static variable `count` is incremented. The static member function `displayCount` can then be used to output the current count of `Counter` instances without needing to instantiate an object, showcasing how `static` can simplify code and enhance functionality.
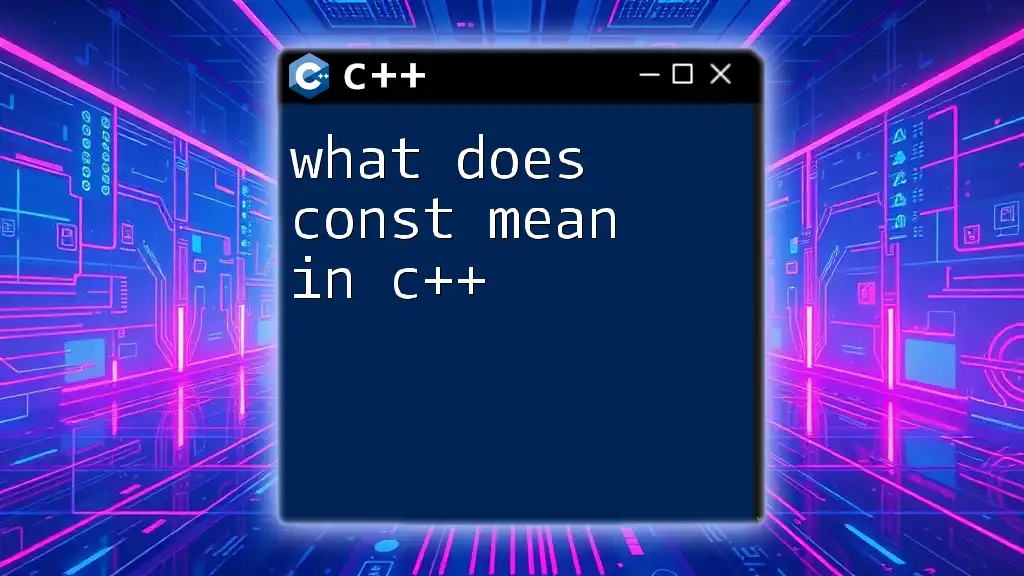
Conclusion
In summary, understanding what static means in C++ is vital for any developer aiming to leverage the language’s capabilities effectively. The `static` keyword provides a robust framework for managing data across various contexts and durations, promoting better memory management and encapsulation. As you continue to explore C++, experiment with static variables and functions to see firsthand how they can enhance your programming practices.
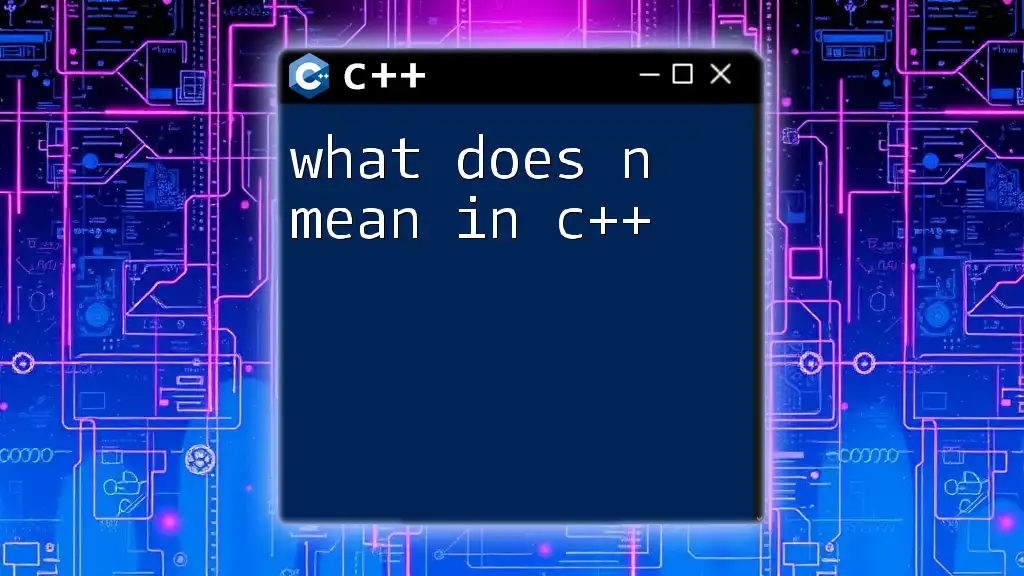
Additional Resources
For more in-depth understanding of C++ memory management techniques and design patterns, consider exploring detailed guides and relevant courses that delve into leveraging static variables effectively.
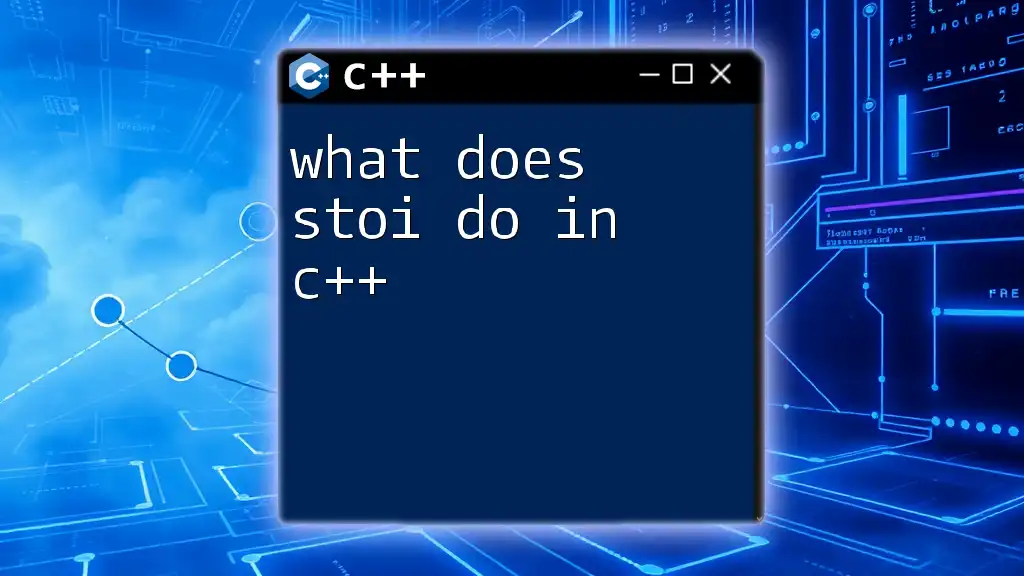
FAQs
What are the main differences between static and non-static variables?
Static variables retain their value across function calls and live for the entire lifetime of the program, while non-static variables exist only for the duration of the function call.
Can you initialize a static variable inline?
Yes, this is permitted. However, the definition of the static variable must occur outside the class for static member variables.
What happens if a static variable is declared in a header file?
A static variable in a header file is defined in a way that each compilation unit gets its copy. This keeps it unique to that unit and avoids linkage issues with other translation units.