The `substr` function in C++ is used to extract a substring from a given string, starting at a specified index and continuing for a specified length.
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World!";
std::string sub = str.substr(7, 5); // Extracts "World"
std::cout << sub << std::endl;
return 0;
}
What is a Substring in C++
A substring is any contiguous sequence of characters within a string. For example, in the string "Hello, World!", "Hello" and "World" are both substrings. Understanding substrings is crucial for various programming tasks, such as text searching, data parsing, and even in applications involving user input.
Real-World Applications
Substrings find utility in numerous scenarios. Whether you're extracting user information from a text file or manipulating strings to create more readable outputs, mastering the concept of substrings is essential for effective programming in C++.
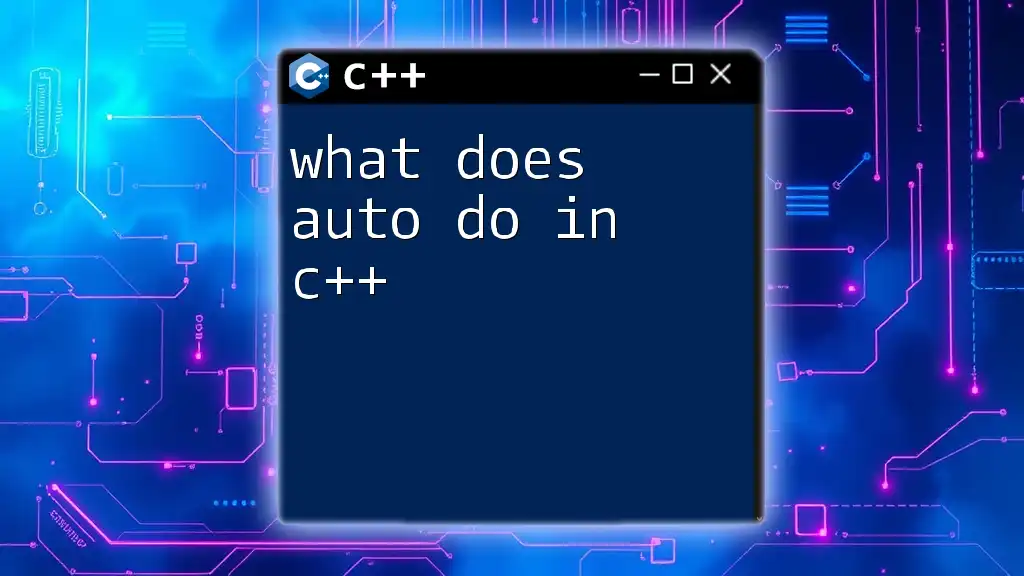
Understanding the substr Function in C++
The `substr` function is part of the C++ Standard Library and is used to obtain a substring from a given string.
Basic Syntax of the substr Function
The syntax for the `substr` function is straightforward:
string.substr(start_index, length);
- start_index: This is the zero-based index at which you wish to start the substring.
- length: This optional parameter specifies the number of characters to include in the substring. If not provided, the substring will extend to the end of the original string.
Return Value
The `substr` function returns a new string that contains the requested substring extracted from the original string.
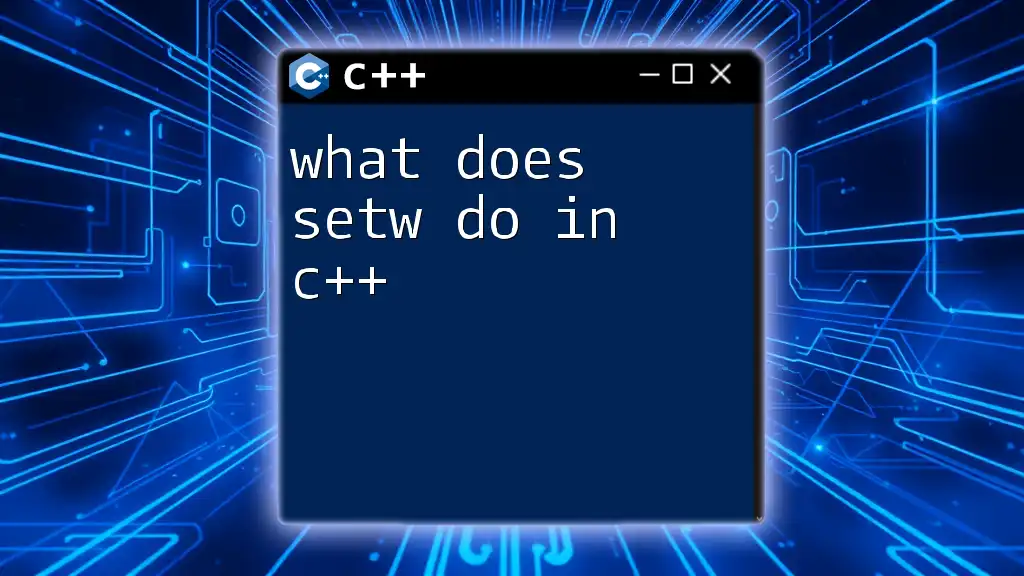
How to Use Substring in C++
To begin using the `substr` function, you first need to include the necessary headers for string manipulation. Here's a basic example:
#include <iostream>
#include <string>
using namespace std;
Next, you can create a string and use the `substr` function. Consider the following example where we extract a substring:
string example = "Hello, World!";
string sub = example.substr(7, 5); // "World"
cout << sub << endl; // Output: World
In this code, we start the substring at index `7` and specify that we want a length of `5`. The result is "World".
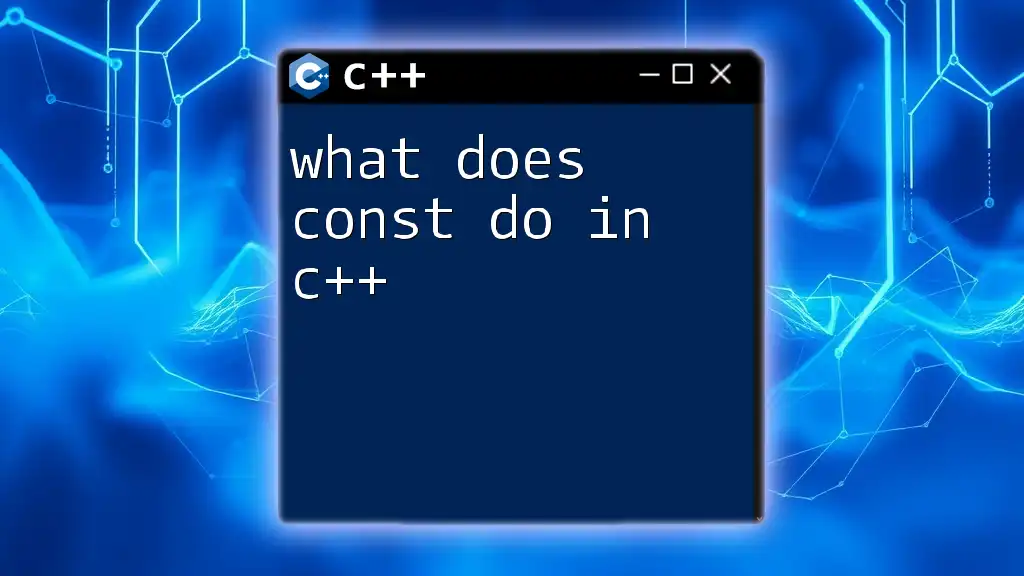
Example Scenarios for Using substr in C++
Extracting a Word from a Sentence
The `substr` function is particularly useful for extracting individual words from a longer sentence. For instance:
string sentence = "Learning C++ can be fun.";
string word = sentence.substr(9, 3); // "C++"
cout << word << endl; // Output: C++
In this example, we start at index `9` to extract the substring "C++", which has a length of `3`.
Trimming a String
Another practical use for `substr` is trimming whitespace or unwanted characters from the ends of a string. Here's how it can be done:
string text = " Trim this text ";
string trimmed = text.substr(2, text.size() - 4); // "Trim this text"
cout << trimmed << endl; // Output: Trim this text
Here, we use `substr` to capture the intended portion of the string while excluding leading and trailing spaces.
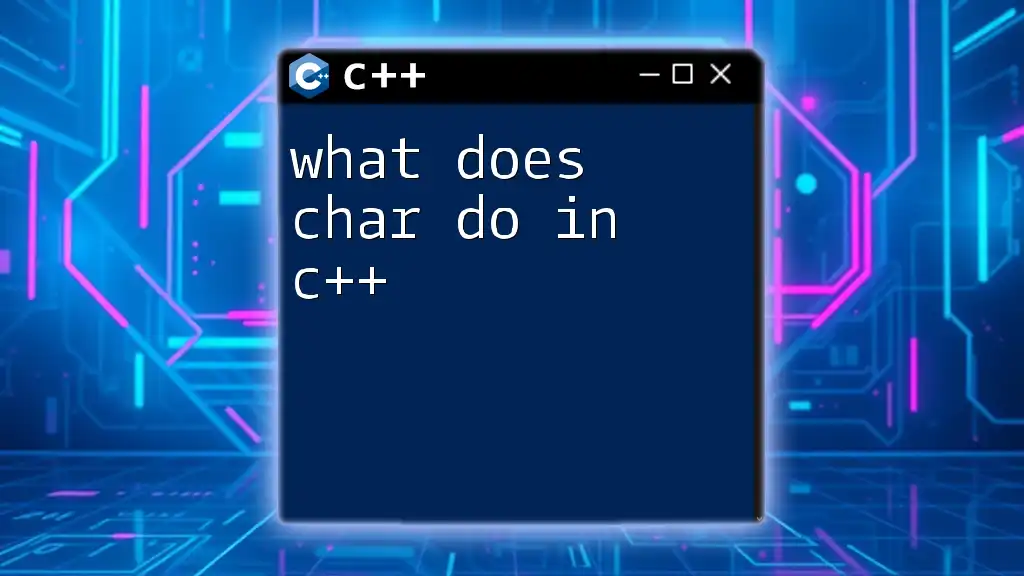
Error Handling with the substr Function in C++
It's essential to handle potential errors when using the `substr` function, particularly when the specified indices are out of the valid range. If you attempt to call `substr` with indices that exceed the string's length, you can encounter exceptions.
For instance, consider the following:
try {
string test = "Error handling";
string invalid = test.substr(20, 5); // This will cause an out-of-range error
} catch (const out_of_range& e) {
cout << "Out of range: " << e.what() << endl;
}
In this code, we attempt to extract a substring starting at index `20`, which does not exist. By wrapping our code in a try-catch block, we can effectively catch the out-of-range exception and handle it gracefully.
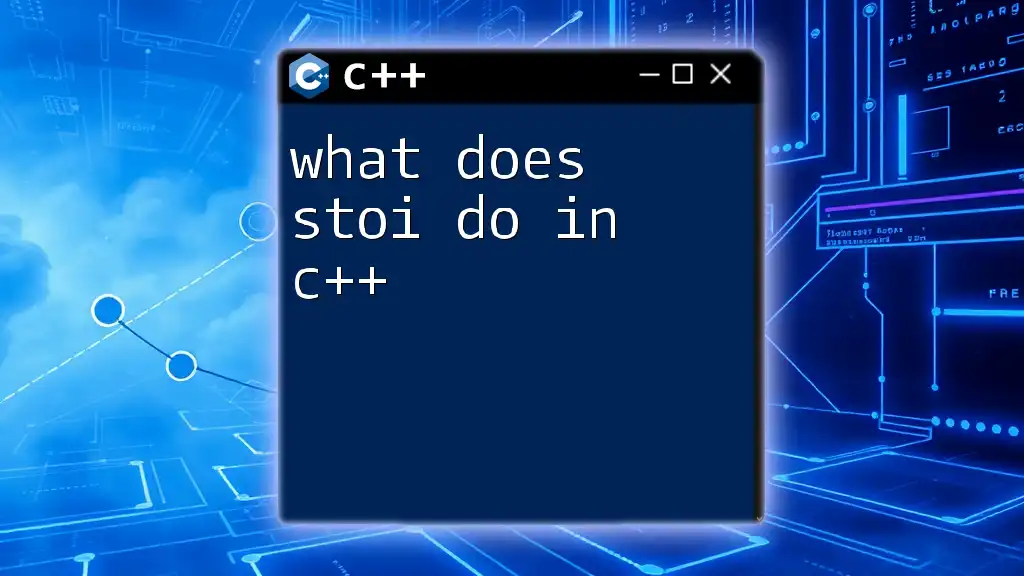
Performance Considerations
When dealing with large strings or performing multiple substring operations, it's crucial to be mindful of performance. Each call to `substr` creates a new string, which can lead to increased memory use and slower execution times in performance-critical applications.
Best Practices
To optimize string manipulation, consider:
- Use `substr` judiciously and avoid excessive calls within loops.
- Where possible, operate on string references instead of creating new strings repeatedly.
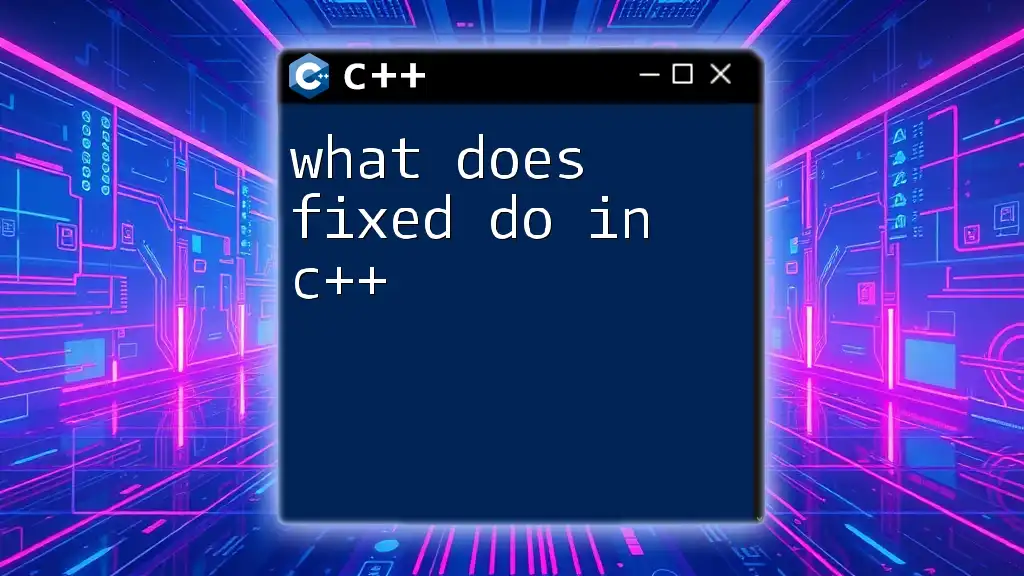
Common Use Cases of the C++ Substr Function
The `substr` function is a common tool in many programming tasks, particularly in data processing and text manipulation. Examples include:
- Parsing CSV files: Extracting fields from a delimited string.
- User input validation: Checking specific parts of an input using substrings.
- Text searching and manipulation: Modifying specific parts of user-generated content.
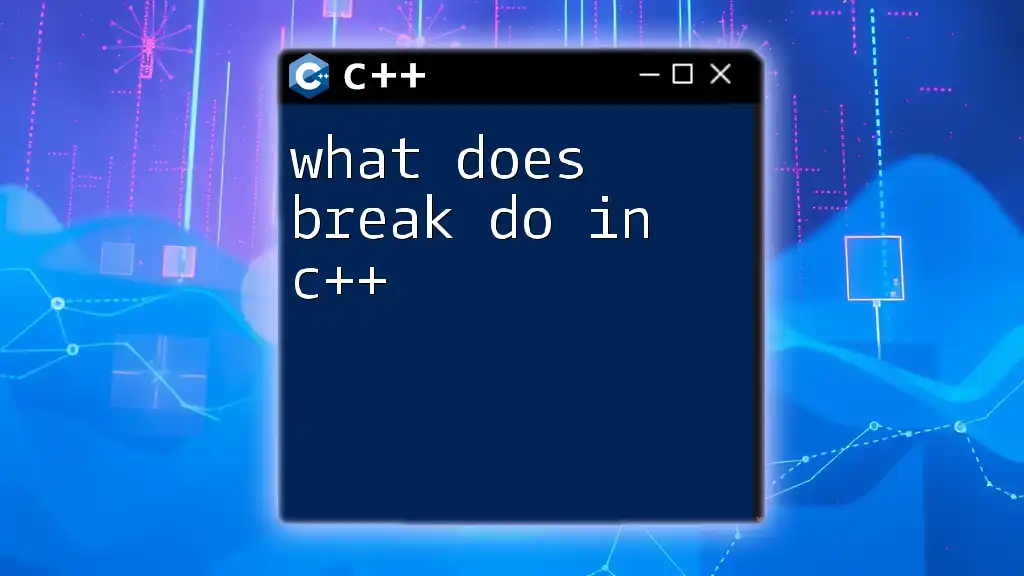
Conclusion
In summary, understanding what does substr do in C++ is fundamental for anyone looking to manipulate strings effectively. The `substr` function is versatile and can be used in various practical scenarios to extract meaningful portions of a string. Practice using `substr` with different strings to solidify your understanding and become proficient in string manipulation techniques in C++.
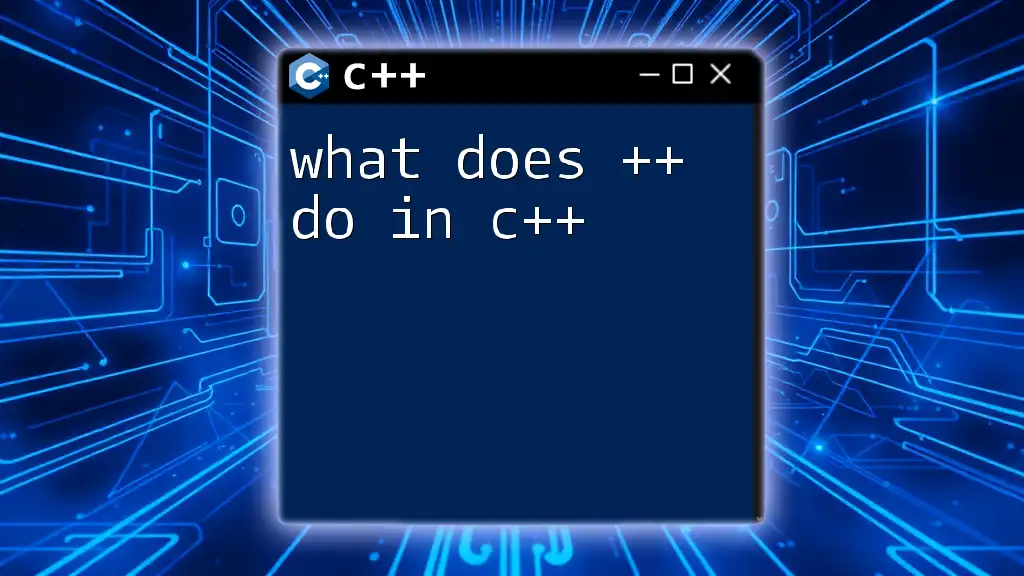
Call to Action
If you found this guide helpful, consider following our blog or enrolling in our courses for more specialized C++ programming insights and tutorials.
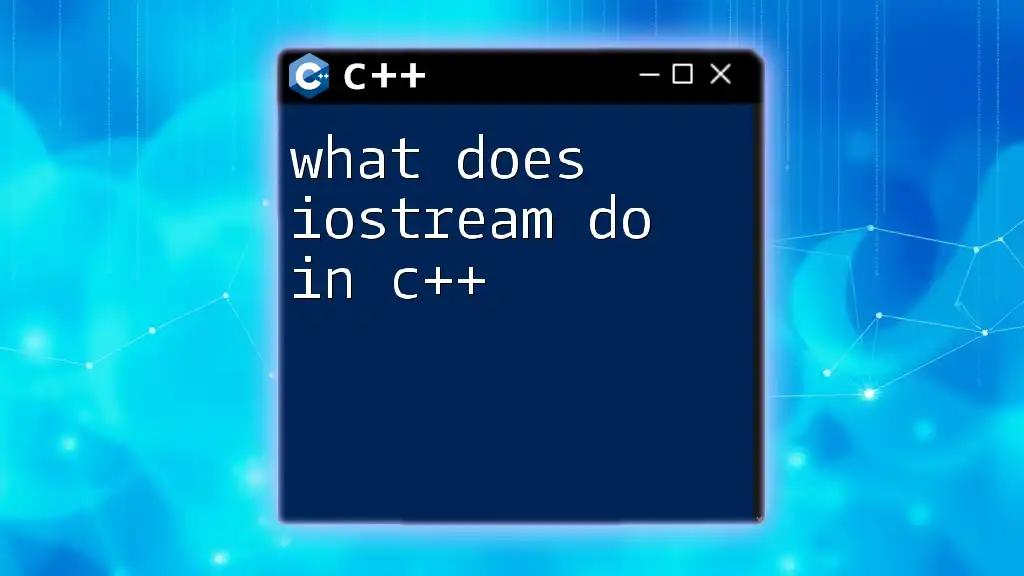
Further Reading and Resources
To expand your knowledge further, refer to the official C++ documentation and explore additional topics related to string manipulation and other C++ functionalities.