In C++, `n` often represents an integer variable used to indicate the size of an array, a loop counter, or a specific value, depending on the context of the code.
Here's a simple code snippet demonstrating its use as a loop counter:
#include <iostream>
using namespace std;
int main() {
int n = 5; // Example value for n
for (int i = 0; i < n; i++) {
cout << "Iteration " << i + 1 << endl;
}
return 0;
}
Why Naming Conventions Matter
Naming conventions play a crucial role in programming, particularly in languages like C++. A well-chosen variable name can enhance code readability, allowing other developers (or even your future self) to quickly understand the purpose of a variable. Misleading or overly concise variable names can create confusion and hinder the code's maintainability.
Common Variable Naming Practices
In C++, developers often adhere to several common naming practices:
- Pascal Case: Each word in the identifier is capitalized (e.g., `MyVariable`).
- Camel Case: The first word is lowercase, and each subsequent word is capitalized (e.g., `myVariable`).
- Snake Case: Words are separated by underscores (e.g., `my_variable`).
Best Practices for Variable Names
The best practice in variable naming is to use meaningful names. While single-letter names like 'n' can be practical in specific contexts, they should generally be avoided when they don’t convey information about their purpose. The clarity of the code often outweighs the brevity of variable names.
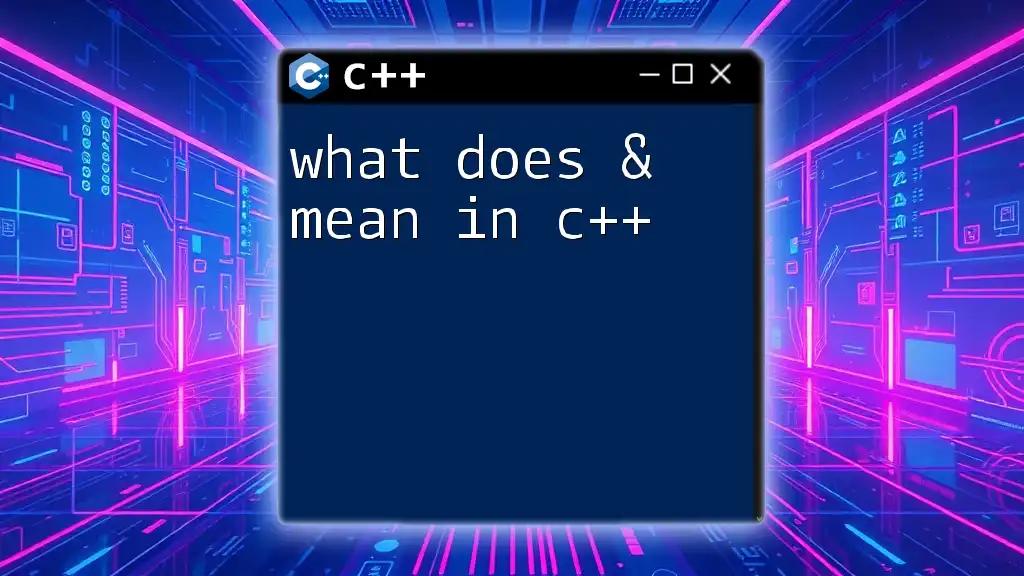
Common Uses of 'n' in C++
The letter 'n' is frequently employed as a variable name in C++ programming. It typically denotes a number or count in various contexts, which we will explore in detail.
'n' as a Variable for Count
One of the most prevalent uses of 'n' is as a counter in loops. This makes it easier to understand the number of iterations taking place.
Example:
for (int n = 0; n < 10; n++) {
std::cout << n << " ";
}
In this example, 'n' represents the current iteration of the loop, starting from 0 and incrementing until it reaches 9. The output will be a sequence of numbers from 0 to 9. This usage illustrates how 'n' allows for clear counting and iteration in code.
'n' as an Array Size
Another common application of 'n' is when defining the size of arrays. This provides clarity about the total number of elements an array can hold.
Example:
int arr[n];
In this example, 'n' specifies how many integers the array `arr` will contain. Using 'n' in this context is a standard practice, although it’s essential to ensure that 'n' is initialized before use to prevent undefined behavior.
'n' in Mathematical Functions
The letter 'n' is often used in mathematical algorithms or functions to represent a quantity or element count. This allows for concise expressions of mathematical ideas.
Example:
int factorial(int n) {
return (n <= 1) ? 1 : n * factorial(n - 1);
}
In this recursive function, 'n' represents the number for which we are calculating the factorial. The function reads straightforwardly, and using 'n' helps to keep the mathematical context clear.
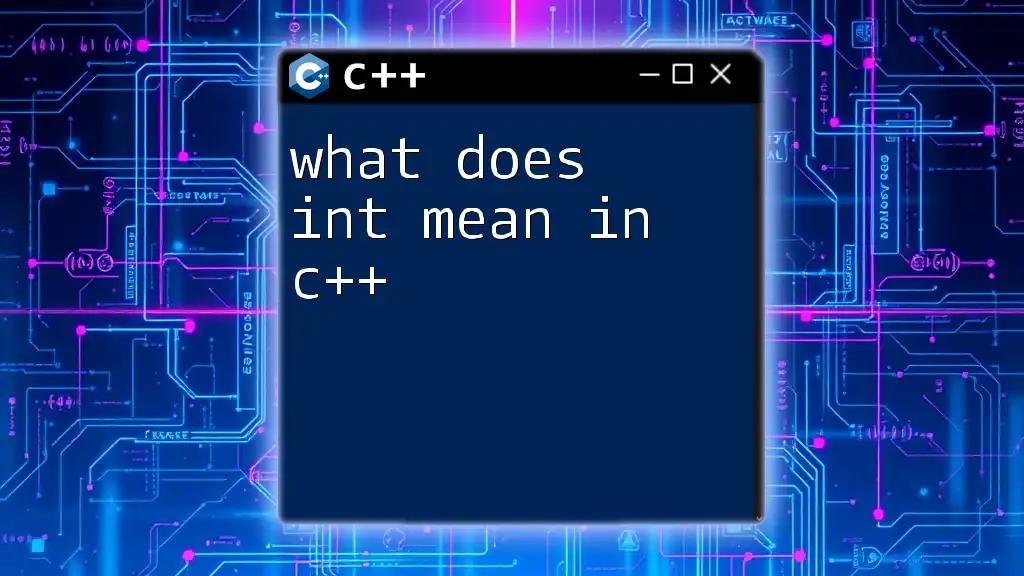
Case Studies: 'n' in Algorithms
'n' in Sorting Algorithms
In algorithm analysis, 'n' is a common variable to denote the number of elements being processed in sorting algorithms. It is vital in understanding the algorithm's complexity.
Example Code Snippet:
void bubbleSort(int arr[], int n) {
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
std::swap(arr[j], arr[j + 1]);
}
}
}
}
In this bubble sort implementation, 'n' indicates the size of the input array `arr`. This allows us to explain the time complexity of the algorithm, which is O(n^2) in the worst case, as the nested loops run for 'n' iterations.
'n' in Search Algorithms
Similarly, 'n' is often seen in search algorithms, where it typically denotes the number of elements being searched.
Example Code Snippet:
bool linearSearch(int arr[], int n, int x) {
for (int i = 0; i < n; i++) {
if (arr[i] == x) {
return true;
}
}
return false;
}
In this linear search function, 'n' indicates the total number of elements in the array `arr`. As the algorithm checks each element in the array, it's easy to see how the concept of 'n' helps clarify the method's efficiency.
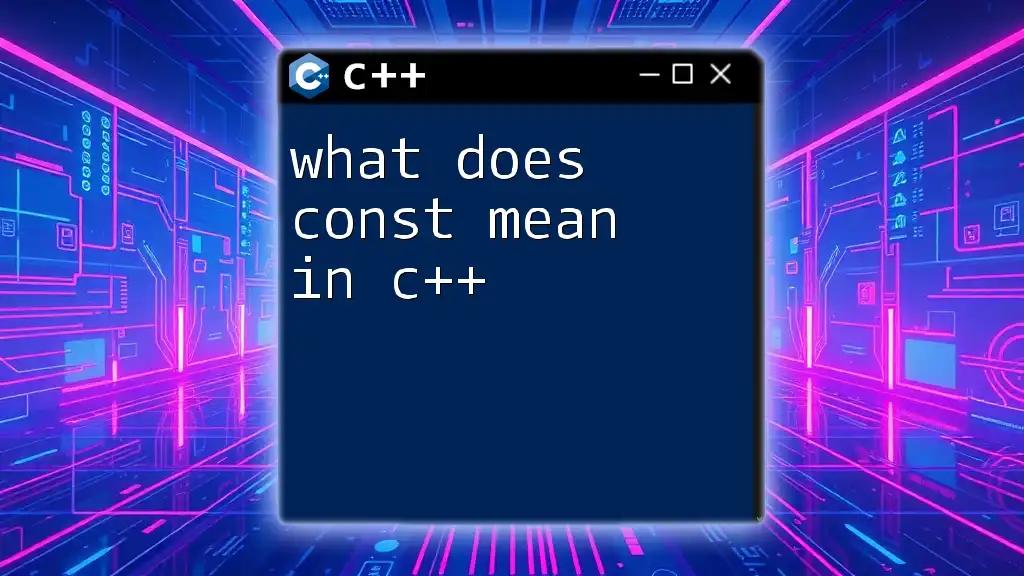
Similar Single-Letter Variables
Beyond 'n', several other single-letter variables are commonly used in programming.
'm' for Memory/Size
In some contexts, particularly when dealing with multiple dimensions or arrays, 'm' may be used to denote a second dimension or the number of items in a collection.
'k' for Number of Elements or Thousands
Another frequent variable in mathematical formulas or data processing is 'k', often used to denote the number of groups/elements, particularly when another variable like 'n' is already in use.
The Importance of Context
Being aware of these conventions underscores the importance of context when choosing variable names. While 'n' is a universally understood variable in many situations, it’s crucial to ensure that your code is readable and well-understood by others or yourself in the future.

Conclusion
In summary, the letter 'n' in C++ is a widely accepted naming practice used primarily to signify counts, array sizes, and elements in algorithms. By adhering to proper naming conventions, developers can create more maintainable and understandable code. Remember, while 'n' may be a convenient variable, clear communication through meaningful naming is key to effective programming.

Call to Action
As you continue your journey in learning C++, challenge yourself to implement what you've learned about the use of 'n' and other variables in your practice code. Share your thoughts or questions with your peers or in developer communities to deepen your understanding!

Additional Resources
For further reading and exploration, consider checking out tutorials on C++ best practices, algorithm analysis, and variable conventions to enhance your programming skills!