In C++, a boolean is a data type that can hold one of two possible values: `true` or `false`, often used for conditional statements and logical operations.
Here's a simple code snippet demonstrating the use of a boolean in C++:
#include <iostream>
using namespace std;
int main() {
bool isSunny = true; // True represents sunny weather
if (isSunny) {
cout << "It's a sunny day!" << endl;
} else {
cout << "It's not a sunny day." << endl;
}
return 0;
}
Understanding Boolean Values
In C++, Boolean variables are a fundamental part of programming that allow for decision-making processes. These variables can hold only two possible values: true or false.
Boolean values are crucial in control flow structures such as if statements, loops, and conditions. By using Booleans, programmers can create dynamic and responsive applications that react differently based on user input or other criteria.

What is `bool` in C++?
The `bool` data type in C++ is specifically designed to represent Boolean values. It is a simple but powerful data type that allows developers to manipulate and evaluate conditions effectively.
To declare a Boolean variable, you can use the following syntax:
bool isActive;
isActive = true; // or false
Using `bool` not only simplifies conditional checks but also contributes to making the code more readable. When it comes to memory, `bool` generally uses one byte, but it might be optimized for performance by compilers.
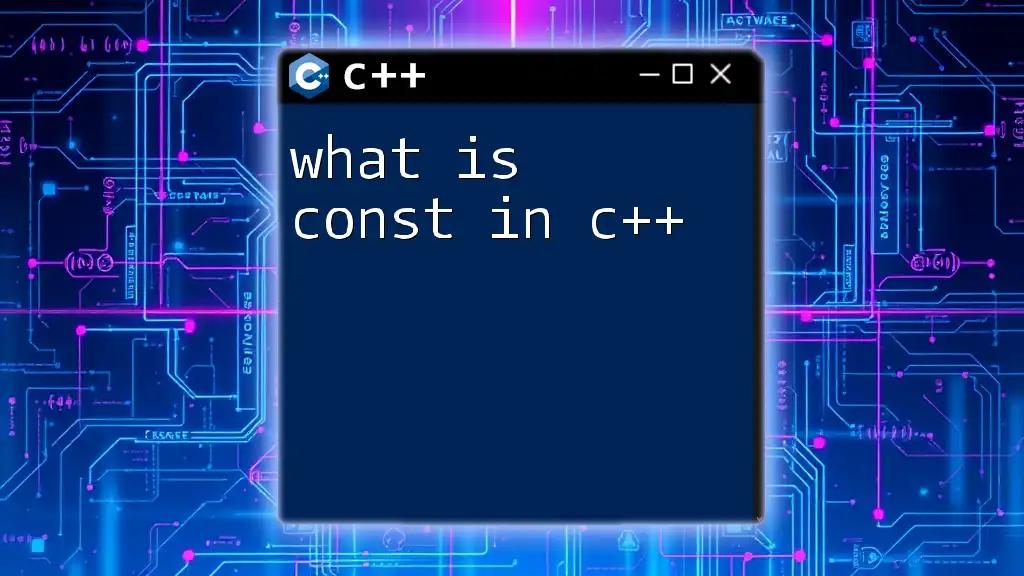
Using Boolean Logic
Logical Operators
Logical operators are fundamental when working with Boolean values. Here are three primary logical operators:
- AND (`&&`): Evaluates to true if both operands are true.
- OR (`||`): Evaluates to true if at least one operand is true.
- NOT (`!`): Evaluates to the opposite of the Boolean value.
Example: Using these operators in conditions can create complex logical checks:
bool a = true;
bool b = false;
bool result = a && b; // result is false
Control Flow with Booleans
Booleans drive the flow of control in C++. They are often utilized in conditional statements. For instance, an `if` statement works seamlessly with Boolean conditions:
if (isActive) {
// Code to execute if isActive is true
}
The if-else structure is a perfect example of directly utilizing Boolean values, allowing you to define two possible execution paths based on the evaluation of a Boolean condition:
if (isActive) {
// Active code block
} else {
// Inactive code block
}
To further illustrate how nested logic can work with Booleans, consider this example:
if (age > 18) {
if (isActive) {
// Code for active adults
}
}
Here, the program first checks if the age is greater than 18 and then verifies if the user is active, allowing for layered decision-making.
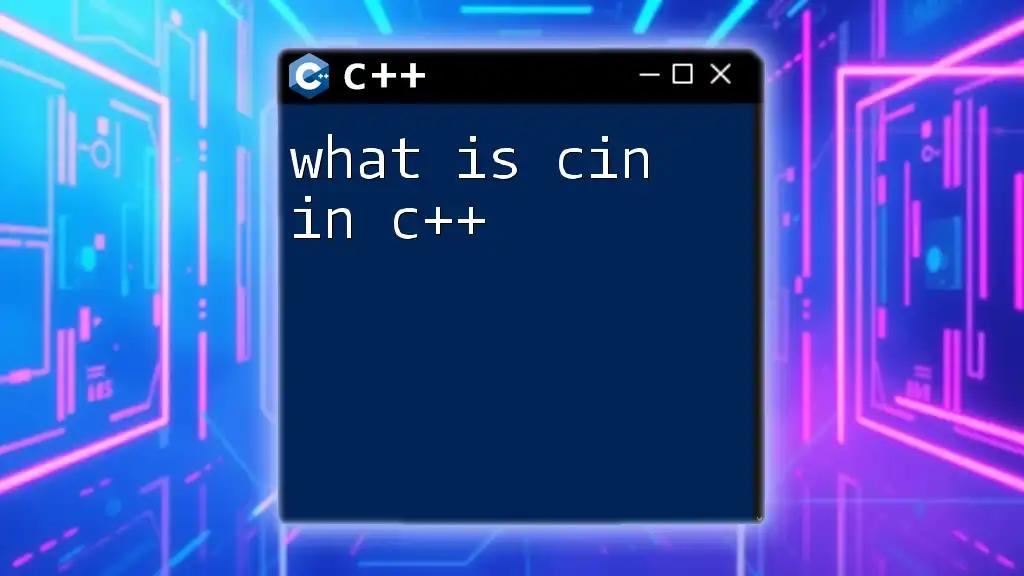
Boolean Expressions
A Boolean expression evaluates to either true or false. Understanding how Boolean expressions work is vital for control flow.
Common examples of Boolean expressions include:
- Basic comparisons (e.g., `a > b`, `x == y`)
- Compound expressions that utilize logical operators.
Here’s how you might define a boolean expression that checks eligibility based on two conditions:
bool isEligible = (age >= 18) && (citizenship == true);

Common Scenarios Using Boolean
Boolean values lend themselves to a variety of practical applications. One common use is as flags or state indicators in programs. For instance, you could create a toggle for user preferences:
bool darkMode = false; // Represents a user preference
darkMode = !darkMode; // Toggles the state of darkMode
This toggle could allow users to switch between light and dark themes in an application. User input validation can also heavily rely on Boolean checks, ensuring that data meets criteria before being processed.

Best Practices for Boolean Usage
To write clean and effective code, it is essential to follow best practices when using Boolean values:
-
Use meaningful names: Boolean variables should have names that reflect their purpose. Instead of generic names like `flag`, choose descriptive ones such as `isUserLoggedIn`.
-
Avoid negatives: When checking conditions, avoid using negatives as it can lead to confusion. Instead of checking `if (!isValid)`, prefer `if (isInvalid)`.
Good naming example:
- Good: `isUserLoggedIn`
- Bad: `notLoggedIn`

Errors and Pitfalls with Booleans
Despite their simplicity, there are common pitfalls when working with Boolean logic. One crucial mistake is confusing the assignment operator `=` with the equality operator `==`.
For example, the following line incorrectly assigns a value instead of comparing:
bool isEqual = (a == b); // Correct
bool isEqual = (a = b); // Incorrect; leads to assignment
This kind of mistake can lead to unexpected behavior in your code. Additionally, using parentheses in complex Boolean expressions is essential for ensuring clarity of logic. This practice helps avoid logic errors that can arise from operator precedence rules.

Conclusion
In summary, understanding what is Boolean in C++ is critical for effective programming. Boolean values and the `bool` data type form the backbone of decision-making and control flow in your applications. By mastering Boolean logic, expressions, and best practices, you will heighten the quality and maintainability of your code. Embrace these concepts and practice regularly, as they are foundational skills for any aspiring C++ developer.
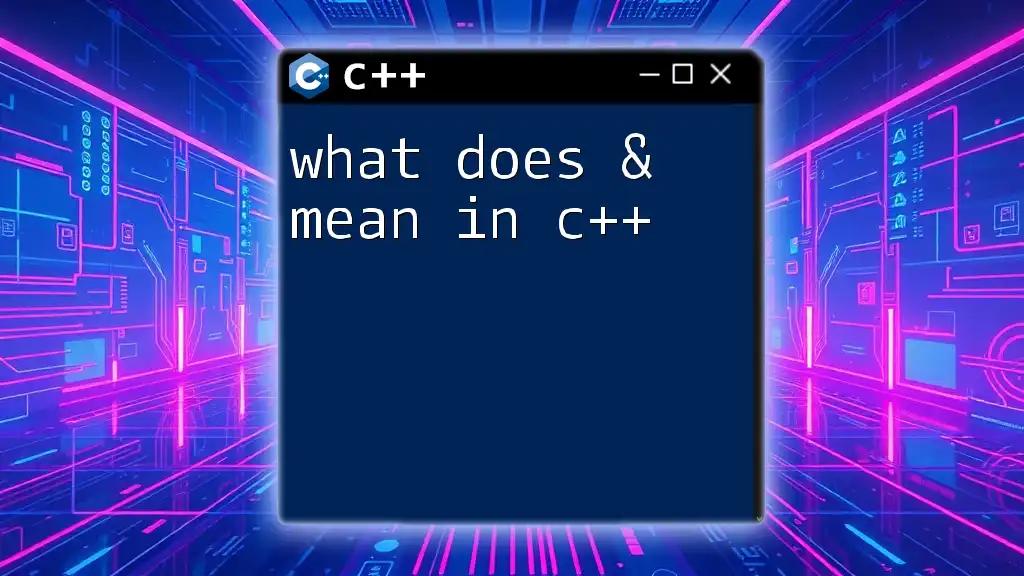
Additional Resources
For those looking to deepen their understanding, consider exploring more readings and exercises related to Boolean logic and data types in C++. Each concept you master will empower your programming journey even further.