The `setw` function in C++ is used to set the width of the next input/output field, allowing for formatted display of data in a console application.
Here's a code snippet that demonstrates its usage:
#include <iostream>
#include <iomanip> // Needed for setw
int main() {
std::cout << std::setw(10) << "Hello" << std::endl;
std::cout << std::setw(10) << "World!" << std::endl;
return 0;
}
What is `setw`?
The `setw` function in C++ is part of the `<iomanip>` library and stands for "set width." It is used to control the width of the output when displaying data in the console. This function is particularly useful for formatting strings, integers, and floating-point numbers, making output more readable and structured, especially when dealing with tabular data or aligned text.
Syntax of `setw`
The syntax for using `setw` is simple:
std::setw(int width)
- Width: This parameter specifies the minimum number of characters to be written to the output stream. If the data to be printed is shorter than the specified width, the output will be padded with spaces by default (or other specified characters).
How to Use `setw`
To use `setw`, you must first include the necessary header file:
#include <iomanip>
Basic Example of `setw`
Let’s start with a simple example to illustrate how `setw` works:
#include <iostream>
#include <iomanip>
int main() {
std::cout << std::setw(10) << "Hello" << std::endl;
std::cout << std::setw(10) << "World" << std::endl;
return 0;
}
Explanation: In this code, both "Hello" and "World" are output with a minimum width of 10 characters. As a result, if the string is shorter than 10 characters, spaces are added before the text to ensure right alignment, thereby improving readability.
Using `setw` with Different Data Types
Integers
You can also use `setw` for integer values, which helps in aligning them in a structured format. Consider the following example:
std::cout << std::setw(5) << 42 << std::endl;
This line will right-align the integer 42 within a width of 5 spaces, resulting in an output like this:
42
Floating-point Numbers
Formatting floating-point numbers is another common use of `setw`. Here's an example:
std::cout << std::setw(10) << std::fixed << std::setprecision(2) << 3.14159 << std::endl;
In this case, `std::fixed` ensures the number is represented in fixed-point notation, and `std::setprecision(2)` limits the output to two decimal places. The total width thus becomes 10 characters, providing consistent formatting for floating-point numbers.
Strings
To see how `setw` interacts with strings of varying lengths, consider the following example:
std::cout << std::setw(10) << "Apple" << std::setw(10) << "Banana" << std::setw(10) << "Cherry" << std::endl;
In this case, each fruit name will occupy exactly 10 spaces, regardless of its actual length, leading to a neat, column-aligned output.
Combining `setw` with Other Formatting Functions
Using `setfill`
The `setfill` function can be combined with `setw` to change the padding character. For example:
std::cout << std::setfill('-') << std::setw(10) << "Hi!" << std::endl;
Explanation: This line will replace the default spaces with hyphens, resulting in an output like:
-------Hi!
This feature can significantly enhance the visual organization of outputs.
Using `left`, `right`, and `internal`
C++ provides alignment manipulators such as `left`, `right`, and `internal` that can be useful alongside `setw`.
Left Alignment
You can use `left` to format output with left alignment:
std::cout << std::left << std::setw(10) << "Left" << std::endl;
In this case, "Left" will be positioned at the start of the field, and the remaining space will be padded with spaces to the right.
Right Alignment
By default, output is right-aligned, which can be explicitly shown with:
std::cout << std::right << std::setw(10) << "Right" << std::endl;
Here, "Right" will occupy the last spaces in the field.
Internal Alignment
While less commonly used, `internal` can be effective for numbers that include both signs and values:
std::cout << std::internal << std::setw(10) << 42 << std::endl;
This constructs the output spacing such that the sign of the number remains aligned, providing a unique formatting style.
Common Uses of `setw` in Practice
`setw` is particularly useful in creating formatted outputs for reports, tables, or user interfaces. It allows for an organized presentation of data, making it easier for users to read and analyze.
For example, when displaying results from a calculation, structured output can greatly enhance understanding:
std::cout << std::setw(10) << "Name"
<< std::setw(10) << "Score" << std::endl;
std::cout << std::setw(10) << "Alice"
<< std::setw(10) << 95 << std::endl;
std::cout << std::setw(10) << "Bob"
<< std::setw(10) << 85 << std::endl;
Output:
Name Score
Alice 95
Bob 85
This visibility is essential for any application that relies on displaying multiple pieces of information clearly.
Potential Pitfalls and Best Practices
While `setw` is highly useful, there are limitations. It only applies to the immediate next output operation, so if you want the same width setting for multiple outputs, you must call `setw` each time.
To maximize effectiveness:
- Always include `<iomanip>` to leverage the full set of formatting functions.
- Combine `setw` with alignment manipulators for more organized displays.
- Remember that changing the alignment or filling after using `setw` will apply to future output, so reset alignments as needed for consistency.
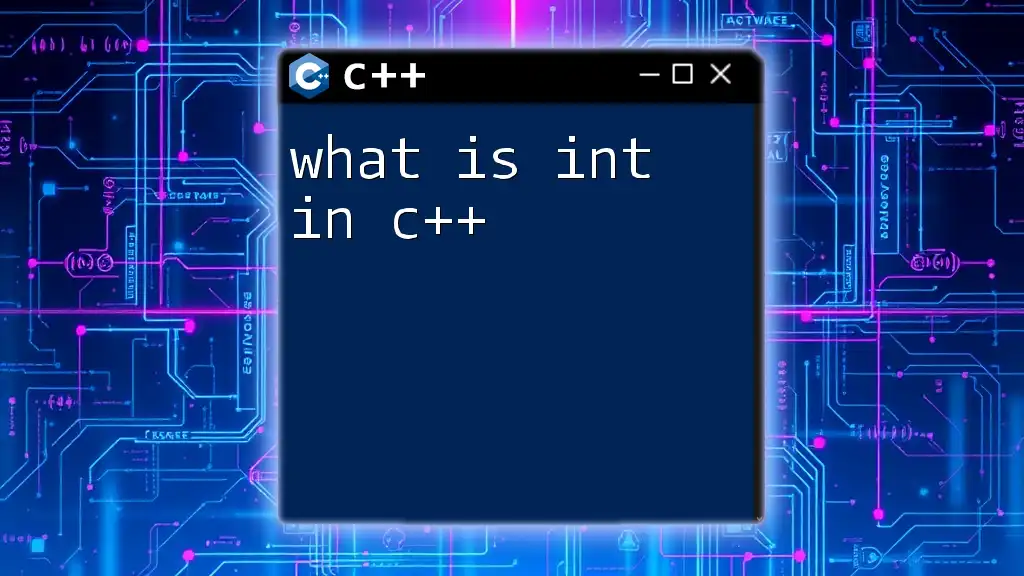
Conclusion
In summary, understanding what is `setw` in C++ opens up powerful formatting capabilities for console output. By utilizing `setw`, alongside other manipulators like `setfill` and alignment options, developers can create clear and organized displays of data. This not only improves readability but also enhances user experience, making the program outputs professional and effective.