Inline C++ refers to a method of defining short functions directly in the code, allowing the compiler to insert the function code at each call site to improve performance by reducing function call overhead. Here's a simple example:
inline int square(int x) {
return x * x;
}
What is Inline in C++?
Definition
In C++, the term inline refers to a function that is expanded in line when it is called, rather than being invoked through the usual function call mechanism. The concept was introduced to enhance performance by reducing the overhead of additional function calls. Unlike regular functions, which require stack manipulation and jumping to the function code during each invocation, inline functions can be directly inserted ("inlined") into the calling code, effectively eliminating the function call overhead.
Purpose of Inline Functions
Inline functions serve a significant purpose in C++. They aim to improve performance by minimizing the function call overhead. When small functions are called repeatedly within a program, the repeated overhead can add up, making the code less efficient. The core benefits of using inline functions include:
- Faster execution: By embedding the function code directly, the program can execute it faster since it avoids the overhead of function calls.
- Reduced function call overhead: Inline functions help in reducing the time taken to transfer control to a function and back.
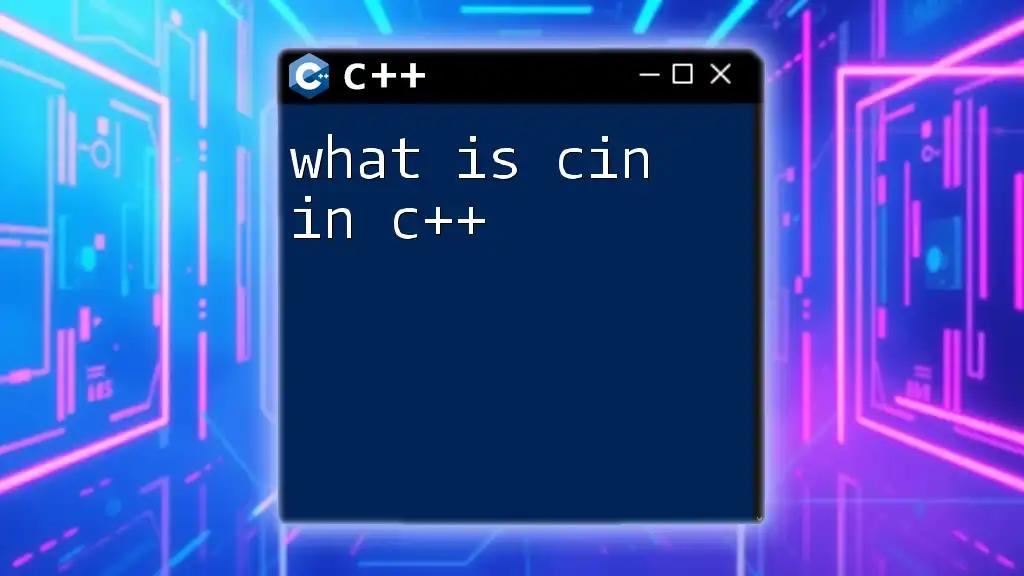
How Inline Functions Work
Inline Function Syntax
The syntax for defining an inline function is straightforward. Here’s how you can declare it in C++:
inline int add(int a, int b) {
return a + b;
}
In this example, the `inline` keyword suggests to the compiler that it should attempt to replace any call to `add` with the actual function body. It is important to note, however, that the compiler is not obliged to adhere to this request.
Compilation and Inlining Process
When the C++ compiler compiles a program that contains inline functions, it examines each inline function to decide if it can be inlined. If inlining is appropriate, the compiler replaces the function call with the function’s code, leading to a more efficient execution.
Compiler Discretion
The inline suggestion provided by the programmer is just that—a suggestion. The compiler has the discretion to ignore it based on various factors. For instance:
- If the function is too complex or has a significant amount of instructions, the compiler may choose not to inline it to avoid code bloat.
- Similarly, if the function’s body exceeds a predefined threshold in terms of size, it may also be left as a traditional function call.
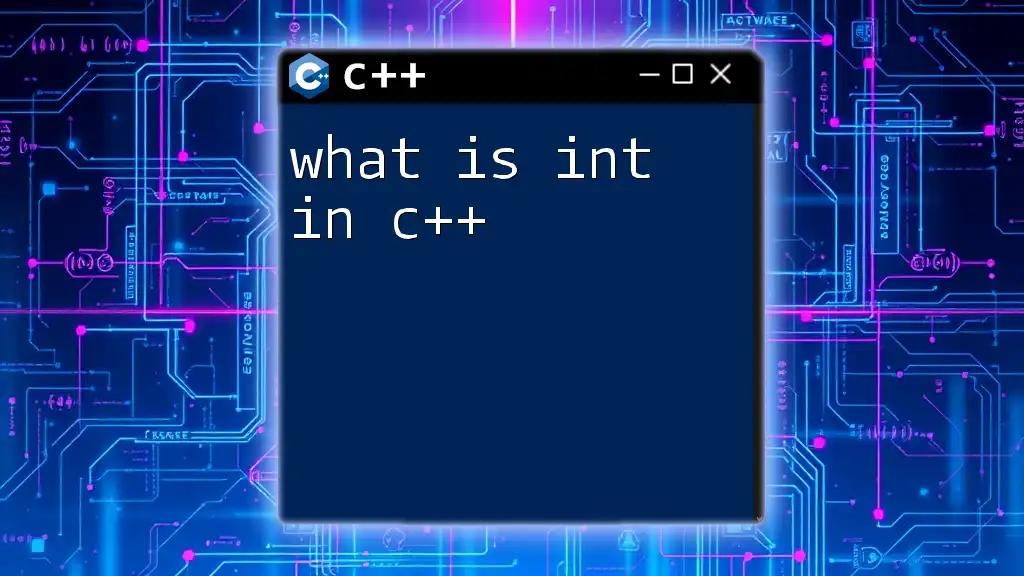
Advantages of Using Inline Functions
Performance Improvement
One of the most notable advantages of inline functions is the potential for performance improvement. For example, consider a scenario where a simple function is called multiple times in a loop. Normally, each function call might require several CPU cycles, which can be avoided when using inline functions.
Here is a simple comparison:
#include <iostream>
inline int square(int x) {
return x * x;
}
int main() {
int sum = 0;
for (int i = 1; i <= 1000; i++) {
sum += square(i); // Inline function call
}
std::cout << "Sum of squares: " << sum << std::endl;
return 0;
}
In the above code, the inline function `square()` avoids the function call overhead during the loop execution, potentially speeding up the program.
Code Readability
Using inline functions can also significantly improve code readability. By breaking larger functions or complex logic into smaller, manageable functions that are declared inline, code becomes cleaner and easier to read. The abstraction allows programmers to understand their code better because they can see the function calls clearly without delving into large blocks of logic.

Disadvantages of Inline Functions
Code Bloat
While inline functions can enhance performance, they can also lead to code bloat. When a function is inlined, its code is copied into every place in the source where the function is called. If an inline function is called from multiple places, this can substantially increase the size of the binary code. Programs with excessive inline expansion may become unwieldy, consuming more memory than intended.
Debugging Challenges
Another potential disadvantage of inline functions comes into play during the debugging process. When stepping through the code in a debugger, it can become more complicated because the function call does not behave like a typical function call. Since the function’s body is inserted directly into the calling code, it may confuse developers trying to trace execution flow.

Best Practices for Using Inline Functions
When to Use Inline Functions
Inline functions are best utilized in specific scenarios:
- When defining small utility functions that are frequently used.
- For performance-critical sections of code where the execution speed is paramount.
- When the function is simple, concise, and easily digestible to improve clarity.
When to Avoid Inline Functions
Conversely, inline functions should be avoided in the following situations:
- When dealing with larger functions that may cause significant code bloat.
- For functions that contain complex logic where performance gains may be negligible.

Real-world Examples
Example 1: Simple Inline Function
Consider a basic inline function that calculates the maximum of two integers:
inline int max(int x, int y) {
return (x > y) ? x : y;
}
This inline function is straightforward, and its usage is effective. By inlining this function, you're reducing the overhead of calling it multiple times across your code.
Example 2: Inline Function in a Class
Inline functions can also be used within class definitions. Here’s a demonstration:
class Rectangle {
private:
int width, height;
public:
Rectangle(int w, int h) : width(w), height(h) {}
inline int area() { return width * height; }
};
In this example, `area()` is an inline member function of the `Rectangle` class. This approach is especially useful for classes, where member functions are often small, and frequent calls can be optimized through inlining.

Conclusion
Inline functions in C++ are powerful tools designed to enhance performance while maintaining a level of code readability. Understanding what inline C++ functions are and when to use or avoid them can greatly influence the design and efficiency of your code. It’s essential to capitalize on the benefits of inline functions while being mindful of their potential drawbacks. With this knowledge, you are better equipped to harness the strengths of inline functions in your programming endeavors.
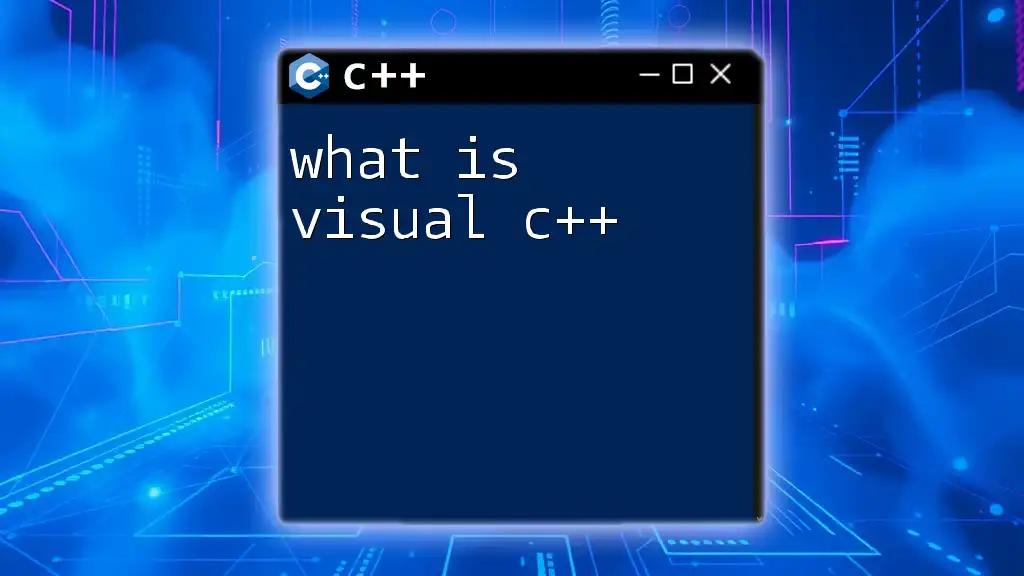
FAQs About Inline Functions
Common Questions
-
What happens if an inline function is too complex? The compiler may choose not to inline it, ultimately defaulting to a regular function call.
-
Can inline functions be recursive? While technically permissible, recursive inline functions should be used cautiously because they may result in infinite inlining, which the compiler generally avoids.
-
Are inline functions always faster than regular functions? Not necessarily, as the effectiveness of inline functions depends on various factors, including the function size and complexity, as well as how many times the function is called.
Final Thoughts
By exploring inline functions, we've opened the door to better performance in C++. Engaging with this topic can deepen your understanding of function call overheads and encourage more efficient coding practices. If you have experiences or questions regarding inline functions, I invite you to share your insights or seek clarification.