An inline function in C++ is a function whose definition is small enough that the compiler replaces the function call with the actual code of the function, potentially improving performance by eliminating the overhead of a function call.
Here’s a code snippet demonstrating an inline function:
inline int add(int a, int b) {
return a + b;
}
What is an Inline Function in C++
An inline function in C++ is a special type of function that enables the compiler to replace the function call with the function's body during the compilation process. Instead of incurring the overhead of a traditional function call, inline functions can provide more efficient code execution by reducing the number of jumps through the program’s control flow.
What Are Inline Functions in C++?
Inline functions possess specific characteristics that set them apart from regular functions. They are typically defined within a class or before the `main` function in the source file. The main purpose of inline functions is to optimize performance by minimizing the function call overhead, which can be particularly beneficial in cases where the function is called multiple times.
However, the use of inline functions comes with pros and cons:
-
Benefits:
- Speed Optimization: Inline functions can lead to faster execution because the call overhead is eliminated.
- Reduction of Function Call Overhead: Inline functions allow your program to save time that would normally be spent in making function calls.
-
Drawbacks:
- Increased Code Size: Expanding the inline function's code at each call site can result in larger binaries.
- Complexity in Debugging Inline Functions: Debugging can be more challenging since inline functions blur the lines of function calls in stack traces.
What is Inline in C++?
The keyword `inline` is what allows you to declare a function as inline. When you precede a function’s declaration with the `inline` keyword, you suggest to the compiler that it should attempt to integrate that function’s code into the caller's code instead of invoking the function traditionally. However, the compiler is not obligated to honor this request.
It's also vital to note that inline functions are typically suitable for small, frequently called functions. If a function is a complex one or contains loops and large amounts of code, compilers usually ignore the `inline` suggestion.
Advantages of Using Inline Functions
Performance Improvements
Inline functions can offer significant speed enhancements, especially in performance-critical applications. For example, if a function is called numerous times throughout a program, replacing these calls with inline code can considerably reduce the overall execution time. Real-world benchmarks often illustrate that smaller and more efficient functions can lead to noticeable performance gains.
Code Readability
Another advantage of inline functions is that they contribute to cleaner and more maintainable code. An inline function helps encapsulate a simple operation and presents it distinctly, making code easier to read and understand. Consider the following comparison:
int square(int x) {
return x * x;
}
Versus calling the function without an inline definition in a program. The clarity of intentions is apparent when using an inline function, allowing programmers to focus on logic rather than function mechanics.
Inline Function Examples in C++
Basic Example
Here’s a simple example of an inline function:
inline int square(int x) {
return x * x;
}
In this example, every time `square(x)` is called, the compiler will replace this call with `x * x`, effectively streamlining the execution. The direct replacement minimizes unnecessary overhead, particularly advantageous when the operation is performed frequently.
Inline Function in a Class
Inline functions are also commonly used within classes. Here's how an inline member function can be applied:
class Rectangle {
public:
inline int area() {
return width * height;
}
private:
int width;
int height;
};
This `area()` function calculates the area of the rectangle and can benefit from being inline—ensuring that when the `area()` method is called, its computation takes place immediately, rather than invoking a standard function call.
Guidelines for Using Inline Functions
When to Use Inline Functions
Utilizing inline functions is most beneficial under specific conditions:
- Small Functions: Opt for inline if the function body is minimal.
- Frequently Called Functions: Inline is ideal when a function is invoked numerous times throughout the program.
- Performance Critical Sections: In performance-intensive applications, inline functions might make a considerable difference.
When Not to Use Inline Functions
Avoid inline functions in the following situations:
- Complex Functions: Functions with extensive logic, loops, or recursion should remain as standard functions—this helps avoid code bloat.
- Debugging Scenarios: When debugging, it may be better to keep functions unlined as it simplifies tracking down issues.
Best Practices for Inline Functions
Keep it Simple
The primary principle for inline functions is simplicity. Aim to write concise functions that accomplish specific tasks rather than complex operations that span multiple lines.
Use Sparingly
While inline functions can be advantageous, a general rule is to use them sparingly to avoid code bloat and maintainability issues.
Profile Your Code
Before integrating inline functions across your codebase, it’s wise to profile your code to understand ifinline functions genuinely enhance performance. The objective should always be to strike a balance between optimization and code readability.
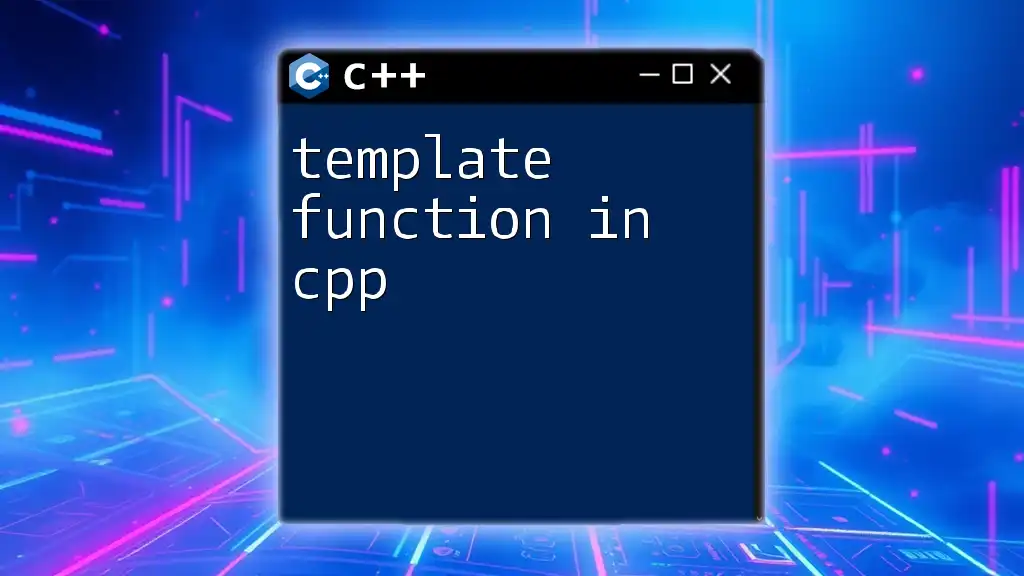
Conclusion
Inline functions in C++ serve as powerful tools for optimizing program performance while contributing to cleaner code. By avoiding unnecessary function call overhead, inline functions can lead to speed enhancements, especially in frequently called scenarios. However, it is vital to use this feature judiciously, keeping in mind the potential trade-offs such as increased code size and debugging complexity. As you delve deeper into C++, remember to experiment with inline functions within your projects to discover their impact on your programming experience.