A pointer in C++ is a variable that stores the memory address of another variable, enabling direct access and manipulation of that variable's data.
int var = 10; // Declare an integer variable
int* ptr = &var; // Declare a pointer and initialize it with the address of var
// Output the value of var using the pointer
cout << "Value of var: " << *ptr << endl; // Dereference the pointer to get the value
Understanding Pointers in C++
What Are Pointers in C++?
In C++, pointers are variables that store memory addresses. This concept is fundamental for efficient memory management and is crucial for creating dynamic data structures. Finding the right balance in using pointers effectively can lead to optimized performance and memory usage in your applications.
Key Terminology
Pointer: A pointer is essentially a variable that contains the address of another variable. This allows the program to manipulate data indirectly and efficiently.
Dereferencing: The dereferencing operator, denoted by `*`, is used to access the value at the address stored in a pointer. When you dereference a pointer, you are retrieving the value of the variable that it points to.
Address-of Operator: The address-of operator, represented by `&`, is used to obtain the memory address of a variable. When combined with pointers, this operator is crucial for initializing and manipulating pointers.
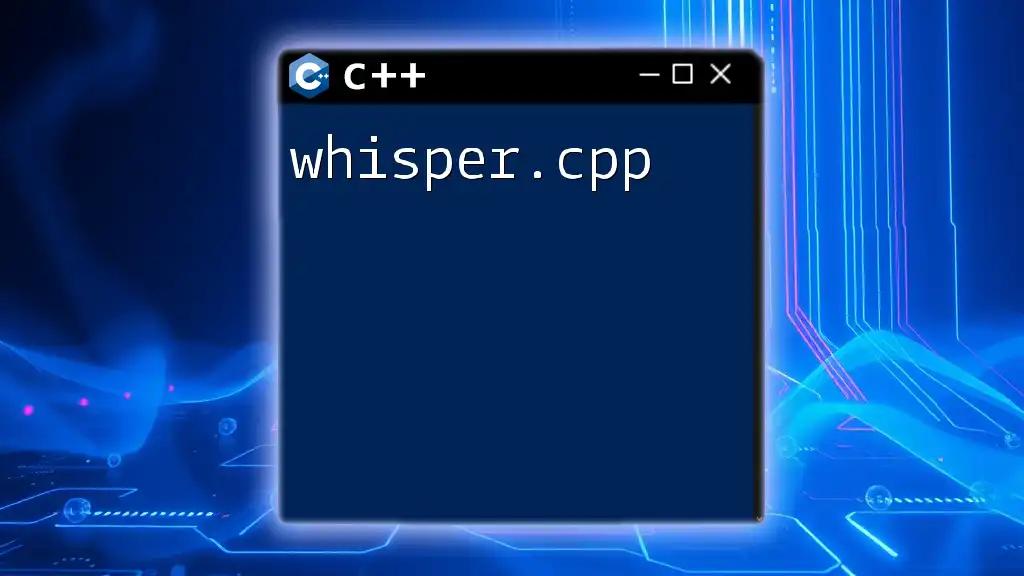
How to Declare and Initialize Pointers
Pointer Declaration
Declaring a pointer consists of specifying the data type it will point to, followed by an asterisk (`*`). For example:
int* ptr; // A pointer that will point to an integer
Pointer Initialization
To make a pointer useful, you must initialize it by storing the address of a variable. For instance:
int var = 10;
int* ptr = &var; // Pointer initialized to the address of var
This means that `ptr` now holds the memory address where `var` is stored, allowing `ptr` to access or modify `var` indirectly.

Accessing Values Using Pointers
Dereferencing a Pointer
When a pointer is initialized correctly, you can access the variable's value stored at its memory address by dereferencing it. For example:
int value = *ptr; // Dereferencing to get the value at ptr
In this case, `value` will now contain the value `10`, the current value of `var`.
Changing Values via Pointers
One of the powerful features of pointers is their ability to modify variables. By dereferencing a pointer, you can change the value of the variable it points to:
*ptr = 20; // Changing value of var to 20 via pointer
After this operation, the value of `var` will now be `20`, demonstrating how pointers allow for direct manipulation of data.
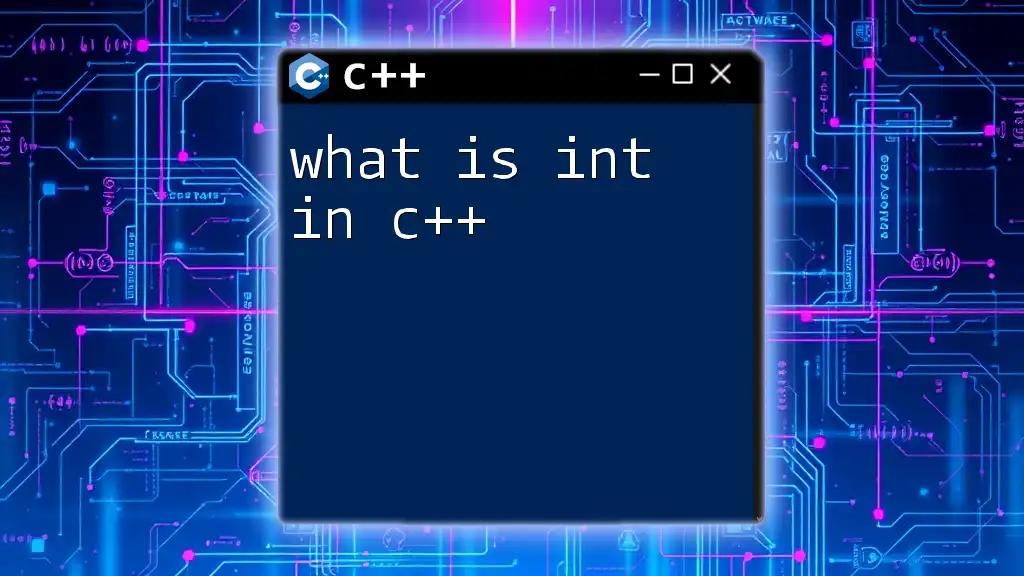
Pointer Types
Null Pointers
A null pointer is a pointer that does not point to any valid memory location. It is often initialized with the keyword `nullptr`, which denotes a pointer that is intentionally left unassigned. This is useful for error checking and can prevent bugs associated with dereferencing random memory locations.
Void Pointers
Void pointers, or generic pointers, can point to any data type. A void pointer does not have a specific data type, making it more flexible in certain scenarios:
void* ptrVoid;
However, it requires explicit typecasting before dereferencing because the compiler does not know what type of data it points to.
Pointers to Pointers
A pointer to a pointer is a more advanced concept where you have a pointer that stores the address of another pointer. This allows for multi-level data structures, such as when dynamically allocating a 2D array:
int** ptrToPtr = &ptr; // Pointer that stores the address of another pointer
This feature is particularly useful in complex data structures and dynamic memory management.
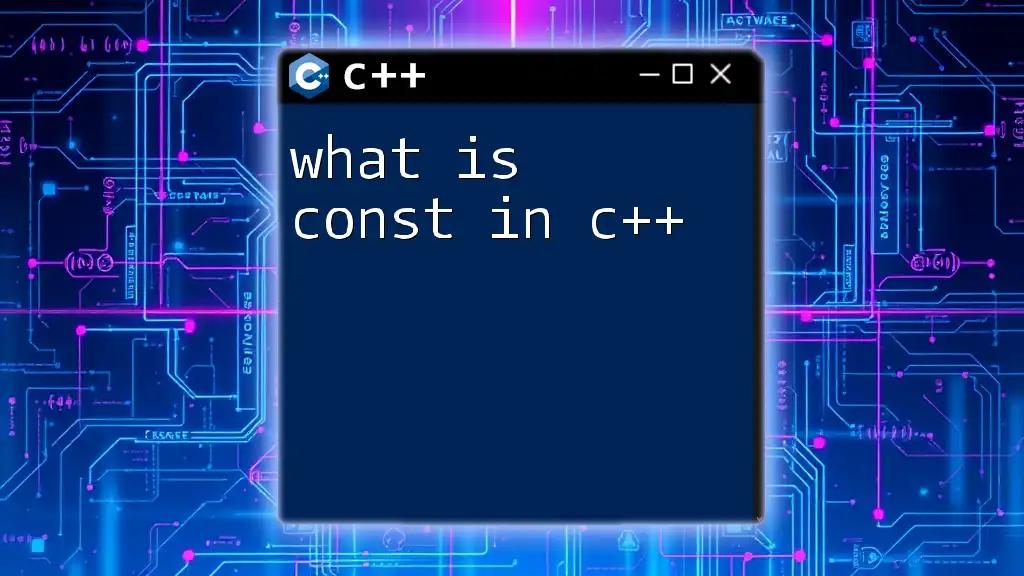
Pointer Arithmetic
Understanding Pointer Arithmetic
Pointer arithmetic involves adding or subtracting integers to/from pointers. This arithmetic allows you to navigate through arrays easily. For example:
ptr++; // Moving to the next memory address
When pointers point to array elements, incrementing the pointer will point it to the next element in the array based on its data type size.
Real-World Example
Consider an array of integers and how pointers can be used to navigate through it:
int arr[3] = {1, 2, 3};
int* ptr = arr;
for (int i = 0; i < 3; i++) {
std::cout << *(ptr + i) << std::endl; // Accessing array elements using pointer
}
In this example, `ptr` starts at the beginning of the array, and the loop iteratively dereferences successive elements, demonstrating how pointers facilitate streamlined access to array contents.

Common Mistakes with Pointers
Dangling Pointers
A dangling pointer arises when a pointer continues to point to a memory location that has been deallocated. This situation can occur when you delete a pointer but do not reset it to `nullptr`, leading to potential undefined behavior if you try to dereference it afterward.
Memory Leaks
Improper use of pointers can result in memory leaks, which occur when memory allocated dynamically (e.g., using the `new` keyword) is not properly deallocated (using `delete`). Always ensure that every `new` has a corresponding `delete` to free memory when it is no longer needed.
Improper Dereferencing
Dereferencing uninitialized or null pointers leads to program crashes or erratic behavior. Always ensure pointers are initialized before dereferencing them.
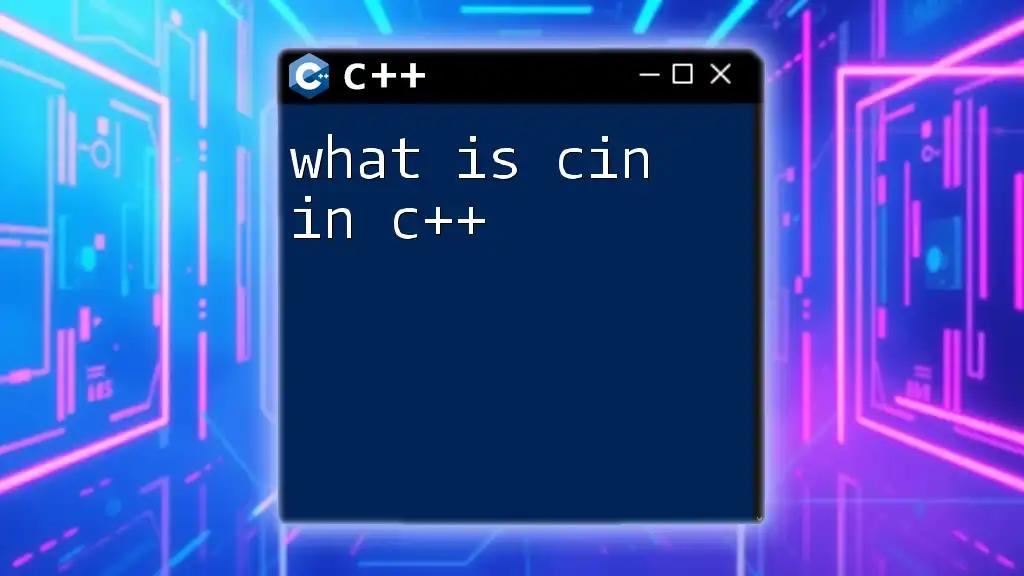
Conclusion
Pointers are an indispensable feature of C++ that provide flexibility and power in memory management. Understanding how to use them correctly can greatly enhance your programming capabilities. By carefully managing pointers and employing best practices, you can avoid common pitfalls and make the most of your C++ programming experience.

Additional Resources
For those interested in mastering pointers in C++, consider exploring comprehensive textbooks, online tutorials, and interactive coding platforms dedicated to enhancing your C++ skills.