In C++, a literal is a fixed value that is directly represented in the source code, such as numbers, characters, or strings, without requiring any computation.
int num = 5; // Integer literal
char letter = 'A'; // Character literal
const char* greeting = "Hello, World!"; // String literal
What is a Literal in C++?
A literal in C++ is essentially a fixed value that is represented directly in the code. These values can be numbers, characters, strings, or boolean values, which allow programmers to express constants without needing to declare variables. For example, in the code `int x = 10;`, the number `10` is an integer literal. Using literals correctly is crucial for avoiding errors and improving code readability.
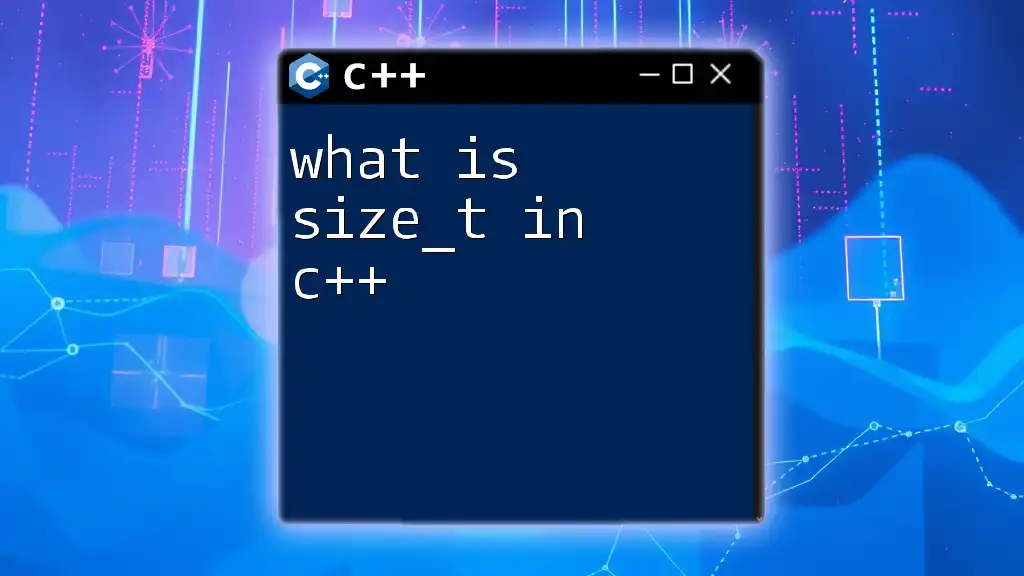
Types of Literals in C++
C++ offers various types of literals, each with its characteristics that serve different purposes. Understanding these types is essential for effectively utilizing them in your programs.
Integer Literals
Integer literals represent whole numbers and can appear in different formats. They are defined as follows:
-
Decimal: The default format. For example, `42` is a decimal integer literal.
int decimalLiteral = 42;
-
Octal: Represented by a leading `0`. For example, `052` represents the octal number 52, which is 42 in decimal.
int octalLiteral = 052; // 42 in decimal
-
Hexadecimal: Denoted by a prefix `0x`. For example, `0x2A` is a hexadecimal literal for 42.
int hexLiteral = 0x2A; // 42 in decimal
-
Binary: Introduced in C++14 with a prefix of `0b`. For example, `0b101010` represents the binary value for 42.
int binaryLiteral = 0b101010; // 42 in decimal
Floating-Point Literals
Floating-point literals represent real numbers and can store decimal values. These literals can be expressed in either standard or scientific notation.
-
Standard Notation: For example, `3.14` or `-0.0012`.
float standardLiteral = 3.14f; // The 'f' indicates a float type
-
Scientific Notation: Represented by the letter `e` to signify exponent. For example, `2.5e3` equals `2500.0`.
double scientificLiteral = 2.5e3; // Represents 2500.0
Character Literals
Character literals represent single characters. They are enclosed in single quotes, like `'A'` or `'3'`. Special characters can be included using escape sequences.
-
Example:
char charLiteral = 'a'; char escapeChar = '\n'; // New line character
String Literals
String literals represent a sequence of characters enclosed in double quotes. In C++, they are of type `const char*`.
-
Standard String Literal: This is a regular string of characters.
const char* stringLiteral = "Hello, World!";
-
Raw String Literals: Enclosed in `R` followed by a pair of parentheses. This format is useful for strings that contain backslashes or quotes.
const char* rawStringLiteral = R"(C:\Users\Name\Documents\)";
Boolean Literals
Boolean literals represent the two possible truth values, `true` and `false`. These literals are fundamental for control structures, such as if statements.
-
Example:
bool isTrue = true; bool isFalse = false;
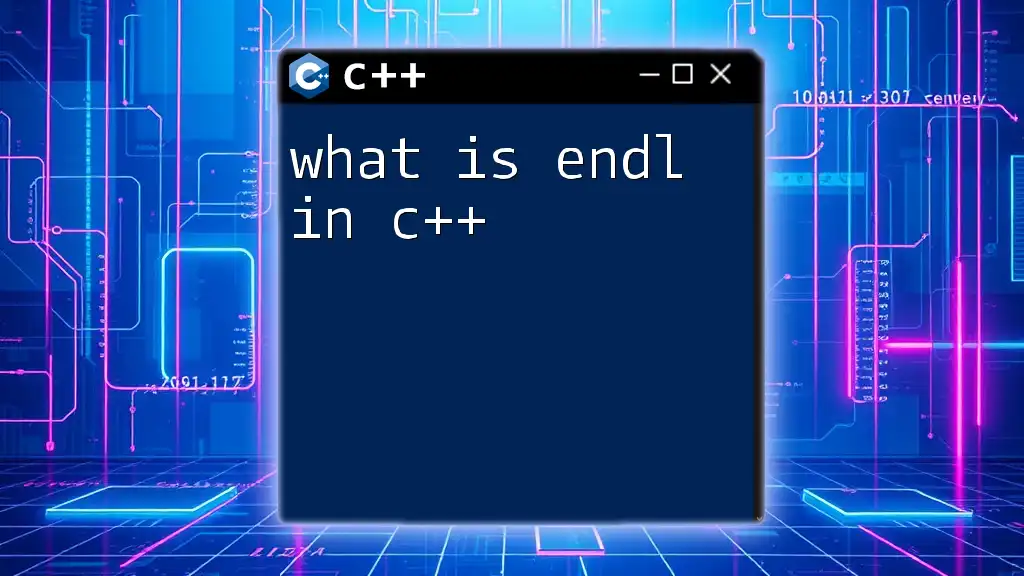
User-Defined Literals
C++ allows programmers to define their own literals to enhance code readability. User-defined literals can be created based on existing types, providing a way to make the code express its intent more clearly.
-
Example: Here’s how to create a user-defined literal that represents meters.
long double operator"" _m(long double m) { return m; // User-defined literal that remains in meters } long double distance = 5.0_m; // Represents 5 meters
By defining a user-defined literal, you enhance code readability and conceptual clarity, making the code more intuitive.
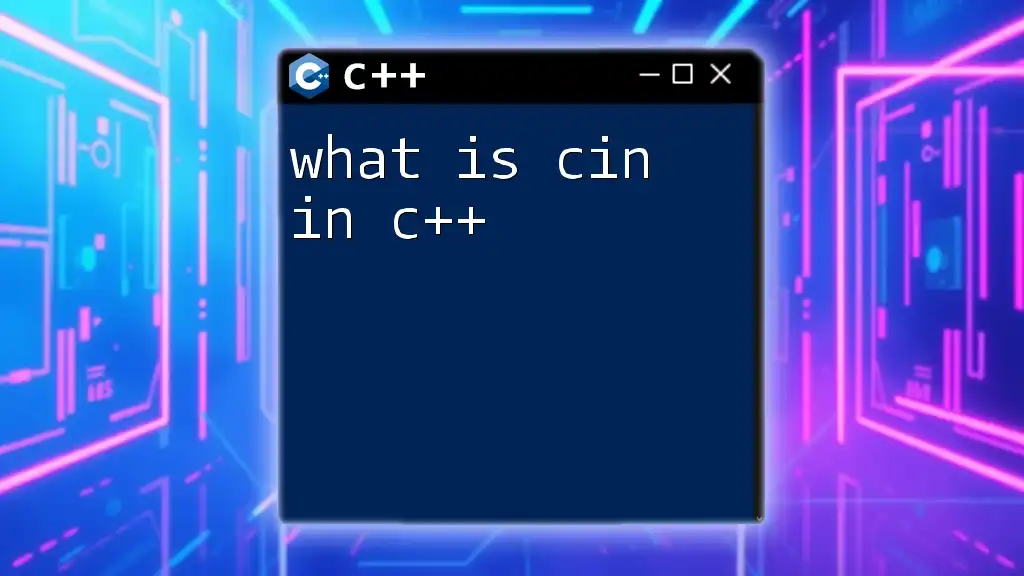
Advantages of Using Literals
Using literals comes with several advantages:
-
Code Readability: They provide clarity about the intent of values within the code. Seeing `42` is much more understandable than seeing `x` without context.
-
Simplicity: Literals are easy to use and straightforward since they don't require a variable declaration.
-
Type Safety: Literals are evaluated at compile-time, which minimizes the possibility of runtime errors.
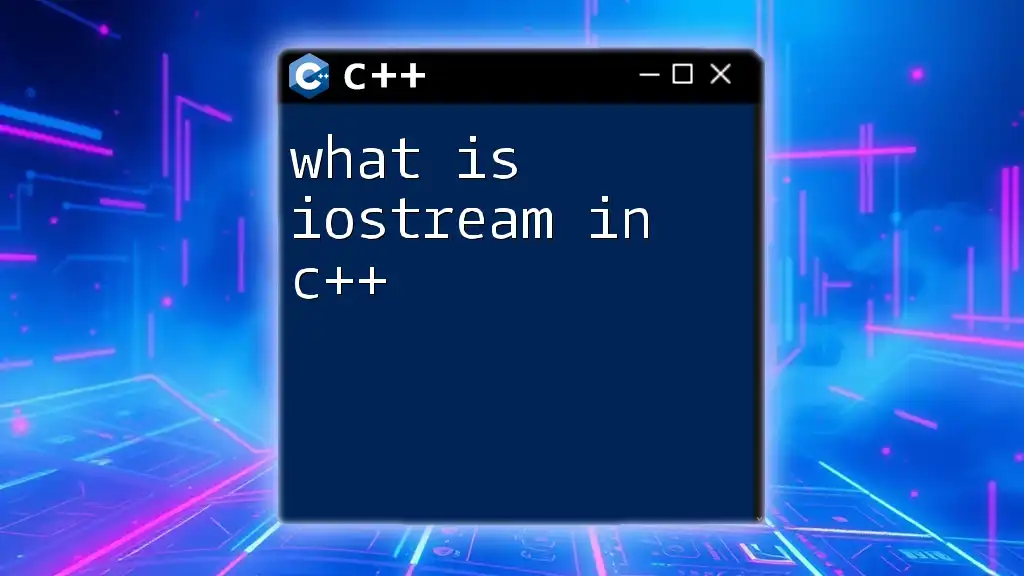
Common Pitfalls and Best Practices
While literals are simple to use, there are some common pitfalls you should be aware of:
-
Type Mismatch: Ensure the literal type matches the expected variable type. For example, assigning `3.14` directly to an `int` will result in data loss.
int x = 3.14; // Warning: implicit conversion from double to int
-
Using Raw String Literals: Raw string literals can be tricky when they inadvertently include special characters. Always double-check their content.
Best Practices
-
Always specify the type explicitly when necessary, particularly with floating-point literals, using `f` for floats and `l` for long doubles.
-
Use user-defined literals to enhance clarity, especially for units of measurement or custom data types.
-
Avoid magic numbers in your projects; use named constants or macros to give context to literals.
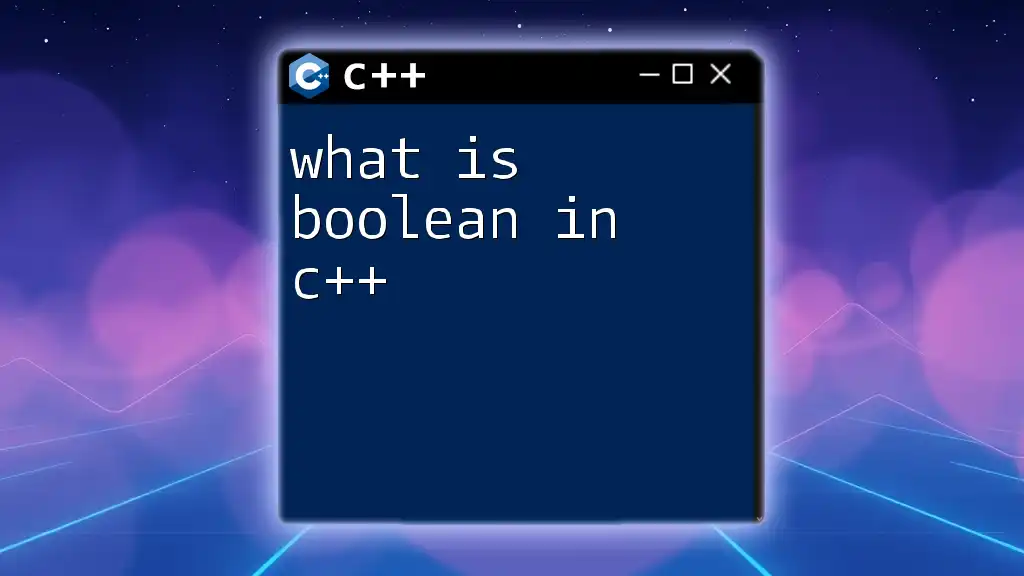
Conclusion
Understanding what is a literal in C++ is fundamental for any C++ developer. Literals form the building blocks of your code, allowing you to express values directly. Mastering their use will not only enhance your programming skills but also improve the quality of your code. Experiment with different types of literals and leverage user-defined literals to create clean and understandable code.