To set up Visual Studio Code for C++, you need to install the C++ extension, configure the compiler path, and create a `tasks.json` file for build tasks.
// Example tasks.json configuration in .vscode folder
{
"version": "2.0.0",
"tasks": [
{
"label": "build",
"type": "shell",
"command": "g++",
"args": [
"-g",
"${file}",
"-o",
"${fileDirname}/${fileBasenameNoExtension}.exe"
],
"group": {
"kind": "build",
"isDefault": true
},
"problemMatcher": ["$gcc"],
"detail": "Generated task by C/C++ extension."
}
]
}
Installing Visual Studio Code
Downloading VS Code
To get started with how to setup visual code for C++, you first need to download Visual Studio Code. Navigate to the [official Visual Studio Code website](https://code.visualstudio.com/). Here, you’ll find options for different operating systems. Ensure you select the correct version for your OS—Windows, macOS, or Linux.
Installation Steps
Windows Installation Process
Once you’ve downloaded the installer:
- Run the executable file.
- Follow the installation prompts.
- Select options that suit your preferences, such as adding VS Code to your PATH.
macOS Installation Process
On macOS, downloading is straightforward:
- Open the downloaded `.dmg` file.
- Drag Visual Studio Code into the Applications folder.
- Launch the application from your Applications menu.
Linux Installation Process
For Linux users, there are various ways to install VS Code depending on your distribution:
- Debian/Ubuntu: You can download the `.deb` package or use snap:
sudo snap install --classic code
- Fedora: Install using the `rpm` package or through dnf:
sudo dnf install code
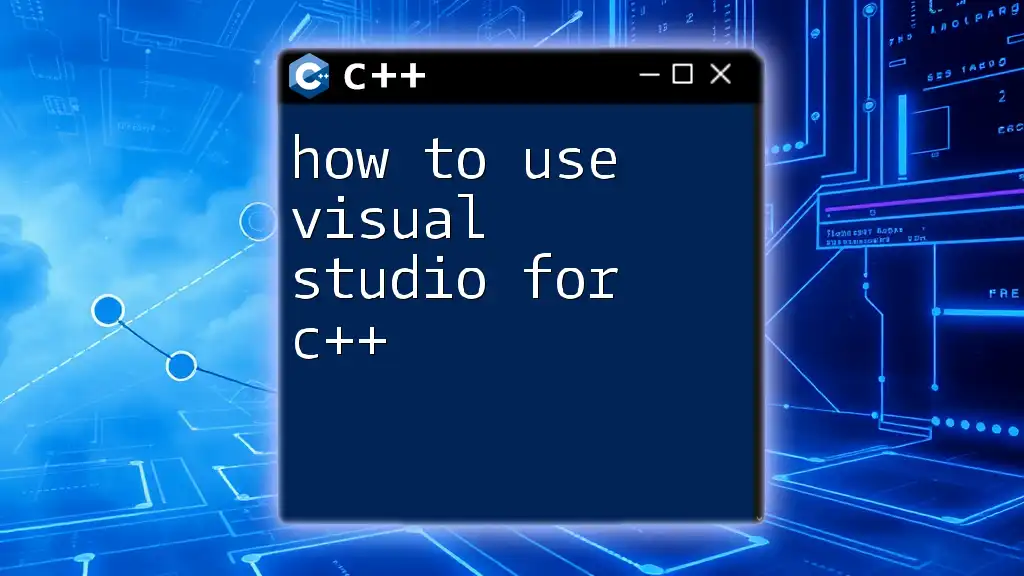
Setting Up C++ Compiler
Installing MinGW on Windows
For Windows users, MinGW (Minimalist GNU for Windows) is an excellent choice.
- Download the MinGW installer from [MinGW’s official website](http://www.mingw.org/).
- Run the installer and select the C++ Compiler option during the installation process.
- After installation, you'll add MinGW to your system's PATH:
- Right-click on Computer and select Properties, then Advanced system settings.
- Click on Environment Variables, and find the Path variable in the system variables.
- Add the path to your MinGW `bin` directory, typically `C:\MinGW\bin`.
Verifying the Installation
Open the Command Prompt and run:
g++ --version
This command should return the installed version of g++.
Installing g++ on macOS
For macOS users, the easiest way to install the C++ compiler is through Homebrew:
- If Homebrew isn't installed, open your Terminal and paste:
/bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)"
- Once Homebrew is installed, run:
brew install gcc
Verifying Installation
To confirm that the g++ compiler is correctly installed, run:
g++ --version
Installing g++ on Linux
For Linux, installing g++ depends on your package manager.
-
Debian/Ubuntu: Use:
sudo apt update sudo apt install g++
-
Fedora: Run:
sudo dnf install gcc-c++
Confirming the Installation
Check your g++ installation with:
g++ --version
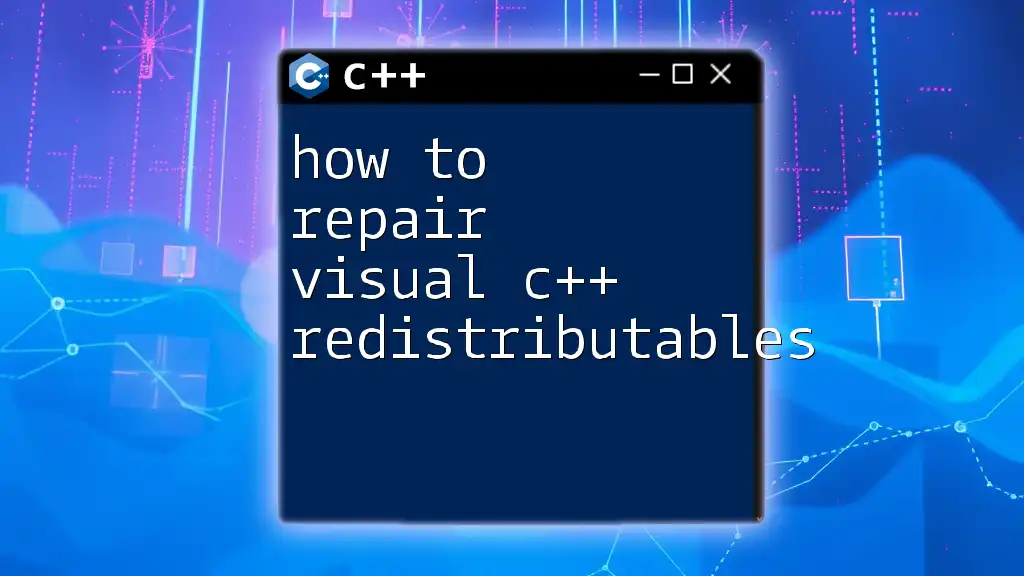
Configuring VS Code for C++
Installing Essential Extensions
To make your experience smoother while learning how to setup visual code for C++, consider installing a couple of essential extensions:
- C/C++ Extension by Microsoft: This extension provides debugging support and code suggestions, significantly enhancing productivity.
To install:
- Open VS Code.
- Go to Extensions (or press `Ctrl + Shift + X`).
- Search for C/C++ and click Install.
- Code Runner Extension: This allows you to run your code snippets with just one click or a simple shortcut.
Setting Up Your Development Environment
Once you have your extensions, it’s time to create a new workspace:
- Open VS Code and create a new folder where you can keep your C++ projects.
- Inside your folder, create a new file, for instance, `hello.cpp`.
Configuring Settings
You can further customize VS Code by editing the `settings.json` file:
- Go to File > Preferences > Settings.
- Click the `{}` icon at the top right to edit them directly.
- Configure compiler paths and other preferences that suit your workflow.
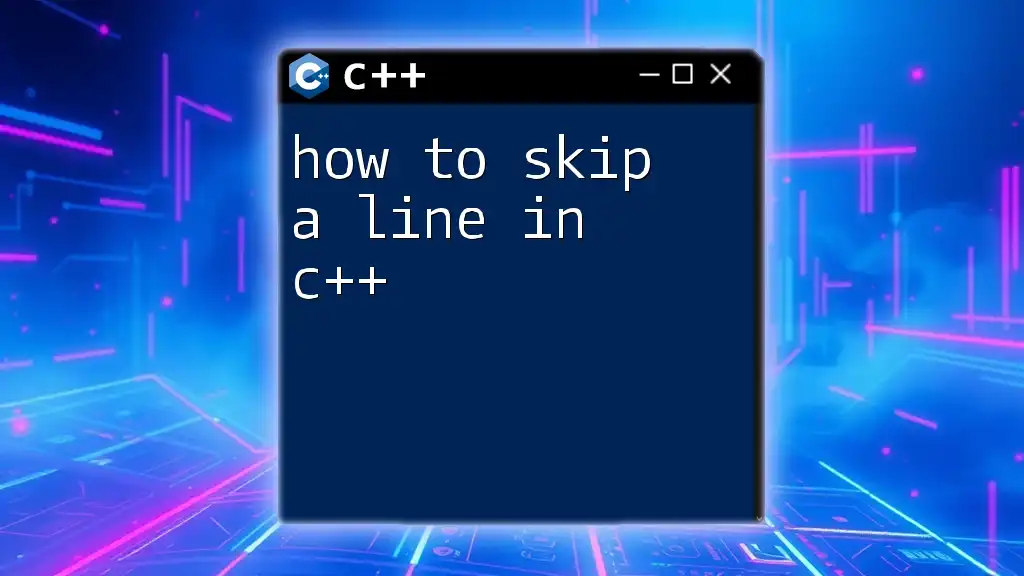
Writing Your First C++ Program
Creating a Simple "Hello World" Program
Let’s dive into your first C++ program. In the file `hello.cpp`, write the following code to print "Hello, World!" to the console:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
Compiling and Running the Program
After writing your code, it's time to compile and run it.
-
Using the Terminal in VS Code:
- Open the terminal within VS Code (`Ctrl + ` or View > Terminal).
- Compile your code using:
g++ hello.cpp -o hello
- Then run it:
./hello
-
Using Code Runner: If you’ve installed the Code Runner extension, you can simply right-click in the editor and select Run Code.
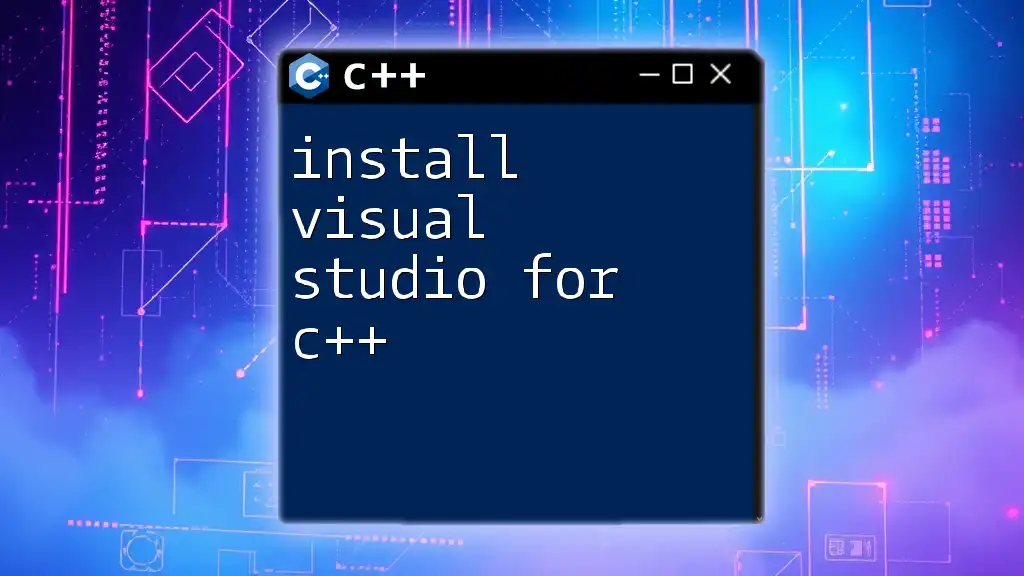
Debugging in VS Code
Setting Up the Debugger
To efficiently debug your code, you need to set up the debugger in VS Code:
- Click on the debug icon on the Activity Bar.
- Click the gear icon to create a new launch configuration.
- Edit the `launch.json` file that appears. A sample configuration for C++ looks like this:
{
"version": "0.2.0",
"configurations": [
{
"name": "Debug C++",
"type": "cppdbg",
"request": "launch",
"program": "${workspaceFolder}/hello",
"args": [],
"stopAtEntry": false,
"cwd": "${workspaceFolder}",
"environment": [],
"externalConsole": false,
"MIMode": "gdb",
"setupCommands": [
{
"text": "-enable-pretty-printing",
"description": "Enable pretty printing"
}
],
"preLaunchTask": "build",
"miDebuggerPath": "/usr/bin/gdb",
}
]
}
Debugging Your Code
Set breakpoints by clicking in the gutter next to the line numbers in your code. You can then start debugging by pressing `F5`. During the debugging session, you'll be able to inspect variables, step over functions, and analyze the program flow effectively.
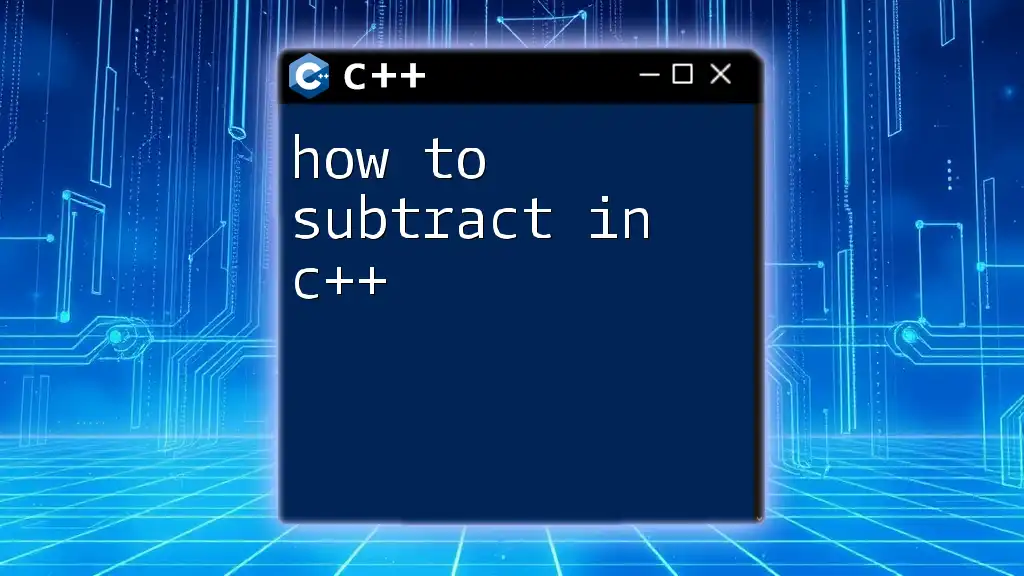
Advanced Configuration Options
Customizing Key Bindings
VS Code allows you to customize key bindings to enhance your development speed.
- Go to File > Preferences > Keyboard Shortcuts.
- Search for commands and set shortcuts that make sense to your workflow, like running the code, debugging, etc.
Using Multiple Workspaces
If you're working on multiple projects, you can create several workspaces. Simply create a new folder for each project and open it in VS Code. You can easily switch between workspaces, keeping your projects organized.
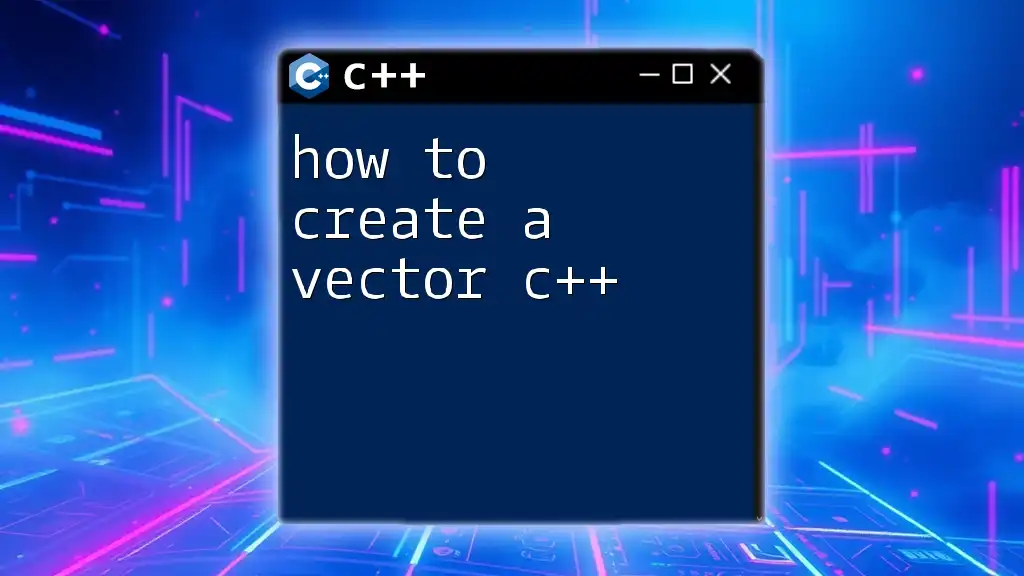
Troubleshooting Common Issues
Compilation Issues
If you encounter any compilation errors, check the following:
- Ensure that your g++ path is correctly set in your system's PATH environment variable.
- Make sure you’re in the directory of the `.cpp` file when you compile.
Running Issues
If your program doesn't run:
- Double-check the compiled file’s name and ensure you're running the correct command.
- Check for runtime errors or misconfigurations in your code.
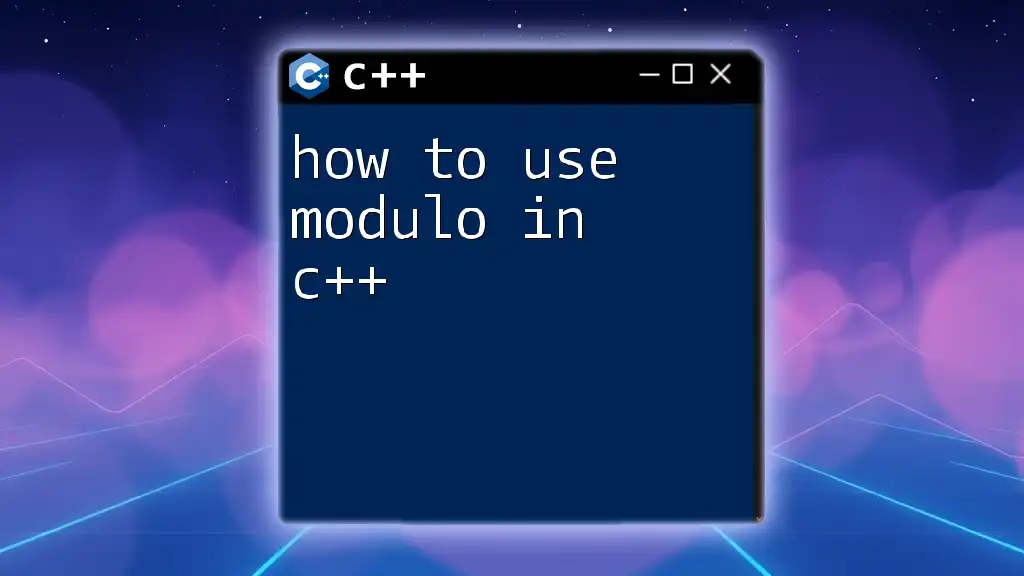
Conclusion
Setting up Visual Studio Code for C++ development can seem daunting, but by following these steps, you should feel confident in configuring your environment. Taking the time to properly set up your IDE and compiler will significantly enhance your coding experience, allowing you to focus on creating amazing programs. Don’t hesitate to share your experiences or any issues you encounter during your journey into C++ programming!