In C++, you can loop through a vector using a range-based for loop to easily access each element, as shown in the following example:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
Understanding Vectors in C++
What is a Vector?
A vector in C++ is a dynamic array that can grow or shrink in size at runtime. Unlike normal arrays, which have a fixed size, vectors allocate memory as required, making them flexible and versatile for various programming needs.
Vectors also provide various built-in functions for easy manipulation, such as adding, removing, and accessing elements. They are part of the Standard Template Library (STL), which focuses on the efficiency and reusability of code.
Initializing a Vector
To start using vectors in your C++ programs, you first need to declare and initialize them. This process is straightforward:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
}
In this example, we include the `<vector>` header to utilize the vector functionalities. The variable `numbers` is declared as a vector of integers and is initialized with a list of integers. This demonstrates the ease of using a vector compared to fixed-size arrays.
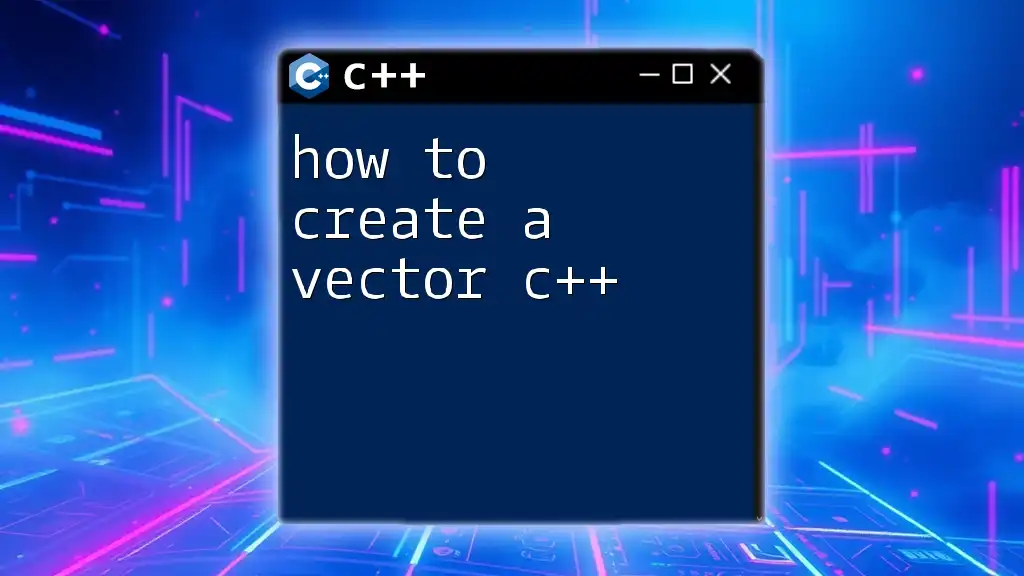
Why Iterate Through a Vector?
When working with vectors, knowing how to loop through a vector in C++ is crucial. Iteration allows you to access and process each element, enabling a wide range of operations like calculations, transformations, and conditional filtering. Without iteration, managing the data stored in a vector would be cumbersome and inefficient.
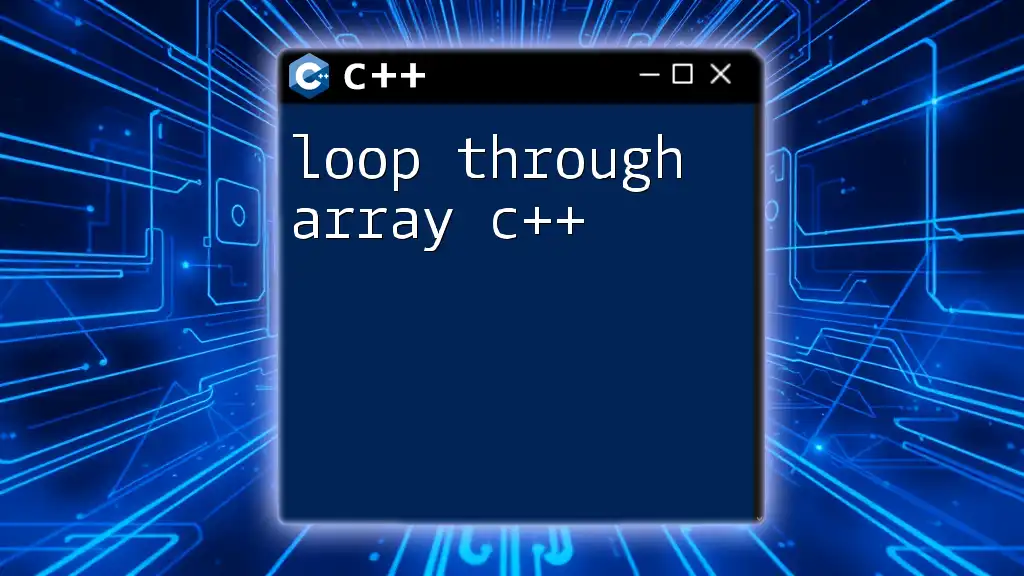
Different Ways to Iterate Through a Vector
Using a Traditional For Loop
One of the most basic yet effective methods to iterate through a vector is by using a traditional for loop. This method is flexible and straightforward, allowing control over the index:
for (size_t i = 0; i < numbers.size(); ++i) {
std::cout << numbers[i] << " ";
}
In this snippet, we declare a loop that runs as long as `i` is less than the size of the vector. The `size()` function returns the total number of elements, ensuring we stay within bounds.
Using a Range-Based For Loop
Introduced in C++11, the range-based for loop simplifies the syntax when you want to iterate through every element of a vector without worrying about indices:
for (auto& num : numbers) {
std::cout << num << " ";
}
In this case, `auto&` automatically deduces the type of `num`, eliminating the need for manual indexing. It's a clean and expressive way to iterate, making the code easier to read.
Using Iterators
Another approach is to use iterators, which are generalized pointers that can traverse through container elements. Iterators provide a level of abstraction, allowing you to write code that can interchangeably work with different STL containers.
for (std::vector<int>::iterator it = numbers.begin(); it != numbers.end(); ++it) {
std::cout << *it << " ";
}
In this example, `begin()` returns an iterator pointing to the first element, while `end()` points just past the last element. Using the iterator allows you to dereference it with `*it` to access the actual value.
Using `std::for_each`
C++ provides standard library algorithms like `std::for_each`, which allows you to apply a function to each element in the vector. This can be especially useful for operations that require a callback function:
#include <algorithm>
std::for_each(numbers.begin(), numbers.end(), [](int num) {
std::cout << num << " ";
});
The lambda function passed to `std::for_each` takes each element `num` in the vector and performs the operation defined within its body, such as printing it out.
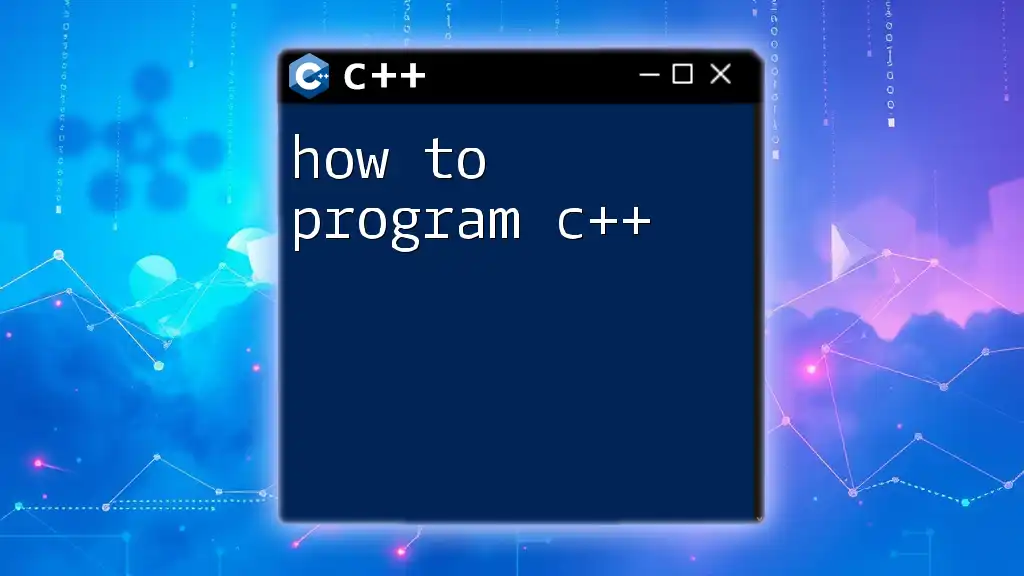
Performance Considerations
When to Use Each Method
While all methods are effective for iterating through a vector, each has its benefits and use cases. The traditional for loop offers fine control, while range-based loops provide cleaner syntax. Iterators bring flexibility, especially when you may want to switch containers, and `std::for_each` is ideal for functional programming approaches.
Consideration of Vector Size
The performance of these methods can vary based on the size of the vector. For instance, direct index access with a traditional for loop can be faster for smaller vectors, while for larger amounts of data, using iterators might lead to more optimized memory access.
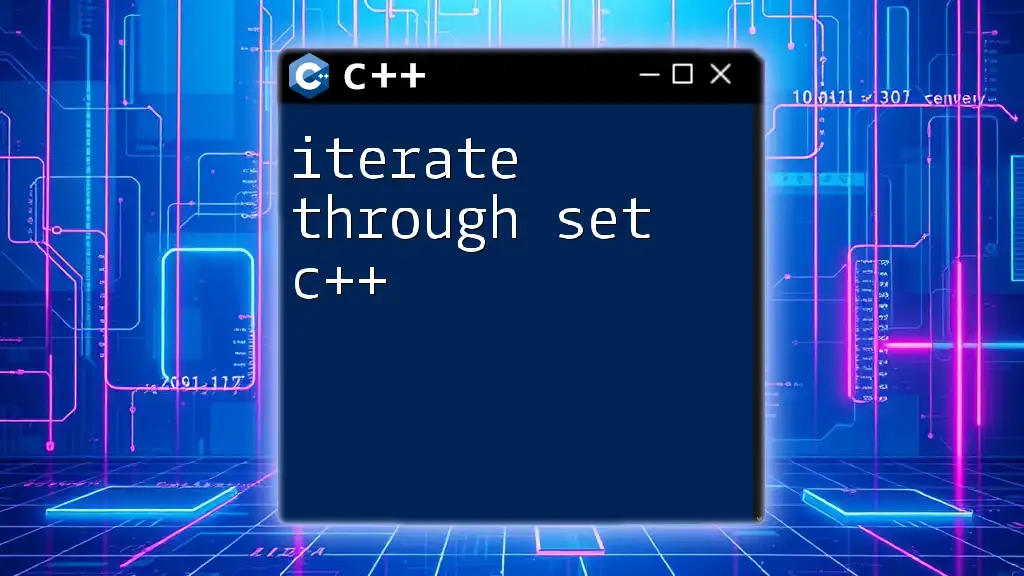
Common Mistakes While Looping Through a Vector
When learning how to iterate over a vector in C++, being aware of common mistakes is vital. Here are several pitfalls to avoid:
- Out-of-Bounds Errors: Ensure your code does not access index values outside the vector's size.
- Invalid Iterators: Avoid using iterators after modifying the vector structure (e.g., adding or removing elements).
- Dereferencing Null: Be cautious with iterators; dereferencing an invalid iterator can lead to crashes.
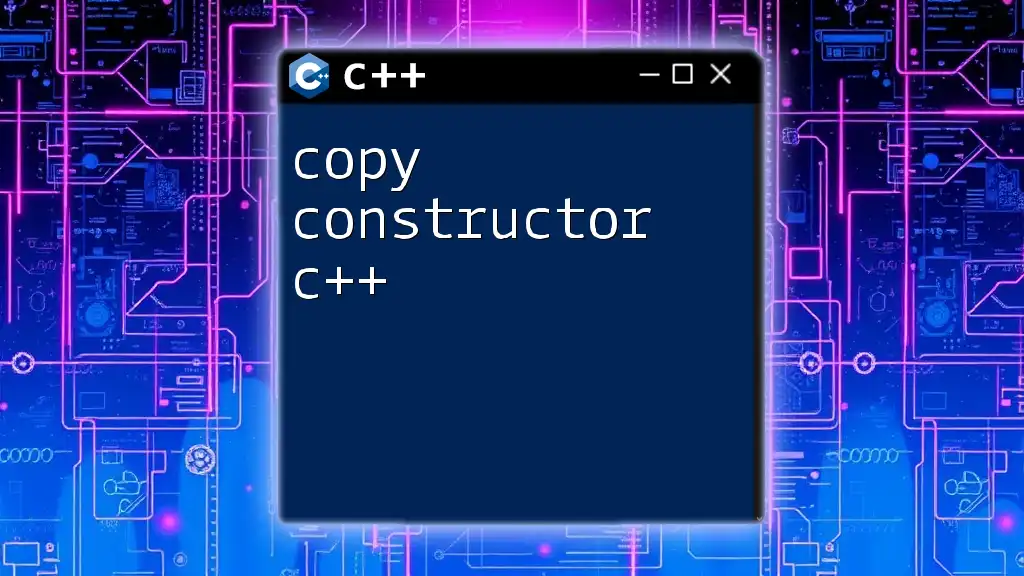
Conclusion
In this guide, we've explored multiple ways to loop through a vector in C++, each with its specific advantages. From traditional for loops to modern range-based loops and iterators, knowing these methods equips you with the tools necessary for effective data manipulation.
As you continue your programming journey, remember that practice is key. Experiment with the different iteration methods and find what suits your style and needs best. Happy coding!
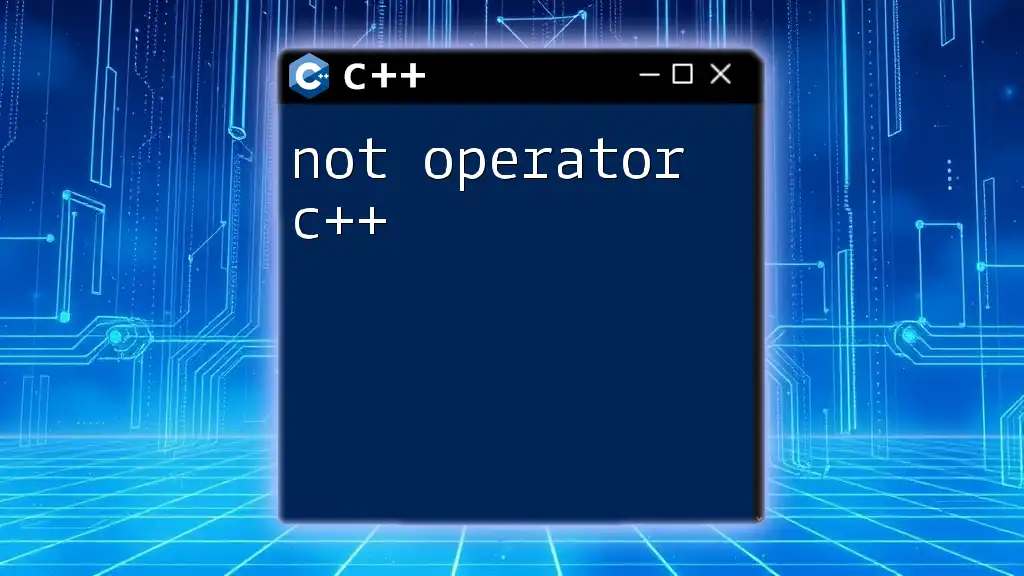
Frequently Asked Questions
What Is the Best Way to Iterate Through a Vector in C++?
While there's no one-size-fits-all answer, the best method depends on your requirements. For straightforward iterations, the range-based for loop is generally preferred for its readability. If you need to manipulate index values, the traditional for loop could be advantageous.
Can I Use While Loops to Iterate Through a Vector?
Certainly! A while loop can be employed as well:
size_t i = 0;
while (i < numbers.size()) {
std::cout << numbers[i++] << " ";
}
This method functions similarly to the traditional for loop but offers a different style of writing the iteration.
Are There Alternatives to Vectors in C++?
Yes, besides vectors, C++ offers containers such as lists, sets, and maps. Each has its purpose and characteristics, making them suitable for various scenarios. Understanding their differences will help you choose the right container for your specific needs.
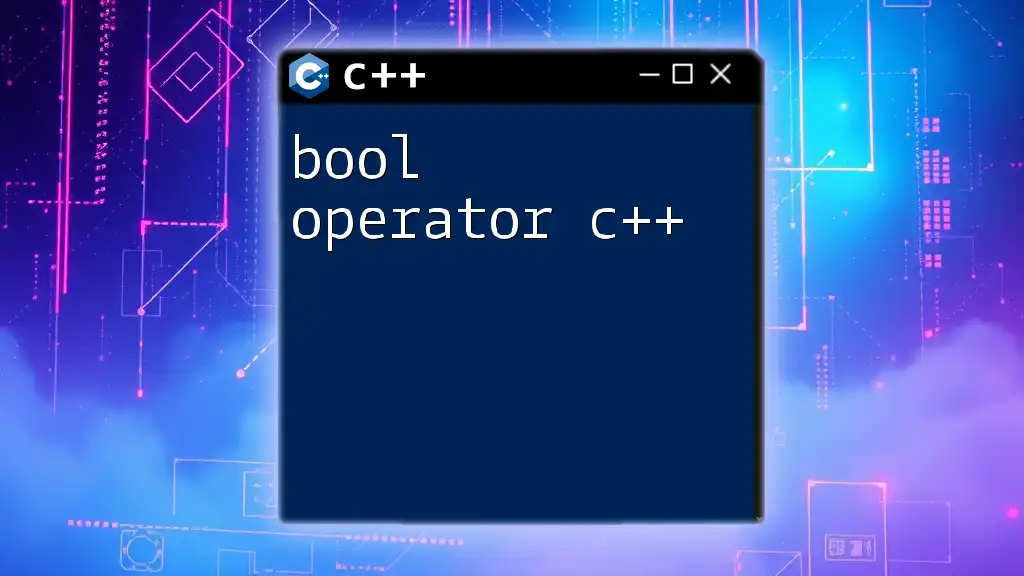
Call to Action
To master C++, dive deeper into vector operations and iteration techniques. We encourage you to share your experiences in the comments below or to ask any further questions you have about looping through vectors. Stay curious and keep coding!