In C++, you can iterate through a `set` using an iterator or a range-based for loop to access each unique element, as shown in the following example:
#include <iostream>
#include <set>
int main() {
std::set<int> mySet = {1, 2, 3, 4, 5};
// Using range-based for loop
for (int num : mySet) {
std::cout << num << " ";
}
return 0;
}
Understanding Sets in C++
In C++, a set is a container that stores unique elements following a specific order. Unlike other collections, such as vectors or lists, sets automatically manage duplicates by eliminating them. This makes sets extremely useful when data integrity is crucial, like when you're dealing with unique identifiers.
Syntax for Using Set
To use a set, you must include the `<set>` header. You can initialize a set with various data types, typically integers or strings:
#include <set>
std::set<int> mySet;
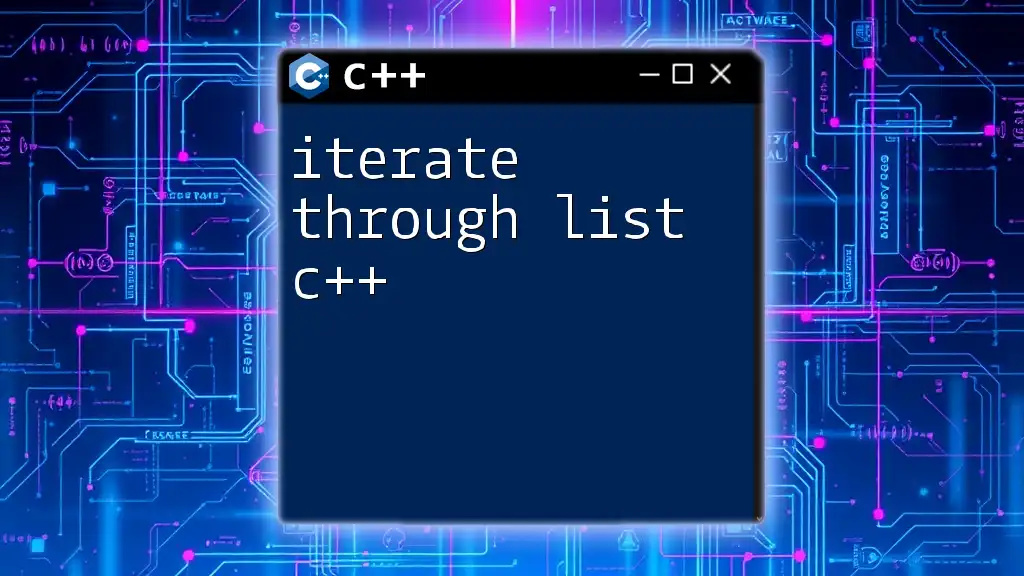
Methods for Iterating Through a Set
Using Iterators
Iterators are a standardized way to traverse elements within C++ containers. They provide a way to access elements sequentially, without exposing the underlying structure.
Forward Iteration with Iterators
Using iterators provides precise control over the traversal of elements. Here's how you can iterate through a set:
std::set<int> mySet = {1, 2, 3, 4, 5};
for (std::set<int>::iterator it = mySet.begin(); it != mySet.end(); ++it) {
std::cout << *it << std::endl;
}
In this example:
- `mySet.begin()` returns an iterator pointing to the first element of the set.
- `mySet.end()` returns an iterator pointing just past the last element.
- The loop continues until the iterator reaches the end, while *`it` dereferences the iterator to access the actual value.
This method is particularly powerful for more complex operations, as it allows for more advanced manipulations.
Range-based For Loop
If you're working with C++11 or newer, you can utilize range-based for loops, which simplify the syntax and improve code readability. Here's how it looks:
for (const auto& element : mySet) {
std::cout << element << std::endl;
}
In this code:
- `auto` automatically deduces the type of `element`.
- The loop iterates through each element in `mySet`, making your code cleaner and less error-prone.
Standard Library Algorithms
The C++ Standard Library offers powerful algorithms for performing operations on sets. One useful function is `std::for_each`, combined with lambda expressions:
std::for_each(mySet.begin(), mySet.end(), [](int elem) {
std::cout << elem << std::endl;
});
This technique allows you to encapsulate functionality succinctly and promotes cleaner code. The lambda does the printing of each element, making the process straightforward.
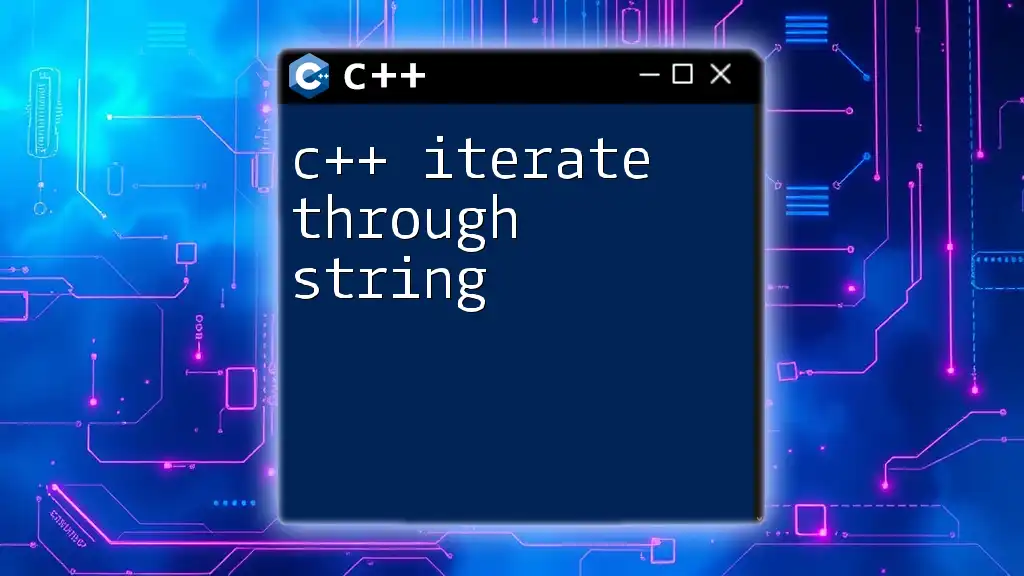
Modifying Elements While Iterating
When working with sets, one of the critical aspects to understand is the implications of modifying the set while iterating. If you want to remove elements based on certain criteria without causing invalid iterator access, you can do this safely:
for (auto it = mySet.begin(); it != mySet.end(); ) {
if (*it % 2 == 0) {
it = mySet.erase(it); // Safe deletion while iterating
} else {
++it; // Move to next item
}
}
In this example:
- The `erase` method not only removes the element but also returns a new iterator pointing to the next element, which allows you to continue iterating safely without skipping elements.
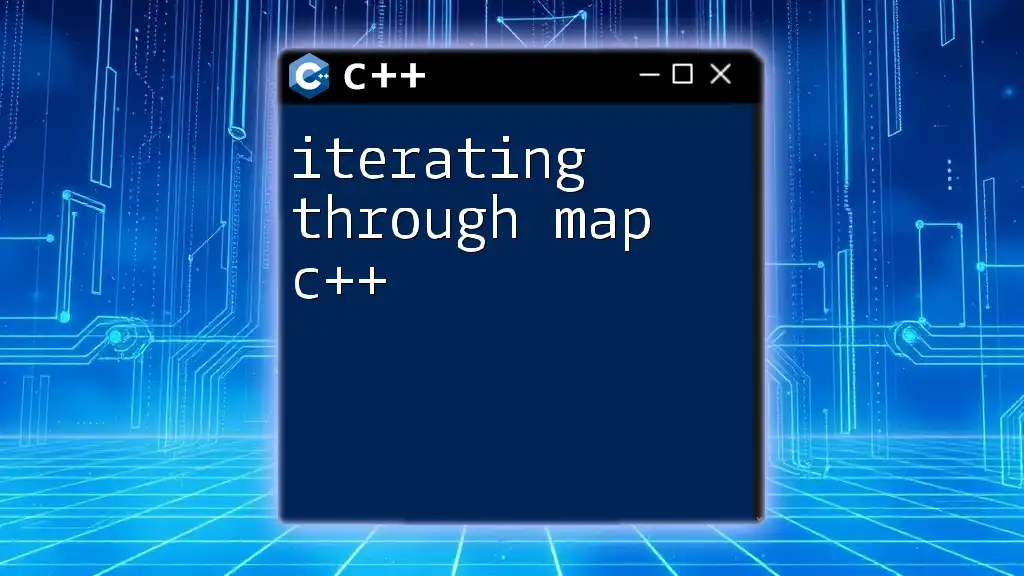
Common Pitfalls to Avoid
While iterating over a set, keep in mind several common issues that may arise:
-
Dereferencing Invalid Iterators: If you modify the set (like erasing elements) while iterating without proper care, you risk dereferencing an invalid iterator, leading to undefined behavior. Always ensure to update iterators appropriately after modifications.
-
Using the Wrong Iterator Type: It's crucial to know the distinction between const and non-const iterators. Use const iterators when you only need read access to elements, as this helps signal intent and avoid unintended modifications.
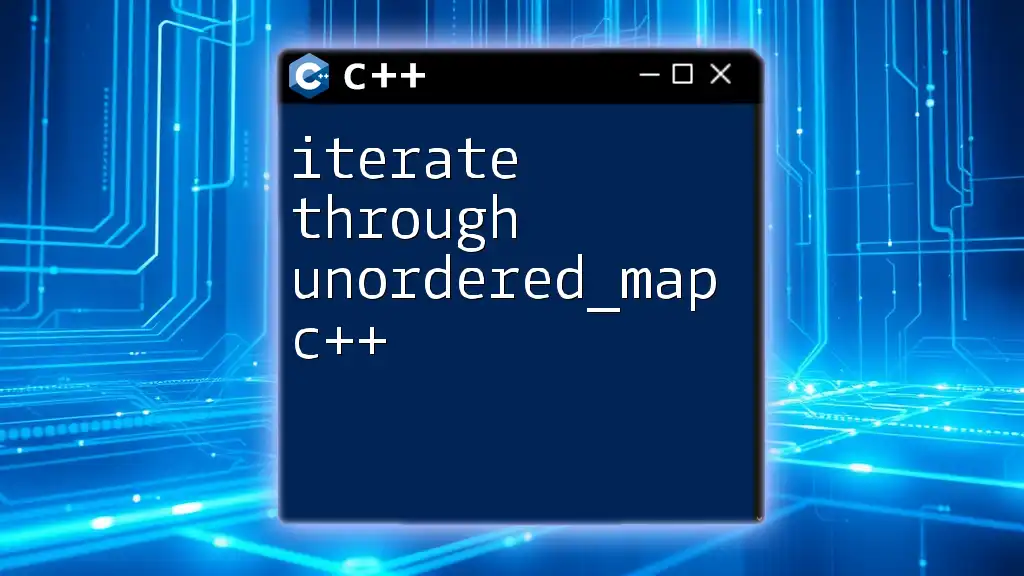
Conclusion
In summary, iterating through a set in C++ is a fundamental skill that can significantly enhance data manipulation capabilities. Whether you use iterators, range-based for loops, or standard library algorithms, understanding these methods can make your code more efficient and expressive.
Encouragement to Practice
Take some time to practice these various methods of iteration. Experimenting with sets and their iteration techniques will strengthen your C++ programming skills.
Resources for Further Learning
To further your learning, consider exploring C++ documentation, online courses, and programming communities where you can ask questions and engage with other learners.
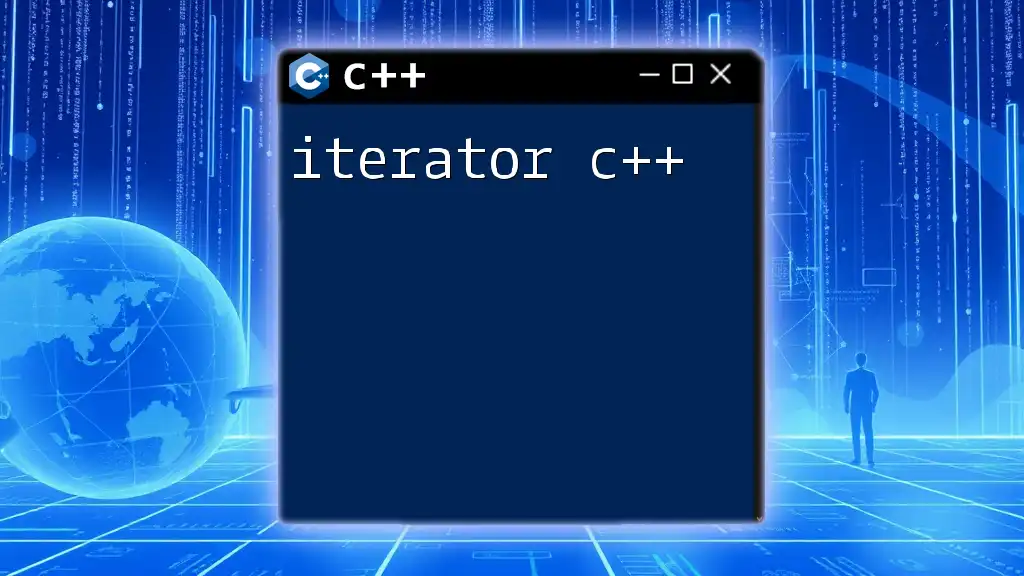
Call to Action
Feel free to share your experiences or ask questions about how to iterate through sets in C++ in the comments section. Embrace the journey of mastering this powerful programming language!