To iterate through a list in C++, you can use a range-based for loop, which simplifies the process of accessing each element in the list.
#include <iostream>
#include <list>
int main() {
std::list<int> myList = {1, 2, 3, 4, 5};
for (int item : myList) {
std::cout << item << " ";
}
return 0;
}
Understanding C++ Lists
What is a List in C++?
A list in C++ refers to an ordered collection of elements that can grow or shrink in size dynamically. The list is part of the Standard Template Library (STL) and is particularly useful when you need to maintain the order of elements or require frequent insertions and deletions.
Differences Between List and Other Containers
C++ provides multiple collection types, and understanding their differences is crucial:
- `std::list` is a doubly linked list. Elements can be inserted or removed efficiently from both ends and in the middle, but accessing elements by index is linear in complexity.
- `std::vector` is a dynamic array that offers fast indexing but requires contiguous memory allocation. Inserting or deleting from the middle or front is costly as it may involve shifting of elements.
- `std::deque` (double-ended queue) allows for fast insertions and deletions at both ends like `std::list`, but supports random access like `std::vector`.
Choosing `std::list` over others often comes down to specific use cases where the dynamic nature of the data structure is a priority, especially if frequent insertions and deletions are expected at arbitrary positions.
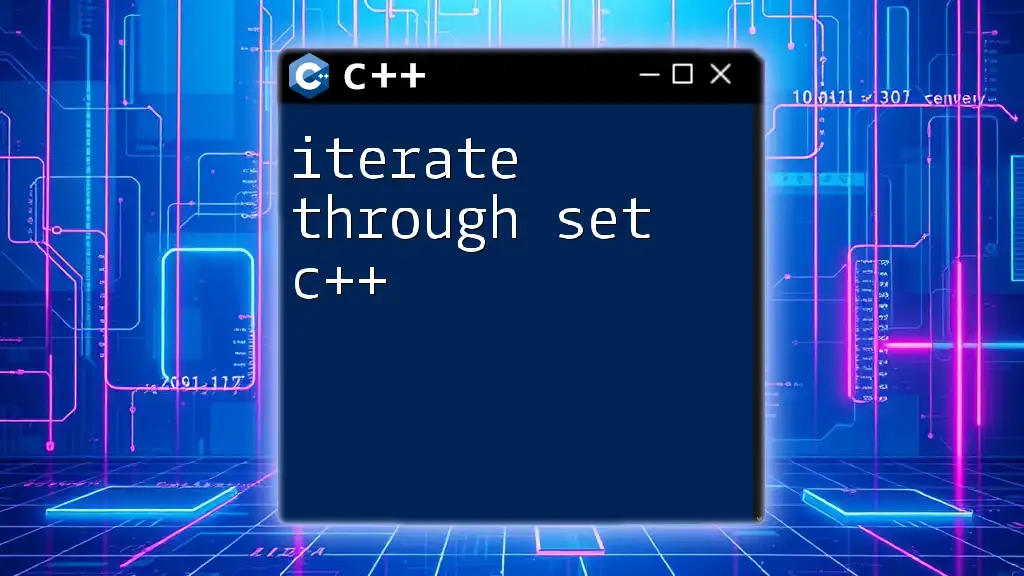
C++ List Syntax
Declaring a List
To declare a list, you need to include the `<list>` header file. The syntax generally looks like this:
#include <list>
std::list<int> myList;
Initializing a List
You can initialize a list using different methods:
- Default Initialization: Creates an empty list.
- List with Elements: Initialize with a set of elements right away.
Example:
std::list<int> myList = {1, 2, 3, 4, 5};
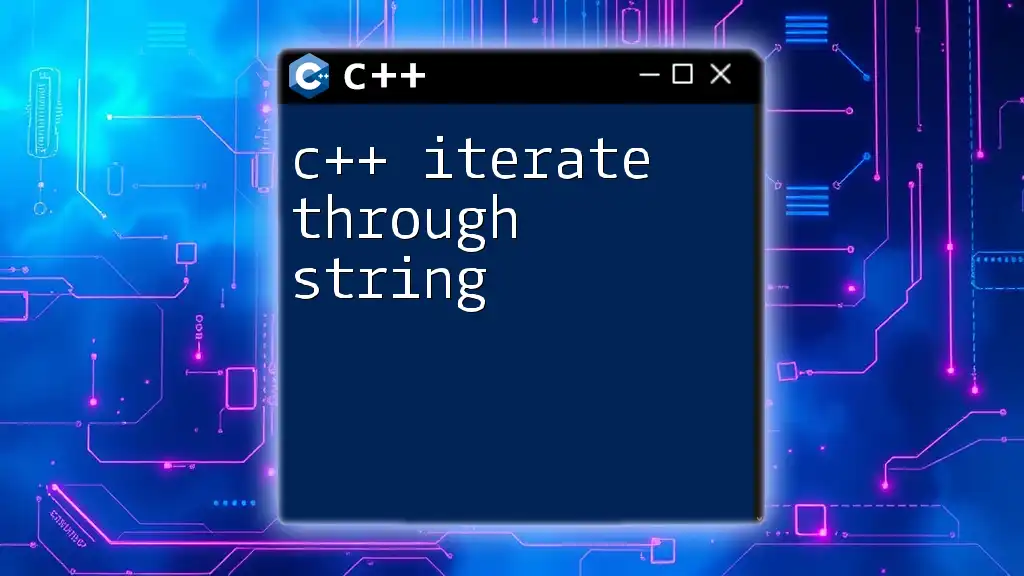
Iteration Basics
What is Iteration?
Iteration is the process of accessing each element in a data structure systematically. In C++, iterating through a list is crucial for tasks such as searching, modifying values, or processing elements based on specific criteria.
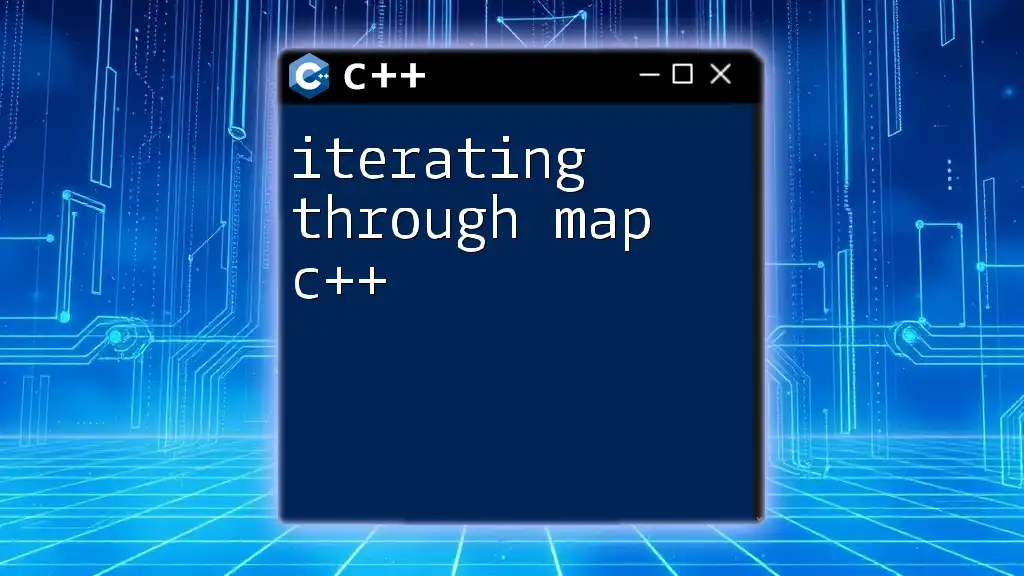
Methods to Iterate Through a C++ List
Using Iterators
Introduction to Iterators
Iterators are objects that allow you to traverse a container without exposing its underlying representation. They provide a uniform way of accessing elements, making code more readable and maintainable.
Basic Iteration with Iterators
Using iterators is a common method for iterating through lists. Here’s an example:
for (std::list<int>::iterator it = myList.begin(); it != myList.end(); ++it) {
std::cout << *it << " ";
}
In this code, `it` starts at the beginning of `myList` and continues moving forward until it reaches the end. The `*it` syntax is used to dereference the iterator, accessing the value it points to.
Range-based For Loop
Simplifying Iteration
Introduced in C++11, range-based for loops simplify the syntax for iterating through collections like lists, enhancing readability.
Example Usage
Here’s how you can make use of a range-based for loop to iterate through a list:
for (const int &val : myList) {
std::cout << val << " ";
}
This structure eliminates the need for iterators explicitly, making the code cleaner and easier to read. The `const` reference allows for efficiency while ensuring that the original list elements remain unchanged.
Using Standard Algorithms
Introduction to Algorithms in STL
C++ STL includes powerful algorithms that can operate on containers, including lists. These algorithms often utilize iterators to access the elements.
For_each Algorithm
The `std::for_each` algorithm can be an effective way to apply a function to each element in a list. This can be an anonymous function (lambda expression) as shown in the example below:
#include <algorithm>
std::for_each(myList.begin(), myList.end(), [](int val) {
std::cout << val << " ";
});
This method makes the code not only concise but also allows for functional programming techniques within C++.
Other Iteration Techniques
Using Recursion
Iteration can also be accomplished through recursion, particularly when dealing with more complex data access patterns. While recursive methods may not be optimal for large lists due to potential stack overflow, they can provide elegant solutions for smaller problems.
Here’s a simple recursive function that iterates through a `std::list`:
void printList(const std::list<int>& lst) {
if (lst.empty()) return;
std::cout << lst.front() << " ";
std::list<int> rest(lst.begin() + 1, lst.end());
printList(rest);
}
This function continues to print the front element of the list and then recursively calls itself on the rest of the elements.
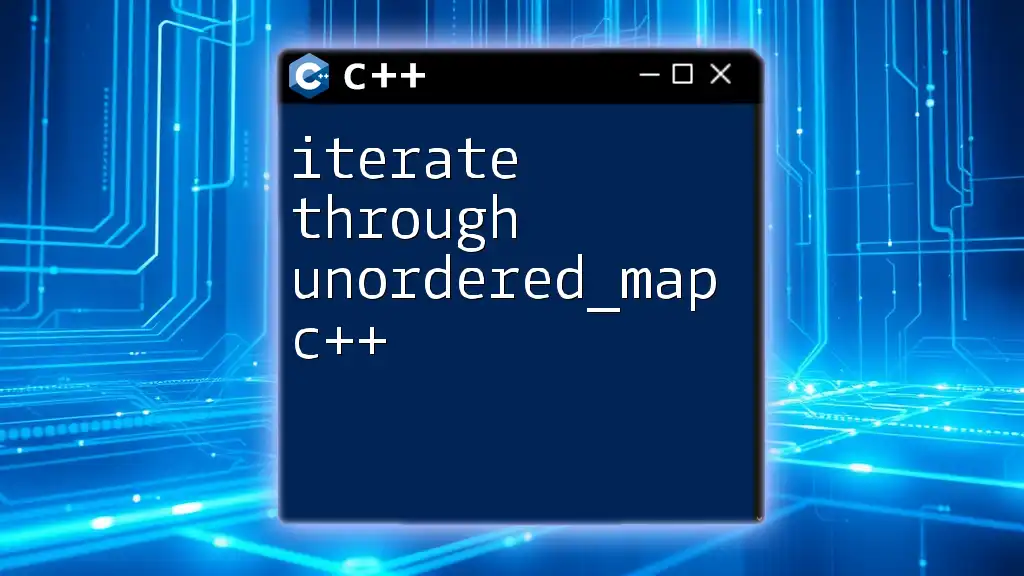
Common Pitfalls and Best Practices
Issues with Iterators
When working with iterators, one common pitfall is invalidating iterators. This typically happens when you remove or modify elements in the list during iteration, which can lead to undefined behavior or crashes.
To maintain iterator validity, you should:
- Avoid modifying the list while iterating through it.
- Use methods such as `std::list::erase` that return an iterator to the next valid element after removal.
Performance Considerations
When deciding on an iteration method, consider the following:
- Use iterators for complex iterations that involve element removals or modifications.
- Employ range-based for loops for simple read-only operations.
- Use `std::for_each` for a functional style of programming, especially when working with predefined algorithms.
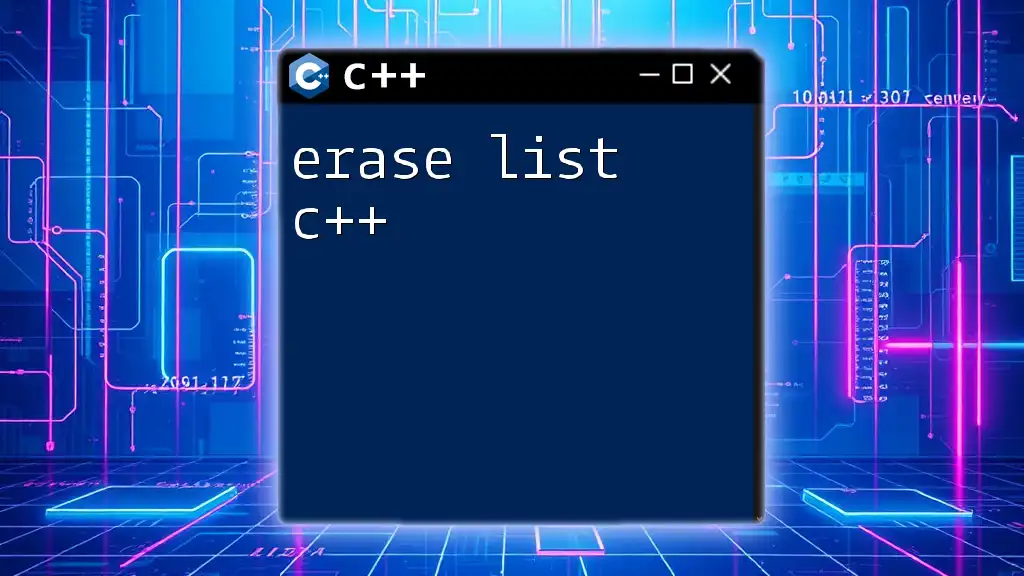
Conclusion
Mastering how to iterate through a list in C++ is essential for efficient programming in the language. By understanding the available methods and their implications, you can write cleaner, more effective code while leveraging the power of C++ STL.
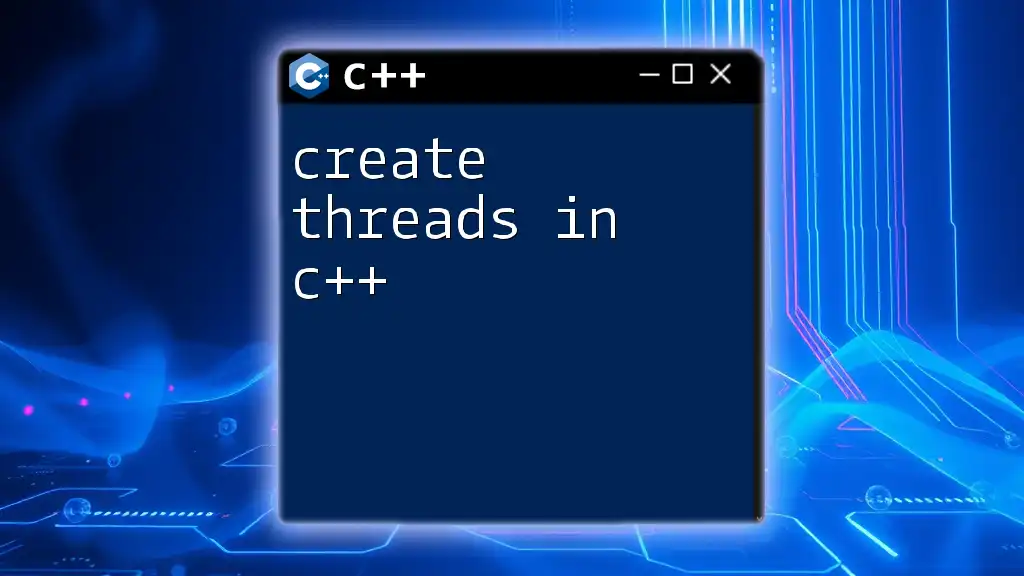
Additional Resources
For further mastery, consider exploring C++ documentation, online tutorials, and dedicated books on STL and algorithms to deepen your understanding. Engaging with practical examples and honing your skills is the key to becoming proficient in utilizing lists and their iteration techniques in C++.