To reverse a vector in C++, you can use the `std::reverse` function from the `<algorithm>` header, as shown in the following code snippet:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
std::reverse(vec.begin(), vec.end());
for(int n : vec) std::cout << n << " "; // Output: 5 4 3 2 1
return 0;
}
Understanding Vectors in C++
What is a Vector?
In C++, a vector is a dynamic array that can resize itself automatically when an element is added or removed. Vectors are part of the Standard Template Library (STL), which provides a collection of pre-defined classes and functions for use in C++ programming. One of the key benefits of vectors over standard arrays is that vectors can adjust their size, making them a versatile option for storing collections of data.
Basic Operations with Vectors
Before diving into the topic of reversing a vector in C++, it's essential to understand some basic operations that can be performed with vectors.
Creating a Vector:
To create a vector, you typically include the vector library and define a vector variable as follows:
#include <vector>
std::vector<int> myVector; // Creates an empty vector of integers
Adding Elements:
You can add elements to a vector using the `push_back()` method. Here’s how you can do it:
myVector.push_back(1); // Adds 1 to the vector
myVector.push_back(2); // Adds 2 to the vector
These operations allow you to build your vector dynamically.
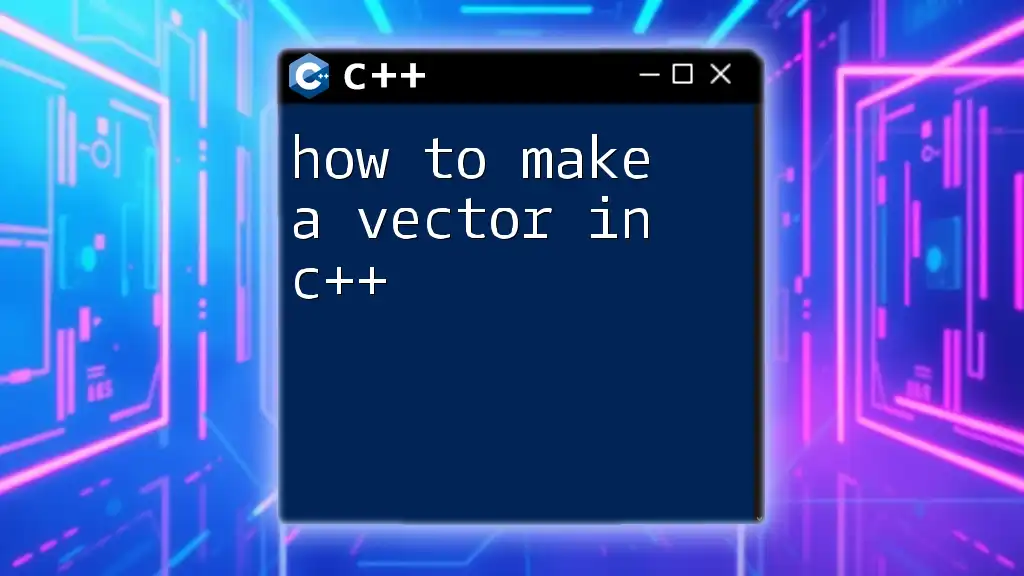
Why Reverse a Vector?
Reversing a vector can be beneficial in multiple scenarios. For example, you might need to reverse a collection of data to display it in a different order, undo actions or navigate backward through a sequence of elements. By learning how to reverse a vector in C++, you'll be well-equipped to handle these situations efficiently.
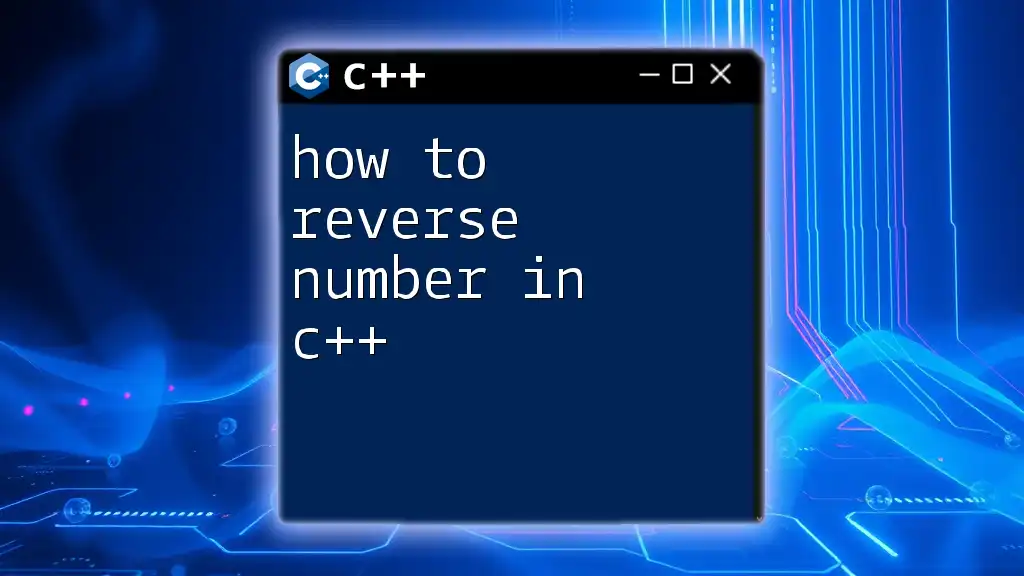
Methods to Reverse a Vector in C++
Utilizing std::reverse from the Standard Library
What is std::reverse?
`std::reverse` is a function provided by the C++ Standard Library in the `<algorithm>` header that allows programmers to reverse the order of elements within a range. This method is often preferred for its simplicity and efficiency.
Example of Using std::reverse
Here's a straightforward implementation using `std::reverse`:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
std::reverse(vec.begin(), vec.end());
for (int x : vec) {
std::cout << x << " ";
}
return 0;
}
In this code, `vec.begin()` and `vec.end()` are used to specify the range of elements to reverse. After calling `std::reverse`, the vector now contains the elements in reverse order: 5, 4, 3, 2, 1.
Manual Method of Reversing a Vector
Step-by-Step Explanation
If you prefer more control or want to implement the reversal without relying on the standard library, you can do so manually using a loop. This involves swapping the elements from the start and the end of the vector until you meet in the middle.
Example of Manual Reversal
The following example illustrates how to reverse a vector manually:
#include <iostream>
#include <vector>
void reverseVector(std::vector<int>& vec) {
int start = 0;
int end = vec.size() - 1;
while (start < end) {
std::swap(vec[start], vec[end]);
start++;
end--;
}
}
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
reverseVector(vec);
for (int x : vec) {
std::cout << x << " ";
}
return 0;
}
In this implementation, the function `reverseVector` takes a vector by reference and swaps its elements until the start index is greater than or equal to the end index. The use of `std::swap()` ensures that the elements are interchanged correctly, and the loop terminates once all elements have been reversed.
Using Recursion to Reverse a Vector
How Recursion Works
Recursion involves a function calling itself to solve smaller subproblems. This approach can be particularly elegant for reversing a vector, allowing you to take advantage of the function call stack.
Example of Recursion for Reversing a Vector
Here is an example of reversing a vector using recursion:
#include <iostream>
#include <vector>
void reverseVectorRecursively(std::vector<int>& vec, int start, int end) {
if (start >= end) return; // Base case to stop recursion
std::swap(vec[start], vec[end]); // Swap the elements
reverseVectorRecursively(vec, start + 1, end - 1); // Recursive call
}
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
reverseVectorRecursively(vec, 0, vec.size() - 1);
for (int x : vec) {
std::cout << x << " ";
}
return 0;
}
In the `reverseVectorRecursively` function, the recursive approach reduces the problem size by one for each function call, keeping track of the start and end indices. The base case ensures that the recursion stops when the starting index becomes equal to or greater than the ending index.
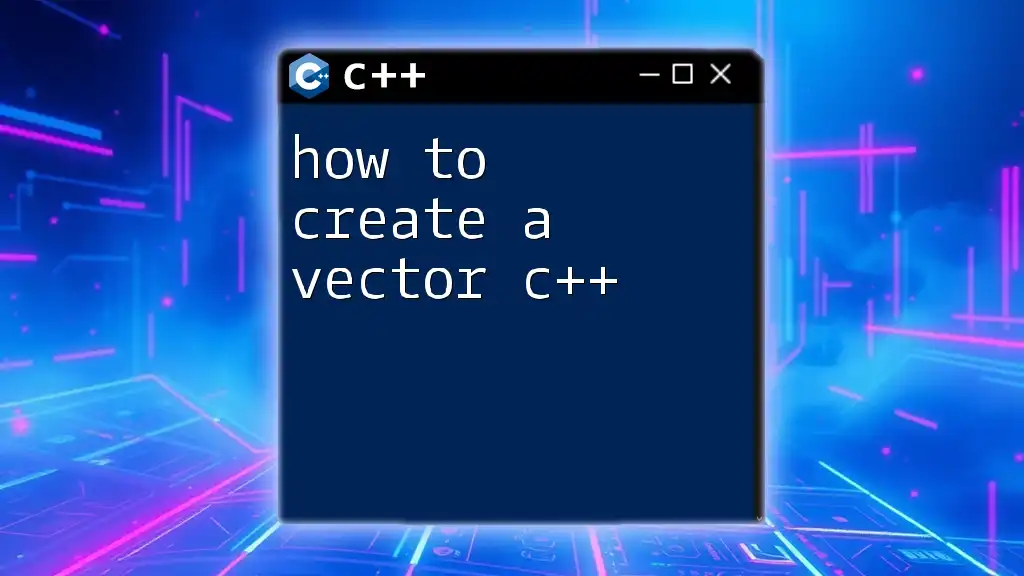
Performance Considerations
Time Complexity of Reversing a Vector
The time complexity of all the methods outlined for reversing a vector—whether using `std::reverse`, manual iteration, or recursion—is O(n), where n is the number of elements in the vector. This means that the time taken to reverse the vector grows linearly with the size of the vector.
Memory Usage
The memory usage also varies depending on the method used. The `std::reverse` function operates in place, thus requiring only a constant amount of extra memory. Similarly, the manual method also reverses the vector in place. However, if you choose to create a copy of the vector for the purpose of reversal, it can lead to increased memory consumption.
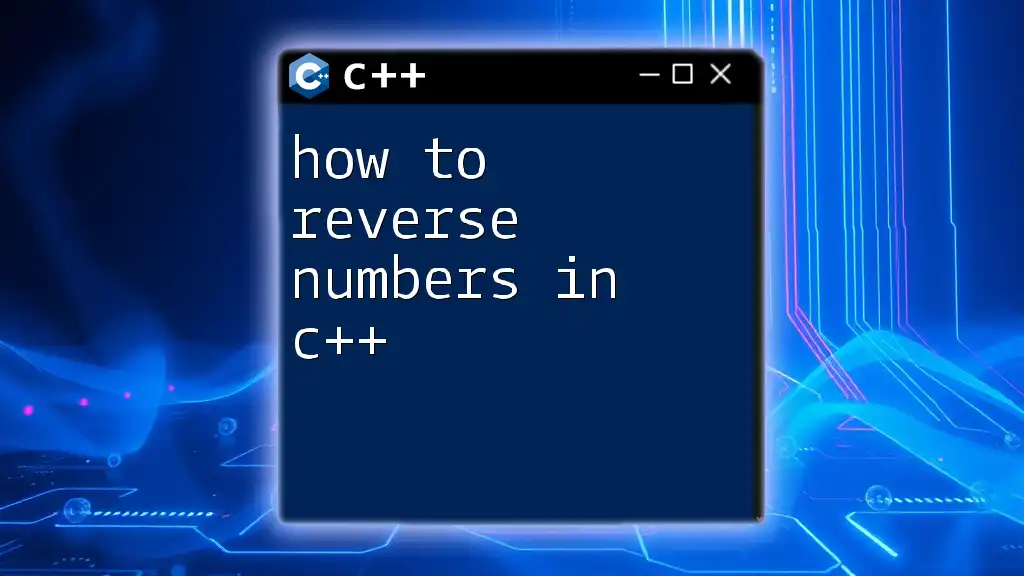
Conclusion
In summary, you now have several techniques for learning how to reverse a vector in C++. By utilizing the built-in `std::reverse` function, manually swapping elements, or employing recursion, you can efficiently reverse the order of elements in your vectors. Each method has its own merits, and understanding them will allow you to make informed choices based on your specific requirements.
With practice, you'll gain deeper insights into vector operations and become adept at handling a variety of data manipulation tasks in C++. Feel free to explore beyond this article and implement these techniques in your coding projects!