To create a list in C++, you can use the `std::list` container from the Standard Template Library (STL), which allows for dynamic size and provides efficient insertions and deletions.
#include <iostream>
#include <list>
int main() {
std::list<int> myList = {1, 2, 3, 4, 5}; // Creating a list of integers
for (int n : myList) std::cout << n << " "; // Output: 1 2 3 4 5
return 0;
}
Understanding Lists in C++
What is a List?
A list is a common data structure that allows you to store a collection of elements. Unlike arrays, lists are dynamic in size, which means they can grow and shrink as needed. This offers several advantages:
- Dynamic Resizing: You can easily add or remove elements without worrying about memory constraints.
- Efficient Insertions and Deletions: Lists allow efficient insertion and deletion operations compared to arrays, particularly when you need to add or remove elements from the middle.
Types of Lists in C++
There are various types of lists in C++:
-
Singly Linked List: Each element points to the next one. Good for scenarios where you mostly traverse and insert at the front.
-
Doubly Linked List: Each element points to both its next and previous elements. This allows for more flexibility in navigation.
-
Circular Linked List: A variation where the last element points back to the first. Useful for applications that require looping through the list.
Each type has its own specific use cases, and understanding them is vital for utilizing lists effectively.
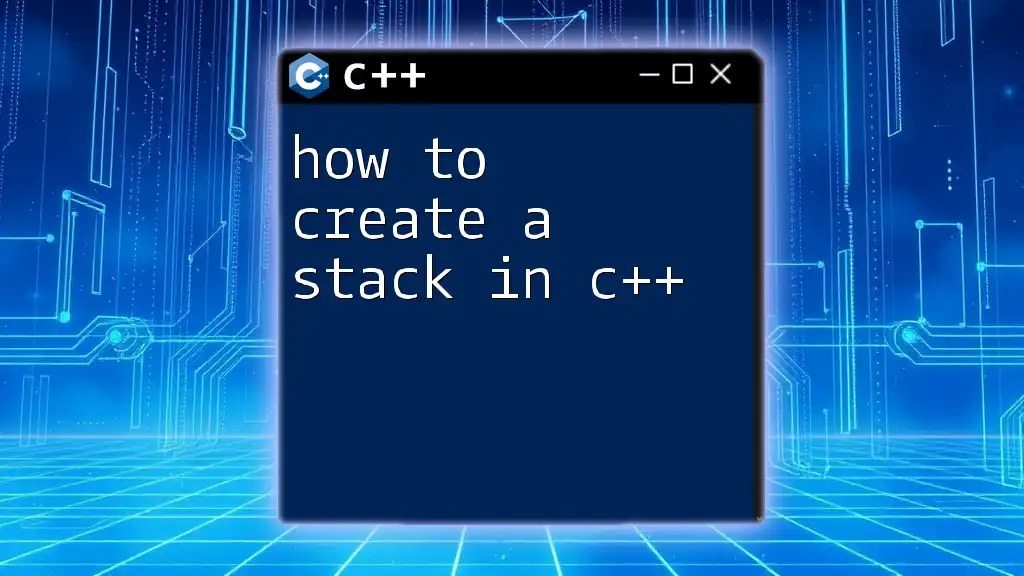
How to Make a List in C++
Setting Up Your Development Environment
Before you start coding, ensure you have the necessary tools:
- Compiler: You need a C++ compiler; popular options include GCC and MSVC.
- IDE: An Integrated Development Environment like Code::Blocks, Visual Studio, or even simple code editors like VS Code can make coding easier.
Setting up your development environment is crucial, especially for beginners, as it allows you to compile and run your code seamlessly.
The C++ Standard Library and List
The Standard Template Library (STL) is a powerful part of C++ that provides several data structures, including lists. Using the STL makes your code more efficient and easier to read.
The primary type for lists in STL is `std::list`. It offers several benefits:
- Convenience: You don't need to implement the list from scratch.
- Rich Functionality: `std::list` comes with built-in functions for various operations, like adding, removing, and sorting elements.
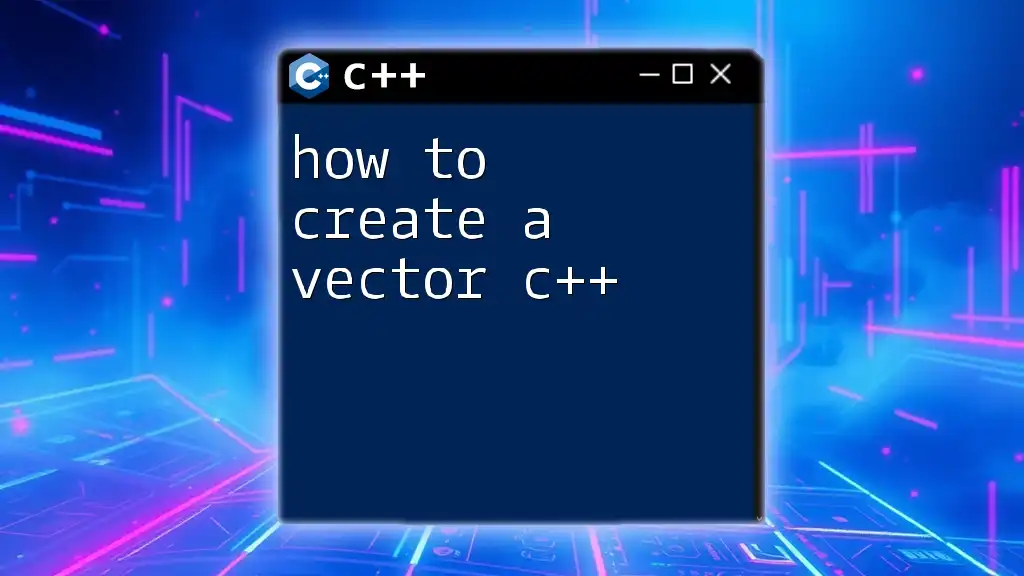
Getting Started with std::list
Creating a List
To create a list in C++, you simply declare it using the `std::list` syntax. Here’s how to create an empty list of integers:
#include <iostream>
#include <list>
int main() {
std::list<int> myList; // Creating an empty list of integers
return 0;
}
In this example, we've included the `<list>` header from the STL, allowing us to use the `std::list` class.
Adding Elements to a List
Adding elements to your list can be done in several ways.
Using push_back()
You can add elements to the end of the list using `push_back()`. For example:
myList.push_back(10);
myList.push_back(20);
This will add `10` first, followed by `20`, making the list `10, 20`.
Using push_front()
To add elements to the front of the list, you can use `push_front()`:
myList.push_front(5);
After this operation, your list would look like `5, 10, 20`.
Accessing Elements in a List
Accessing elements in a list is straightforward:
Front and Back
To access the first and last elements, you can use the `front()` and `back()` functions:
std::cout << "First element: " << myList.front() << std::endl;
std::cout << "Last element: " << myList.back() << std::endl;
These functions let you quickly get the values at the ends of the list without iterating through it.
Iterating Through a List
You can iterate through a list using iterators. A modern and simple way to do this is with a ranged-for loop:
for (int x : myList) {
std::cout << x << " ";
}
This will print all elements in your list sequentially.
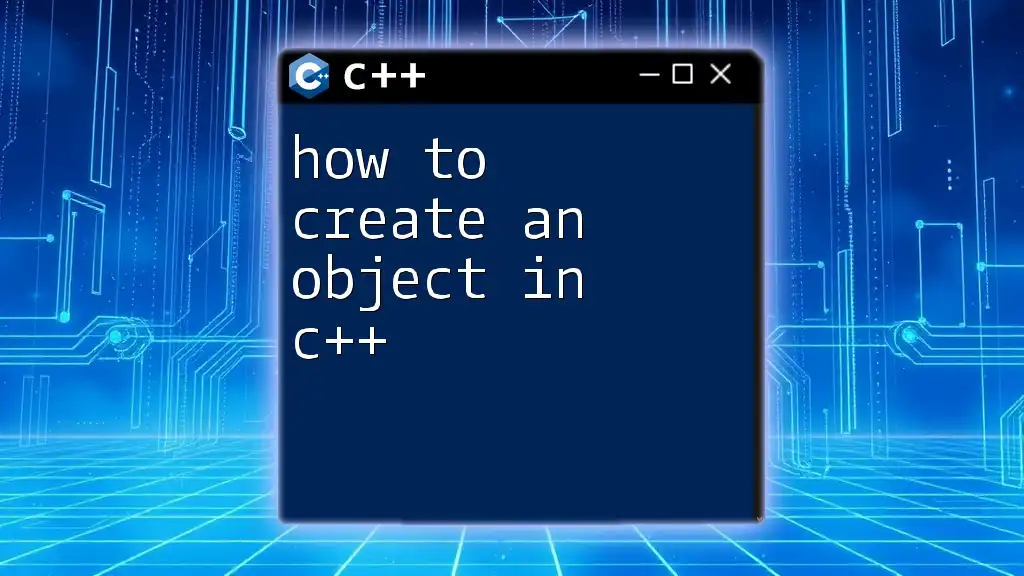
Removing Elements from a List
Using pop_back() and pop_front()
To remove the last or the first element of the list, you can use `pop_back()` and `pop_front()`:
myList.pop_back(); // Removes the last element
myList.pop_front(); // Removes the first element
These operations allow you to manage your list dynamically by removing unwanted elements easily.
Using erase()
To remove a specific element from the list, you can combine `erase()` with `find()`:
#include <algorithm> // Required for std::find
myList.erase(std::find(myList.begin(), myList.end(), 10));
This will remove the first occurrence of `10` from your list.
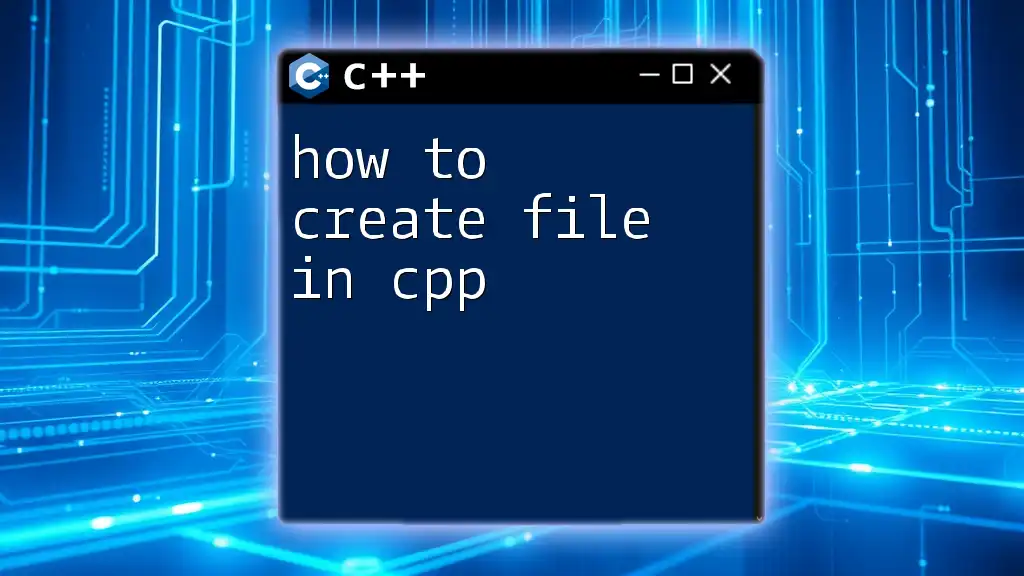
Common Operations on Lists
Sorting a List
To easily sort a list, you can use the `sort()` method provided by `std::list`. For example:
myList.sort();
This line will sort your list in ascending order. The sort is stable and ensures that equivalent elements retain their relative order.
Reversing a List
You can reverse the order of elements in your list with the `reverse()` method:
myList.reverse();
This is particularly useful when you want to process elements in the reverse order of their insertion.
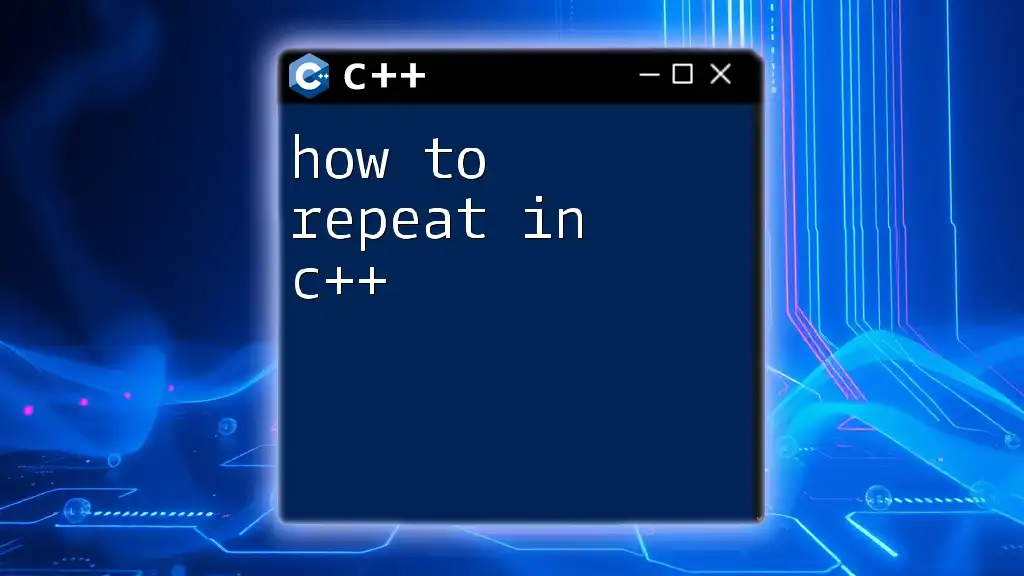
Conclusion
In this guide, we have explored how to create a list in C++ thoroughly. We've discussed the fundamentals of lists, their types, and how to utilize `std::list` effectively for various operations like adding, accessing, removing, sorting, and reversing elements.
Understanding lists provides a crucial building block for more advanced data structures and algorithms, and we encourage you to practice creating and manipulating lists in your own projects. With the knowledge from this article, you're well on your way to mastering C++ and its powerful capabilities!
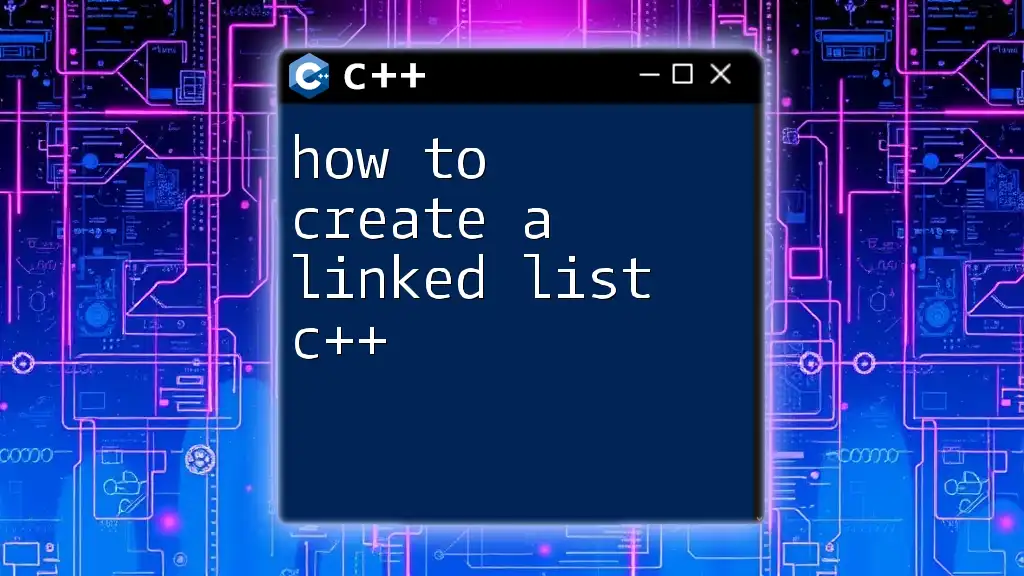
Additional Resources
For further exploration, consult the official C++ documentation for `std::list` and consider delving into textbooks and online courses focused on data structures and algorithms in C++.