To skip a line in C++, you can use the `std::cout` stream with `\n` or `std::endl`, both of which will insert a new line in the output.
Here's an example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl; // skips to a new line
std::cout << "This is the next line." << std::endl;
std::cout << "Another way to skip a line:\n"; // skips to a new line
std::cout << "This is also the next line." << std::endl;
return 0;
}
Understanding Line Skips in C++
What is a Line Skip?
In programming, a line skip refers to the concept of moving the output cursor to the beginning of the next line. This is essential for improving the readability and aesthetics of the output from your programs. When you print messages to the console or a file, strategically skipping lines can make information clearer to the user, thus enhancing the overall user experience.
Common Use-Cases
Line skipping is particularly beneficial in scenarios like:
- User Interfaces: When displaying information, a clean layout can significantly improve usability.
- Console Applications: Separating outputs or error messages allows users to discern critical parts of information more easily.

Methods to Skip a Line in C++
Using `std::endl`
`std::endl` is a manipulator in C++ that not only inserts a newline character but also flushes the output buffer. This means that any output generated is sent to the terminal or console immediately, which can be essential when real-time output is required.
Code Example
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
std::cout << "This skips a line." << std::endl;
return 0;
}
In this example, the message "Hello, World!" is displayed, followed by a line skip, enabling the next message to appear on a new line. This can be particularly important in interactive applications where timely feedback is crucial.
Explanation
The function of `std::endl` goes beyond just skipping a line; it also flushes the stream, which means that it forces the output buffer to be written out immediately. Using `std::endl` excessively can impact performance because flushing the output buffer frequently can slow down your program.
Using `\n` Escape Sequence
The `\n` character serves as a newline escape sequence in C++. It directly instructs the output stream to move to a new line without flushing the buffer as `std::endl` does.
Code Example
#include <iostream>
int main() {
std::cout << "Hello, World!\n";
std::cout << "This also skips a line.\n";
return 0;
}
In this instance, each message appears on its own line due to the inclusion of `\n` at the end of each statement. This method is efficient for code that does not require immediate output to be displayed.
Explanation
The `\n` character is straightforward and typically more efficient than `std::endl` for merely skipping lines. It does not force a flush of the output buffer, making it suitable for performance-sensitive applications where output speed is important.
Combining `\n` with Other Outputs
You can use multiple `\n` characters in succession to create space between messages. This approach is particularly useful if you want to enhance the visual separation between different sections of output.
Code Example
#include <iostream>
int main() {
std::cout << "Hello, World!\n\n\n";
std::cout << "This line has extra space above." << std::endl;
return 0;
}
In this example, "Hello, World!" is followed by three new line skips, resulting in more visual separation from the next output line, which can enhance clarity.
Explanation
Using multiple `\n` gives you flexibility in formatting output. However, it’s essential to strike a balance and not overdo it, as too much space can make the output appear disjointed or cluttered.

Choosing Between `std::endl` and `\n`
Performance Considerations
While both `std::endl` and `\n` achieve similar results in terms of skipping lines, their implications can differ. Frequent use of `std::endl` can lead to performance bottlenecks due to repeated flushing of the output buffer. In most cases, unless immediate feedback is necessary (e.g., debugging lines or interactive user prompts), prefer using `\n` for simply creating line breaks.
Readability and Consistency
Maintaining consistency in how you handle line breaks is crucial for the maintainability of your code. Opt for one method predominantly throughout your project to ensure that anyone reading your code can understand the style and reasoning behind your formatting choices. It’s a good practice to document these choices so that team members adhere to the same standards.
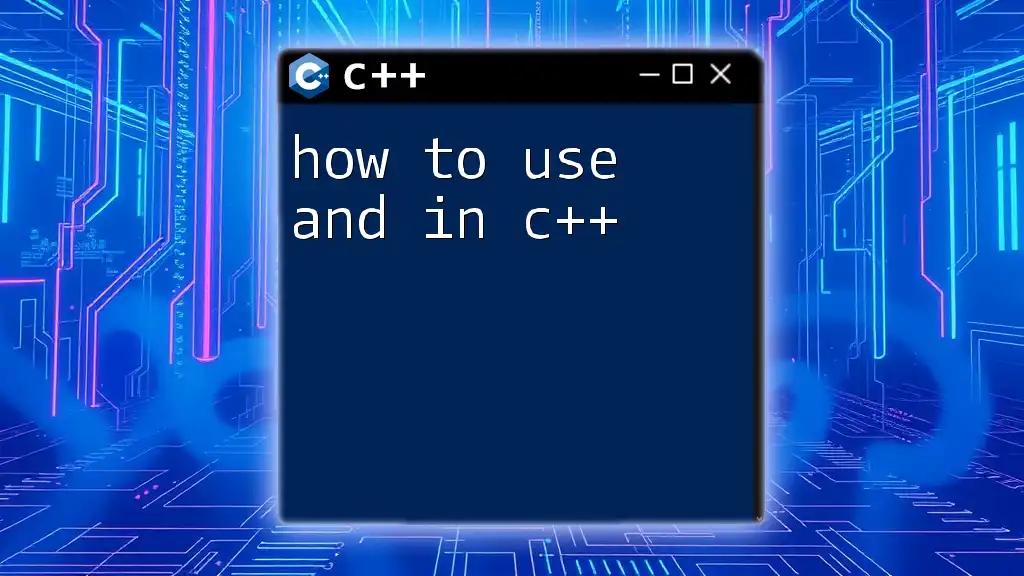
Additional Tips for Formatted Output
Custom Formatting Functions
Creating custom functions to handle output formatting can enhance code modularity and readability. Make a helper function that includes desired output formatting.
Code Example
#include <iostream>
void printWithLineSkip(const std::string& message) {
std::cout << message << std::endl << std::endl;
}
int main() {
printWithLineSkip("Hello, World!");
printWithLineSkip("This uses a custom function.");
return 0;
}
Here, the function `printWithLineSkip` outputs a message along with a line break, encapsulating the behavior you desire in a single reusable function. Using such functions can provide clarity about your program’s intent and significantly simplify complex output requirements.
Using Libraries for Advanced Formatting
For advanced text formatting and output handling, consider exploring libraries like `fmt` or `Boost.Format`. These libraries can provide more robust tools for formatting output as well as enhanced performance and maintainability.
In conclusion, understanding how to skip a line in C++ is vital as it contributes to the overall clarity and quality of your output. Different methods exist for this task, each with its own use-cases and performance implications. Embrace consistent formatting practices to ensure your code remains readable and maintainable through its life cycle.