In C++, you can end a line or output a new line by using the `std::endl` manipulator or the newline character `'\n'` in your output streams. Here's an example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl; // Using std::endl
std::cout << "This is a new line.\n"; // Using '\n'
return 0;
}
Understanding Line Endings in C++
What is a Line Ending?
In programming, a line ending signifies the end of one line of text and the beginning of another. In the context of C++, understanding how to effectively use line endings is essential for creating clear and readable output. Unlike some languages that might allow for automatic line breaks, C++ requires explicit definitions for line endings.
Types of Line Endings in C++
Newline character (`\n`)
The newline character is the most common way to indicate a line break in C++. When you use `\n`, it tells the program to move the cursor to the next line. This is useful when outputting strings or text that should be visually separate.
Carriage Return (`\r`)
Historically, computing systems used the carriage return character as a control character that moved the cursor to the beginning of the line. While it is largely obsolete in modern programming practices, understanding it helps in recognizing how text is formatted across platforms.
Carriage Return followed by Newline (`\r\n`)
Some systems, particularly Windows, utilize both a carriage return and a newline (i.e., `\r\n`) to signify the end of a line. Recognizing this distinction is important when writing programs intended to operate across different operating systems.
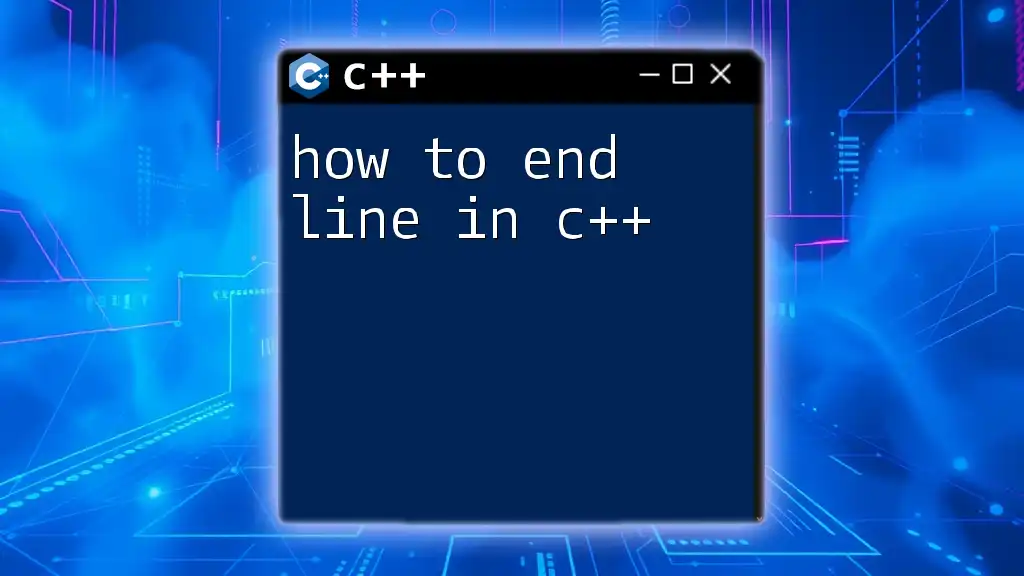
Using Line Endings in C++
Printing with Line Endings
In C++, you typically use `std::cout` to print to the console. There are a couple of straightforward ways to end a line, either with `std::endl` or the newline character `\n`.
Example Code Snippet
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl; // using std::endl
std::cout << "This is a new line." << "\n"; // using \n
return 0;
}
In this example, both approaches effectively create a new line. However, there are slight differences. While `std::endl` not only adds a newline but also flushes the output buffer, using `\n` simply moves the cursor down without flushing. This could affect performance in scenarios involving frequent output.
Ending Lines in Different Situations
Inside Functions
When working inside functions, a clear output is vital for debugging and readability. Always consider how outputs will look once printed.
Example Code Snippet
void printMessage() {
std::cout << "Function says: Hello!" << std::endl;
}
This ensures every call to `printMessage` results in a clean, standalone line of text.
In Loops
When using loops to print multiple lines, proper line endings help maintain clarity.
Example Code Snippet
for(int i = 0; i < 5; i++) {
std::cout << "Count: " << i << "\n";
}
This loop prints each count on a new line, which promotes easier reading of the console output and helps you quickly identify patterns.
Common Mistakes
Forgetting Line Endings
One common error is neglecting to include line endings at appropriate places. This can result in cluttered output where lines run together, making it hard to read.
Mixing Line Ending Characters
Another frequent mistake is the inconsistent use of line-ending characters. Mixing `\n` and `\r\n` can lead to confusion and unexpected results, especially when transferring files between different operating systems.
Example Code Snippet
std::cout << "Line one." << "\r\n" << "Line two."; // incorrect usage
In this example, the mixed use of line endings can create issues with output formatting, especially when expecting consistent results.
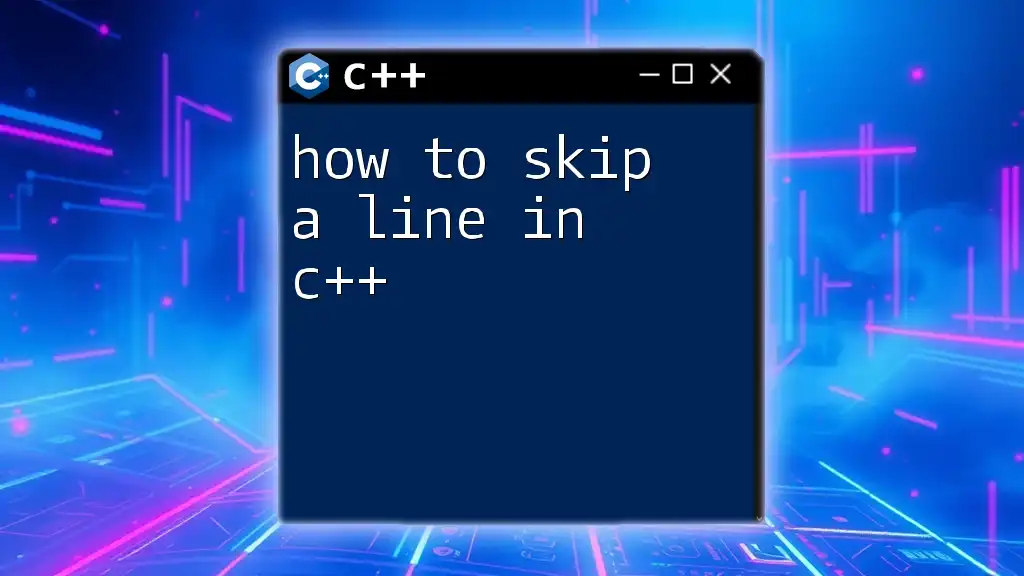
Advanced Techniques
Customizing Line Endings
C++ allows you to define such specifications in your own classes. By implementing a custom printing function, you can set default line endings according to your needs.
Example Code Snippet
class CustomPrinter {
public:
void printWithCustomEnd(const std::string& message, const std::string& ending = "\n") {
std::cout << message << ending;
}
};
Here, the method `printWithCustomEnd` allows you to specify what line ending to use, making your output flexibility a powerful tool.
Using File Streams
Line endings also play a crucial role when outputting text to files. Managing line endings carefully becomes important to ensure cross-platform compatibility.
Example Code Snippet
#include <fstream>
int main() {
std::ofstream outFile("output.txt");
outFile << "Hello, File!" << std::endl;
outFile.close();
}
In this example, the newline character used will influence how text is parsed by other programs, particularly those sensitive to line endings, like text editors.
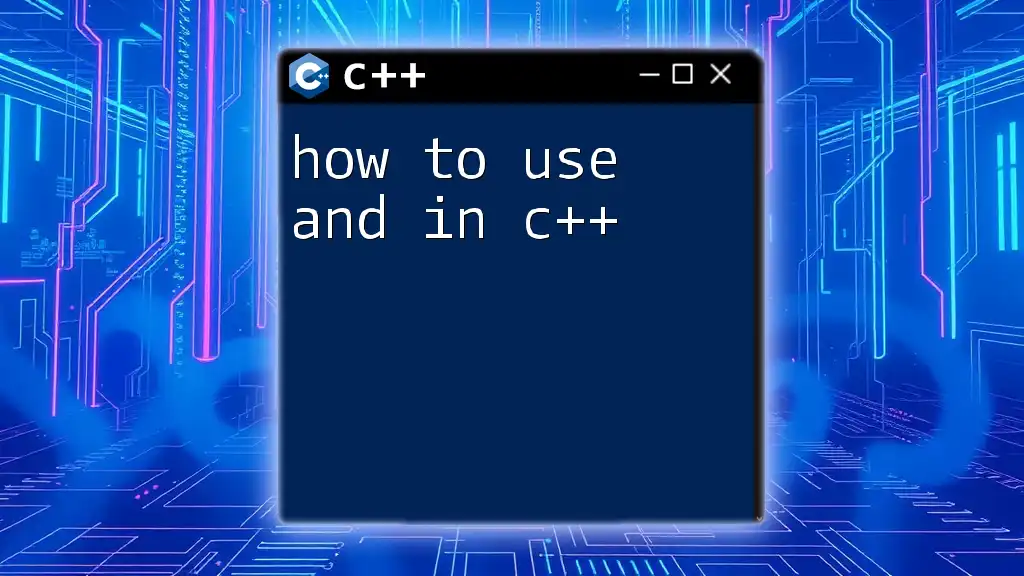
Conclusion
Understanding how to end a line in C++ is a foundational skill that affects not only the output quality of your programs but also user experience. Proper usage ensures your code remains clean, understandable, and efficient.
Encourage best practices by consistently defining how you use line endings, depending on the context in which they are applied. Mastering this simple yet crucial aspect of C++ programming will enhance your coding proficiency and drive towards writing cleaner code.