In C++, you can calculate the power of a number using the `pow` function from the `<cmath>` library, which takes two arguments: the base and the exponent.
#include <iostream>
#include <cmath>
int main() {
double base = 2.0;
double exponent = 3.0;
double result = pow(base, exponent);
std::cout << base << " raised to the power of " << exponent << " is " << result << std::endl;
return 0;
}
Understanding Powers in Mathematics
Exponentiation is a fundamental mathematical operation which involves raising a number to the power of another, known as the base and the exponent. Specifically:
- Base: The number that you want to raise to a certain power (e.g., \(2\) in \(2^3\)).
- Exponent: The power to which the base is raised (e.g., \(3\) in \(2^3\)).
- Result (Power): The output of the exponentiation operation (e.g., \(8\) in \(2^3 = 8\)).
Understanding this concept is crucial for implementing power calculations in any programming language like C++.
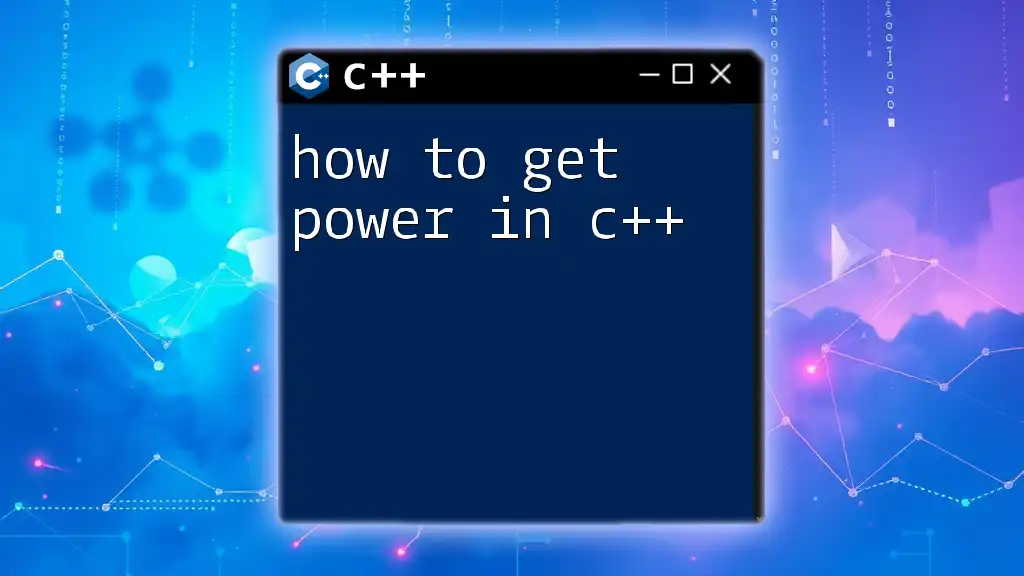
Methods to Do Powers in C++
There are several approaches to calculate powers in C++. Let’s explore the most common methods you can use.
Using the pow() Function from cmath
The `<cmath>` library in C++ provides the `pow()` function, which is a straightforward way to perform exponentiation. Here’s how to use it:
To include the functions from this library, simply add:
#include <cmath>
Syntax of the `pow()` function:
double pow(double base, double exponent);
Example:
#include <iostream>
#include <cmath>
int main() {
double base = 2.0;
double exponent = 3.0;
double result = pow(base, exponent);
std::cout << base << " raised to the power of " << exponent << " is " << result << std::endl;
return 0;
}
Explanation of Output
In the example above, when you run the program, it prints:
`2 raised to the power of 3 is 8`.
This demonstrates how easy it is to perform power calculations using the built-in `pow()` function.
Implementing Custom Power Function
Although `pow()` is convenient, you might want to implement your own power functions for greater flexibility or specific conditions. Here's how you can do this.
Why Create a Custom Function?
There are several reasons to consider writing a custom power function:
- Flexibility in control flow
- Optimization for specific use cases
- Better handling of edge cases
Recursive Version
You can create a simple recursive function for power calculations like this:
double power(double base, int exponent) {
if (exponent == 0)
return 1;
else if (exponent < 0)
return 1 / power(base, -exponent);
else
return base * power(base, exponent - 1);
}
int main() {
double result = power(2.0, 3);
std::cout << "Result: " << result << std::endl;
return 0;
}
Iterative Version
Alternatively, you can also create an iterative version of a power function, which may be more efficient for large exponents:
double power_iterative(double base, int exponent) {
double result = 1.0;
for (int i = 0; i < exponent; i++) {
result *= base;
}
return result;
}
int main() {
double result = power_iterative(2.0, 3);
std::cout << "Result: " << result << std::endl;
return 0;
}
Discussion on Performance
When evaluating performance between recursive and iterative methods, consider the following:
- Recursive functions: They are often easier to read and write but can lead to stack overflow if the exponent is too large.
- Iterative functions: They generally use less memory and are often faster as they do not involve the overhead of multiple function calls.
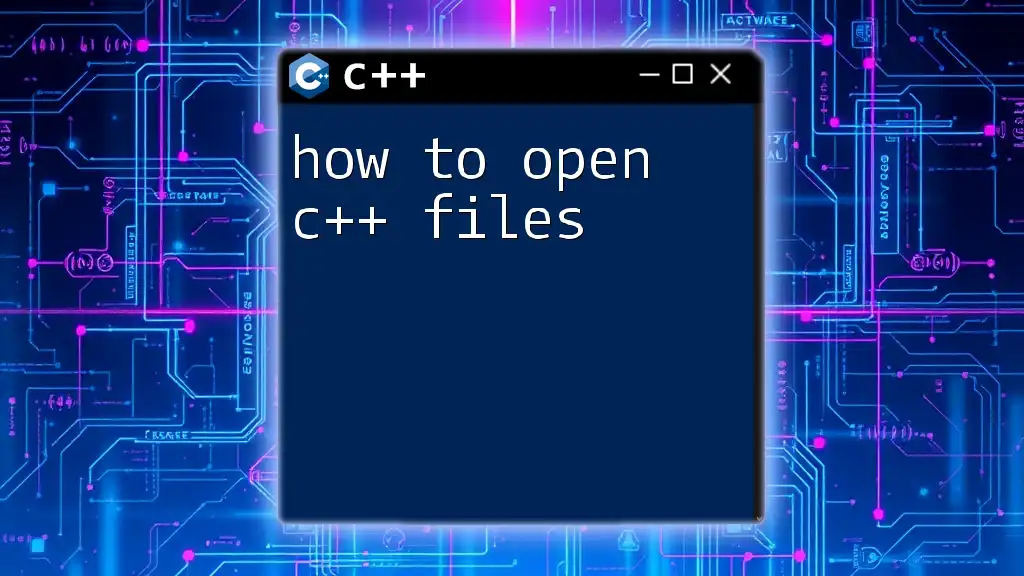
Handling Edge Cases in Power Calculations
When you calculate powers, it is important to handle certain edge cases to ensure accuracy and robustness in your implementations.
Negative Exponents
The interpretation of a negative exponent is that the base raised to the negative of an exponent equals the reciprocal:
\[ x^{-n} = \frac{1}{x^n} \]
This is appropriately handled in both the recursive and iterative implementations mentioned earlier.
Zero Exponent
A common rule in mathematics is that anything raised to the power of zero is equal to one. This behavior is built into the previously mentioned power functions, ensuring that they return the correct result when given \(0\) as the exponent.
Edge Cases
Considerations for edge cases might include:
-
Negative bases: Depending on the exponent’s parity,
- A negative base raised to an even exponent yields a positive result.
- A negative base raised to an odd exponent yields a negative result.
-
Non-integer Exponents: When working with floating-point numbers as exponents, it is essential to check if the base is non-negative since, mathematically, raising a negative base to a fractional power can lead to complex results.
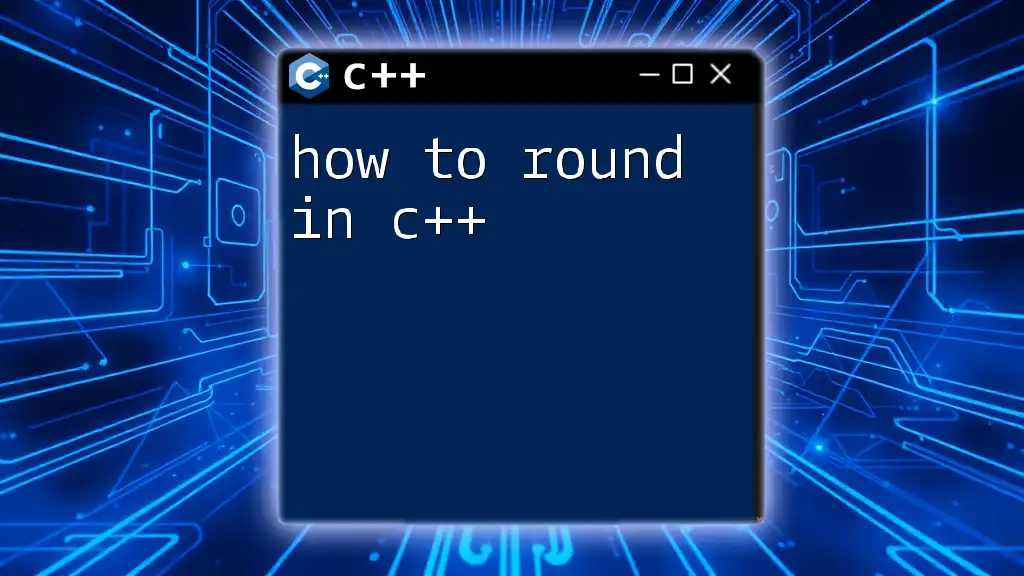
Conclusions
In this article, we explored how to do power in C++ using built-in functions and custom implementations. We learned how to use the `pow()` function from the `<cmath>` library, as well as how to create both recursive and iterative functions for your own calculations.
By understanding the importance of handling edge cases, you are well-prepared to implement power functionalities consistent with mathematical principles.
Final Tips
When working with powers in C++, always strive for optimality and clarity. Choose the method that best suits the problem context, and ensure that edge cases are well managed for accurate results. Stay curious, and explore more functionalities to elevate your C++ programming skills.