Pointers in C++ are variables that store the memory address of another variable, allowing for efficient manipulation of memory and data structures. Here's a simple example:
#include <iostream>
using namespace std;
int main() {
int var = 42; // Declare an integer variable
int* ptr = &var; // Declare a pointer and initialize it to the address of var
cout << "Value of var: " << var << endl; // Output the value of var
cout << "Value of ptr: " << *ptr << endl; // Output the value at the address stored in ptr
return 0;
}
What are Pointers?
Definition of Pointers
Pointers are special variables that store memory addresses instead of data values. Unlike regular variables that hold a specific data type (like integer or character), pointers refer directly to the location in memory where the data is stored. This ability to reference memory locations is a powerful feature of C++, enabling efficient memory management and manipulation.
Pointer Syntax in C++
In C++, declaring a pointer involves using the `*` operator. The syntax for declaring a pointer is straightforward. For example, if you want to create a pointer that points to an integer, you would declare it as follows:
int* ptr; // Pointer to an integer
Using pointers effectively often requires understanding their syntax intimately, as they will be essential for tasks related to memory management and data manipulation throughout your coding experience.
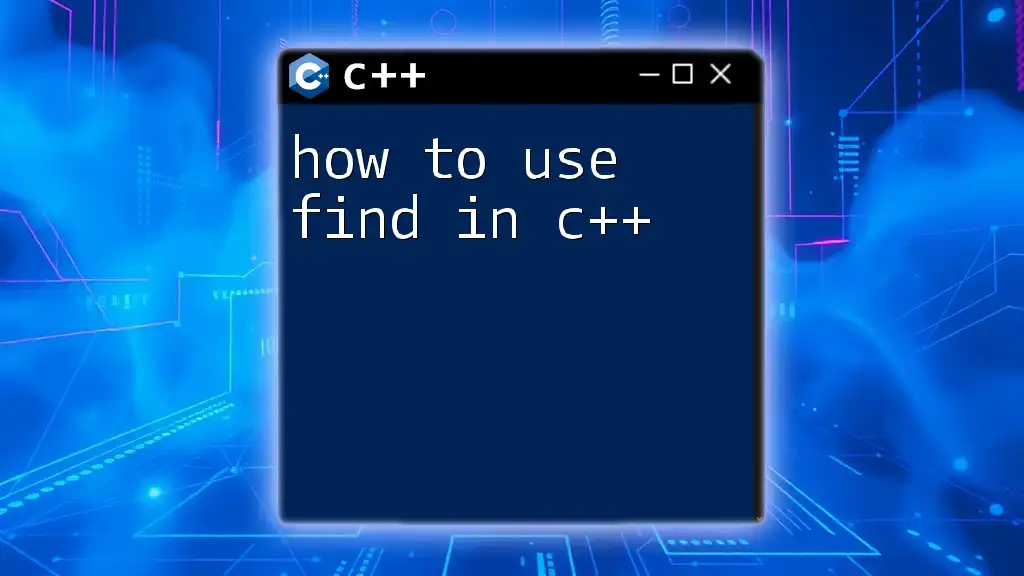
How to Declare and Initialize Pointers
Pointer Declaration
To declare a pointer, you need to specify the type of data it will point to, followed by an asterisk `*`. Here’s how you can declare pointers of different types:
float* floatPtr; // Pointer to float
char* charPtr; // Pointer to char
double* doublePtr; // Pointer to double
Initializing Pointers
After declaring a pointer, you can initialize it using the address-of operator `&`, which fetches the memory address of a variable. For instance, if you have an integer variable `num`, you can initialize a pointer to point to its address like so:
int num = 5;
int* ptr = # // Pointer initialized to the address of num
This means that `ptr` now holds the memory address of the variable `num`, allowing indirect access to its value.
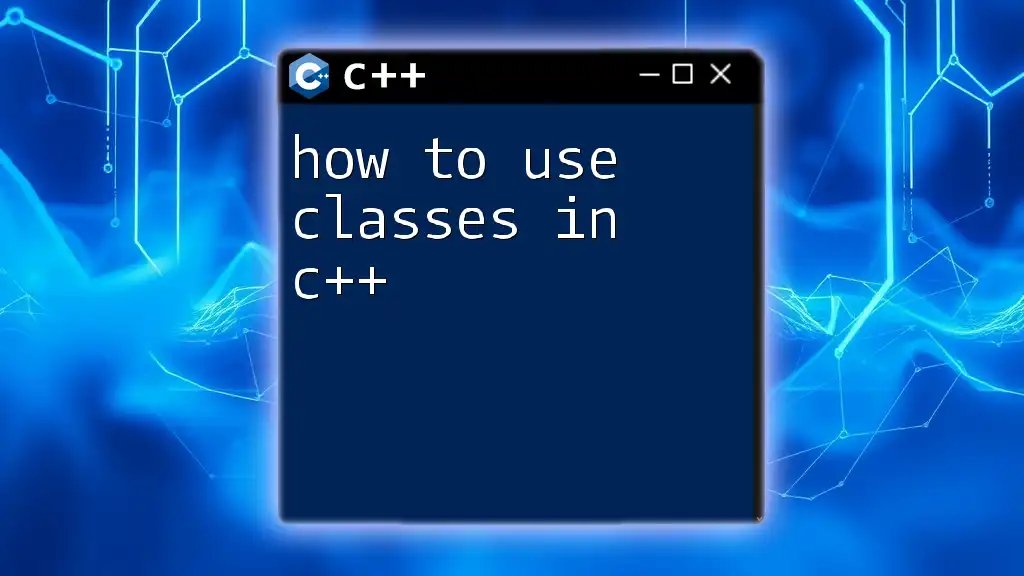
Dereferencing Pointers
What is Dereferencing?
Dereferencing a pointer means accessing the value located at the memory address that the pointer is referencing. This is accomplished using the dereference operator `*`. For example:
int value = *ptr; // value is now 5
Here, `value` will receive the integer stored in the address that `ptr` points to.
Changing Values Using Dereferencing
You can also modify the value of a variable through its pointer by dereferencing it and assigning a new value. For example:
*ptr = 10; // num is now 10
After this operation, the value of `num` becomes 10, demonstrating how pointers can be used to manipulate data indirectly.
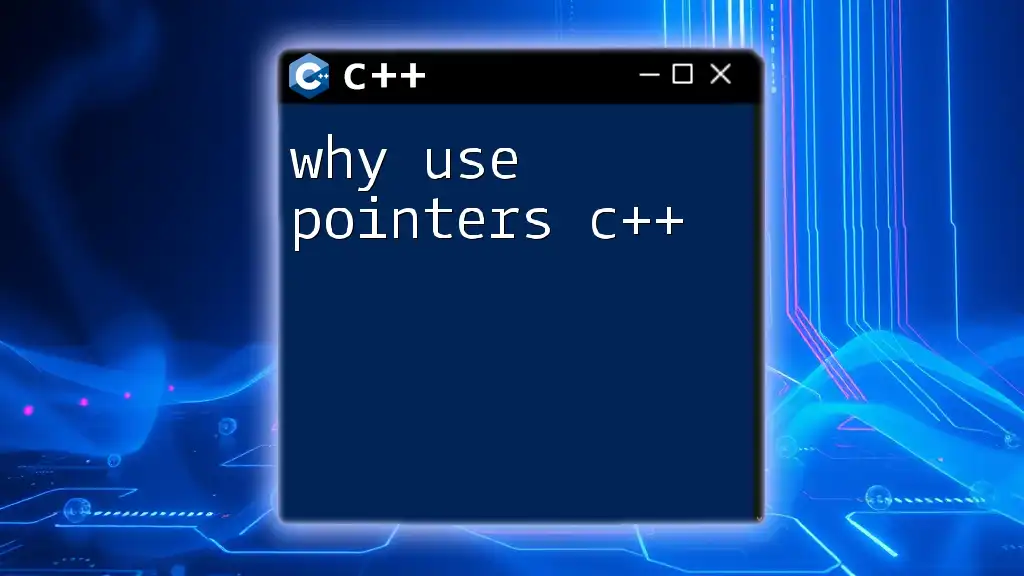
Pointer Arithmetic
Introduction to Pointer Arithmetic
Pointer arithmetic allows you to perform calculations using pointers, making it useful for iterating through arrays and manipulating memory locations efficiently. When you increment a pointer, it points to the next memory location based on its data type size.
Examples of Pointer Arithmetic
Incrementing pointers is simple. For instance, consider an array:
int arr[] = {1, 2, 3};
int* p = arr; // Points to the first element of the array
p++; // Now points to the second element (2)
In this scenario, when you increment `p`, it moves to the next integer in memory, so it now points to the value `2`.
You can also access elements directly through pointer offsets:
int secondValue = *(p + 1); // Accesses the third element (3)
This demonstrates how pointer arithmetic enables seamless navigation through arrays.
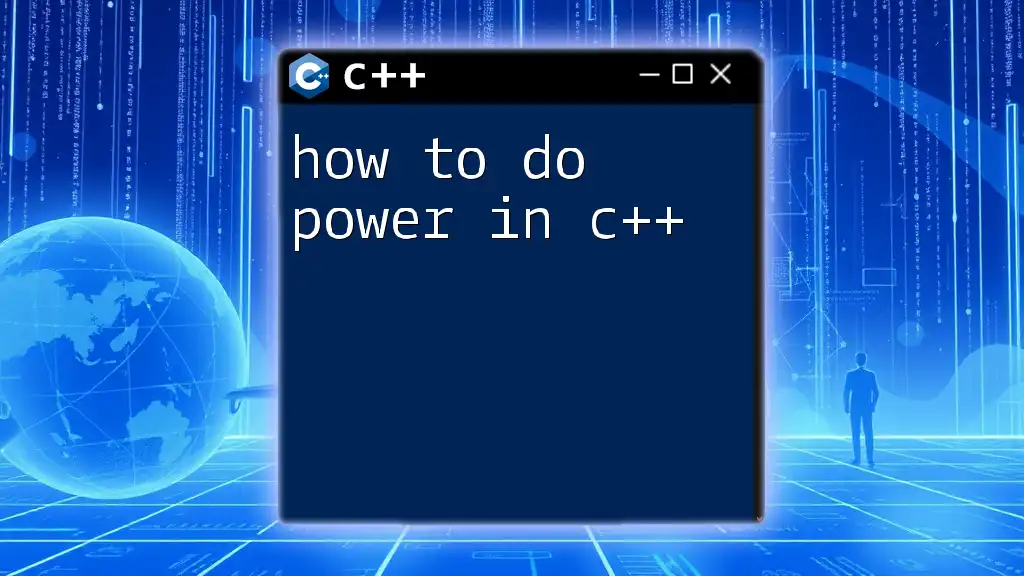
Pointers and Arrays
Relationship Between Pointers and Arrays
In C++, arrays are closely related to pointers. When an array is declared, the name of the array acts as a pointer to its first element. This means that you can manipulate arrays using pointers efficiently.
Example of Using Pointers with Arrays
Here is an example that demonstrates how to use pointers in conjunction with arrays:
int arr[] = {10, 20, 30};
int* arrPtr = arr; // No need for & operator
for(int i = 0; i < 3; i++) {
cout << *(arrPtr + i) << endl; // Output: 10, 20, 30
}
In this loop, `arrPtr` queries the array using pointer arithmetic, showing the power and efficiency of pointers with arrays.
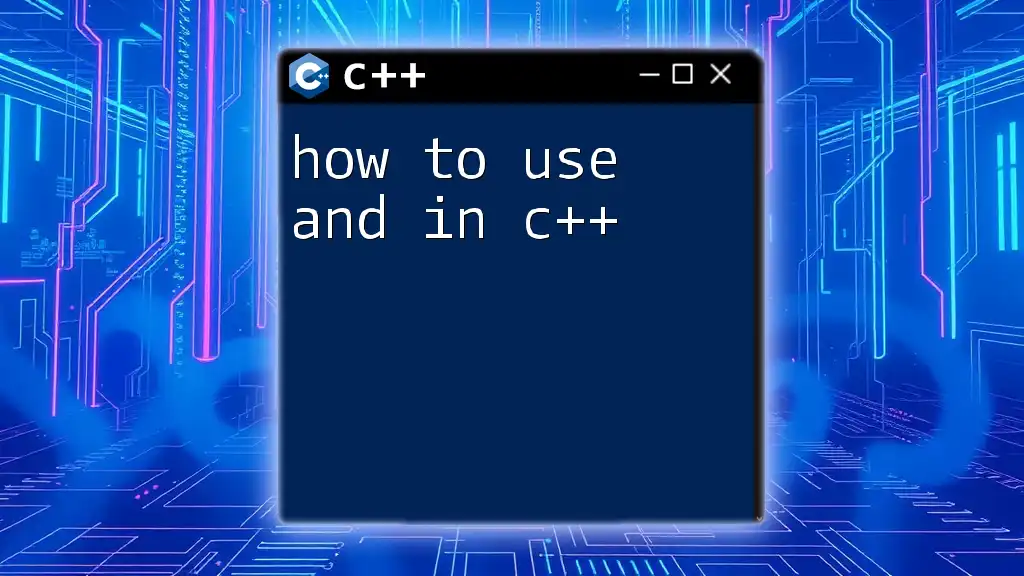
Pointers and Functions
Passing Pointers to Functions
Pointers can also be passed to functions, allowing functions to modify the original variable directly. This technique reduces memory usage and enhances performance, especially when dealing with large structures or objects.
Example Function Using Pointers
Below is an illustrative example of a function that increments a variable using pointers:
void increment(int* ptr) {
(*ptr)++;
}
int main() {
int num = 5;
increment(&num); // num is now 6
}
In this case, the `increment` function receives a pointer to `num`, enabling it to modify `num` directly.
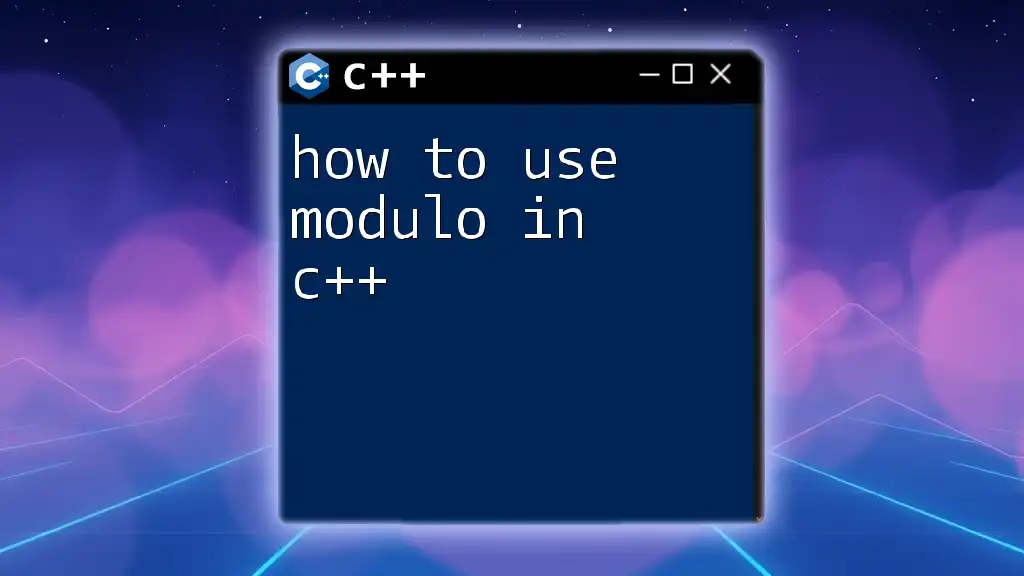
Dynamic Memory Allocation
What is Dynamic Memory?
Dynamic memory allocation allows you to request memory at runtime using pointers. This method is essential when you do not know how much memory you will need ahead of time.
Using `new` and `delete` Operators
In C++, you allocate memory dynamically using the `new` operator and release it with the `delete` operator to prevent memory leaks. Here’s how you do it:
int* dynamicArray = new int[5]; // Allocates an array of 5 integers
delete[] dynamicArray; // Deallocating memory
In this example, `dynamicArray` can store five integers, dynamically allocated during runtime.
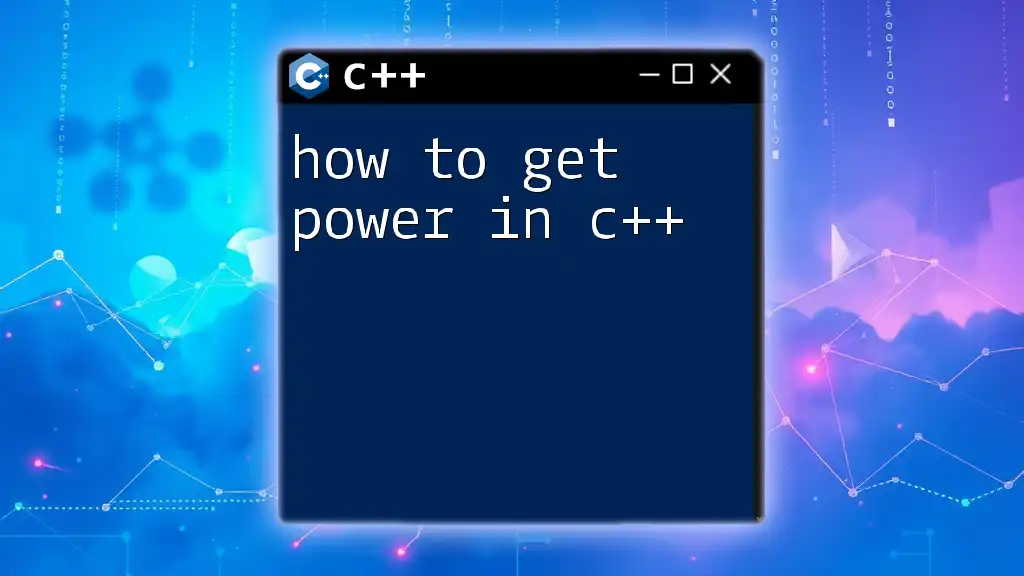
Common Pointer Pitfalls
Null Pointers
A null pointer is a pointer that does not point to any valid memory location. It is a good practice to initialize pointers to `nullptr` to avoid undefined behavior.
int* ptr = nullptr; // Safe initialization
Dangling Pointers
Dangling pointers occur when a pointer still points to a memory location that has been deallocated. Avoiding dangling pointers is crucial for maintaining memory integrity.
int* danglingPtr = new int;
delete danglingPtr; // danglingPtr now points to deallocated memory
In this case, after `delete`, `danglingPtr` should no longer be used, as it points to an invalid location.
Memory Leaks
Memory leaks happen when dynamically allocated memory is not properly deallocated, leading to wasted resources. Always ensure you free dynamically allocated memory using `delete` or `delete[]` to prevent memory leaks.
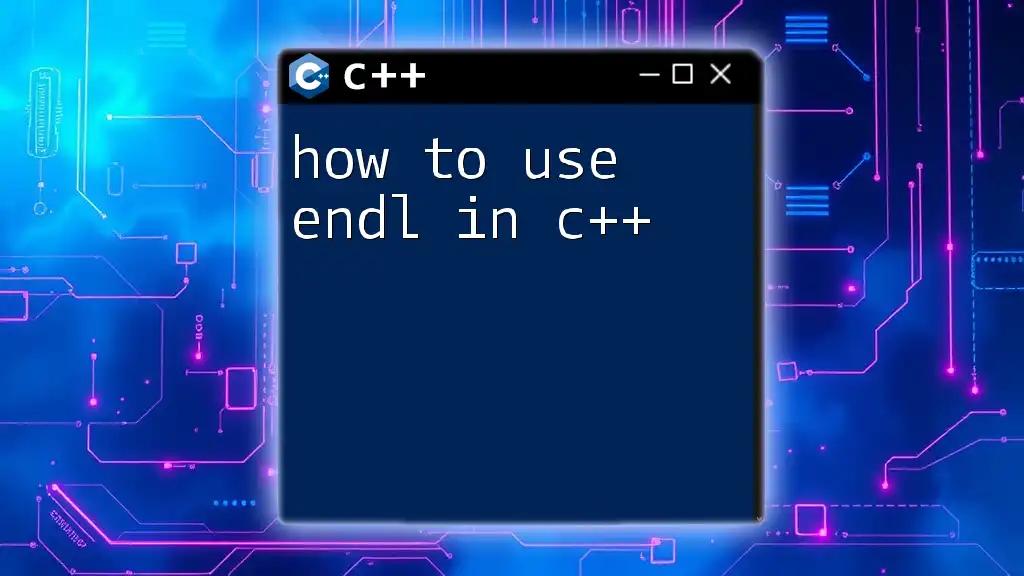
Conclusion
In summary, mastering how to use pointers in C++ is crucial for effective programming in the language. Pointers not only enable you to manage memory more efficiently but also enhance your ability to implement complex data structures and algorithms. By practicing these concepts and examples, you will gain confidence in using pointers effectively in your C++ applications.