To use `ifstream` in C++ for reading data from files, you need to include the `<fstream>` header, create an `ifstream` object, and utilize its methods to open and read from a specified file.
Here’s a simple example:
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream inputFile("example.txt");
std::string line;
if (inputFile.is_open()) {
while (getline(inputFile, line)) {
std::cout << line << std::endl;
}
inputFile.close();
} else {
std::cout << "Unable to open file";
}
return 0;
}
What is ifstream?
`ifstream` stands for "input file stream," and it is used in C++ to read data from files. Understanding how to use `ifstream` is crucial for file handling, as it provides an easy way to access and manipulate external data sources. File streams in C++ are part of the `<fstream>` library and allow for efficient reading operations.
It's essential to distinguish between different stream types in C++. While `ifstream` is dedicated to reading from files, `ofstream` is used for writing to files, and `fstream` can handle both reading and writing operations. Knowing this difference clarifies how to effectively utilize streams in various scenarios.
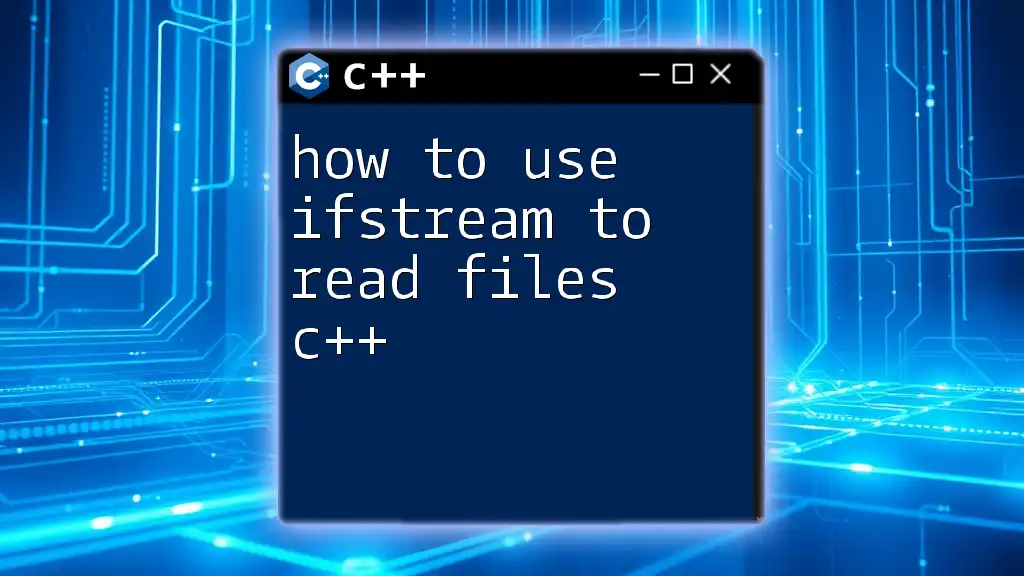
Setting Up an ifstream Example
Including Necessary Headers
Before you can use `ifstream`, you'll need to include the appropriate header files in your program. This is how you do it:
#include <iostream>
#include <fstream>
#include <string>
- `<iostream>` is included for input/output stream operations.
- `<fstream>` is where `ifstream` and `ofstream` are defined.
- `<string>` is included for handling string variables.
Creating an ifstream Object
To read from a file, you first need to declare an `ifstream` object. Here’s the syntax for creating an `ifstream` object:
std::ifstream inputFile;
This statement creates an instance of `ifstream` named `inputFile`, which you will use to perform the file reading operations.
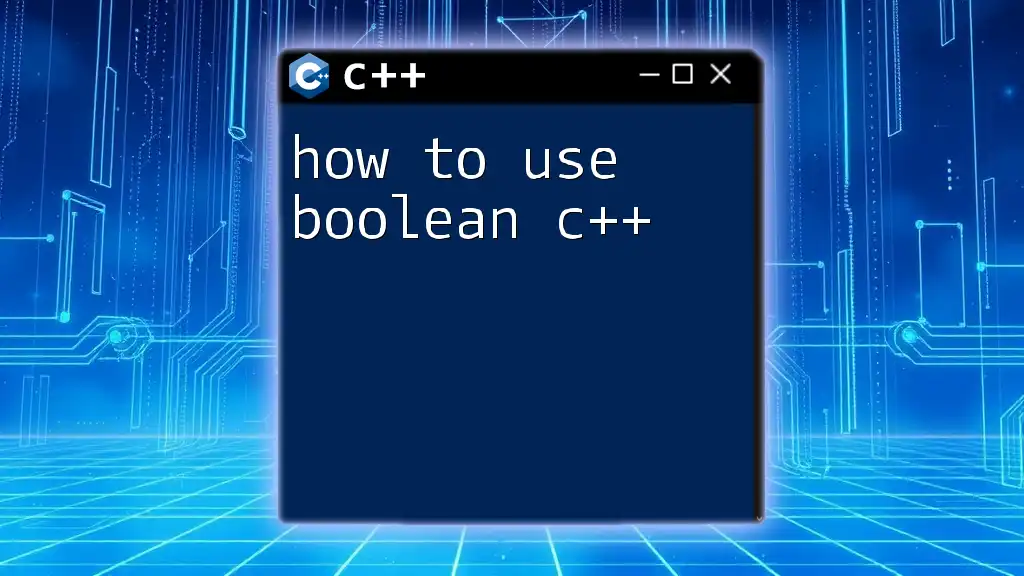
How to Open a File Using ifstream
Opening a File
To read data from a file, you must first open it using the `open` method associated with the `ifstream` object. The basic syntax looks like this:
inputFile.open("example.txt");
You can supply either a relative or an absolute path. If you provide a relative path, ensure your working directory contains the specified file. If the file does not exist or cannot be opened, future read operations will fail.
Checking if the File is Open
After attempting to open a file, it's critical to verify whether the file is successfully opened. You can do this by checking if the `ifstream` object is open:
if (!inputFile.is_open()) {
std::cerr << "Failed to open the file." << std::endl;
}
This check helps in identifying any issues with file access, such as permission problems or incorrect paths, allowing you to address these before attempting to read data.
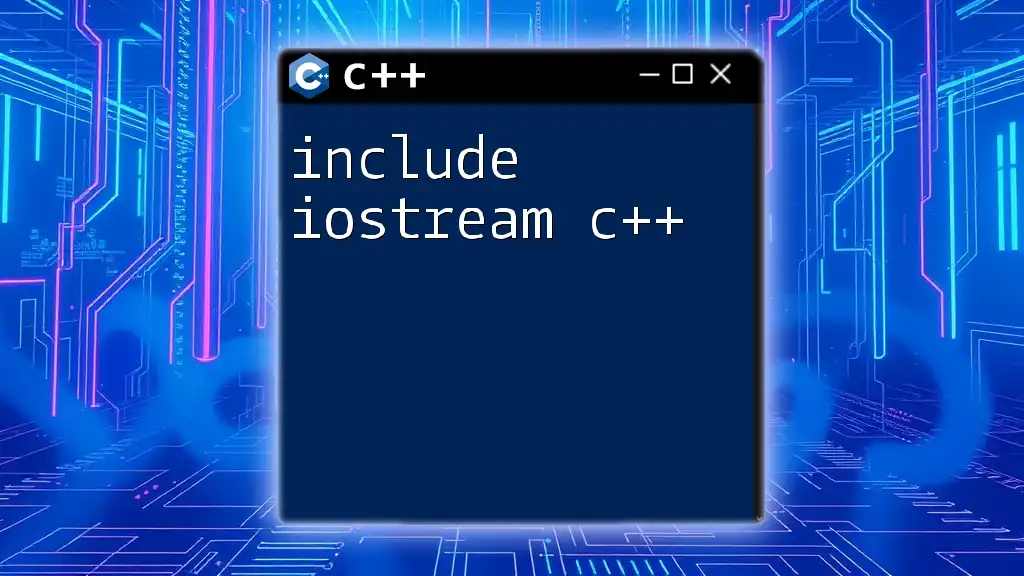
Reading Data from a File
Using getline to Read Strings
One of the simplest ways to read lines from a file is by using the `getline` function. It reads a whole line and stores it in a string variable. Here is an example:
std::string line;
while (getline(inputFile, line)) {
std::cout << line << std::endl;
}
In this code snippet, `getline` reads each line of the file until the end is reached, allowing you to process each line individually. It effectively handles spaces within the line, making it a versatile choice for reading formatted text.
Reading Data Types
You can also read different data types directly from the file stream using the extraction operator `>>`. For example, to read an integer:
int number;
inputFile >> number;
This code will extract the next integer from the file's content and store it in the `number` variable. `ifstream` automatically handles whitespace, meaning it skips over spaces and newlines when searching for input.
Handling Errors During Reading
It's vital to maintain data integrity while reading files. If there is an error during the reading process, you will want to catch it. You can do this by checking the input state after a read operation:
if (inputFile.fail()) {
std::cerr << "Error reading data from file." << std::endl;
}
By addressing errors right after attempting to read, you can gracefully manage issues such as unexpected formats or corrupted data.
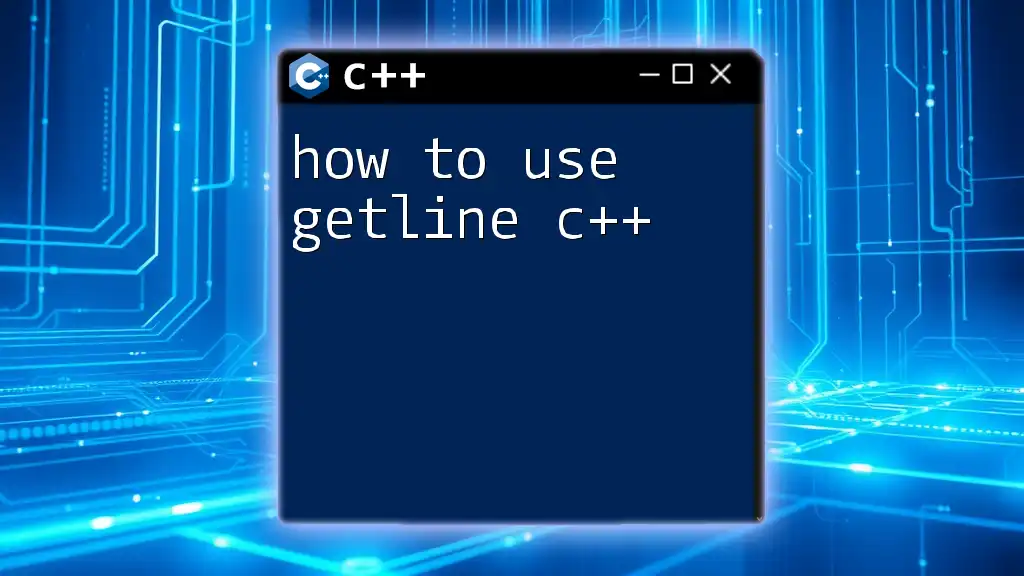
Closing the ifstream Object
Why is it Important to Close a File?
When you're done with a file, it's essential to close it to free up system resources and ensure that all the data has been flushed and written correctly. Neglecting to close files can lead to memory leaks and other issues in larger applications.
How to Properly Close an ifstream
Closing an `ifstream` is straightforward and can be accomplished using the `close` method like so:
inputFile.close();
This command helps manage your resources efficiently, avoiding potential issues associated with open file handles.
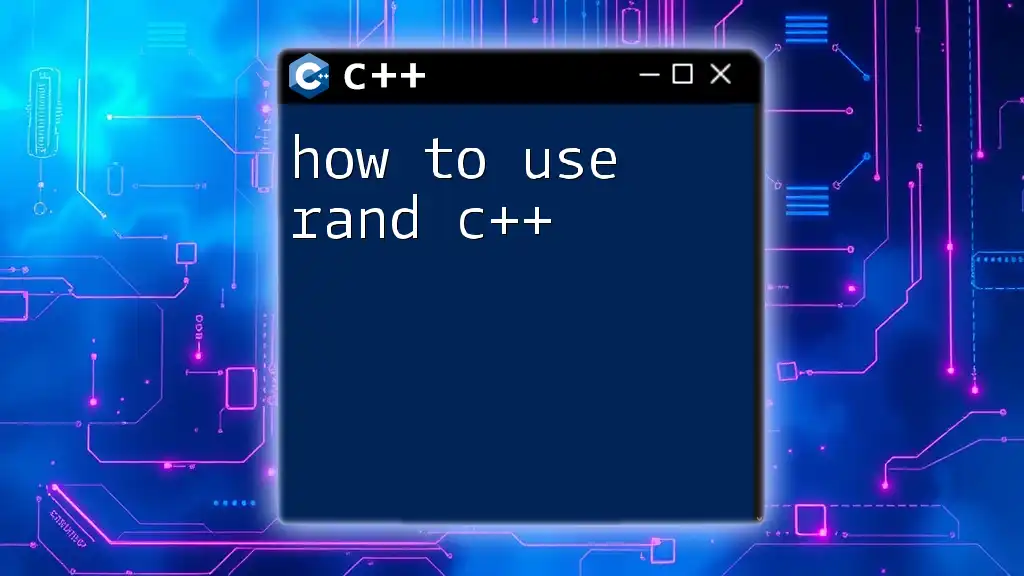
Complete ifstream C++ Example
Bringing all the concepts together, here’s a complete example that demonstrates how to use `ifstream` in C++:
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream inputFile("example.txt");
if (!inputFile.is_open()) {
std::cerr << "Failed to open the file." << std::endl;
return 1;
}
std::string line;
while (getline(inputFile, line)) {
std::cout << line << std::endl;
}
inputFile.close();
return 0;
}
In this example, you see how to incorporate multiple functionalities of `ifstream`, from opening a file to reading and closing it. This serves as a foundational template for file reading in your C++ projects.
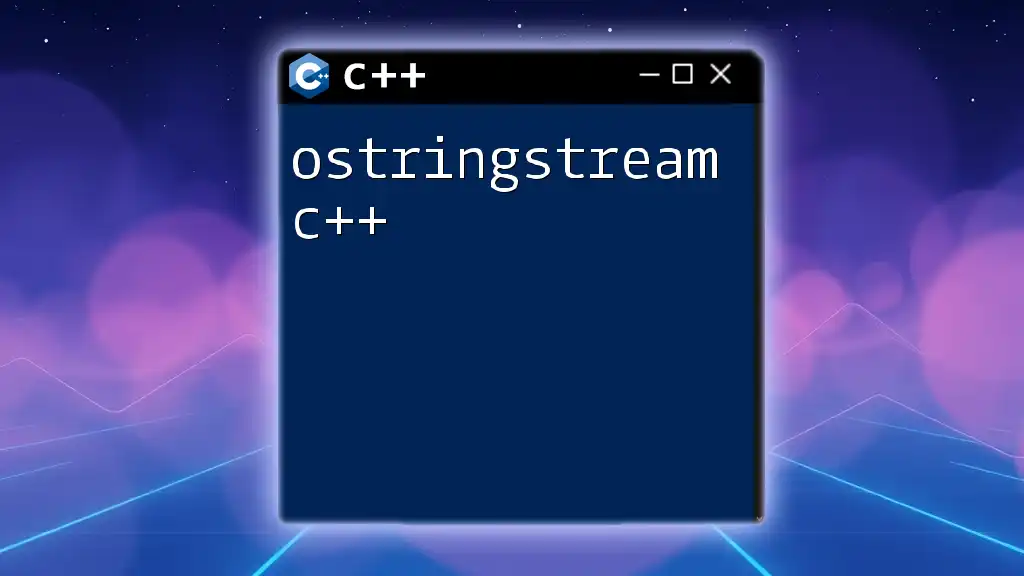
Common Issues and Troubleshooting Tips
Common File Opening Issues
Issues such as missing files or permission problems are frequent when working with files. Always ensure the path is correct and that the file has the necessary read permissions. If you encounter issues, revisiting the file's location and validating its accessibility can solve many problems.
Handling Empty Files
An empty file can lead to confusion during read operations. Before processing, you can check the state of the file stream:
if (inputFile.peek() == std::ifstream::traits_type::eof()) {
std::cerr << "The file is empty." << std::endl;
}
This snippet allows you to conveniently handle empty files and provide user feedback.
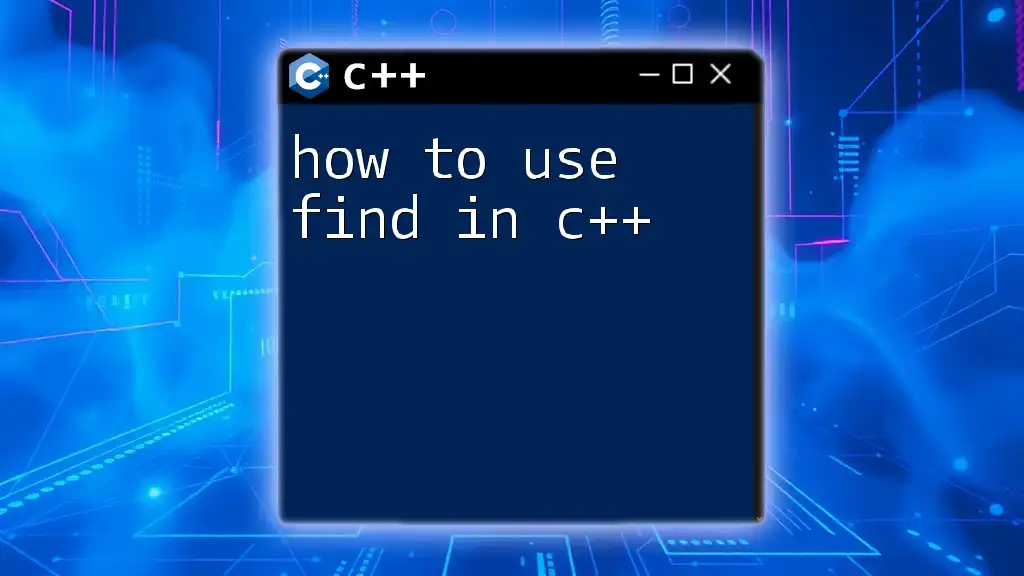
Conclusion
Mastering how to use `ifstream` in C++ is pivotal for file handling and plays a crucial role in many applications. By understanding the structure of file streams, you can read data efficiently, handle errors adeptly, and manage resources effectively. I encourage you to experiment with `ifstream` using different file formats to deepen your understanding of file operations in C++.
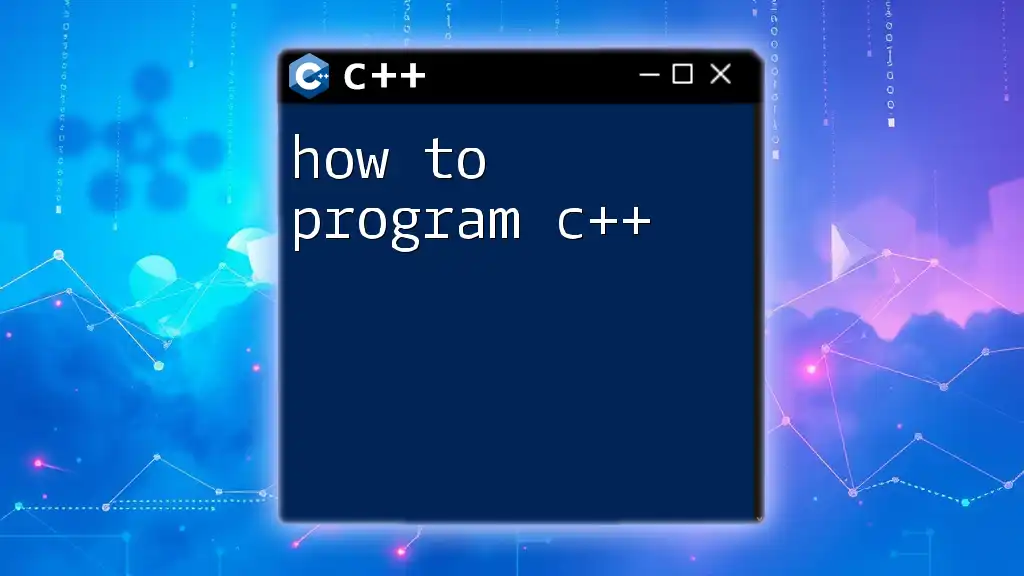
Additional Resources
For further reading, check the official C++ documentation on file streams, and consider online courses that delve deeper into C++ programming concepts. Practice is key to grasping these techniques, so explore various file-handling scenarios to improve your skills.