To use `llama.cpp`, you need to include the relevant headers, configure the model, and input your prompts for processing; here’s a quick example of how to load a model and generate a completion:
#include "llama.h"
int main() {
Llama::Model model("path/to/model");
std::string response = model.generate("What is artificial intelligence?");
std::cout << response << std::endl;
return 0;
}
What is llama.cpp?
Overview of llama.cpp
llama.cpp is a utility designed for implementing the LLaMA (Large Language Model Meta AI), which enables developers to leverage advanced natural language processing capabilities within their C++ applications. By providing a streamlined interface and a collection of optimized commands, llama.cpp allows programmers to integrate sophisticated AI functionalities into their projects with ease.
Features of llama.cpp
One of the standout features of llama.cpp is its lightweight and efficient performance, making it an ideal choice for applications that require speed and responsiveness. Here are some key features:
- C++ Compatibility: Designed with C++ in mind, allowing for seamless integration with existing C++ codebases.
- User-Friendly Interface: Simplifies the complexity of neural network interactions, making the commands easy to understand and implement.
- Extensible Architecture: Facilitates the addition of custom features and plugins, enhancing functionality without compromising performance.
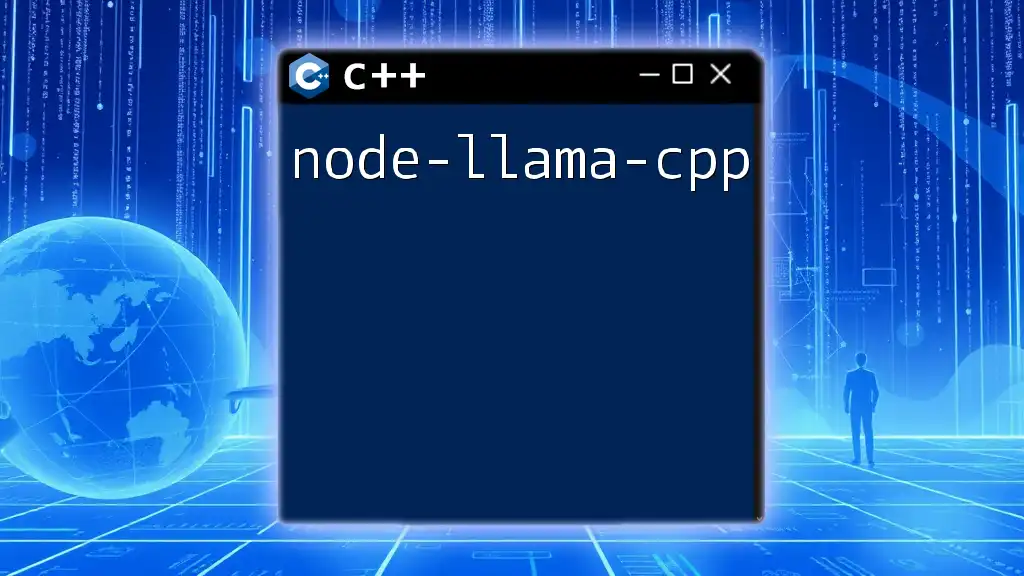
Getting Started with llama.cpp
Installation Prerequisites
System Requirements
Before diving into how to use llama.cpp, it’s essential to ensure that your system meets the basic requirements. Generally, you will need:
- A modern computer with at least 8GB of RAM.
- A compatible C++ compiler, such as GCC or Clang.
- Proper system libraries to support C++ compilation.
Installation Steps
Getting started with llama.cpp requires a few simple installation steps. Here’s how to do it:
- Clone the Repository:
You can clone the llama.cpp repository directly from GitHub:
git clone https://github.com/yourusername/llama.cpp.git
- Navigate to the Directory:
Change your current directory to the llama.cpp folder:
cd llama.cpp
- Compile the Project:
Use the `make` command to compile the project:
make
After these steps, you should have a functional version of llama.cpp installed on your system.
Setting Up Your Development Environment
Using llama.cpp effectively requires compatibility with your chosen development environment. Here are some popular IDEs optimized for C++, along with setup tips:
- Visual Studio: Create a new C++ project and link the llama.cpp library in your project settings.
- CLion: Use CMake to manage your project dependencies, ensuring llama.cpp is included.
- Code::Blocks: Set up the workspace to include llama.cpp and adjust compiler settings as needed.
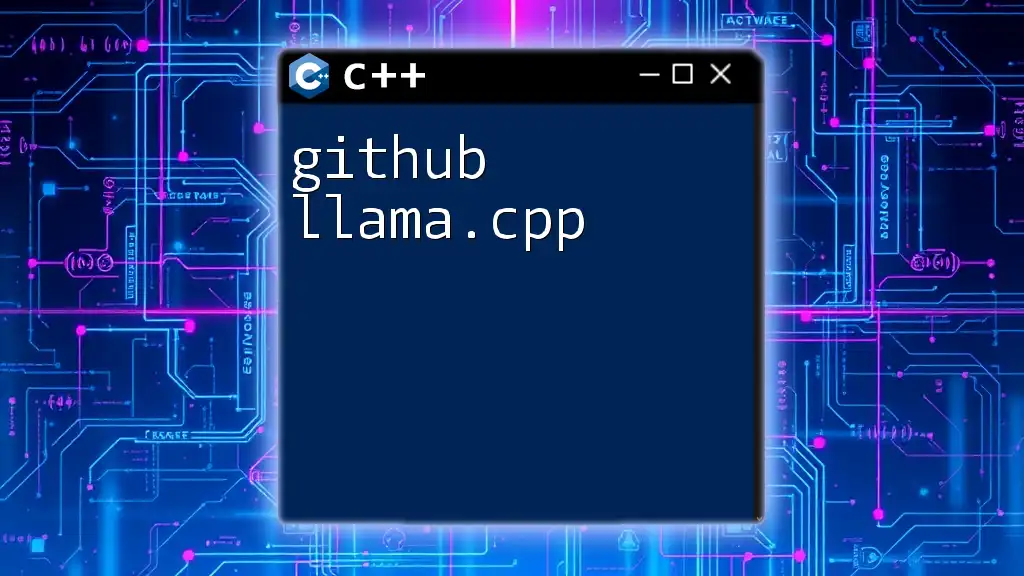
Basic Commands in llama.cpp
Understanding the Command Structure
The syntax of llama.cpp commands follows a clear and concise structure, ensuring ease of use. Commands generally start with the object instance followed by the specific method to invoke, like so:
model.method_name(parameters);
Commonly Used Commands
Command 1: Initializing LLaMA
To utilize the LLaMA model, you first need to initialize it. This sets up the model with the appropriate configurations. Example:
LlamaModel model;
model.initialize("model/path");
This command points the model to the directory where the LLaMA files are stored.
Command 2: Text Generation
One of the primary features of llama.cpp is generating text based on prompts. The command to generate text looks like this:
std::string result = model.generate("Prompt text");
The "Prompt text" acts as input for the model, and result will contain the generated output.
Command 3: Setting Parameters
Users can customize the model's behavior by adjusting parameters like temperature and max tokens. Here’s how to set these parameters:
model.set_parameters({{"temperature", 0.7}, {"max_tokens", 100}});
This command configures the model to have a moderate level of creativity while capping the output length.
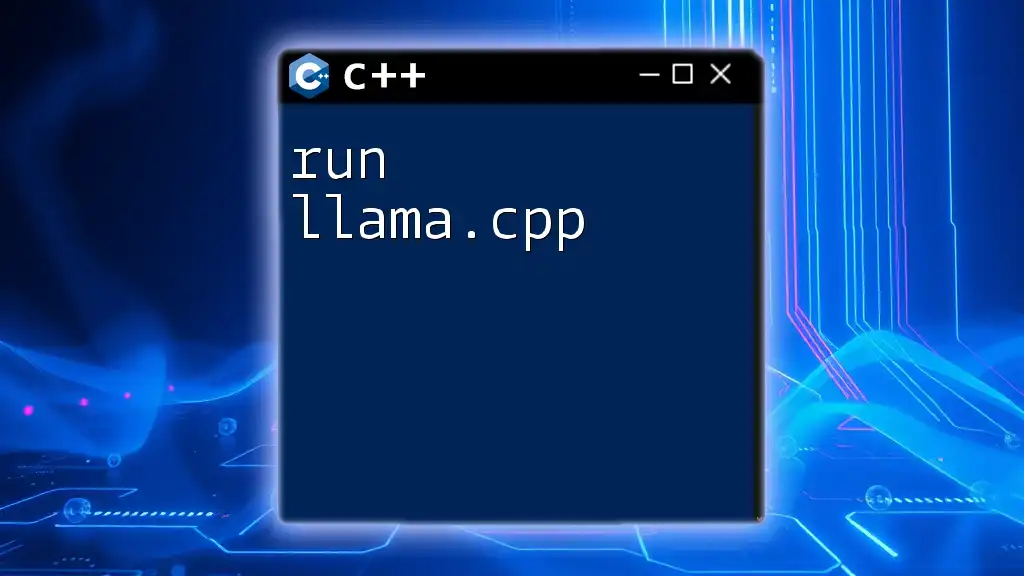
Advanced Usage of llama.cpp
Customizing Your LLaMA Model
Fine-tuning with User Data
Fine-tuning a LLaMA model enables it to better respond to specific types of input, enhancing its performance for particular applications. Here’s a basic outline of how to fine-tune:
- Gather your dataset.
- Use the llama.cpp fine-tuning function (code example omitted for brevity) to adjust the model with your data.
Incorporating Additional Features
The extensibility of llama.cpp allows developers to integrate additional features or plug-ins. This modularity means you can use libraries or frameworks that add specific functionalities, such as data preprocessing or sentiment analysis.
Performance Optimization
To maximize the performance of your llama.cpp implementations, consider the following tips:
- Memory Usage: Monitor and optimize memory consumption by limiting the size of loaded models and managing data structures effectively.
- Batch Processing: Instead of processing one input at a time, consider using batch processing to improve throughput.
- Asynchronous Execution: Use multithreading or async execution to keep your UI responsive while handling AI tasks.
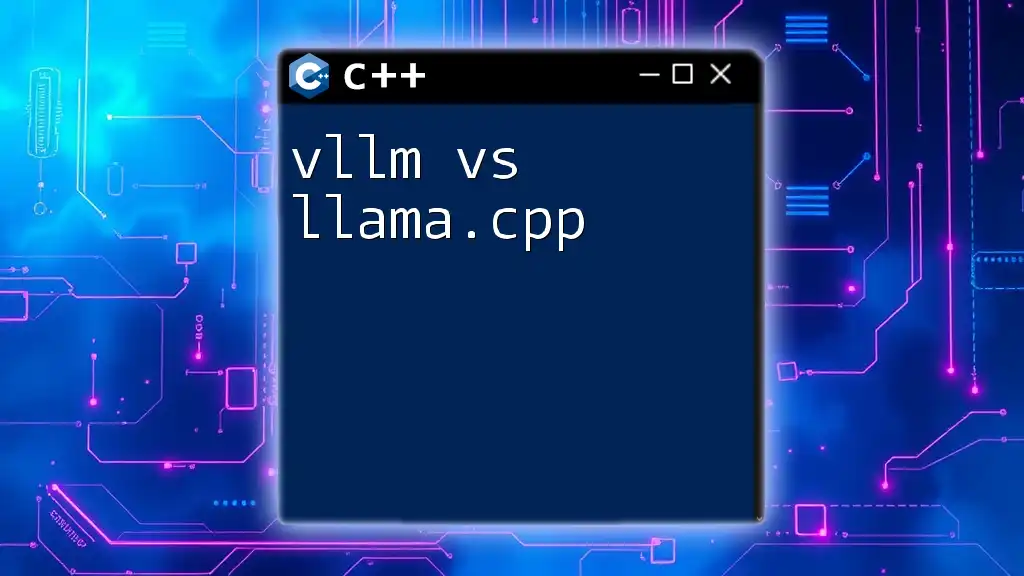
Debugging Common Issues
Identifying Common Problems
Even experienced developers may encounter issues while using llama.cpp. Some frequent problems include:
- Model not found: Ensure the path specified in the initialization command is correct.
- Memory allocation errors: This often occurs when the system is running out of resources. Optimize your memory usage.
Using Logging and Debugging Tools
Implementing logging can be invaluable for tracking down issues. Consider using libraries like `spdlog` or `glog` to maintain detailed logs of application behavior. This can help you quickly identify and rectify issues as they arise.
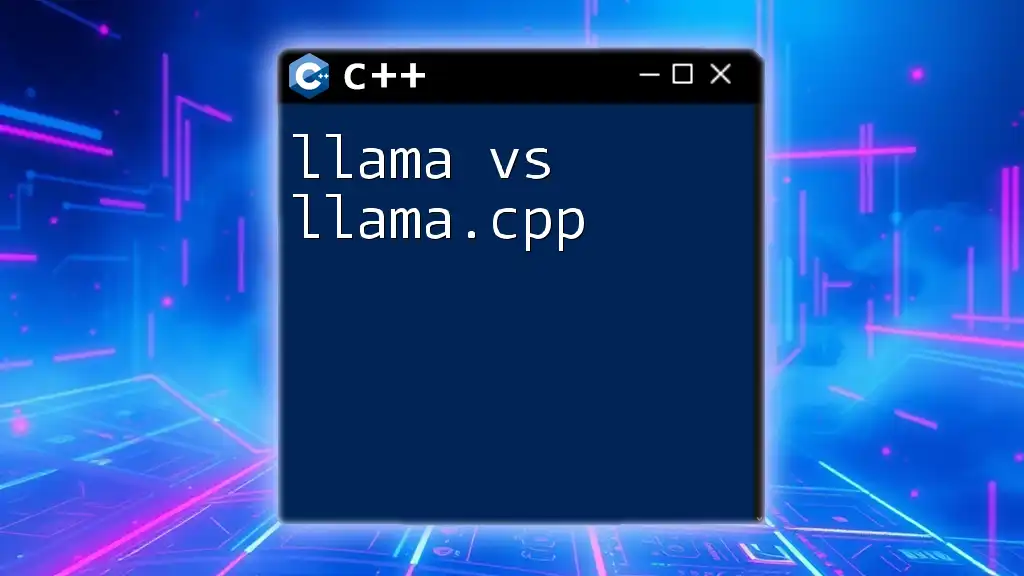
Conclusion
Mastering how to use llama.cpp can significantly enhance your ability to create intelligent applications that leverage AI for text generation and language processing. Experiment with the commands, build your own projects, and don’t hesitate to engage with the community to share your experiences. The future of programming with LLaMA is exciting, and learning llama.cpp will keep you on the cutting edge of technology.
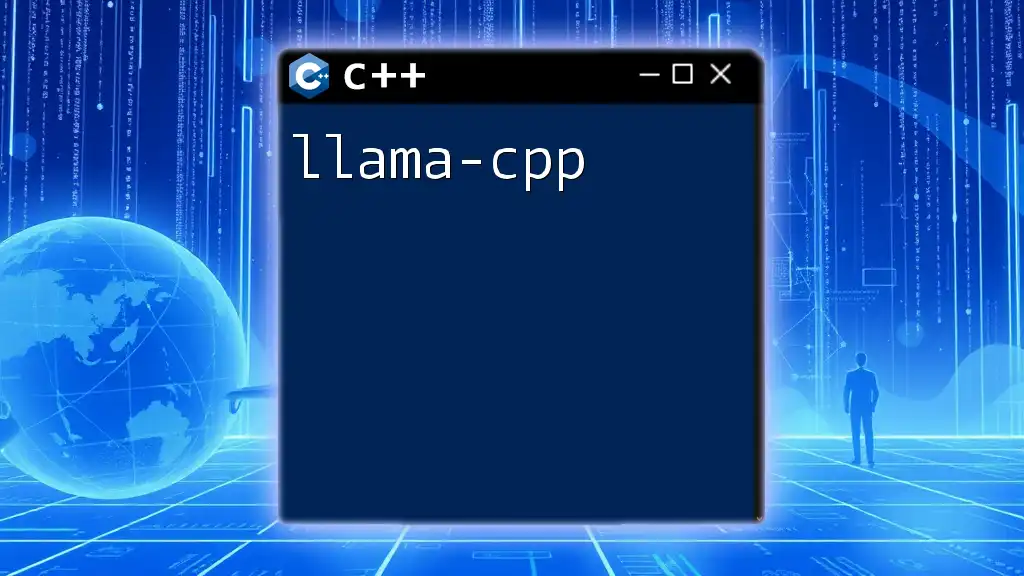
Additional Resources
To deepen your understanding and proficiency with llama.cpp, explore the following resources:
- Official documentation and repositories related to llama.cpp.
- Online forums and communities for discussions on best practices and advanced features.
- Recommended readings on C++ programming and AI applications to complement your learning.
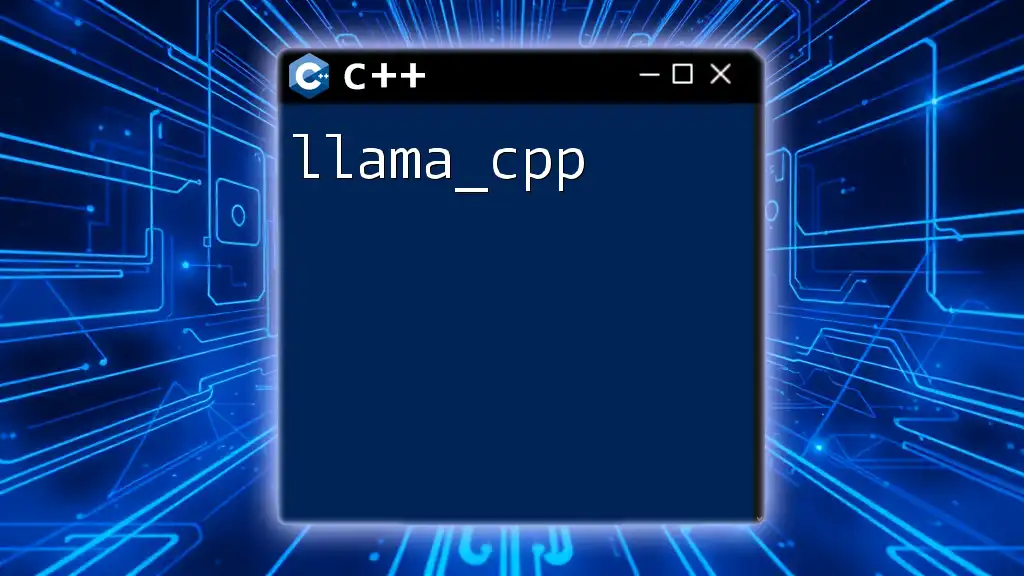
FAQs
What is llama.cpp used for?
Llama.cpp is primarily used for implementing the LLaMA model, allowing developers to generate text and interact with advanced AI capabilities within C++ applications.
Can I modify llama.cpp for personal projects?
Yes, llama.cpp is generally open-source, allowing users to modify it as needed for personal projects, subject to its licensing agreement.
Is there a community for support?
Yes, several forums and chat rooms are dedicated to discussing llama.cpp where you can seek help, share ideas, and learn from other users' experiences.