The term "llama" typically refers to the machine learning model, while "llama.cpp" is an implementation or interface that allows users to interact with or utilize this model through C++ commands.
Here's a simple code snippet illustrating how to include the `llama.cpp` header in your C++ project:
#include "llama.cpp"
// Your code to initialize and use the Llama model goes here
Understanding Llama
What is Llama?
Llama is a framework designed for C++ programming that focuses on providing developers with a straightforward and efficient way to build applications. Its historical roots trace back to earlier C++ frameworks that aimed to abstract complex programming paradigms while retaining the language's performance advantages.
Key Features of Llama
One of the standout features of Llama is its emphasis on performance and speed. By optimizing key processes, Llama allows applications to run faster compared to other frameworks. Additionally, Llama’s object-oriented programming capabilities make it easy for developers to manage large codebases through modular design.
For example, consider a simple application that involves creating animal characters:
class Animal {
public:
virtual void speak() { std::cout << "Generic animal sound"; }
};
This code snippet illustrates how Llama enables developers to define base classes that can be extended, promoting clean architecture and reusability.
Use Cases for Llama
Llama is particularly well-suited for applications that require high-performance computation, such as:
- Game Development: Real-time performance is critical, making Llama a wise choice for game engines.
- Scientific Computing: Applications demanding intense numerical computations benefit from Llama’s optimized processing capabilities.
- Embedded Systems: Lightweight applications that require fast execution can leverage Llama’s strengths effectively.
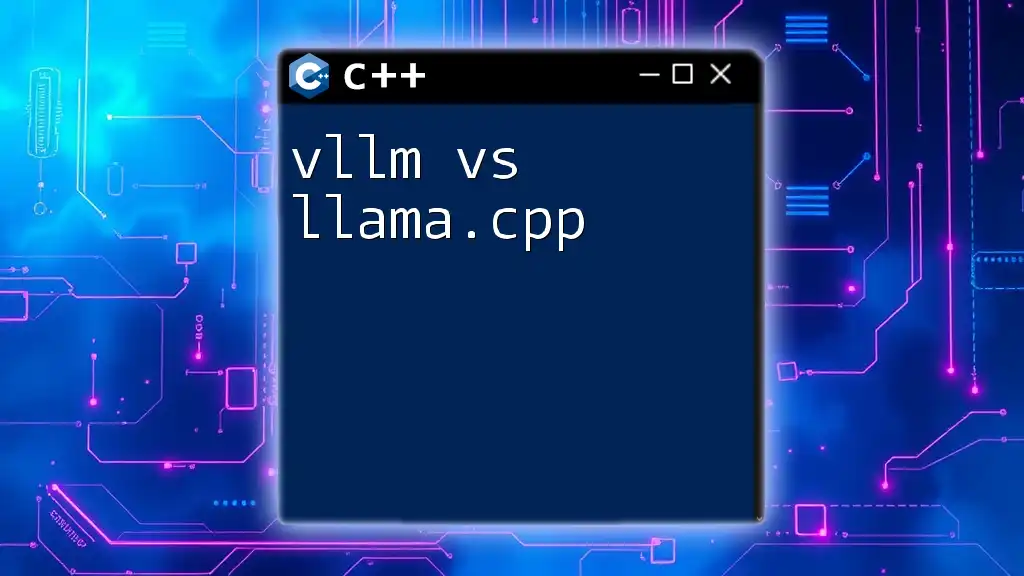
Transitioning to Llama.cpp
What is Llama.cpp?
Llama.cpp is an evolution of the original Llama framework that integrates modern C++ standards. This evolution is not just about aesthetics; it refines the structure and usability of libraries, making them more compatible with contemporary software development practices.
Key Features of Llama.cpp
One of the major enhancements in Llama.cpp is its incorporation of modern C++ standards, which improves safer coding practices and facilitates easier debugging. Llama.cpp also offers enhanced functionality, making it easier for developers to implement features that were cumbersome in the original Llama.
Here’s a simple example that demonstrates a basic "Hello, World!" implementation using Llama.cpp:
#include <iostream>
int main() {
std::cout << "Hello from Llama.cpp!" << std::endl;
return 0;
}
This straightforward example underscores Llama.cpp’s ease of use while still standing on the shoulders of the solid foundation laid by Llama.
When to Use Llama.cpp
Llama.cpp shines in scenarios that take advantage of enhanced performance and modern programming techniques. Some ideal use cases include:
- Large-Scale Applications: Projects that require maintainability and scalability can greatly benefit from Llama.cpp's advanced features.
- Collaborative Development: Teams familiar with modern C++ standards will find Llama.cpp’s structure more approachable and user-friendly.
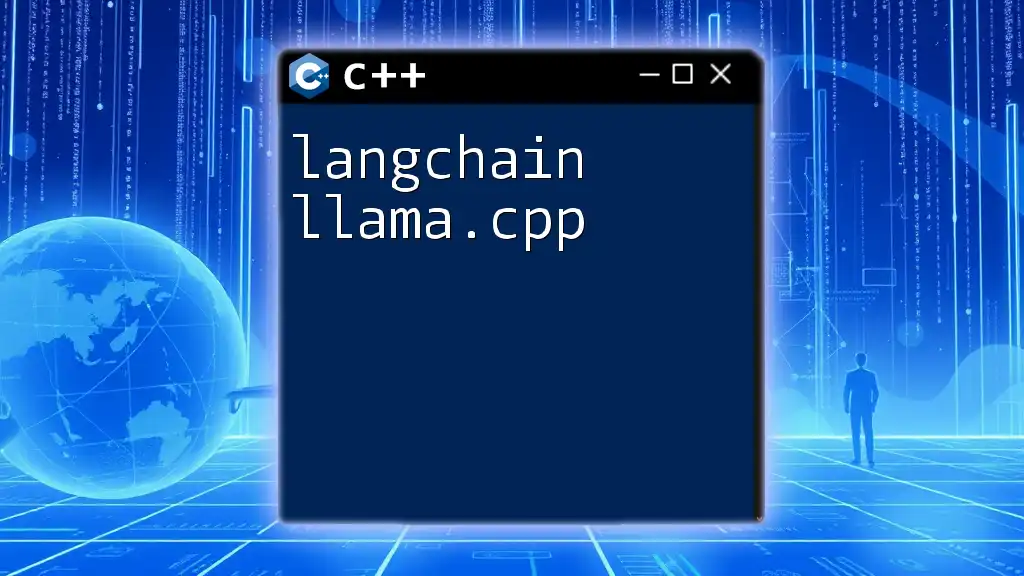
Llama vs Llama.cpp
Structural Differences
The structural differences between Llama and Llama.cpp are significant. For example, while Llama encourages basic class declarations, Llama.cpp introduces refined syntax with an emphasis on const-correctness.
Consider the following comparison of class declarations in both frameworks:
In Llama:
class Animal {
public:
void speak() { std::cout << "Roar"; }
};
In Llama.cpp:
class Animal {
public:
void speak() const { std::cout << "Roar"; }
};
Notice how Llama.cpp enforces const-correctness, which is a crucial feature for ensuring that methods do not unintentionally alter object data.
Performance Comparisons
When it comes to performance, Llama.cpp typically outperforms Llama due to its better optimization and modern techniques. Benchmarks can vary depending on the specific implementations, but generally, Llama.cpp shows improvements in:
- Memory Management: More efficient memory allocation reduces overhead, resulting in faster execution.
- Multithreading Support: Utilizing modern C++ features allows Llama.cpp to manage multiple threads more effectively than Llama.
Community Support and Documentation
The community support surrounding these frameworks differs greatly. Llama, being an older framework, has a smaller community and less extensive documentation. On the other hand, Llama.cpp has a more robust community presence, with plentiful resources for learning, including:
- Online Tutorials: Comprehensive guides that help beginners adapt to Llama.cpp.
- Active Forums: Chances to ask questions and receive rapid solutions from fellow developers.
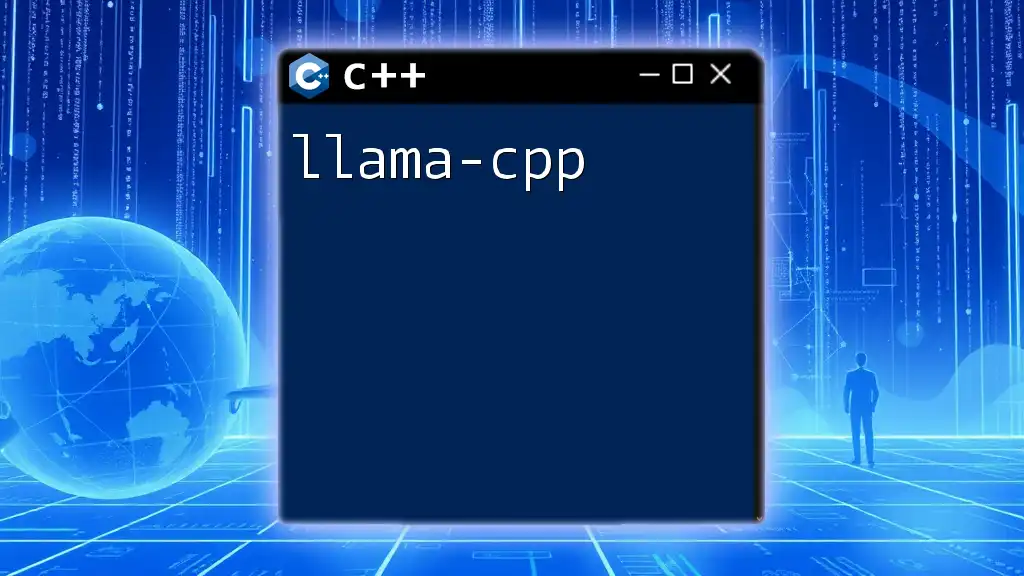
Practical Guidelines for Choosing Between Llama and Llama.cpp
Considerations Before Making a Choice
Choosing between Llama and Llama.cpp involves evaluating various project requirements. Key considerations include:
- Performance Expectations: If performance is paramount, Llama.cpp typically provides a better framework.
- Features Needed: Assess if the additional features available in Llama.cpp align with your project goals.
- Team Expertise: The familiarity of your team with modern C++ standards may guide the selection.
Recommendations for Beginners
For those new to C++, starting with Llama will provide a gentler learning curve due to its simpler paradigms. However, Llama.cpp is the recommended path for long-term projects as it aligns closely with modern development practices. A good starting point for learning would include:
- Online Courses: Many can be found covering Llama.cpp, benefiting from project-based learning.
- Documentation: Utilizing the official documentation for Llama and Llama.cpp will accelerate learning and clear doubts.
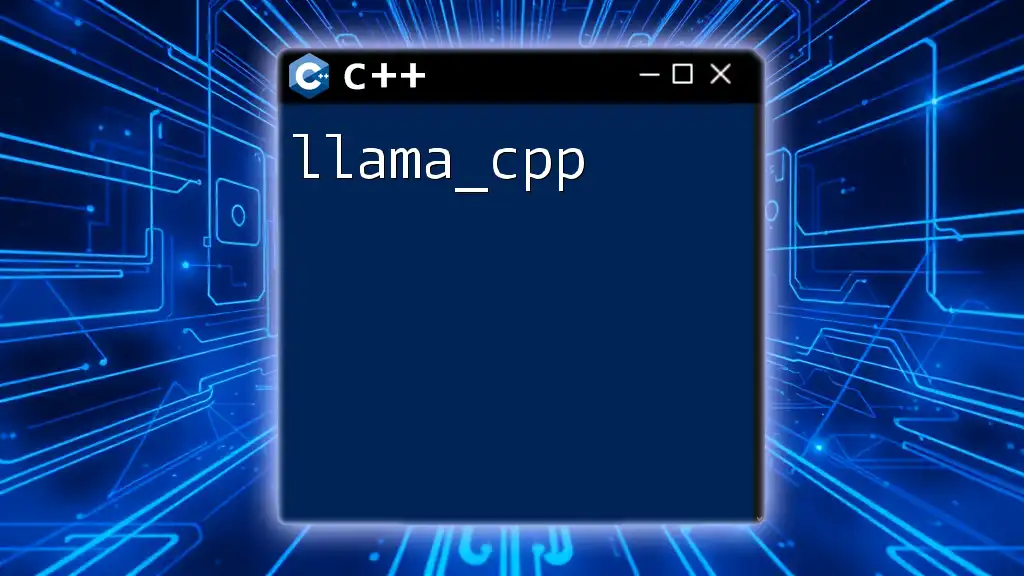
Conclusion
In summary, the comparison of llama vs llama.cpp highlights a notable evolution in programming frameworks. While Llama provides foundational capabilities, Llama.cpp enriches the user experience with modern improvements that facilitate better coding practices and performance outcomes.
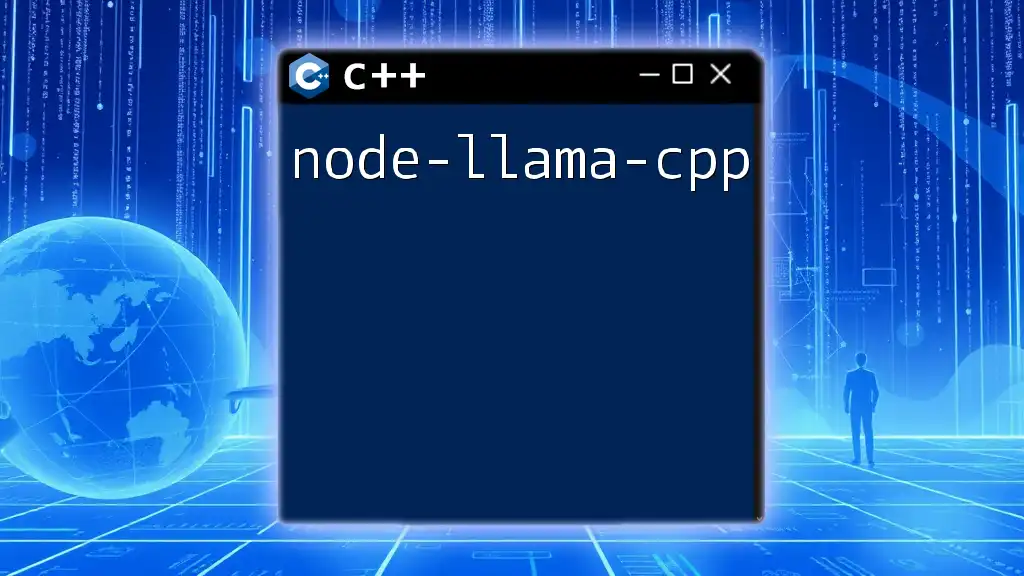
Call to Action
Feel free to leave comments or share your experiences with either framework. We encourage you to share this article with fellow programmers who are interested in learning about C++ commands in a quick and effective manner.